Modal
Vue Bootstrap 5 Modal component
Use MDB modal plugin to add dialogs to your site for lightboxes, user notifications, or completely custom content.
Note: Read the API tab to find all available options and advanced customization
Basic example
Click the button to launch the modal.
<template>
<!-- Button trigger modal -->
<MDBBtn
color="primary"
aria-controls="exampleModal"
@click="exampleModal=true"
>
Launch demo modal
</MDBBtn>
<MDBModal
id="exampleModal"
tabindex="-1"
labelledby="exampleModalLabel"
v-model="exampleModal"
>
<MDBModalHeader>
<MDBModalTitle id="exampleModalLabel"> Modal title </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>...</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="exampleModal = false">Close</MDBBtn>
<MDBBtn color="primary">Save changes</MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
},
setup() {
const exampleModal = ref(false);
return {
exampleModal,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const exampleModal = ref(false);
</script>
Advanced examples
Click the buttons to launch the modals.
Frame modal
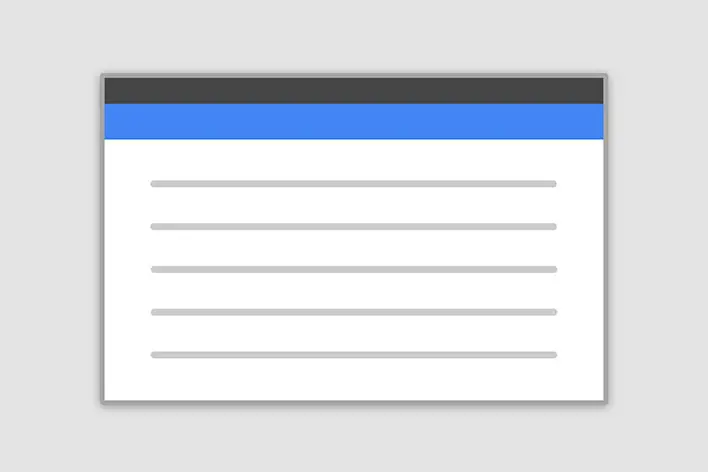
Position
Side modal
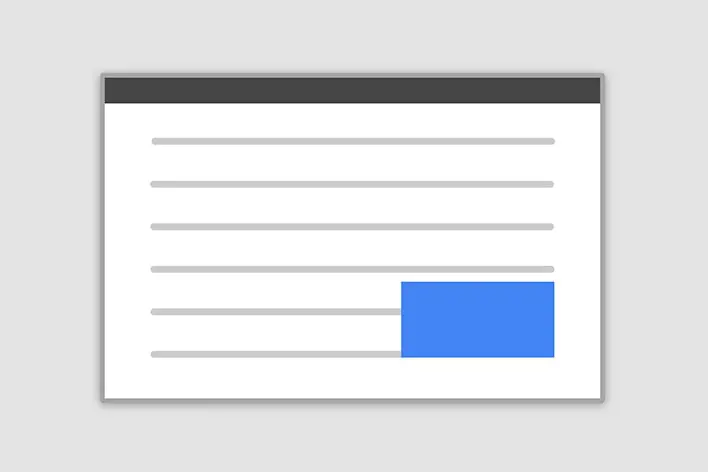
Position
Central modal
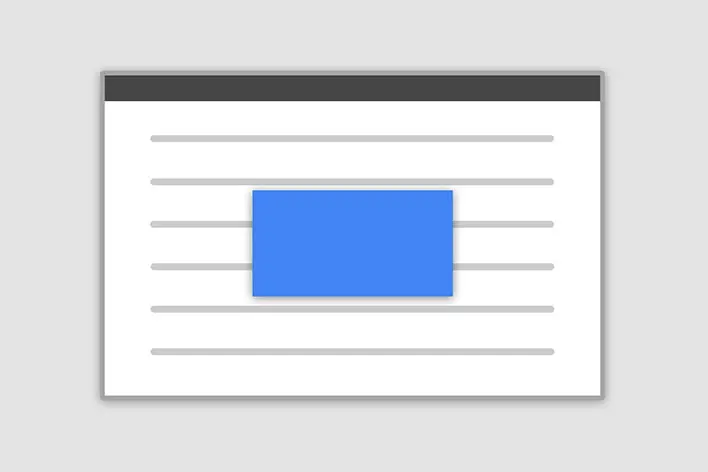
Size
How it works
Before getting started with MDB modal component, be sure to read the following as our menu options have recently changed.
