Ripple
Vue Bootstrap 5 Ripple
The ripple directive provides a radial action in the form of a visual ripple expanding outward from the user’s touch. Ripple is a visual form of feedback for touch events providing users a clear signal that an element is being touched.
Note: Read the API tab to find all available options and advanced customization
Basic example
By default, ripple is added to every MDBBtn
component and it does not require any
additional classes or attributes.
<template>
<MDBBtn color="primary" size="lg">Primary</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
A directive can be added to any element. Thanks to this you can use it to apply the ripple
effect to the given clickable element - for example to the image wrapped in
<a>
element.
<template>
<a v-mdb-ripple>
<img alt="example" class="img-fluid rounded" src="https://mdbootstrap.com/img/new/standard/city/043.webp" />
</a>
</template>
<script>
import { mdbRipple } from "mdb-vue-ui-kit";
export default {
directives: {
mdbRipple
}
};
</script>
<script setup lang="ts">
import { mdbRipple as vMdbRipple } from "mdb-vue-ui-kit";
</script>
Colors
By using color
key you can change the color of the ripple.
You can use the colors from basic MDB palette, for example
color: "primary"
or color: "danger"
.
<template>
<MDBBtn color="light" :ripple="{ color: 'primary' }">Primary</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'secondary' }">Secondary</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'success' }">Success</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'danger' }">Danger</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'warning' }">Warning</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'info' }">Info</MDBBtn>
<MDBBtn color="dark" :ripple="{ color: 'light' }">Light</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'dark' }">Dark</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
You can also use any CSS color name:
<template>
<MDBBtn color="light" :ripple="{ color: 'red' }">Red</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'green' }">Green</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'blue' }">Blue</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'yellow' }">Yellow</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'orange' }">Orange</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'purple' }">Purple</MDBBtn>
<MDBBtn color="light" :ripple="{ color: 'aqua' }">Aqua</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
Or simply use the hex color code:
<template>
<MDBBtn color="light" :ripple="{ color: '#c953d6' }">#c953d6</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#44c6e3' }">#44c6e3</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#fcc834' }">#fcc834</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#386f06' }">#386f06</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#c1303a' }">#c1303a</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#a57c3e' }">#a57c3e</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#192ced' }">#192ced</MDBBtn>
<MDBBtn color="light" :ripple="{ color: '#525740' }">#525740</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
Duration
By using duration
key you can modify the duration of the ripple (in
milliseconds).
<template>
<MDBBtn color="primary" size="lg">Default (500ms)</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ duration: 1000 }">Duration 1s</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ duration: 3000 }">Duration 3s</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ duration: 5000 }">Duration 5s</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ duration: 10000 }">Duration 10s</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
Centered
If you set centered
key to true
, the ripple will always start in the
middle of an element.
<template>
<MDBBtn color="primary" size="lg" :ripple="{ centered: true }">Centered ripple</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
Unbound
If you set unbound
key to true
, the ripple won't be bonded to the
given element and it will exceed its borders.
<template>
<MDBBtn color="primary" :ripple="{ unbound: true, color: 'dark' }">Unbound</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
Radius
By using radius
key you can modify the radius of the ripple. The numeric value is
expressed in pixels, for example: radius: 10
.
<template>
<MDBBtn color="primary" size="lg">Default</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ radius: 10 }">10</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ radius: 25 }">25</MDBBtn>
<MDBBtn color="primary" size="lg" :ripple="{ radius: 50 }">50</MDBBtn>
</template>
<script>
import { MDBBtn } from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn
}
};
</script>
<script setup lang="ts">
import { MDBBtn } from "mdb-vue-ui-kit";
</script>
Examples
You can apply the ripple directive to any element. Options can be passed as an object, for
example:
v-mdb-ripple="{ color: 'secondary', centered: true }"
.
