Angular Bootstrap Modals / Angular Bootstrap Popup
Angular Modals - Bootstrap 4 & Material Design
Angular Bootstrap modal is a dialog box/popup window which can be used for lightboxes, user notifications, UI enhancements, e-commerce components and many other cases.
It's easily customized. You can manipulate size, position, and content.
If you want to learn about advanced usage of Modals, read our free tutorial Creating Automated web application .
This documentation presents the basic options and examples of modals. If you need more advanced help have a look at additional pages of the documentation listed below:
Basic example
Below is a most basic Modal example with a button triggering Modal via data attribute.
Click the button to trigger Modal.
Position & Sizes
Click buttons below to launch modals demo
Central Modal
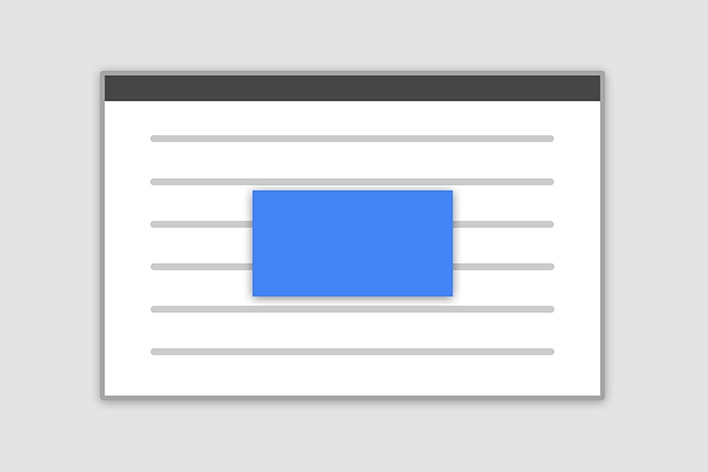
Size
Fluid Modal
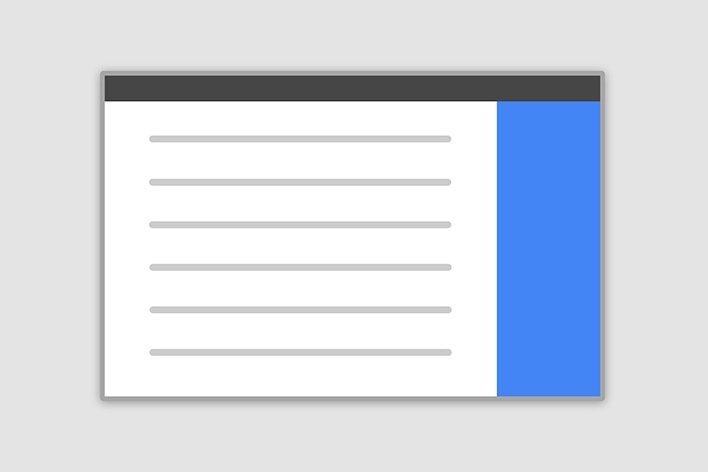
Position
Forms, styles & Predefined templates
Click buttons below to see live previews
Advanced usage
If you want to learn about advanced usage of Modals, read our free tutorial "Creating Automated web application" .
Position and size
To change the position or size of the modal add one of the following classes to the
.modal-dialog
div.
Central modal
Note: Medium size is a default value, so there isn't a dedicated class for it.
.modal-sm
Small Modal
.modal-lg
Large Modal
.modal-fluid
Full Width Modal
Side modal
Note: To make it works properly, apart from adding a class for a position, you also need to add special
class
.side-modal
to
.modal-dialog
div.
Note 2: If you want to change a direction of modal animation, add class
.top
,
.right
,
bottom
or
.left
to the
.modal
div.
.modal-side
+
.modal-top-right
Top Right
.modal-side
+
.modal-top-left
Top Left
.modal-side
+
.modal-bottom-right
Bottom Right
.modal-side
+
.modal-bottom-right
Bottom Left
Fluid modal
Note: As in the previous example - to make it works properly, apart from adding a class for a position,
you also need to add special class
.modal-full-height
to
.modal-dialog
div.
Note 2: If you want to change a direction of modal animation, add class
.top
,
.right
,
bottom
or
.left
to the
.modal
div.
.modal-full-height
+
.modal-right
Right
.modal-full-height
+
.modal-left
Left
.modal-full-height
+
.modal-bottom
Bottom
.modal-full-height
+
.modal-top
Top
Frame modal
Note: As in the previous examples - to make it works properly, apart from adding a class for a position,
you also need to add special class
.modal-frame
to
.modal-dialog
div.
Note 2: If you want to change a direction of modal animation, add class
.top
,
.right
,
bottom
or
.left
to the
.modal
div.
.modal-frame
+
.modal-bottom
Bottom
.modal-frame
+
.modal-top
Top
Scrolling long content
When modal become too long for the user’s viewport or device, it should scroll of the page itself.
To do this, add style="overflow-y: auto"
to div with mdbModal
directive.
Vertically centered
Add
.modal-dialog-centered
to
.modal-dialog
to vertically center the modal.
Tooltips and popovers
Tooltips and popovers can be placed within modals as needed.
Using the grid
Utilize the Bootstrap grid system within a modal by nesting
.container-fluid
within the
.modal-body
. Then, use the normal grid system classes as you would anywhere else.
Varying modal content
Have a bunch of buttons that all trigger the same modal with slightly different contents?
import { Component, ViewChild } from '@angular/core';
@Component({
selector: 'modal-content-example',
templateUrl: 'modal-content.html',
})
export class ModalContentComponent {
@ViewChild('content') public contentModal;
public name: string;
show(value:string){
this.name = value;
this.contentModal.show();
}
}
Remove animation
For modals that simply appear rather than fade into view, remove the
.fade
class from your modal markup.
Accessibility
Be sure to add
role="dialog"
and
aria-labelledby="..."
, referencing the modal title, to
.modal
, and
role="document"
to the
.modal-dialog
itself. Additionally, you may give a description of your modal dialog with aria-describedby on
.modal
.
Embedding YouTube & Vimeo videos
Have a look at this examples to see how it works:
Remove backdrop
To remove
backdrop add
[config]="{backdrop: false, ignoreBackdropClick: true}"
attribute to the modal markup
Using show and hide functions in typescript file
Use Angular @ViewChild
decorator to get access to modal functions in your typescript file. In following example you can click on button to open modal and it will hide automatically after 3 seconds.
import { Component, ViewChild } from '@angular/core';
import { ModalDirective } from 'your path to modal directive';
@Component({
selector: 'show-and-hide-modal-example',
templateUrl: './show-and-hide-modal.component.html',
})
export class ShowAndHideModalComponent {
@ViewChild('demoBasic') demoBasic: ModalDirective;
showAndHideModal() {
this.demoBasic.show();
setTimeout(() => {
this.demoBasic.hide();
}, 3000);
}
}