-
Modals are positioned over everything else in the document and remove scroll from the
<body>
so that modal content scrolls instead. - Clicking on the modal “backdrop” will automatically close the modal.
- Bootstrap only supports one modal window at a time. Nested modals aren’t supported as we believe them to be poor user experiences.
-
Modals use
position: fixed
, which can sometimes be a bit particular about its rendering. Whenever possible, place your modal HTML in a top-level position to avoid potential interference from other elements. You’ll likely run into issues when nesting aMDBModal
within another fixed element. -
Once again, due to
position: fixed
, there are some caveats with using modals on mobile devices. -
Due to how HTML5 defines its semantics,
the
autofocus
HTML attribute has no effect in Bootstrap modals. To achieve the same effect, use some custom JavaScript:
<template>
<MDBModal v-on:shown="setFocus" v-model="show">
<MDBModalBody>
<MDBInput id="myInput" />
</MDBModalBody>
</MDBModal>
<MDBBtn @click="show = true">Open modal</MDBBtn>
</template>
<script>
import { MDBModal, MDBModalBody, MDBInput, MDBBtn } from 'mdb-vue-ui-kit';
import { ref } from 'vue'
export default {
components: {
MDBModal,
MDBModalBody,
MDBInput,
MDBBtn,
},
setup() {
const setFocus = () => {
const myInput = document.getElementById('myInput');
myInput.focus();
}
const show = ref(false);
return {
setFocus,
show,
};
},
};
</script>
<script setup lang="ts">
import { MDBModal, MDBModalBody, MDBInput, MDBBtn } from 'mdb-vue-ui-kit';
import { ref } from 'vue'
const setFocus = () => {
const myInput = document.getElementById('myInput') as HTMLInputElement;
myInput.focus();
}
const show = ref(false);
</script>
Modal components
Below is a static MDBModal
example (meaning its
position
and display
have been overridden). Included are the
MDBModalHeader
, MDBModalBody
(required for padding
),
and MDBModalFooter
(optional). MDBModalHeader
is by default included
with dismiss action button. You can also provide another explicit dismiss action, e.g. inside
MDBModalFooter
component.
<template>
<MDBModal
tabindex="-1"
style="position: static; display: block; height: auto"
:modelValue="true"
removeBackdrop
:keyboard="false"
:focus="false"
>
<MDBModalHeader>
<MDBModalTitle>Modal title</MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>
<p>Modal body text goes here.</p>
</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary"> Close </MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
</script>
Position
To change the position of the modal add one of the following properties to the
MDBModal
Top right: side
+
position="top-right"
Top left: side
+
position="top-left"
Bottom right: side
+
position="bottom-right"
Bottom left: side
+
position="bottom-right"
Note: If you want to change the direction of modal animation, add
position="top | right | bottom | left"
property to the MDBModal
.
<template>
<!-- Button trigger modal -->
<MDBBtn
color="primary"
aria-controls="exampleSideModal1"
@click="exampleSideModal1 = true"
class="mb-2"
>
Top right
</MDBBtn>
<!-- Modal example - top right -->
<MDBModal
side
position="top-right"
direction="right"
id="exampleSideModal1"
tabindex="-1"
labelledby="exampleSideModalLabel1"
v-model="exampleSideModal1"
>
<MDBModalHeader color="info" class="text-white">
<MDBModalTitle id="exampleSideModalLabel1"> Product in the cart </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>
<MDBRow>
<MDBCol col="3" class="text-center">
<i class="fas fa-shopping-cart fa-4x text-info"></i>
</MDBCol>
<MDBCol col="9">
<p>Do you need more time to make a purchase decision?</p>
<p>No pressure, your product will be waiting for you in the cart.</p>
</MDBCol>
</MDBRow>
</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="info"> Go to the cart </MDBBtn>
<MDBBtn color="outline-info" @click="exampleSideModal1 = false"> Close </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBRow,
MDBCol,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBRow,
MDBCol,
},
setup() {
const exampleSideModal1 = ref(false);
return {
exampleSideModal1,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBRow,
MDBCol,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const exampleSideModal1 = ref(false);
</script>
Frame
To make the modal "frame-like" add frame
property to the
MDBModal
element. You also need to specify the position
property
with bottom
or top
values.
