Touch
Bootstrap 5 Touch
This directive allows you to improve the user experience on mobile touch screens by calling methods on the following custom events: pinch, swipe, tap, press, pan and rotate.
Note: Read the API tab to find all available options and advanced customization
Note: This method is intended only for users of mobile touch screens, so it won't work for the mouse or keyboard events.
Press
Press calls the chosen method when the touch event on the element lasts longer than 250 miliseconds.
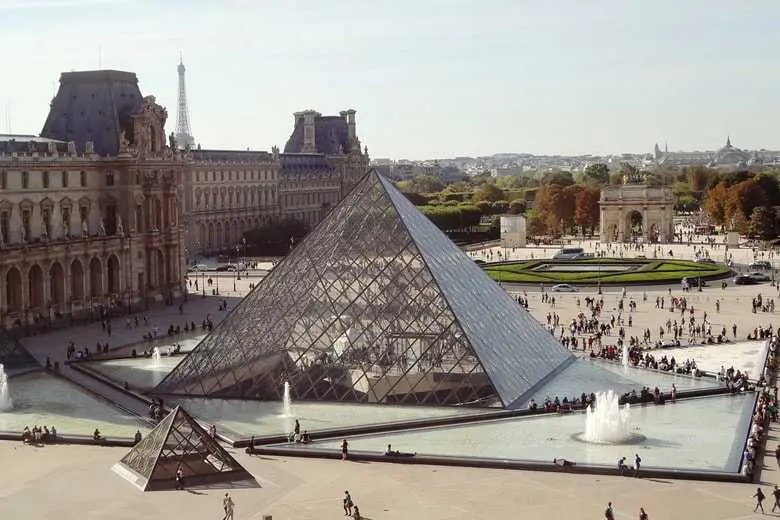
Hold the button to remove the mask from the image
<template>
<div>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
<div
class="mask"
style="background-color: rgba(0, 0, 0, 0.6)"
id="remove-bg"
>
<div
class="d-flex justify-content-center align-items-center h-100"
>
<p class="text-white mb-0">
Hold the button to remove the mask from the image
</p>
</div>
</div>
</div>
<div class="my-3">
<MDBBtn color="primary" v-mdb-touch:press="pressCallback">
Tap & hold to show image
</MDBBtn>
</div>
</div>
</template>
<script>
import { mdbTouch, MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
},
directives: {
mdbTouch
},
setup() {
const pressCallback = () => {
const removeBg = document.getElementById("remove-bg");
removeBg.style.backgroundColor = "rgba(0,0,0,0)";
};
return {
pressCallback
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch, MDBBtn } from "mdb-vue-ui-kit";
const pressCallback = () => {
const removeBg = document.getElementById("remove-bg");
removeBg.style.backgroundColor = "rgba(0,0,0,0)";
};
</script>
Press duration
Touch event press with custom duration.
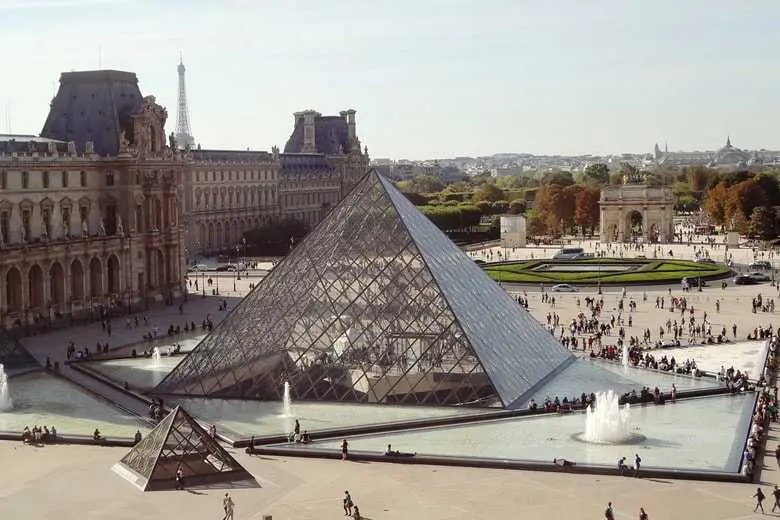
Hold the button to remove the mask from the image with 5s
<template>
<div>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
<div
class="mask"
style="background-color: rgba(0, 0, 0, 0.6)"
id="change-bg"
>
<div
class="d-flex justify-content-center align-items-center h-100"
>
<p class="text-white mb-0">
Hold the button to remove the mask from the image with
5s
</p>
</div>
</div>
</div>
<div class="my-3">
<MDBBtn
color="primary"
v-mdb-touch:press="{
callback: pressDurationCallback,
time: 5000
}"
>
Tap & hold to show image
</MDBBtn>
</div>
</div>
</template>
<script>
import { mdbTouch, MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
},
directives: {
mdbTouch
},
setup() {
const pressDurationCallback = () => {
const changeBg = document.getElementById("change-bg");
changeBg.style.backgroundColor = "rgba(111,33,231,.4)";
};
return {
pressDurationCallback
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch, MDBBtn } from "mdb-vue-ui-kit";
const pressDurationCallback = () => {
const changeBg = document.getElementById("change-bg");
changeBg.style.backgroundColor = "rgba(111,33,231,.4)";
};
</script>
Tap
The callback on tap event is called with an object containing origin field - the x and y cooridinates of the user's touch.
