Free UI/UX design course
Learn how to create exceptional designs by reading my new tutorial.
Start learningSass / scss
You've probably noticed that I like to use the phrase "something on steroids" for a tool that significantly improves on some other tool.
This time will be no exception - you can think of sass as CSS on steroids! 💪
Sass provides a lot of functionality that good old CSS lacks so much. I mean things like variables, nesting, partials, mixins, extend/inheritance or operators.
Thanks to sass, CSS becomes closer to a real programming language.
As I mentioned before, t's hard to imagine a serious web project without using sass. Therefore, let's not wait any longer and learn the basics of this extremely useful tool.
But first, let's clear up one more thing. As you may have noticed, there are two names in the title of this lesson - sass and scss. What is the difference between them?
In short, they are the same thing, but they use different syntax.
In sass, for example, we don't use curly braces and semicolons.
The scss syntax, on the other hand, is much more similar to regular CSS, which I find makes it easier to use. Therefore, in this tutorial we will use scss and this name will be used.
How to use scss in MDB?
The scss code is not supported by browsers, so to be able to use scss you need a preprocessor, i.e. a program that will convert your scss code to plain CSS, readable by all browsers.
The vite starter gives you a preprocessor and the ability to use scss in MDB projects.
Let's see if it works.
Step 1: Open the file src/scss/style.scss
. You will find one line of code there, which is an import of MDB styles:
@import 'mdb-ui-kit/css/mdb.min.css';
Step 2: Above this import put the following scss code:
$color: red;
body {
background-color: $color;
}
@import 'mdb-ui-kit/css/mdb.min.css';
Note that this is not CSS code (we will learn later what exactly it is and what id does). If you put it in a normal CSS file, it wouldn't work. You can test if you want.
However, since you are working on an scss file, and thanks to the Vite starter, there is a preprocessor in the background that will take this code and convert it to plain CSS. When you save your changes, you'll see that the code worked and your live preview background turned red!
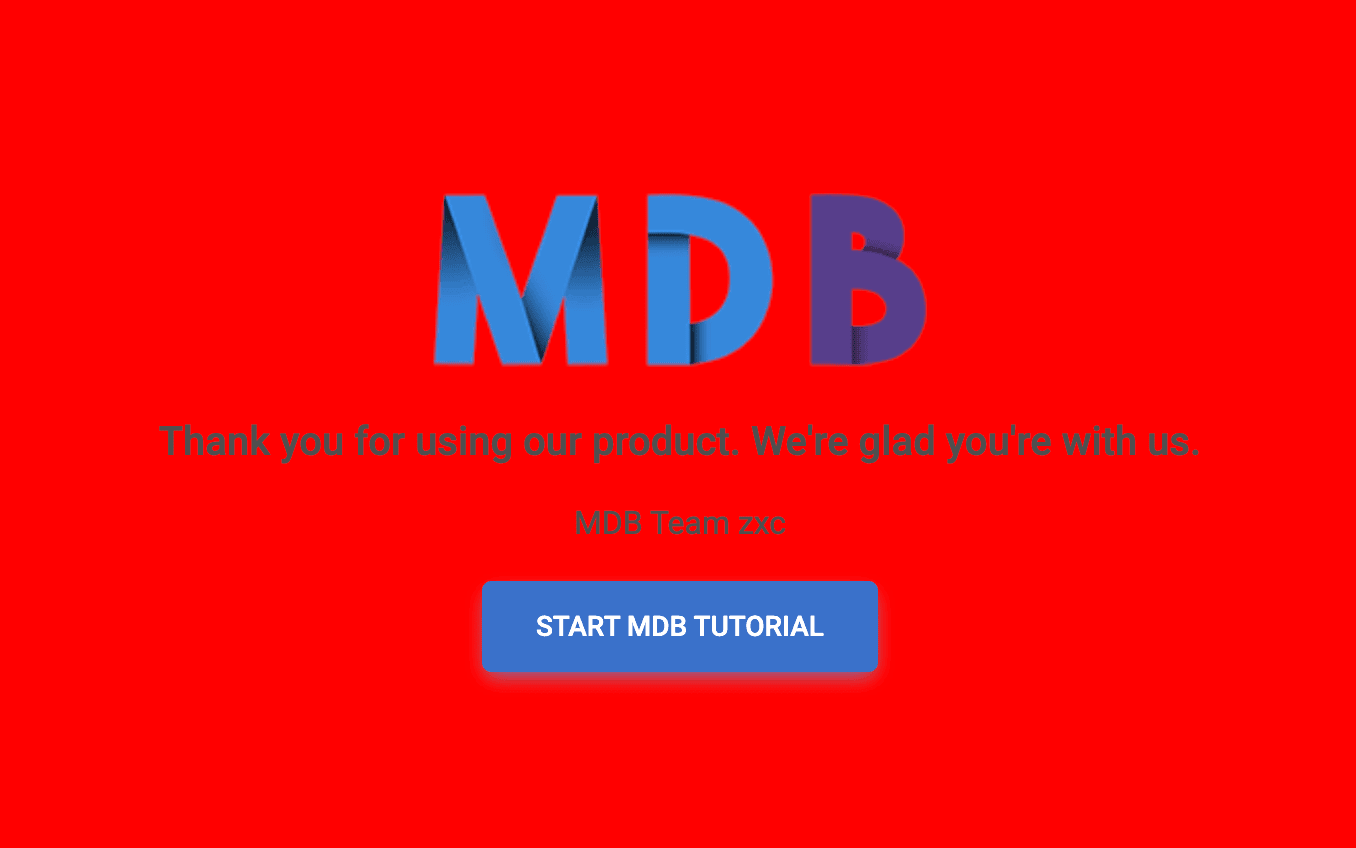
Now that we know that everything works as it should, let's learn the basics of scss. You can (and should) test all the examples below as I showed you above - by pasting them into the src/scss/style.scss
file above the MDB style import.
Variables
A variable is simply a way to store some information that we can then reuse in many places in our code, instead of repeating ourselves meaninglessly.
