Masks
Vue Bootstrap 5 Masks
Masks alter the visibility of an element by either partially or fully hiding it. Masks are used to make content more visible by providing a proper contrast. They are most often used on images.
Without mask
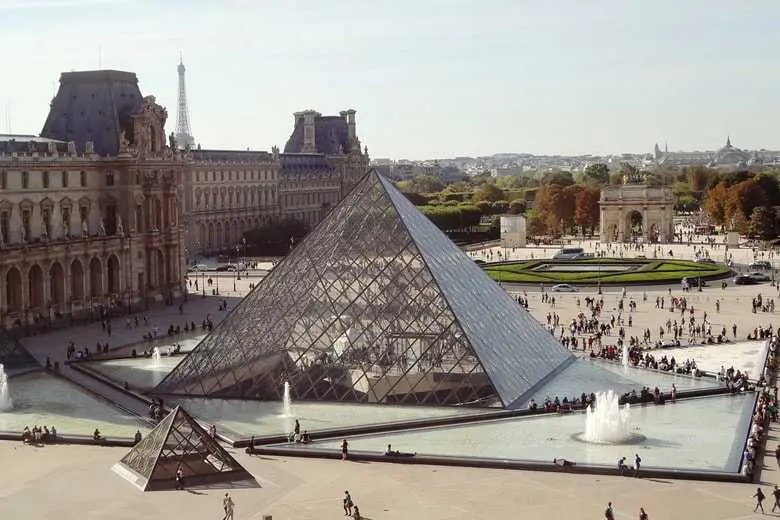
Can you see me?
With mask
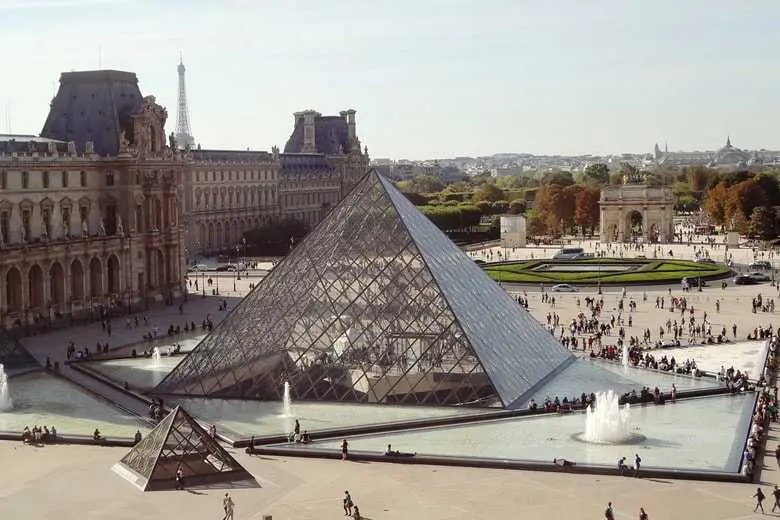
Can you see me?
Basic example
A simple example of mask usage.
<template>
<div class="bg-image">
<img src="https://mdbootstrap.com/img/new/standard/city/053.webp" class="w-100" />
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)"></div>
</div>
</template>
How it works
A detailed explanation of how the masks work in MDB:
-
Masks require
.bg-image
wrapper which sets a position to relative, overflow to hidden, and properly center the image. -
The inside
.bg-image
wrapper as the first child we place animg
element with the source link -
Below is the actual mask. We set a color and opacity via
rgba
code and inline CSS. In the section, you will find a detailed explanation of how RGBA colors work with masks - If you want to put a text on the image you have to place it within the .mask wrapper. To center it you have to use flex utilities (explained in the section below).
RGBA
By manipulating RGBA code you can change the color and opacity of the mask.