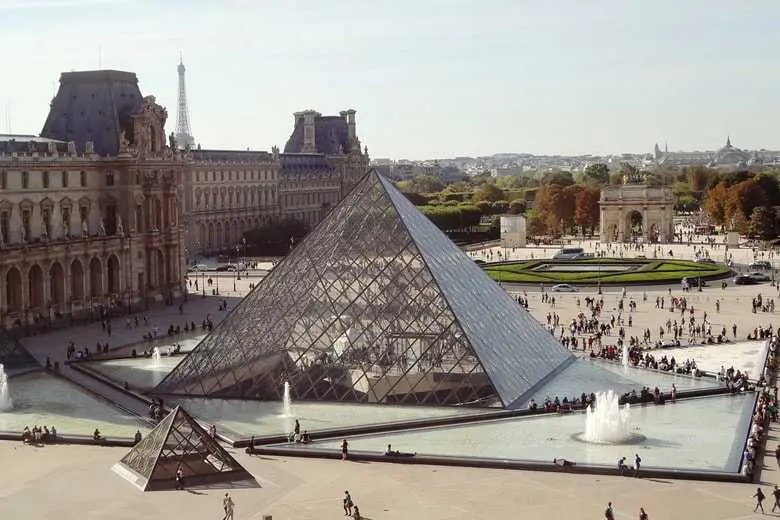
Tap button to change a color
<template>
<div>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
<div
class="mask"
style="background-color: rgba(0, 0, 0, 0.6)"
id="img-tap"
>
<div
class="d-flex justify-content-center align-items-center h-100"
>
<p class="text-white mb-0">Tap button to change a color</p>
</div>
</div>
</div>
<div class="my-3">
<MDBBtn color="primary" v-mdb-touch:tap="tapCallback">
Tap to change a color
</MDBBtn>
</div>
</div>
</template>
<script>
import { mdbTouch, MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
},
directives: {
mdbTouch
},
setup() {
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const tapCallback = () => {
const imgTap = document.querySelector("#img-tap");
imgTap.style.backgroundColor = colorGen();
};
return {
tapCallback
};
}
};
</script>
<script>
import { mdbTouch as vMdbTouch, MDBBtn } from "mdb-vue-ui-kit";
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const tapCallback = () => {
const imgTap = document.querySelector("#img-tap");
imgTap.style.backgroundColor = colorGen();
};
</script>
Double Tap
Set default taps to touch event.