Variables are such a basic thing that I could never understand why they weren't introduced in CSS from the beginning. In the latest versions, we can fortunately use variables directly in CSS, but older browsers do not support it.
In scss, we can use variables without fear, because they will be recompiled to plain CSS code, supported by every browser.
Scss uses the $
symbol to make something a variable. Have a look at the example below:
This is how we use variables in scss:
$color-primary: #3B71CA;
.btn-primary {
background-color: $color-primary;
}
And this is what the final CSS recompiled from the scss code will look like:
.btn-primary {
background-color: #3B71CA;
}
When the scss code is compiled, the preprocessor takes the value of the #color-primary
variable (which is #3B71CA
) and set it into the background-color
CSS property of the .btn-primary
class.
The result is plain CSS at the end, where the .btn-primary
class has the property background-color
: #3B71CA;
This is an extremely useful tool, especially when creating brand colors. Thanks to variables, we can maintain consistency throughout the project, not having to worry that when we want to change the color of our brand, we will have to make corrections in dozens of places. All we have to do is do it in one place - in the scss variable.
Nesting
This is probably my favorite scss feature.
Note that when you write HTML code, there is a visible hierarchy in it - elements can be nested one into another, which allows you to keep order and harmony in the code.
In CSS, unfortunately, we cannot do this, because nesting is not possible.
But in scss it is! Thanks to this, we can do miracles like in the example below:
This is how we use nesting in scss:
nav {
ul {
margin: 0;
padding: 0;
list-style: none;
}
li { display: inline-block; }
a {
display: block;
padding: 6px 12px;
text-decoration: none;
}
}
CSS output:
nav ul {
margin: 0;
padding: 0;
list-style: none;
}
nav li {
display: inline-block;
}
nav a {
display: block;
padding: 6px 12px;
text-decoration: none;
}
Modules
Another extremely useful thing is the ability to split scss into multiple files. Using @use
rule we can load one scss file into another.
Just type @use
+ module name:
This is how we use modules in scss:
// _base.scss file
$font-stack: Helvetica, sans-serif;
$primary-color: #333;
body {
font: 100% $font-stack;
color: $primary-color;
}
// styles.scss file
@use 'base';
.inverse {
background-color: base.$primary-color;
color: white;
}
CSS output:
body {
font: 100% Helvetica, sans-serif;
color: #333;
}
.inverse {
background-color: #333;
color: white;
}
Mixins
Mixins allow you to group CSS declarations and reuse them later in multiple places in your project. This helps keep your code much more organized.