<template>
<!-- Button trigger modal -->
<MDBBtn
color="primary"
aria-controls="exampleFrameModal1"
@click="exampleFrameModal1 = true"
class="mb-2"
>
Top
</MDBBtn>
<!-- Modal frame top example -->
<MDBModal
frame
position="top"
id="exampleFrameModal1"
tabindex="-1"
labelledby="exampleFrameModal1"
v-model="exampleFrameModal1"
>
<MDBModalBody class="py-1">
<div class="d-flex justify-content-center align-items-center my-3">
<h4>
<MDBBadge color="primary">v52gs1</MDBBadge>
</h4>
<p class="pt-3 mx-4">
We have a gift for you! Use this code to get a
<strong>10% discount</strong>.
</p>
<MDBBtn color="primary" size="sm" class="ms-2"> Use it </MDBBtn>
<MDBBtn color="info" size="sm" @click="exampleFrameModal1 = false">
No, thanks
</MDBBtn>
</div>
</MDBModalBody>
</MDBModal>
<!-- Modal frame top example-->
</template>
<script>
import {
MDBModal,
MDBModalBody,
MDBBtn,
MDBBadge
} from "mdb-vue-ui-kit";
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalBody,
MDBBtn,
MDBBadge
},
setup() {
const exampleFrameModal1 = ref(false);
return {
exampleFrameModal1
}
}
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalBody,
MDBBtn,
MDBBadge
} from "mdb-vue-ui-kit";
import { ref } from 'vue';
const exampleFrameModal1 = ref(false);
</script>
Static backdrop
When staticBackdrop
property is added to MDBModal
, the modal will
not close when clicking outside it. Click the button below to try it.
<template>
<!-- Button trigger modal -->
<MDBBtn
color="primary"
aria-controls="staticBackdropLabel"
@click="staticBackdrop = true"
>
Launch static backdrop modal
</MDBBtn>
<!-- Modal -->
<MDBModal
id="staticBackdrop"
tabindex="-1"
labelledby="staticBackdropLabel"
v-model="staticBackdrop"
staticBackdrop
>
<MDBModalHeader>
<MDBModalTitle id="staticBackdropLabel"> Modal title </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>...</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="staticBackdrop = false"> Close </MDBBtn>
<MDBBtn color="primary"> Understood </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
},
setup() {
const staticBackdrop = ref(false);
return {
staticBackdrop,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const staticBackdrop = ref(false);
</script>
Scrolling long content
When modals become too long for the user’s viewport or device, they scroll independent of the page itself. Try the demo below to see what we mean.
You can also create a scrollable modal that allows scroll the modal body by adding
scrollable
property to MDBModal
.
<template>
<MDBBtn
color="primary"
aria-controls="exampleModalLongTitle"
@click="exampleModalLong = true"
>
Launch demo modal
</MDBBtn>
<MDBModal
class="modal fade"
id="exampleModalLong"
tabindex="-1"
labelledby="exampleModalLongTitle"
v-model="exampleModalLong"
>
<MDBModalHeader>
<MDBModalTitle id="exampleModalLongTitle"> Modal title </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody> ... long body </MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="exampleModalLong = false"> Close </MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
<MDBBtn
color="primary"
aria-controls="exampleModalScrollableTitle"
@click="exampleModalScrollable = true"
>
Launch demo modal
</MDBBtn>
<MDBModal
id="exampleModalScrollable"
tabindex="-1"
labelledby="exampleModalScrollableTitle"
v-model="exampleModalScrollable"
scrollable
>
<MDBModalHeader>
<MDBModalTitle id="exampleModalScrollableTitle"> Modal title </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody> ... long content </MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="exampleModalScrollable = false"> Close </MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
},
setup() {
const exampleModalLong = ref(false);
const exampleModalScrollable = ref(false);
return {
exampleModalScrollable,
exampleModalLong,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const exampleModalLong = ref(false);
const exampleModalScrollable = ref(false);
</script>
Vertically centered
Add centered
to MDBModal
to vertically center the modal.
<template>
<MDBBtn
color="primary"
aria-controls="exampleModalCenter"
@click="exampleModalCenter = true"
>
Vertically centered modal
</MDBBtn>
<MDBModal
id="exampleModalCenter"
tabindex="-1"
labelledby="exampleModalCenterTitle"
v-model="exampleModalCenter"
centered
>
<MDBModalHeader>
<MDBModalTitle id="exampleModalCenterTitle"> Modal title </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>
<p>
Cras mattis consectetur purus sit amet fermentum. Cras justo odio, dapibus ac
facilisis in, egestas eget quam. Morbi leo risus, porta ac consectetur ac,
vestibulum at eros.