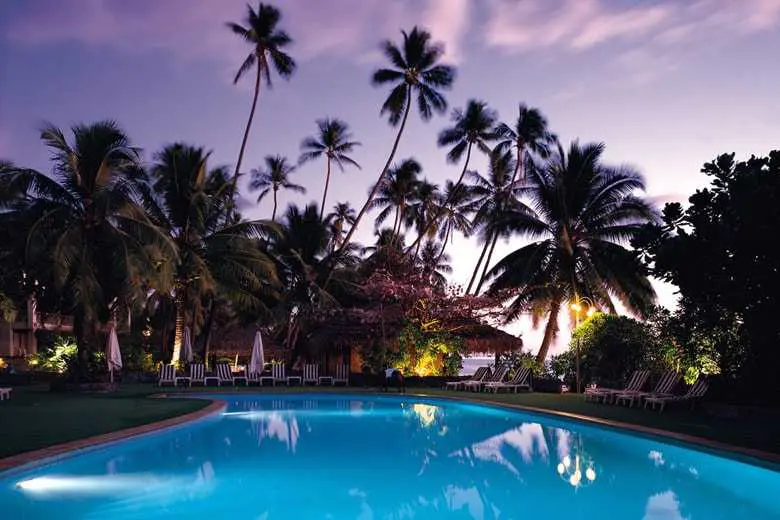
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Button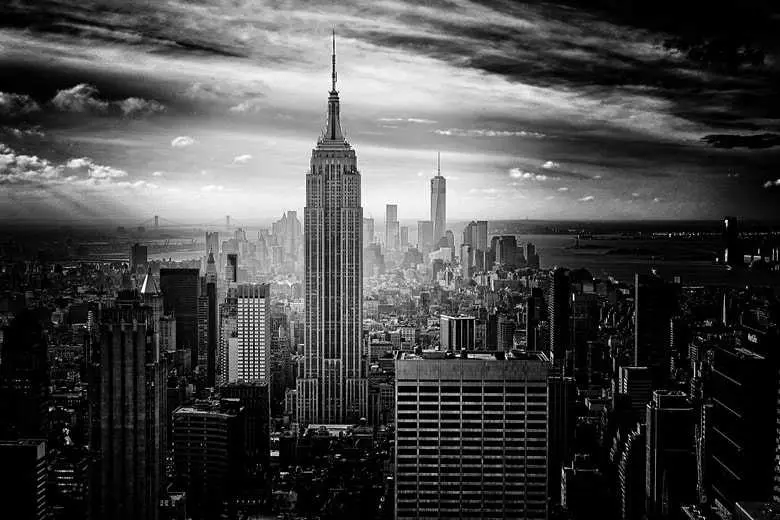
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Button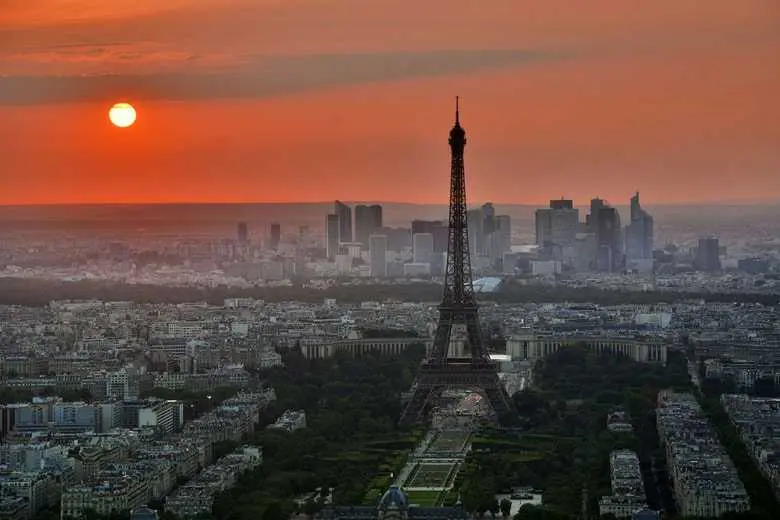
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Button
<template>
<MDBRow>
<MDBCol md="4">
<MDBCard style="max-width: 22rem">
<a v-mdb-ripple>
<MDBCardImg src="https://mdbootstrap.com/img/new/standard/city/024.webp" top alt="..." />
</a>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and
make up the bulk of the card's content.
</MDBCardText>
<MDBBtn color="primary">Button</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4">
<MDBCard style="max-width: 22rem">
<a v-mdb-ripple>
<MDBCardImg src="https://mdbootstrap.com/img/new/standard/city/025.webp" top alt="..." />
</a>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and
make up the bulk of the card's content.
</MDBCardText>
<MDBBtn color="primary">Button</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4">
<MDBCard style="max-width: 22rem">
<a v-mdb-ripple>
<MDBCardImg src="https://mdbootstrap.com/img/new/standard/city/032.webp" top alt="..." />
</a>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and
make up the bulk of the card's content.
</MDBCardText>
<MDBBtn color="primary">Button</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</template>
<script>
import {
mdbRipple,
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBCardImg,
MDBCol,
MDBRow
} from "mdb-vue-ui-kit";
export default {
components: {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBCardImg,
MDBCol,
MDBRow
},
directives: {
mdbRipple
}
};
</script>
<script setup lang="ts">
import {
mdbRipple as vMdbRipple,
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBCardImg,
MDBCol,
MDBRow
} from "mdb-vue-ui-kit";
</script>
Ripple - API
Import
<script>
import {
mdbRipple
} from 'mdb-vue-ui-kit';
</script>
Properties
Property | Type | Default | Description |
---|---|---|---|
centered
|
Boolean | false |
Centers position of the ripple. |
color
|
String | "" |
Changes color of the ripple. |
duration
|
Number | 500 |
Sets duration of the animation in milliseconds. |
radius
|
Number | diameter / 2 |
Sets radius value in px. |
unbound
|
Boolean | false |
Sets whether the effect should go beyond the wrapper's boundaries. |