Using v-for loop
Vue v-for loop - free examples & tutorial
Learn how to use v-for loop in vue.
Initial events list
Since the Event
component receives information like the date or title from
the App
(parent) component, it is now the App
component which will be responsible for storing and managing lists of events.
Let's add an initial list of the events into the App.vue
script part inside
of the data()
function:
<script>
import { mdbContainer, mdbRow, mdbCol } from "mdbvue";
import Event from "@/components/Event";
export default {
name: "App",
components: {
mdbContainer,
mdbRow,
mdbCol,
Event
},
data() {
return {
events: [
{
time: "10:00",
title: "Breakfast with Simon",
location: "Lounge Caffe",
description: "Discuss Q3 targets"
},
{
time: "10:30",
title: "Daily Standup Meeting (recurring)",
location: "Warsaw Spire Office"
},
{
time: "11:00",
title: "Call with HRs"
},
{
time: "12:00",
title: "Lunch with Timmoty",
location: "Canteen",
description: "Project evalutation"
}
]
};
},
};
</script>
Now when we have our initial data, let's learn how to use for loop to dynamically
generate all events from the events[]
array.
v-for
Dynamic loop
Let's replace our static Event
code:
<Event time="10:00" title="Breakfast with Simon" location="Lounge Caffe" description="Discuss Q3 targets"/>
<Event time="10:30" title="Daily Standup Meeting (recurring)" location="Warsaw Spire Office"/>
with a dynamic call:
<Event
v-for="event in events"
:time="event.time"
:title="event.title"
:location="event.location"
:description="event.description"
/>
The v-for
is quite self-descriptive. It loops through each
element within the events[]
array and creates an instance of
each Event
component.
In order to access attributes of a particular event we are defining its alias
in the v-for
declaration:
v-for="event in events"
Now we can access it, i.e. its title calling event.title
.
The alias for a single element can have any name, we could use shorter version
and call it e
:
<Event
v-for="e in events"
:time="e.time"
:title="e.title"
:location="e.location"
:description="e.description"
/>
However, it's a good practice to use a descriptive name to
make your code easier to understand. As you also noticed within
the v-for
loop we are mapping our element attributes i.e.
event.time
(right side) to the Event
time
property (left side):
(Event component instance property) :time = "event.time"
(events[] array element)
Unique key
Everything works like a charm so far. At least in a browser. However, if we look into our console we will notice the following Warning:
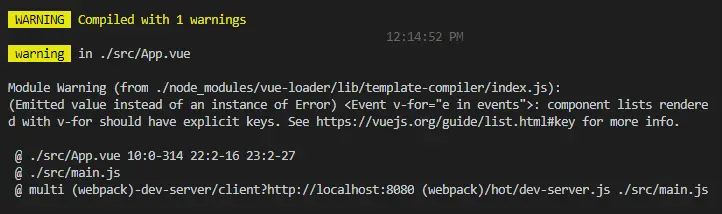
This warning tells us that each element should contain a unique key. This helps Vue to access each element. Let's quickly check why this is important.
With the help of a small Chrome extension - Vue.js devtools we can check the Virtual DOM of our application. This is how it looks now:
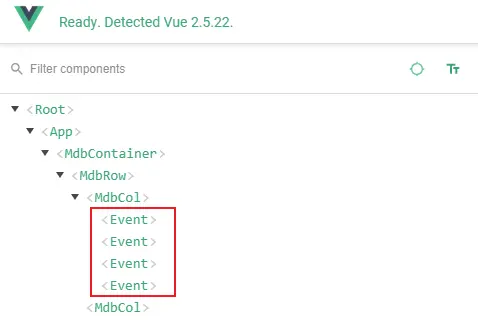
As you can see, all Event
component instances look exactly the same.
If we want to update a specific one it's difficult to access a particular one. We can quickly
fix this. Let's update our loop:
<Event
v-for="(event, index) in events"
:index="index"
:time="event.time"
:title="event.title"
:location="event.location"
:description="event.description"
:key="index"
/>
And check our code again using the same extension:
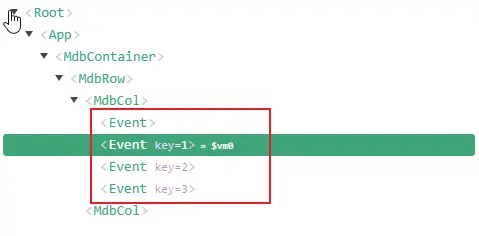
As you can see each Event has now a unique key (the first element has by default key=0, therefore it is not displayed).
Let's quickly review the new v-for
code. We added the second parameter (index) parameter to our loop:
v-for="(event, index) in events"
This is an auto incremented index, which means it increases value with each iteration.
We have bound it to the key
property which will help Vue to quickly access a specific
item without needing to loop through all the elements within the array.
We also added an extra property index:
and mapped it to the same value. This will
help us within the application, to access a specific Event
from the source code.
Since we have added mapping to a new property, the last step to do is to extend a list of
properties in the Event.vue
file. Let's add a new prop to the props list:
props: {
index: {
type: Number
},
time: {
type: String
},
title: {
type: String
},
location: {
type: String
},
description: {
type: String
}
},