To make a mixin, you start by using the @mixin
directive and give it a label. In this example, the mixin is named theme
. Within the parentheses, we use the variable $theme
to allow us to pass in any desired theme. Once the mixin has been created, you can utilize it in your CSS code by using the @include
directive followed by the mixin's name.
This is how we use mixins in scss:
@mixin theme($theme: DarkGray) {
background: $theme;
box-shadow: 0 0 1px rgba($theme, .25);
color: #fff;
}
.info {
@include theme;
}
.alert {
@include theme($theme: DarkRed);
}
.success {
@include theme($theme: DarkGreen);
}
CSS output:
.info {
background: DarkGray;
box-shadow: 0 0 1px rgba(169, 169, 169, 0.25);
color: #fff;
}
.alert {
background: DarkRed;
box-shadow: 0 0 1px rgba(139, 0, 0, 0.25);
color: #fff;
}
.success {
background: DarkGreen;
box-shadow: 0 0 1px rgba(0, 100, 0, 0.25);
color: #fff;
}
Extend/Inheritance
The @extend
function allows you to transfer a set of CSS attributes from one selector to another. In this example, we are creating a simple message system for errors, warnings, and successes by utilizing placeholder classes, which work in tandem with extend. Placeholder classes are a unique type of class that only appear when they are extended and help maintain organized and tidy CSS code.
This is how we use extend in scss:
/* This CSS will print because %message-shared is extended. */
.message-shared {
border: 1px solid #ccc;
padding: 10px;
color: #333;
}
// This CSS won't print because %equal-heights is never extended.
.equal-heights {
display: flex;
flex-wrap: wrap;
}
.message {
@extend .message-shared;
}
.success {
@extend .message-shared;
border-color: green;
}
.error {
@extend .message-shared;
border-color: red;
}
.warning {
@extend .message-shared;
border-color: yellow;
}
CSS output:
/* This CSS will print because .message-shared is extended. */
.message, .success, .error, .warning {
border: 1px solid #ccc;
padding: 10px;
color: #333;
}
.success {
border-color: green;
}
.error {
border-color: red;
}
.warning {
border-color: yellow;
}
The code above specifies that .message
, .success
, .error
, and .warning
should function in the same manner as .message-shared
. This means that wherever message-shared
is used, .message
, .success
, .error
, and .warning
will also be present. This results in the generated CSS, where all of these classes will possess the same CSS properties as .message-shared
, allowing you to avoid writing multiple class names in HTML elements.
In scss, you can extend most basic CSS selectors in addition to placeholder classes, but using placeholders is the simplest method to ensure that you're not extending a nested class, which can cause unintended selectors in your CSS.
It's important to note that the CSS in .equal-heights
wasn't generated because .equal-heights
was never extended.
Operators
Performing calculations in CSS can be very useful. Scss offers a variety of standard mathematical operators like +
, -
, *
, math.div()
, and %
. In the example below, we'll do some basic math to calculate the widths for an article and aside.
This is how we use operators in scss:
.container {
display: flex;
}
article[role="main"] {
width: math.div(600px, 960px) * 100%;
}
aside[role="complementary"] {
width: math.div(300px, 960px) * 100%;
margin-left: auto;
}
CSS output:
.container {
display: flex;
}
article[role="main"] {
width: 62.5%;
}
aside[role="complementary"] {
width: 31.25%;
margin-left: auto;
}
These are the most important sass/scss features. If you want to learn more, I recommend you the official guide on which this lesson is based.
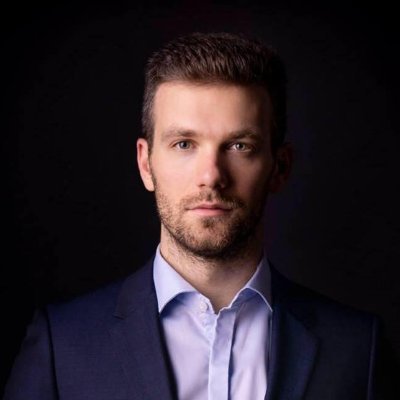
About author
Michal Szymanski
Co Founder at MDBootstrap / Listed in Forbes „30 under 30" / Open-source enthusiast / Dancer, nerd & book lover.
Author of hundreds of articles on programming, business, marketing and productivity. In the past, an educator working with troubled youth in orphanages and correctional facilities.