Color
rgba(18, 102, 241, 0.6)
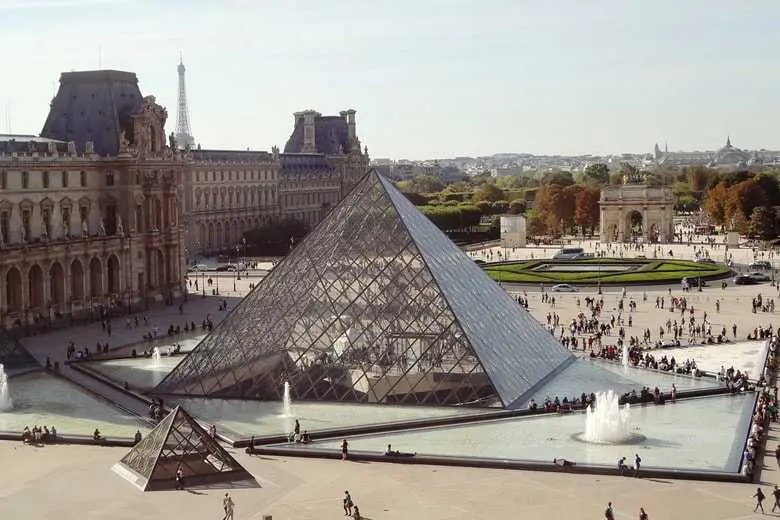
rgba(178, 60, 253, 0.6)
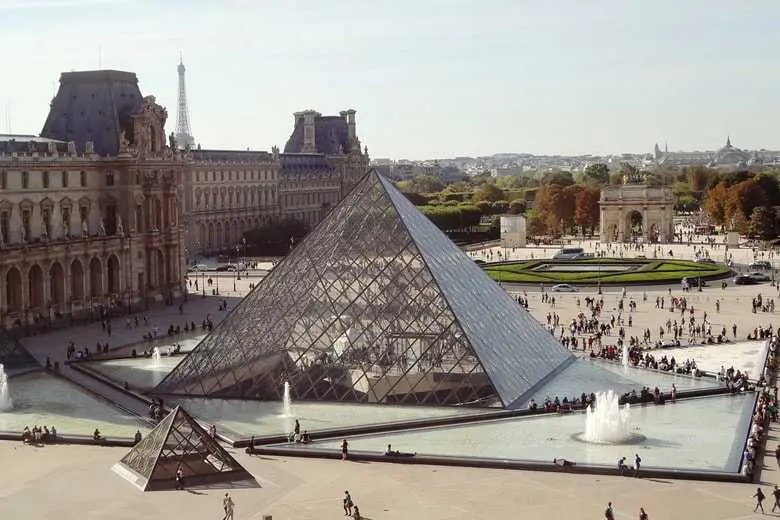
rgba(0, 183, 74, 0.6)
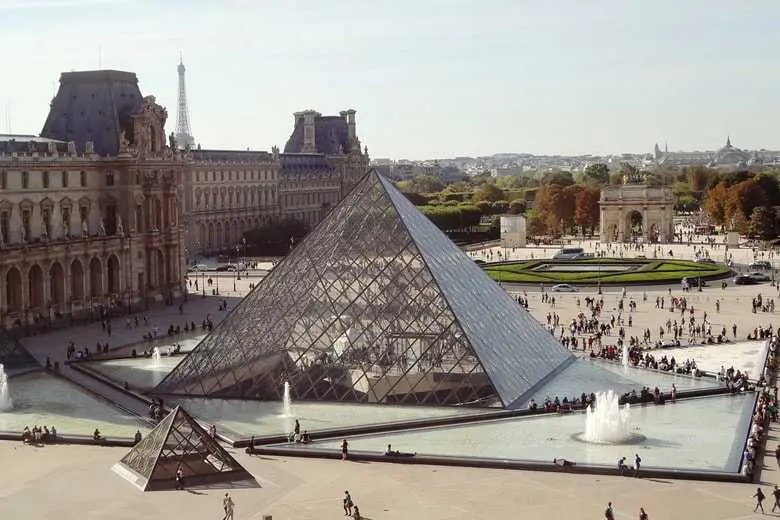
rgba(249, 49, 84, 0.6)
