Badges
Vue Bootstrap 5 Badges component
Documentation and examples for Vue badges, our small count and labeling component.
Note: Read the API tab to find all available options and advanced customization
Basic example
Example heading New
<template>
Example heading <MDBBadge color="primary">New</MDBBadge>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge
}
};
</script>
<script setup lang="ts">
import { MDBBadge } from "mdb-vue-ui-kit";
</script>
Sizes
Badges scale to match the size of the immediate parent element by using relative font sizing
and em
units. As of v5, badges no longer have focus or hover styles for links.
Example heading New
Example heading New
Example heading New
Example heading New
Example heading New
Example heading New
<template>
<div>
<h1>Example heading
<MDBBadge color="primary">New</MDBBadge>
</h1>
<h2>Example heading
<MDBBadge color="primary">New</MDBBadge>
</h2>
<h3>Example heading
<MDBBadge color="primary">New</MDBBadge>
</h3>
<h4>Example heading
<MDBBadge color="primary">New</MDBBadge>
</h4>
<h5>Example heading
<MDBBadge color="primary">New</MDBBadge>
</h5>
<h6>Example heading
<MDBBadge color="primary">New</MDBBadge>
</h6>
</div>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge
}
};
</script>
<script setup lang="ts">
import { MDBBadge } from "mdb-vue-ui-kit";
</script>
Colors
Background colors
Use our background utility classes to quickly change the appearance of a badge. Please note
that when using Bootstrap’s default
color="light"
property, you’ll likely need a text color utility like
.text-dark
for proper styling. This is because background properties do not set
anything but background-color
.
<template>
<MDBBadge color="primary">Primary</MDBBadge>
<MDBBadge color="secondary">Secondary</MDBBadge>
<MDBBadge color="success">Success</MDBBadge>
<MDBBadge color="danger">Danger</MDBBadge>
<MDBBadge color="warning" class="text-dark">Warning</MDBBadge>
<MDBBadge color="info">Info</MDBBadge>
<MDBBadge color="light" class="text-dark">Light</MDBBadge>
<MDBBadge color="dark">Dark</MDBBadge>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge
}
};
</script>
<script setup lang="ts">
import { MDBBadge } from "mdb-vue-ui-kit";
</script>
Conveying meaning to assistive technologies:
Using color to add meaning only provides a visual indication, which will not be conveyed to
users of assistive technologies – such as screen readers. Ensure that information denoted by
the color is either obvious from the content itself (e.g. the visible text), or is included
through alternative means, such as additional text hidden with the
.visually-hidden
class.
Badge colors
You can also use smoother badge colors with badge
property
<template>
<MDBBadge badge="primary">Primary</MDBBadge>
<MDBBadge badge="secondary">Secondary</MDBBadge>
<MDBBadge badge="success">Success</MDBBadge>
<MDBBadge badge="danger">Danger</MDBBadge>
<MDBBadge badge="warning" class="text-dark">Warning</MDBBadge>
<MDBBadge badge="info">Info</MDBBadge>
<MDBBadge badge="light" class="text-dark">Light</MDBBadge>
<MDBBadge badge="dark">Dark</MDBBadge>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge
}
};
</script>
<script setup lang="ts">
import { MDBBadge } from "mdb-vue-ui-kit";
</script>
Pills
Use the pill
property to make badges more rounded with a larger
border-radius
.
<template>
<MDBBadge badge="primary" pill>Primary</MDBBadge>
<MDBBadge badge="secondary" pill>Secondary</MDBBadge>
<MDBBadge badge="success" pill>Success</MDBBadge>
<MDBBadge badge="danger" pill>Danger</MDBBadge>
<MDBBadge badge="warning" class="text-dark" pill>Warning</MDBBadge>
<MDBBadge badge="info" pill>Info</MDBBadge>
<MDBBadge badge="light" class="text-dark" pill>Light</MDBBadge>
<MDBBadge badge="dark" pill>Dark</MDBBadge>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge
}
};
</script>
<script script lang="ts">
import { MDBBadge } from "mdb-vue-ui-kit";
</script>
Positioned
Use utilities to modify a .badge
and position it in the corner of a link or button.
