Sticky
Angular Bootstrap 5 Sticky
Sticky is a component which allows elements to be locked in a particular area of the page. It is often used in navigation menus.
Note: Read the API tab to find all available options and advanced customization
Basic example
To start using sticky just use a mdbSticky
directive on the element you want to pin
<div style="min-height: 500px" class="text-center" #boundary>
<a
id="sticky"
type="button"
href="https://mdbootstrap.com/docs/angular/getting-started/installation/"
class="btn btn-outline-primary bg-white sticky"
mdbSticky
[stickyBoundary]="boundary"
>Download MDB
</a>
</div>
Sticky bottom
You can pin element to bottom by adding
[stickyPosition]="'bottom'"
<div style="min-height: 500px" class="text-center" #boundary>
<img
id="MDB-logo"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
mdbSticky
[stickyBoundary]="boundary"
[stickyPosition]="'bottom'"
[stickyDirection]="'both'"
/>
</div>
Boundary
Set [stickyBoundary]="boundary"
so that sticky only works inside the parent
element. Remember to set the correct parent height.
<div style="min-height: 500px" class="text-center" #boundary>
<img
id="MDB-logo"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
mdbSticky
[stickyBoundary]="boundary"
[stickyDirection]="'down'"
/>
</div>
Outer element as a boundary
You can specify an element to be the sticky boundary.
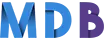
<div
style="min-height: 500px"
class="d-flex flex-column justify-content-center text-center"
>
<div>
<img
id="MDB-logo"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
class="sticky"
mdbSticky
[stickyBoundary]="boundary"
[stickyDirection]="'both'"
/>
</div>
<div #boundary class="mt-auto" style="height: 5rem">Stop here</div>
</div>
Direction
Default direction of sticky component is down
. You can change it by setting
data-mdb-sticky-direction="up"
or
data-mdb-sticky-direction="both"
<div style="min-height: 500px" class="text-center" #boundary>
<a
type="button"
class="btn btn-primary sticky"
mdbSticky
[stickyBoundary]="boundary"
[stickyDirection]="'up'"
>
Up
</a>
<a
type="button"
class="btn btn-primary sticky"
mdbSticky
[stickyBoundary]="boundary"
[stickyDirection]="'down'"
>
Down
</a>
<a
type="button"
class="btn btn-primary sticky"
mdbSticky
[stickyBoundary]="boundary"
[stickyDirection]="'both'"
>
Both
</a>
</div>
Sticky - API
Import
import { MdbStickyModule } from 'mdb-angular-ui-kit/sticky';
…
@NgModule ({
...
imports: [MdbStickyModule],
...
})
Inputs
Name | Type | Default | Description |
---|---|---|---|
stickyActiveClass
|
String | '' |
Set custom active class |
stickyBoundary
|
HTMLElement | null |
set the reference of the boundary element |
stickyDelay
|
Number | 0 |
Set the number of pixels beyond which the item will be pinned |
stickyDirection
|
String | 'down' |
Set the scrolling direction for which the element is to be stikcky |
stickyOffset
|
Number | 0 |
Set the distance from the top edge of the element for which the element is sticky |
stickyPosition
|
String | 'top' |
Set the edge of the screen the item should stick to |
Outputs
Name | Type | Description |
---|---|---|
active
|
EventEmitter<void> | This event fires immediately when sticky start |
inactive
|
EventEmitter<void> | This event fires immediately when sticky stop |
<div style="min-height: 500px" class="text-center" #boundary>
<a
id="sticky"
type="button"
href="https://mdbootstrap.com/docs/jquery/getting-started/download/"
class="btn btn-outline-primary bg-white sticky"
mdbSticky
[stickyBoundary]="boundary"
(active)="onActive()"
>Download MDB
</a>
</div>
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
onActive() {
console.log('active');
}
}