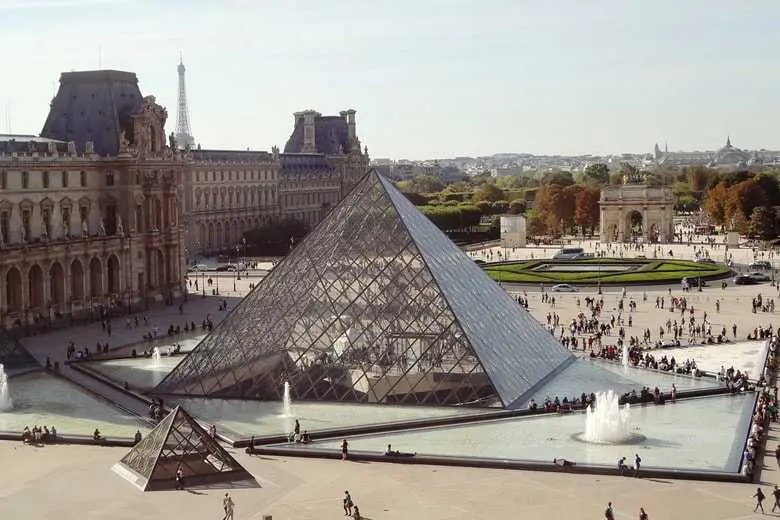
rgba(251, 251, 251, 0.6)
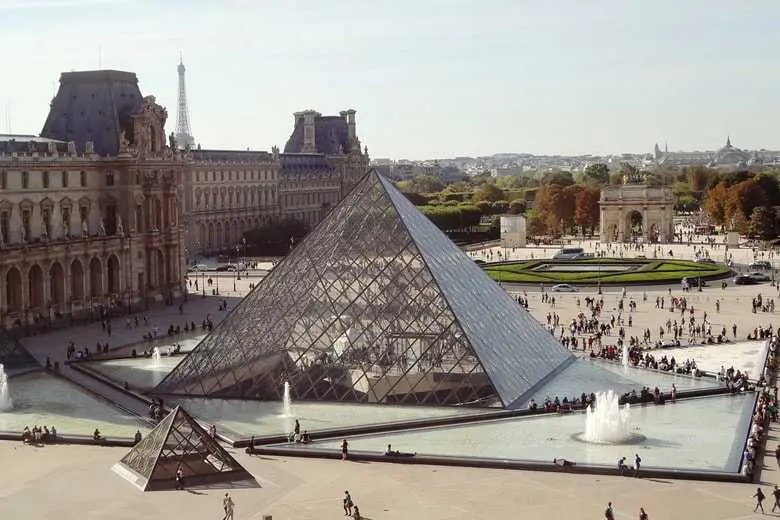
rgba(57, 192, 237, 0.6)
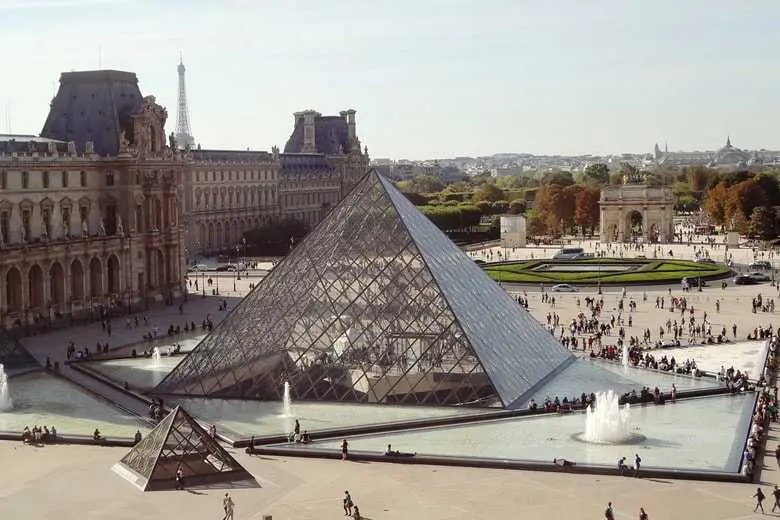
You can even set a fancy gradient as a mask.