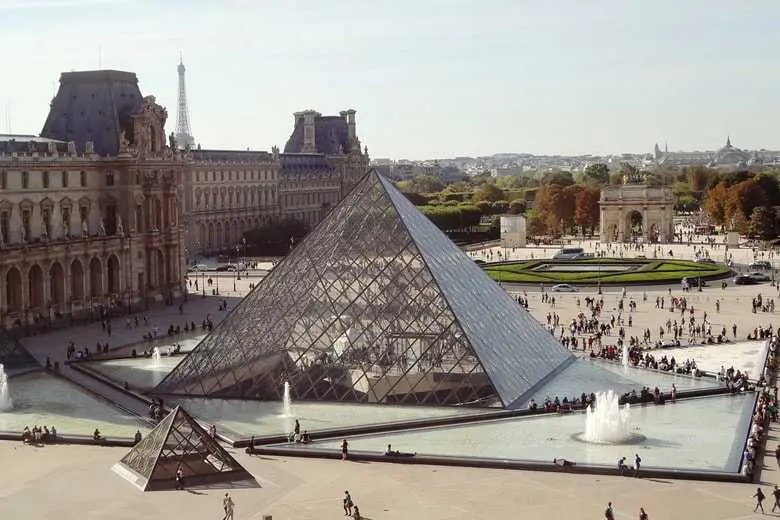
Change background color with 2 taps
<template>
<div>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
<div
class="mask"
style="background-color: rgba(0, 0, 0, 0.6)"
id="img-double-tap"
>
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0">Change background color with 2 taps</p>
</div>
</div>
</div>
<div class="my-3">
<MDBBtn
color="primary"
v-mdb-touch:tap="{ callback: doubleTapCallback, taps: 2 }"
>
Double Tap button
</MDBBtn>
</div>
</div>
</template>
<script>
import { mdbTouch, MDBBtn } from 'mdb-vue-ui-kit';
export default {
components: {
MDBBtn,
},
directives: {
mdbTouch,
},
setup() {
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const doubleTapCallback = () => {
const imgTap = document.querySelector('#img-double-tap');
imgTap.style.backgroundColor = colorGen();
};
return {
doubleTapCallback,
};
},
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch, MDBBtn } from 'mdb-vue-ui-kit';
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const doubleTapCallback = () => {
const imgTap = document.querySelector('#img-double-tap');
imgTap.style.backgroundColor = colorGen();
};
</script>
Pan
The pan event is useful for dragging elements - every time the user moves a finger on the element to which the directive is attached to, the given method is being called with an argument consisting of two keys: x and y (the values corresponds to the horizontal and vertical translation).
<template>
<div>
<div class="bg-image">
<img
v-mdb-touch:pan="{
callback: panCallback,
direction: 'all'
}"
:style="imgPanStyle"
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
id="img-pan"
/>
</div>
</div>
</template>
<script>
import { mdbTouch } from "mdb-vue-ui-kit";
import { computed, reactive } from 'vue';
export default {
directives: {
mdbTouch
},
setup() {
const translate = reactive({
x: 0,
y: 0
});
const imgPanStyle = computed(() => {
return `transform: translate(${translate.x}px, ${translate.y}px)`;
});
const panCallback = (event) => {
translate.x = translate.x + event.x;
translate.y = translate.y + event.y;
};
return {
imgPanStyle,
panCallback
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from "mdb-vue-ui-kit";
import { computed, reactive } from 'vue';
const translate = reactive<{x: number, y: number}>({
x: 0,
y: 0
});
const imgPanStyle = computed<string>(() => {
return `transform: translate(${translate.x}px, ${translate.y}px)`;
});
const panCallback = (event: Event) => {
translate.x = translate.x + event.x;
translate.y = translate.y + event.y;
};
</script>
Pan Left
Pan with only left direction
<template>
<div>
<div class="bg-image">
<img
v-mdb-touch:pan="{
callback: panLeftCallback,
direction: 'left'
}"
:style="imgPanLeftStyle"
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
</div>
</div>
</template>
<script>
import { mdbTouch } from 'mdb-vue-ui-kit';
import { computed, reactive } from 'vue';
export default {
directives: {
mdbTouch,
},
setup() {
const translateLeft = reactive({
x: 0,
y: 0,
});
const imgPanLeftStyle = computed(() => {
return `transform: translate(${translateLeft.x}px, ${translateLeft.y}px)`;
});
const panLeftCallback = (event) => {
translateLeft.x = translateLeft.x + event.x;
translateLeft.y = translateLeft.y + event.y;
};
return {
imgPanLeftStyle,
panLeftCallback,
};
},
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from 'mdb-vue-ui-kit';
