Chips
Angular Bootstrap 5 Chips component
Responsive chips built with the latest Bootstrap 5, Angular and Material Design. Chips (aka tags) make it easier to categorize content and browse ie. through different articles from the same category.
Bootstrap tags and chips categorize content with the use of text and icons. Tags and chips make it easier to browse throughout articles, comments or pages. Their main goal is to provide your visitors with an intuitive way of getting what they want. Just consider, how convenient it is to find all the articles related to web development just by using a tag.
Note: Read the API tab to find all available options and advanced customization
Basic example
Chips can be used to represent small blocks of information. They are most commonly used either for contacts or for tags.
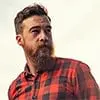
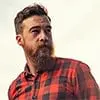
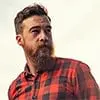
<mdb-chip>Text</mdb-chip>
<mdb-chip>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="Contact Person"
/>
John Doe
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-md">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="Contact Person"
/>
John Doe
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-lg">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="Contact Person"
/>
John Doe
<i class="close fas fa-times"></i>
</mdb-chip>
Outline
You can use customClass
input and btn-outline-color
classes to add outline styling to your chip.
<mdb-chip customClass="chip-outline btn-outline-primary">
Primary
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-secondary">
Secondary
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-success">
Success
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-danger">
Danger
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-warning">
Warnign
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-info">
Info
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-light">
Light
<i class="close fas fa-times"></i>
</mdb-chip>
<mdb-chip customClass="chip-outline btn-outline-dark">
Dark
<i class="close fas fa-times"></i>
</mdb-chip>
Placeholder
Type a name and press enter to add a tag. Click X to remove it.
<mdb-chips>
<mdb-form-control
class="chips-input-wrapper chips-transition"
[class.chips-padding]="tags.length > 0"
>
<mdb-chip *ngFor="let tag of tags" (deleted)="delete(tag)">
<span class="text-chip">{{ tag.name }}</span>
<i class="close fas fa-times" mdbChipDelete></i>
</mdb-chip>
<input
mdbChipsInput
class="form-control chips-input"
(chipAdd)="add($event)"
/>
<label mdbLabel class="form-label">Example label</label>
</mdb-form-control>
</mdb-chips>
import { Component } from '@angular/core';
import { MdbChipAddEvent } from 'mdb-angular-ui-kit/chips';
interface Tag {
name: string;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
tags: Tag[] = [];
add(event: MdbChipAddEvent) {
this.tags.push({ name: event.value });
event.input.clear();
}
delete(tag: Tag) {
const index = this.tags.indexOf(tag);
if (index >= 0) {
this.tags.splice(index, 1);
}
}
}
Initial Value
You can set initial tags by adding them to the tags array.
<mdb-chips class="chips-initial">
<mdb-form-control
class="chips-input-wrapper chips-transition"
[class.chips-padding]="tags.length > 0"
>
<mdb-chip *ngFor="let tag of tags" (deleted)="delete(tag)">
<span class="text-chip">{{ tag.name }}</span>
<i class="close fas fa-times" mdbChipDelete></i>
</mdb-chip>
<input mdbChipsInput class="form-control chips-input" (chipAdd)="add($event)" />
<label mdbLabel class="form-label">Example label</label>
</mdb-form-control>
</mdb-chips>
import { Component } from '@angular/core';
import { MdbChipAddEvent } from 'mdb-angular-ui-kit/chips';
interface Tag {
name: string;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
tags: Tag[] = [
{ name: 'MDBReact' },
{ name: 'MDBAngular' },
{ name: 'MDBVue' },
{ name: 'MDB5' },
{ name: 'MDB' },
];
add(event: MdbChipAddEvent) {
this.tags.push({ name: event.value });
event.input.clear();
}
delete(tag: Tag) {
const index = this.tags.indexOf(tag);
if (index >= 0) {
this.tags.splice(index, 1);
}
}
}
Content Editable
You can use [editable]="true"
input to make a chips content editable.