rgba(57, 192, 237, 0.6)
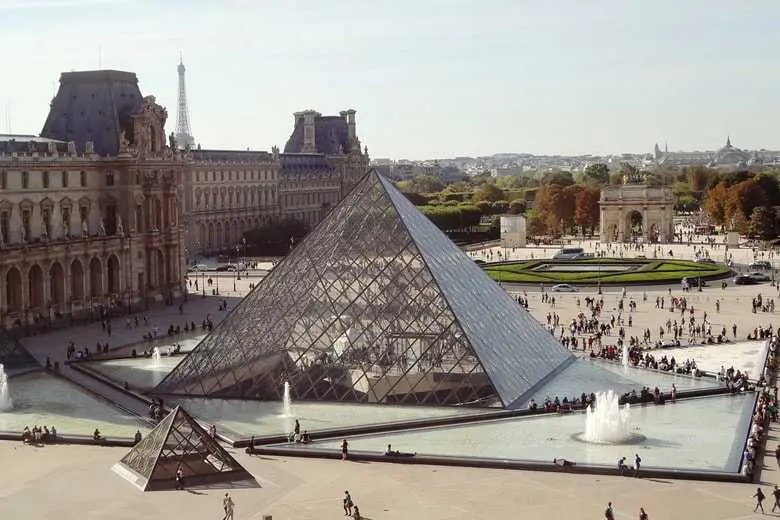
<template>
<div class="bg-image">
<img src="https://mdbootstrap.com/img/new/standard/city/053.webp" class="w-100" />
<div
class="mask"
style="
background: linear-gradient(
45deg,
rgba(29, 236, 197, 0.7),
rgba(91, 14, 214, 0.7) 100%
);
"
></div>
</div>
</template>
Opacity
By changing the last value in the RGBA color you can manipulate the opacity of the mask.
0.0 means fully transparent and 1.0 fully opaque. You can set any value between 0.0 and 1.0.
rgba(0, 0, 0, 0.0)