import { computed, reactive } from 'vue';
const translateLeft = reactive<{x: number, y:number}>({
x: 0,
y: 0,
});
const imgPanLeftStyle = computed<string>(() => {
return `transform: translate(${translateLeft.x}px,{translateLeft.y}px)`;
});
const panLeftCallback = (event: Event) => {
translateLeft.x = translateLeft.x + event.x;
translateLeft.y = translateLeft.y + event.y;
};
</script>
Pan Right
Pan with only right direction
<template>
<div>
<div class="bg-image">
<img
v-mdb-touch:pan="{
callback: panRightCallback,
direction: 'right'
}"
:style="imgPanRightStyle"
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
</div>
</div>
</template>
<script>
import { mdbTouch } from 'mdb-vue-ui-kit';
import { computed, reactive } from 'vue';
export default {
directives: {
mdbTouch,
},
setup() {
const translateRight = reactive({
x: 0,
y: 0,
});
const imgPanRightStyle = computed(() => {
return `transform: translate(${translateRight.x}px, ${translateRight.y}px)`;
});
const panRightCallback = (event) => {
translateRight.x = translateRight.x + event.x;
translateRight.y = translateRight.y + event.y;
};
return {
imgPanRightStyle,
panRightCallback,
};
},
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from 'mdb-vue-ui-kit';
import { computed, reactive } from 'vue';
const translateRight = reactive<{x: number, y: number}>({
x: 0,
y: 0,
});
const imgPanRightStyle = computed<string>(() => {
return `transform: translate(${translateRight.x}px, ${translateRight.y}px)`;
});
const panRightCallback = (event: Event) => {
translateRight.x = translateRight.x + event.x;
translateRight.y = translateRight.y + event.y;
};
</script>
Pan Up/Down
Pan with only up/down direction
<template>
<div>
<div class="bg-image">
<img
v-mdb-touch:pan="{
callback: panVerticalCallback,
direction: 'y'
}"
:style="imgPanVerticalStyle"
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
</div>
</div>
</template>
<script>
import { mdbTouch } from 'mdb-vue-ui-kit';
import { computed, reactive } from 'vue';
export default {
directives: {
mdbTouch,
},
setup() {
const translateVertical = reactive({
x: 0,
y: 0,
});
const imgPanVerticalStyle = computed(() => {
return `transform: translate(${translateVertical.x}px, ${translateVertical.y}px)`;
});
const panVerticalCallback = (event) => {
translateVertical.x = translateVertical.x + event.x;
translateVertical.y = translateVertical.y + event.y;
};
return {
imgPanVerticalStyle,
panVerticalCallback,
};
},
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from 'mdb-vue-ui-kit';
import { computed, reactive } from 'vue';
const translateVertical = reactive<{x: number, y: number}>({
x: 0,
y: 0,
});
const imgPanVerticalStyle = computed<string>(() => {
return `transform: translate(${translateVertical.x}px, ${translateVertical.y}px)`;
});
const panVerticalCallback = (event: Event) => {
translateVertical.x = translateVertical.x + event.x;
translateVertical.y = translateVertical.y + event.y;
};
</script>
Pinch
The pinch event calls the given method with an object containing two keys - ratio and origin. The first one it the ratio of the distance between user's fingers on touchend to the same distance on touchstart - it's particularly useful for scaling images. The second one, similarly as in double tap, is a pair of coordinates indicating the middle point between the user's fingers.