<mdb-chips class="chips-initial">
<mdb-form-control
class="chips-input-wrapper chips-transition"
[class.chips-padding]="tags.length > 0"
>
<mdb-chip
*ngFor="let tag of tags"
[editable]="true"
(deleted)="delete(tag)"
(edited)="edit(tag, $event)"
>
<span class="text-chip">{{ tag.name }}</span>
<i class="close fas fa-times" mdbChipDelete></i>
</mdb-chip>
<input
mdbChipsInput
class="form-control chips-input"
(chipAdd)="add($event)"
/>
<label mdbLabel class="form-label">Example label</label>
</mdb-form-control>
</mdb-chips>
import { Component } from '@angular/core';
import { MdbChipAddEvent } from 'mdb-angular-ui-kit/chips';
interface Tag {
name: string;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
tags: Tag[] = [];
add(event: MdbChipAddEvent) {
this.tags.push({ name: event.value });
event.input.clear();
}
delete(tag: Tag) {
const index = this.tags.indexOf(tag);
if (index >= 0) {
this.tags.splice(index, 1);
}
}
edit(tag: Tag, event: MdbChipEditedEvent) {
if (!event.value) {
this.delete(tag);
return;
}
const index = this.tags.indexOf(tag);
if (index >= 0) {
this.tags[index].name = value;
}
}
}
Chips - API
Import
import { MdbChipsModule } from 'mdb-angular-ui-kit/chips';
import { MdbFormsModule } from 'mdb-angular-ui-kit/forms';
…
@NgModule ({
...
imports: [MdbChipsModule, MdbFormsModule],
...
})
Inputs
MdbChipComponent
Name | Type | Default | Description |
---|---|---|---|
customClass |
string | - |
Allows to set custom classes for a chip |
editable |
boolean | false |
Allows to edit chip content. |
Outputs
MdbChipInputDirective
Name | Type | Description |
---|---|---|
chipAdd |
EventEmitter<MdbChipAddEvent> | Fired on chip add when confirmation key is clicked. |
MdbChipComponent
Name | Type | Description |
---|---|---|
deleted |
EventEmitter<void> | Fired when a chip is deleted. |
edited |
EventEmitter<MdbChipEditedEvent> | Fired after a completed chip edition. |
<mdb-chips>
<mdb-form-control
class="chips-input-wrapper chips-transition"
[class.chips-padding]="tags.length > 0"
>
<mdb-chip *ngFor="let tag of tags" (deleted)="delete(tag)">
<span class="text-chip">{{ tag.name }}</span>
<i class="close fas fa-times" mdbChipDelete></i>
</mdb-chip>
<input
mdbChipsInput
class="form-control chips-input"
(chipAdd)="add($event)"
/>
<label mdbLabel class="form-label">Example label</label>
</mdb-form-control>
</mdb-chips>
import { Component } from '@angular/core';
import { MdbChipAddEvent } from 'mdb-angular-ui-kit/chips';
interface Tag {
name: string;
}
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
tags: Tag[] = [];
add(event: MdbChipAddEvent) {
this.tags.push({ name: event.value });
event.input.clear();
}
delete(tag: Tag) {
const index = this.tags.indexOf(tag);
if (index >= 0) {
this.tags.splice(index, 1);
}
}
}
Advanced types
MdbChipAddEvent
interface MdbChipAddEvent {
value: string;
input: MdbChipsInputDirective;
}
MdbChipEditedEvent
interface MdbChipEditedEvent {
chip: MdbChipComponent;
value: string;
}
CSS variables
As part of MDB’s evolving CSS variables approach, chips now uses local CSS variables on
.chip
and .chips
for enhanced real-time customization. Values for
the CSS variables are set via Sass, so Sass customization is still supported, too.