- fully transparent
rgba(0, 0, 0, 1.0)
- fully opaque
0.1
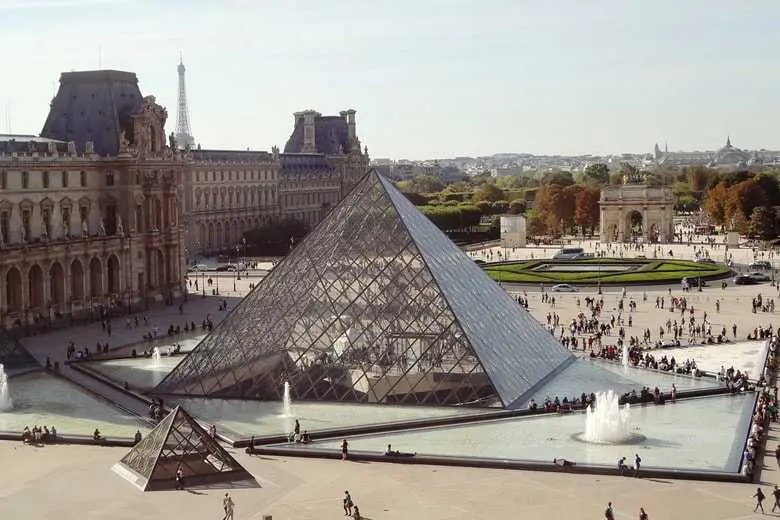
0.3
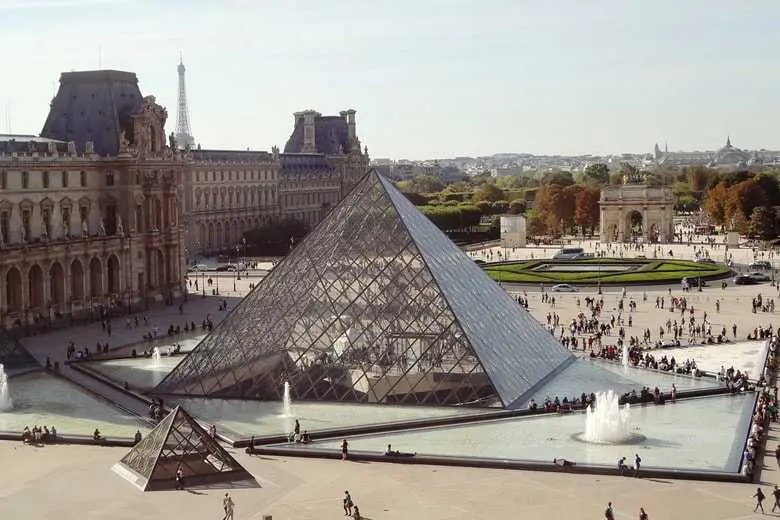
0.55
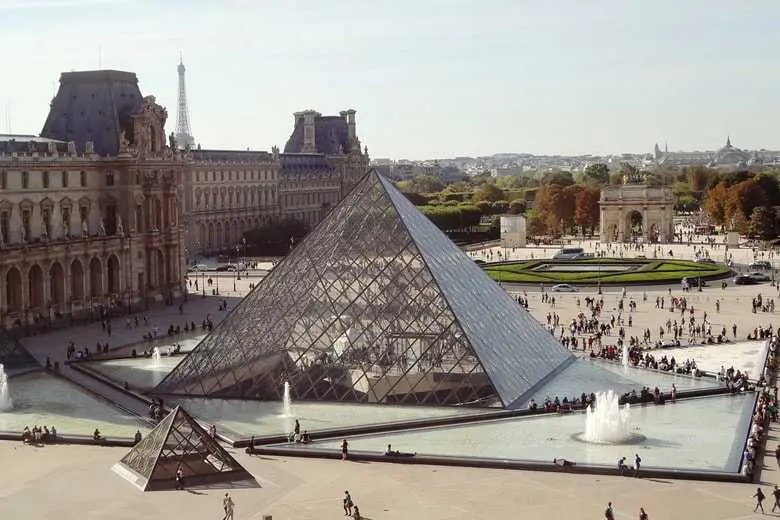
0.7
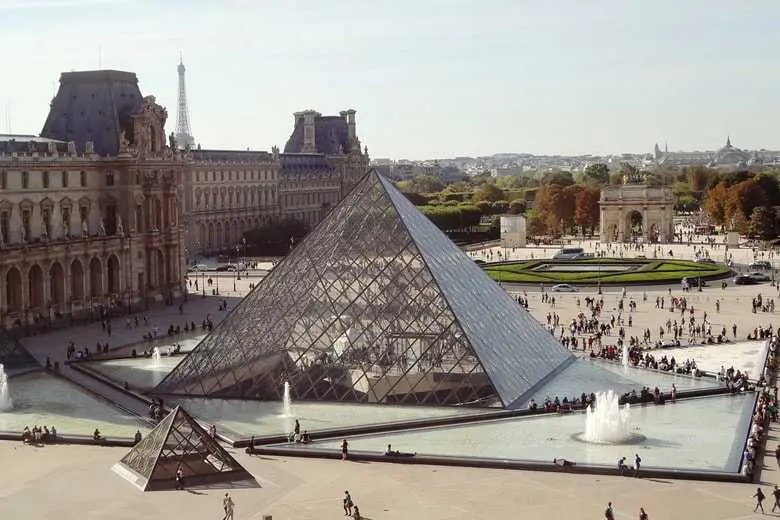
0.8
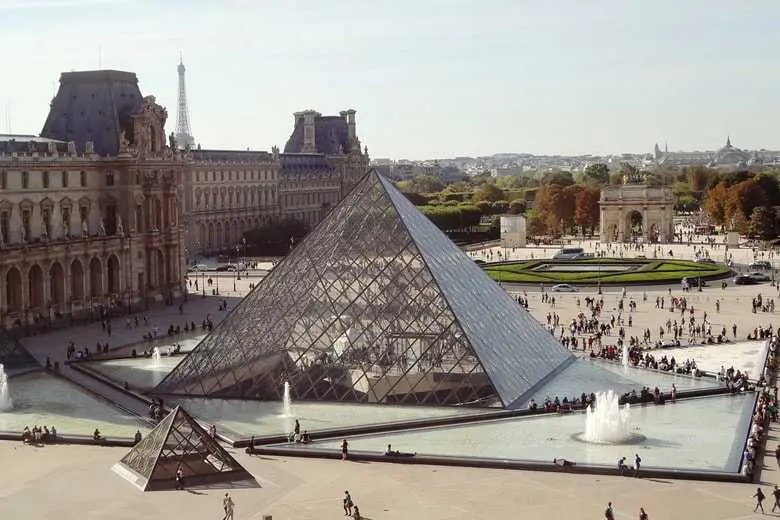
0.9
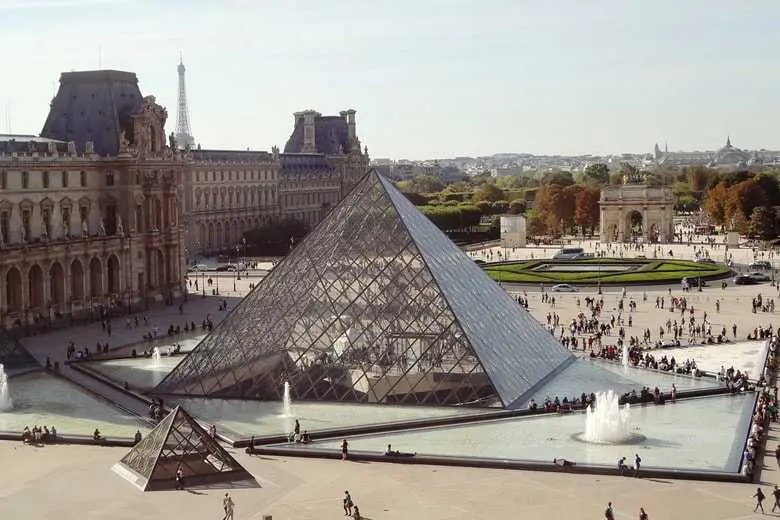
Content
The main goal of the masks is to provide a proper contrast between the image and the content placed on it. Most commonly we put a text on the images with masks.
Within .mask
wrapper place a div
and apply