<template>
<div class="bg-image" id="div-pinch">
<img
v-mdb-touch:pinch="pinchCallback"
:style="pinchImgStyle"
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
id="img-pinch"
/>
</div>
</template>
<script>
import { mdbTouch } from "mdb-vue-ui-kit";
import { computed, ref } from 'vue';
export default {
directives: {
mdbTouch
},
setup() {
const pinchImgScle = ref(1);
const pinchImgTransformOrigin = ref("center");
const pinchImgStyle = computed(() => {
return {
transform: `scale(${pinchImgScle.value})`,
transformOrigin: `${pinchImgTransformOrigin.value}`
};
});
const pinchCallback = (event) => {
pinchImgScle.value = event.ratio;
pinchImgTransformOrigin.value = `${event.origin.x}px ${event.origin.y}px`;
};
return {
pinchCallback,
pinchImgStyle
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from "mdb-vue-ui-kit";
import { computed, ref } from 'vue';
const pinchImgScle = ref(1);
const pinchImgTransformOrigin = ref("center");
const pinchImgStyle = computed(() => {
return {
transform: `scale(${pinchImgScle.value})`,
transformOrigin: `${pinchImgTransformOrigin.value}`
};
});
const pinchCallback = (event: Event) => {
pinchImgScle.value = event.ratio;
pinchImgTransformOrigin.value = `${event.origin.x}px ${event.origin.y}px`;
};
</script>
Swipe Left/Right
The swipe event comes with several modifiers (left, right, up, down, x, y) - each of them will ensure that event will fire only on swipe in that particular direction. If the directive is used without any modifier, the callback method will be called each time the swiping occurs, and the string indicating the direction will be passed as an argument.
This exmaple shows exmaple with left and right
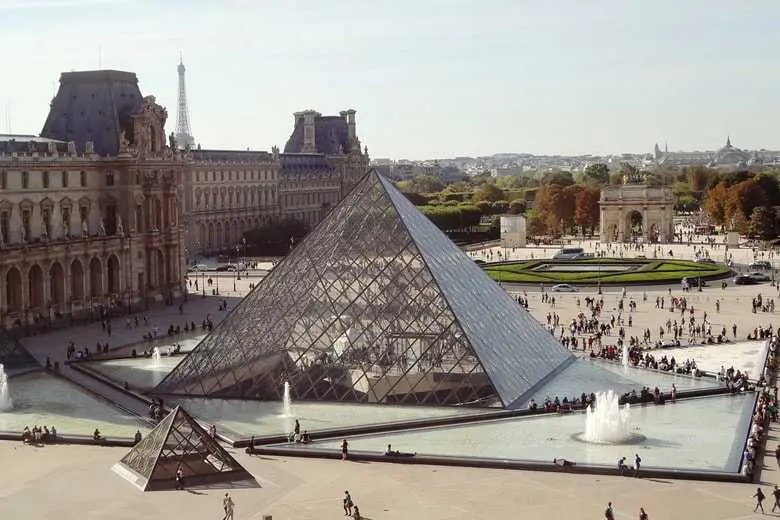
Swipe Left-Right to change a color
<template>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
<div
class="mask"
v-mdb-touch:swipe="{
callback: swipeXCallback,
direction: 'x',
threshold: 50
}"
style="background-color: rgba(0, 0, 0, 0.6)"
id="img-swipe-left-right"
>
<div
class="d-flex justify-content-center align-items-center h-100"
>
<p class="text-white mb-0">
Swipe Left-Right to change a color
</p>
</div>
</div>
</div>
</template>
<script>
import { mdbTouch } from "mdb-vue-ui-kit";
export default {
directives: {
mdbTouch
},
setup() {
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const swipeXCallback = () => {
const imgSwipeX = document.querySelector("#img-swipe-left-right");
imgSwipeX.style.backgroundColor = colorGen();
};
return {
swipeXCallback
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from "mdb-vue-ui-kit";
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const swipeXCallback = () => {
const imgSwipeX = document.querySelector("#img-swipe-left-right");
imgSwipeX.style.backgroundColor = colorGen();
};
</script>
Swipe Up/Down
This exmaple shows exmaple with up and down
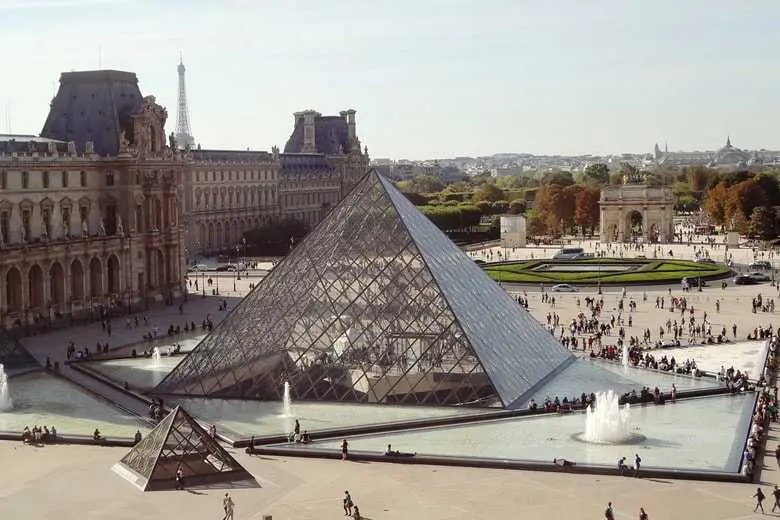
Swipe Up-Down to change a color
<template>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
/>
<div
v-mdb-touch:swipe="{
callback: swipeYCallback,
direction: 'y',
threshold: 50
}"
class="mask"
style="background-color: rgba(0, 0, 0, 0.6)"
id="img-swipe-up-down"
>
<div