// .chip
--#{$prefix}chip-height: #{$chip-height};
--#{$prefix}chip-line-height: #{$chip-line-height};
--#{$prefix}chip-padding-right: #{$chip-padding-right};
--#{$prefix}chip-margin-y: #{$chip-margin-y};
--#{$prefix}chip-margin-right: #{$chip-margin-right};
--#{$prefix}chip-font-size: #{$chip-font-size};
--#{$prefix}chip-font-weight: #{$chip-font-weight};
--#{$prefix}chip-font-color: #{$chip-font-color};
--#{$prefix}chip-bg: #{$chip-bg};
--#{$prefix}chip-border-radius: #{$chip-br};
--#{$prefix}chip-transition-opacity: #{$chip-transition-opacity};
--#{$prefix}chip-img-margin-right: #{$chip-img-margin-right};
--#{$prefix}chip-img-margin-left: #{$chip-img-margin-left};
--#{$prefix}chip-close-padding-left: #{$chip-close-padding-left};
--#{$prefix}chip-close-font-size: #{$chip-close-font-size};
--#{$prefix}chip-close-opacity: #{$chip-close-opacity};
--#{$prefix}chip-outline-border-width: #{$chip-outline-border-width};
--#{$prefix}chip-md-height: #{$chip-md-height};
--#{$prefix}chip-md-br: #{$chip-md-br};
--#{$prefix}chip-lg-height: #{$chip-lg-height};
--#{$prefix}chip-lg-br: #{$chip-lg-br};
--#{$prefix}chip-contenteditable-border-width: #{$chip-contenteditable-border-width};
--#{$prefix}chip-contenteditable-border-color: #{$chip-contenteditable-border-color};
--#{$prefix}chip-icon-color: #{$chip-icon-color};
--#{$prefix}chip-icon-transition: #{$chip-icon-transition};
--#{$prefix}chip-icon-hover-color: #{$chip-icon-hover-color};
// .chips
--#{$prefix}chips-min-height: #{$chips-min-height};
--#{$prefix}chips-padding-bottom: #{$chips-padding-bottom};
--#{$prefix}chips-margin-bottom: #{$chips-margin-bottom};
--#{$prefix}chips-transition: #{$chips-transition};
--#{$prefix}chips-padding-padding: #{$chips-padding-padding};
--#{$prefix}chips-input-width: #{$chips-input-width};
SCSS variables
$chip-height: 32px;
$chip-md-height: 42px;
$chip-lg-height: 52px;
$chip-font-size: 13px;
$chip-font-weight: 400;
$chip-font-color: $body-color;
$chip-line-height: 2;
$chip-padding-right: 12px;
$chip-br: 16px;
$chip-md-br: 21px;
$chip-lg-br: 26px;
$chip-bg: #eceff1;
$chip-margin-y: 5px;
$chip-margin-right: 1rem;
$chip-transition-opacity: 0.3s linear;
$chip-img-margin-right: 8px;
$chip-img-margin-left: -12px;
$chip-icon-color: #afafaf;
$chip-icon-hover-color: #8b8b8b;
$chip-icon-transition: 0.2s ease-in-out;
$chip-outline-border-width: 1px;
$chip-close-font-size: 16px;
$chip-close-line-height: $chip-height;
$chip-close-padding-left: 8px;
$chip-close-opacity: 0.53;
$chip-contenteditable-border-width: 3px;
$chip-contenteditable-border-color: #b2b3b4;
$chips-margin-bottom: 30px;
$chips-min-height: 45px;
$chips-padding-bottom: 1rem;
$chips-input-font-color: $body-color;
$chips-input-font-size: 13px;
$chips-input-font-weight: 500;
$chips-input-height: $chip-height;
$chips-input-margin-right: 20px;
$chips-input-line-height: $chip-height;
$chips-input-width: 150px;
$chips-transition: 0.3s ease;
$chips-focus-box-shadow: 0.3s ease;
$chips-padding-padding: 5px;