flexbox utilities to center the content vertically
and horizontally. Then put the text inside.
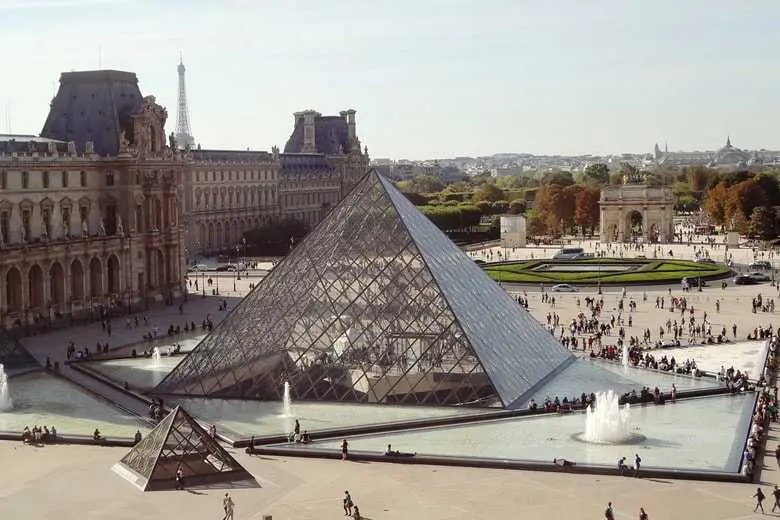
Can you see me?
<template>
<div class="bg-image">
<img
src="https://mdbootstrap.com/img/new/standard/city/053.webp"
class="w-100"
alt="Sample"
/>
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)">
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0">Can you see me?</p>
</div>
</div>
</div>
</template>
Ripple
You can easily add a ripple effect to the image with a mask.
Simply add mdbRipple
directive to wrapper element.