class="d-flex justify-content-center align-items-center h-100"
>
<p class="text-white mb-0">Swipe Up-Down to change a color</p>
</div>
</div>
</div>
</template>
<script>
import { mdbTouch } from "mdb-vue-ui-kit";
export default {
directives: {
mdbTouch
},
setup() {
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const swipeYCallback = () => {
const imgSwipeY = document.querySelector("#img-swipe-up-down");
imgSwipeY.style.backgroundColor = colorGen();
};
return {
swipeYCallback
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from "mdb-vue-ui-kit";
const colorGen = () => {
return `hsla(${Math.random() * 360}, 100%, 50%, 0.6)`;
};
const swipeYCallback = () => {
const imgSwipeY = document.querySelector("#img-swipe-up-down");
imgSwipeY.style.backgroundColor = colorGen();
};
</script>
Rotate
This exmaple shows exmaple with rotate
<div class="bg-image">
<img
v-mdb-touch:rotate="{ callback: rotateCallback, pointers: 2 }"
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="img-fluid"
id="img-rotate"
/>
</div>
<script>
import { mdbTouch } from "mdb-vue-ui-kit";
import { ref } from 'vue';
export default {
directives: {
mdbTouch
},
setup() {
const currentAngle = ref(0);
const rotateCallback = (event) => {
const imgRotate = document.querySelector("#img-rotate");
currentAngle.value += event.change;
imgRotate.style.transform = `rotate(${currentAngle.value}deg)`;
};
return {
rotateCallback
};
}
};
</script>
<script setup lang="ts">
import { mdbTouch as vMdbTouch } from "mdb-vue-ui-kit";
import { ref } from 'vue';
const currentAngle = ref(0);
const rotateCallback = (event: Event) => {
const imgRotate = document.querySelector("#img-rotate");
currentAngle.value += event.change;
imgRotate.style.transform = `rotate(${currentAngle.value}deg)`;
};
</script>
Touch - API
Import
<script>
import {
mdbTouch
} from 'mdb-vue-ui-kit';
</script>
Properties
Tap
Property | Type | Default | Description |
---|---|---|---|
interval
|
number | 500 |
Set interval to tap |
time
|
number | 250 |
Set delays time to tap event |
taps
|
number | 1 |
Set default value of number for taps |
pointers
|
number | 1 |
Set default value of number for pointers |
Press
Property | Type | Default | Description |
---|---|---|---|
time
|
number | 250 |
Set time delays to take tap |
pointers
|
number | 1 |
Set default value of number for pointers |
Swipe
Property | Type | Default | Description |
---|---|---|---|
threshold
|
number | 20 |
Set distance between when event fires |
direction
|
string | all |
Set direction to swipe. Available options: all, right, left, top, up, x, y. |
Rotate
Property | Type | Default | Description |
---|---|---|---|
pointers
|
number | 1 |
Set default value of number for pointers |
Pan
Property | Type | Default | Description |
---|---|---|---|
threshold
|
number | 20 |
Set distance between when event fires |
direction
|
string | all |
Set direction to pan. Available options: all, right, left, top, up, x, y. |
pointers
|
number | 1 |
Set default value of number for pointers |
Pinch
Property | Type | Default | Description |
---|---|---|---|
threshold
|
number | 20 |
Set distance bettwen when event fires |