<template>
<div>
<MDBBtn color="primary">Inbox</MDBBtn>
<MDBBadge
color="danger"
class="translate-middle p-1"
pill
notification
>99+</MDBBadge
>
</div>
</template>
<script>
import { MDBBadge, MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge,
MDBBtn
}
};
</script>
<script>
import { MDBBadge, MDBBtn } from "mdb-vue-ui-kit";
</script>
You can also replace the .badge
class with a few more utilities without a count for a more generic indicator.
<template>
<div>
<MDBBtn color="primary">Profile</MDBBtn>
<MDBBadge
badge="danger"
class="translate-middle p-2 border border-light rounded-circle"
dot
></MDBBadge>
</div>
</template>
<script>
import { MDBBadge, MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge,
MDBBtn
}
};
</script>
<script>
import { MDBBadge, MDBBtn } from "mdb-vue-ui-kit";
</script>
With avatar
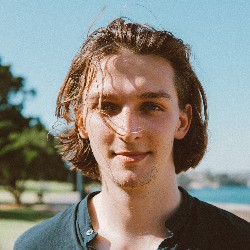
<template>
<div>
<img
class="rounded-4 shadow-4"
src="https://mdbootstrap.com/img/Photos/Avatars/man2.jpg"
alt="Avatar"
style="width: 50px; height: 50px"
/>
<MDBBadge
badge="danger"
class="translate-middle p-2 border border-light rounded-circle"
dot
></MDBBadge>
</div>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge,
}
};
</script>
<script setup lang="ts">
import { MDBBadge, MDBBtn } from "mdb-vue-ui-kit";
</script>
Icon notifications
You can use our icons and
notification
property to create a facebook-like notification.
<template>
<a href="">
<MDBIcon icon="envelope" size="lg"></MDBIcon>
<MDBBadge color="danger" dot></MDBBadge>
</a>
<a href="">
<MDBIcon icon="envelope" size="lg"></MDBIcon>
<MDBBadge color="danger" pill notification>1</MDBBadge>
</a>
<a href="">
<MDBIcon icon="envelope" size="lg"></MDBIcon>
<MDBBadge color="danger" pill notification>999+</MDBBadge>
</a>
</template>
<script>
import { MDBBadge, MDBIcon } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge,
MDBIcon
}
};
</script>
<script setup lang="ts">
import { MDBBadge, MDBIcon } from "mdb-vue-ui-kit";
</script>
Icon inside
You can place an icon inside the badge. Read icons docs to see all the available icons.
<template>
<MDBBadge color="primary" class="p-3 rounded-4">
<i class="fas fa-chart-pie"></i>
</MDBBadge>
</template>
<script>
import { MDBBadge } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge,
}
};
</script>
<script setup lang="ts">
import { MDBBadge } from "mdb-vue-ui-kit";
</script>
It composes nicely inside of the section with features.
<MDBRow>
<MDBContainer class="d-flex mb-5">
<MDBBadge
color="primary"
class="p-3 rounded-4 align-self-start"
>
<i
class="fas fa-cloud-upload-alt fa-lg text-primary fa-fw"
></i>
</MDBBadge>
<div class="d-flex flex-column align-items-start mx-5">
<p class="fw-bold mb-1">Tutorials</p>
<p class="text-muted mb-1 text-start">
Dozens of free tutorials to help you discover the full
potential of MDB.
</p>
<small><a href="">Learn more</a></small>
</div>
</MDBContainer>
<MDBContainer class="d-flex mb-5">
<MDBBadge
color="primary"
class="p-3 rounded-4 align-self-start"
>
<i class="fas fa-database fa-lg text-primary fa-fw"></i>
</MDBBadge>
<div class="d-flex flex-column align-items-start mx-5">
<p class="fw-bold mb-1">Integrations</p>
<p class="text-muted mb-1 text-start">
MDB is integrated with all major technologies and tools.