<template>
<!--Grid row-->
<MDBRow>
<!--Grid column-->
<MDBCol md="6" class="mb-4">
<!-- Default dark color ripple -->
<div class="bg-image" v-mdb-ripple>
<img src="https://mdbootstrap.com/img/new/standard/city/053.webp" class="w-100" />
<div class="mask" style="background-color: rgba(251, 251, 251, 0.6)"></div>
</div>
</MDBCol>
<!--Grid column-->
<!--Grid column-->
<MDBCol md="6" class="mb-4">
<!-- Light color ripple changed via directive argument -->
<div class="bg-image" v-mdb-ripple="{ color: 'light' }">
<img src="https://mdbootstrap.com/img/new/standard/city/053.webp" class="w-100" />
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)"></div>
</div>
</MDBCol>
<!--Grid column-->
</MDBRow>
<!--Grid row-->
</template>
<script>
import { MDBRow, MDBCol, mdbRipple } from 'mdb-vue-ui-kit';
export default {
components: {
MDBRow,
MDBCol,
},
directives: { mdbRipple },
};
</script>
<script setup lang="ts">
import { MDBRow, MDBCol, mdbRipple } from 'mdb-vue-ui-kit';
const vMdbRipple = mdbRipple
</script>
Link
By wrapping a mask in a
element you can change the image into a clickable link.
Regular
Regular link without additional effects.
<template>
<div class="bg-image">
<img src="https://mdbootstrap.com/img/new/standard/city/053.webp" class="w-100" />
<a href="#!">
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)"></div>
</a>
</div>
</template>
With ripple
You can also add mdbRipple
directive to the .bg-image
to achieve
an additional ripple effect.
<template>
<div class="bg-image" style="max-width: 24rem" v-mdb-ripple>
<img src="https://mdbootstrap.com/img/new/standard/city/053.webp" class="w-100" />
<a>
<div class="mask" style="background-color: rgba(251, 251, 251, 0.4)"></div>
</a>
</div>
</template>
<script>
import { mdbRipple } from 'mdb-vue-ui-kit';
export default {
directives: { mdbRipple },
};
</script>
<script setup lang="ts">
import { mdbRipple } from 'mdb-vue-ui-kit';
const vMdbRipple = mdbRipple
</script>
Gradient
These are linear, gradient masks (a variation of our standard masks) that allow you to darken the background around the text in an image, but do not cover the entire image, and therefore partially retain its natural colors.
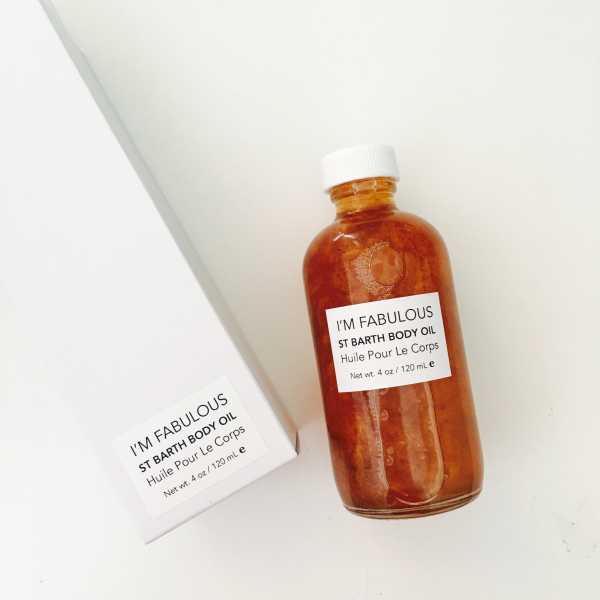
Can you see me?
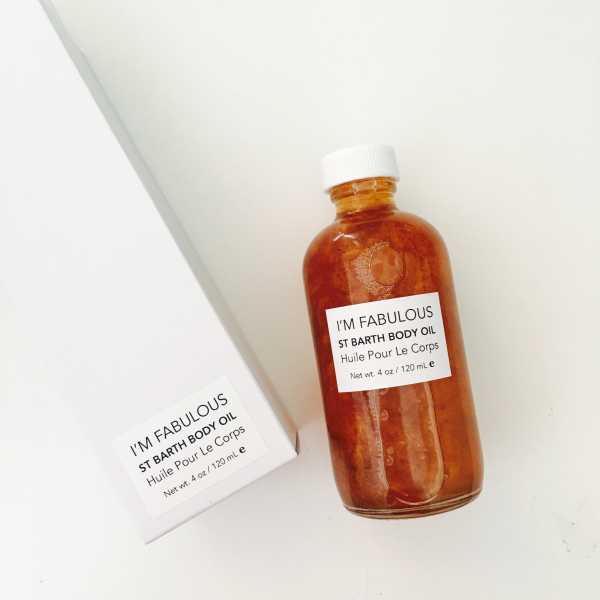
Can you see me?
