Angular project structure
Angular project structure tutorial
Learn how to create project structure in Angular.
Explanation
This is what our project tree looks like:
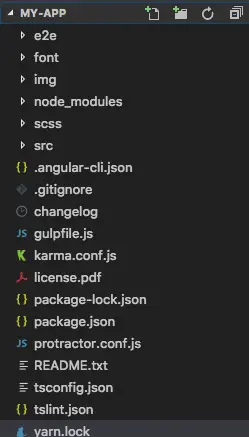
Let's have a look at it step by step:
/e2e/
directory:/node_modules/
/src/
/app/
/asset/
favicon.ico
index.html
main.ts
polyfils.ts
styles.css
test.ts
.angular-cli.json
.gitignore
karma.conf.js
package.json
protractor.config.js
README.md
tsconfig.json
tslint.json
├── e2e
│ ├── app.e2e-spec.ts
│ ├── app.po.ts
│ └── tsconfig.e2e.json
e2e stands for end-to-end and it refers to e2e tests of the website. It contains testing scenarios (scripts) which simulate user behavior. We can simulate a user who visits a website, signs in, navigates to another site, fills the form and logs out.
All 3rd party libraries are installed into this folder when you run npm install
.
Those libraries are bundled into our application. What is important to know is that
you shouldn't include this folder when deploying your application to production
or committing to the git repository. If you move your project to a new location
you should skip this folder and run npm install
in a new location.
├── src
│ ├── app
│ ├── environments
│ ├── favicon.ico
│ ├── index.html
│ ├── main.ts
│ ├── polyfills.ts
│ ├── styles.scss
│ ├── test.ts
│ ├── tsconfig.app.json
│ ├── tsconfig.spec.json
│ └── typings.d.ts
This is where we will keep our application source code.
│ ├── app
│ │ ├── app.component.css
│ │ ├── app.component.html
│ │ ├── app.component.spec.ts
│ │ ├── app.component.ts
│ │ ├── app.module.ts
│ │ └── typescripts
This folder contains our modules and components. Remember: Each Angular application must have at least one module and one component.
This folder contains two files, each for different environments. You will use this file to store environment specific configuration like database credentials or server addresses. By default there are two files, one for production and on for development.
The favicon of our app.
This is is a very simple HTML file. If you open it you will note that there are no references to any stylesheet (CSS) nor JS files. This is because all dependencies are injected during the build process.
This is the starting point for our app. If you ever coded in languages like Java or C you can compare it to a main() method. If you haven't, then just remember that our application starts to execute from this place. This is where we are bootstrapping our first and only module — AppModule.
Polyfils files are used for the compiler to compile our Typescript to specific JavaScript methods which can be parsed by different browsers.
This is global stylesheet file that is by default, included to our project. Keep in mind that each component has its own style component which applies styles only within the scope of given component.
This is a configuration file for Karma. Karma is a testing tool shipped along with Angular, we will cover it one of the later lessons.
This is an important file. It contains the configuration for CLI which defines which 3rd party script or styles should be included, which files should be served as static assets, where compiled code should be placed and much more. We will cover it in later lessons.
This file instructs git which files should be ignored when working with git repository.
Another configuration file for Karma. We will cover that in the future.
This file is mandatory for every npm project. It contains basic information regarding our project (name, description, license etc.) commands which can be used, dependencies — those are packages required by our application to work correctly, and devDepndencies — again a list of packages which are required for our application however only during the development phase. I.e. we need Angular CLI only during development to build a final package however on production we don't need it anymore.
File containing a description of our project. All the information which we would like to provide our userswith before they start using our app.
A bunch of compiler settings. Based on these settings, it will compile code to JS code which that browser can understand.
TSlint is a useful static analysis tool that checks our TypeScript code for readability, maintainability, and functionality errors. This file contains its configuration. We will see how it works in a future lesson.