</p>
<small><a href="">Learn more</a></small>
</div>
</MDBContainer>
</MDBRow>
<MDBRow>
<MDBContainer class="d-flex mb-5">
<MDBBadge
color="primary"
class="p-3 rounded-4 align-self-start"
>
<i class="fas fa-stream fa-lg text-primary fa-fw"></i>
</MDBBadge>
<div class="d-flex flex-column align-items-start mx-5">
<p class="fw-bold mb-1">Backend friendly</p>
<p class="text-muted mb-1 text-start">
MDB is designed to be seamlessly integrated and used with
the backend.
</p>
<small><a href="">Learn more</a></small>
</div>
</MDBContainer>
<MDBContainer class="d-flex mb-5">
<MDBBadge
color="primary"
class="p-3 rounded-4 align-self-start"
>
<i class="fas fa-copy fa-lg text-primary fa-fw"></i>
</MDBBadge>
<div class="d-flex flex-column align-items-start mx-5">
<p class="fw-bold mb-1">Support and community</p>
<p class="text-muted mb-1 text-start">
The MDB team and our global community are there to support
you.
</p>
<small><a href="">Learn more</a></small>
</div>
</MDBContainer>
</MDBRow>
<script>
import { MDBBadge, MDBContainer, MDBRow } from "mdb-vue-ui-kit";
export default {
components: {
MDBBadge,
}
};
</script>
<script setup lang="ts">
import { MDBBadge, MDBContainer, MDBRow } from "mdb-vue-ui-kit";
</script>
Badges - API
Import
<script>
import {
MDBBadge
} from 'mdb-vue-ui-kit';
</script>
Properties
Property | Type | Default | Description |
---|---|---|---|
badge |
String | "" |
Changes badge color with badge-$var class |
color |
String | "" |
Changes badge color with bg-$var class |
pill |
Boolean | false |
Changes badge to rounded-pill |
dot |
Boolean | false |
Changes badge to dot style |
notification |
Boolean | false |
Changes badge to notification style |
tag |
String | "span" |
Changes badge tag |
CSS variables
As part of MDB’s evolving CSS variables approach, badge now use local CSS variables for enhanced real-time customization. Values for the CSS variables are set via Sass, so Sass customization is still supported, too.
Badges CSS variables are in different classes which belong to this component. To make it easier to use them, you can find below all of the used CSS variables.
// .badge
--#{$prefix}badge-padding-x: #{$badge-padding-x};
--#{$prefix}badge-padding-y: #{$badge-padding-y};
@include rfs($badge-font-size, --#{$prefix}badge-font-size);
--#{$prefix}badge-font-weight: #{$badge-font-weight};
--#{$prefix}badge-color: #{$badge-color};
--#{$prefix}badge-border-radius: #{$badge-border-radius};
// .badge-dot
--#{$prefix}badge-border-radius: #{$badge-dot-border-radius};
--#{$prefix}badge-height: #{$badge-dot-height};
--#{$prefix}badge-width: #{$badge-dot-width};
--#{$prefix}badge-margin-left: #{$badge-dot-margin-left};
// .badge-notification
--#{$prefix}badge-font-size: #{$badge-notification-font-size};
--#{$prefix}badge-padding-x: #{$badge-notification-padding-x};
--#{$prefix}badge-padding-y: #{$badge-notification-padding-y};
--#{$prefix}badge-margin-top: #{$badge-notification-margin-top};
--#{$prefix}badge-margin-left: #{$badge-notification-margin-left};
SCSS variables
$badge-font-size: 0.75em;
$badge-font-weight: $font-weight-bold;
$badge-color: $white;
$badge-padding-y: 0.35em;
$badge-padding-x: 0.65em;
$badge-border-radius: 0.27rem;
$badge-dot-border-radius: 4.5px;
$badge-dot-height: 9px;
$badge-dot-width: $badge-dot-height;
$badge-dot-margin-left: -0.3125rem;
$badge-notification-font-size: 0.6rem;
$badge-notification-margin-top: -0.1rem;
$badge-notification-margin-left: -0.5rem;
$badge-notification-padding-y: 0.2em;
$badge-notification-padding-x: 0.45em;