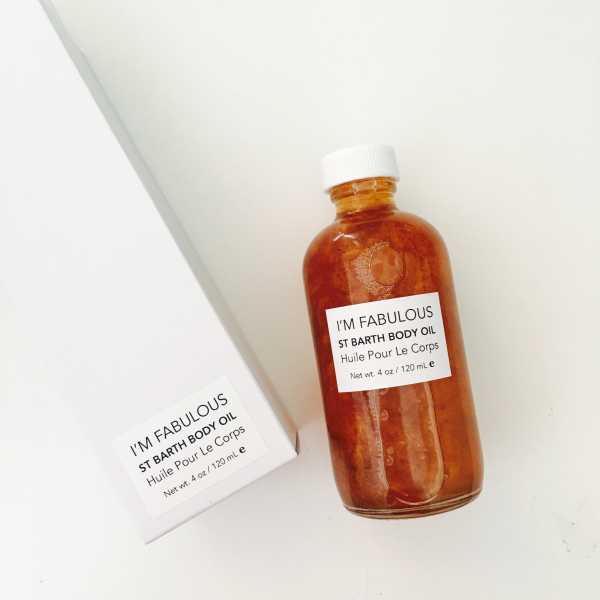
Can you see me?
<template>
<MDBRow>
<MDBCol lg="4" md="12" class="mb-4 mb-lg-0">
<!-- Image with no mask -->
<div class="bg-image rounded-6">
<img
src="https://mdbootstrap.com/img/new/ecommerce/vertical/010.jpg"
class="w-100"
alt="Alternative text"
/>
<!-- Mask -->
<div class="mask">
<div
class="
bottom-0
d-flex
align-items-end
h-100
text-center
justify-content-center
"
>
<div>
<h2 class="fw-bold text-white mb-4">Can you see me?</h2>
</div>
</div>
</div>
</div>
</MDBCol>
<MDBCol lg="4" class="mb-lg-0">
<!-- Image with black mask -->
<div class="bg-image rounded-6">
<img
src="https://mdbootstrap.com/img/new/ecommerce/vertical/010.jpg"
class="w-100"
alt="Alternative text"
/>
<!-- Mask -->
<div
class="mask"
style="
background: linear-gradient(
to bottom,
hsla(0, 0%, 0%, 0) 50%,
hsla(0, 0%, 0%, 0.5)
);
"
>
<div
class="
bottom-0
d-flex
align-items-end
h-100
text-center
justify-content-center
"
>
<div>
<h2 class="fw-bold text-white mb-4">Can you see me?</h2>
</div>
</div>
</div>
</div>
</MDBCol>
<MDBCol lg="4" class="mb-4 mb-lg-0">
<!-- Image with violet mask -->
<div class="bg-image rounded-6">
<img
src="https://mdbootstrap.com/img/new/ecommerce/vertical/010.jpg"
class="w-100"
alt="Alternative text"
/>
<!-- Mask -->
<div
class="mask"
style="
background: linear-gradient(
to bottom,
hsla(0, 0%, 0%, 0),
hsla(263, 80%, 20%, 0.5)
);
"
>
<div
class="
bottom-0
d-flex
align-items-end
h-100
text-center
justify-content-center
"
>
<div>
<h2 class="fw-bold text-white mb-4">Can you see me?</h2>
</div>
</div>
</div>
</div>
</MDBCol>
</MDBRow>
</template>
<script>
import { MDBRow, MDBCol } from 'mdb-vue-ui-kit';
export default {
components: {
MDBRow,
MDBCol
},
};
</script>
<script setup lang="ts">
import { MDBRow, MDBCol } from 'mdb-vue-ui-kit';
</script>