</p>
</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="exampleModalCenter = false"> Close </MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
<MDBBtn
color="primary"
aria-controls="exampleModalCenteredScrollable"
@click="exampleModalCenteredScrollable = true"
>
Vertically centered scrollable modal
</MDBBtn>
<MDBModal
id="exampleModalCenteredScrollable"
tabindex="-1"
labelledby="exampleModalCenteredScrollableTitle"
v-model="exampleModalCenteredScrollable"
centered
scrollable
>
<MDBModalHeader>
<MDBModalTitle id="exampleModalCenteredScrollableTitle">
Modal title
</MDBModalTitle>
</MDBModalHeader>
<MDBModalBody> ... long content </MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="exampleModalCenteredScrollable = false">
Close
</MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
},
setup() {
const exampleModalCenteredScrollable = ref(false);
return {
exampleModalCenteredScrollable,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const exampleModalCenteredScrollable = ref(false);
</script>
Tooltips and popovers
Tooltips and
popovers can be placed within modals as
needed. You can add function on hide
event to manually close any opened popover
or tooltip when closing modal.
<template>
<MDBBtn
color="primary"
aria-controls="exampleModalPopovers"
@click="exampleModalPopovers = true"
>
Launch demo modal
</MDBBtn>
<MDBModal
id="exampleModalPopovers"
tabindex="-1"
labelledby="exampleModalPopoversLabel"
v-model="exampleModalPopovers"
@hide="
() => {
popover1 = false;
tooltip1 = false;
}
"
>
<MDBModalHeader>
<MDBModalTitle id="exampleModalPopoversLabel"> Modal title </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>
<h5>Popover in a modal</h5>
<p>
This
<MDBPopover v-model="popover1" direction="right">
<template #reference>
<MDBBtn color="secondary" size="lg" @click="popover1 = !popover1"
>button</MDBBtn
>
</template>
<template #header>Popover title</template>
<template #body>Popover body content is set in this attribute.</template>
</MDBPopover>
triggers a popover on click.
</p>
<hr />
<h5>Tooltips in a modal</h5>
<p>
<MDBTooltip v-model="tooltip1">
<template #reference><a href="#">This link</a></template>
<template #tip> Tooltip </template>
</MDBTooltip>
and
<MDBTooltip v-model="tooltip2">
<template #reference><a href="#">that link</a></template>
<template #tip> Tooltip </template>
</MDBTooltip>
have tooltips on hover.
</p></MDBModalBody
>
<MDBModalFooter>
<MDBBtn color="secondary" @click="exampleModalPopovers = false"> Close </MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBPopover,
MDBTooltip,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBPopover,
MDBTooltip,
},
setup() {
const exampleModalPopovers = ref(false);
const popover1 = ref(false);
const tooltip1 = ref(false);
const tooltip2 = ref(false);
return {
exampleModalPopovers,
popover1,
tooltip1,
tooltip2,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBPopover,
MDBTooltip,
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const exampleModalPopovers = ref(false);
const popover1 = ref(false);
const tooltip1 = ref(false);
const tooltip2 = ref(false);
</script>
Using the grid
Utilize the Bootstrap grid system within a modal by nesting
MDBContainer fluid{
within the MDBModalBody{
. Then, use the normal
grid system properties/classes as you would anywhere else.
<template>
<MDBBtn color="primary" aria-controls="gridSystemModal" @click="gridSystemModal = true">
Launch demo modal
</MDBBtn>
<MDBModal
id="gridSystemModal"
tabindex="-1"
labelledby="gridModalLabel"
v-model="gridSystemModal"
>
<MDBModalHeader>
<MDBModalTitle id="gridModalLabel"> Grids in modals </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>
<MDBContainer fluid class="bd-example-row">
<MDBRow>
<MDBCol md="4" class="col-example">md="4"</MDBCol>
<MDBCol md="4" class="ms-auto col-example"> md="4" .ms-auto </MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md="3" class="ms-auto col-example"> md="3" .ms-auto </MDBCol>
<MDBCol md="2" class="ms-auto col-example"> md="2" .ms-auto </MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md="6" class="ms-auto col-example"> md="6" .ms-auto </MDBCol>
</MDBRow>
<MDBRow>
<MDBCol sm="9" class="col-example">
Level 1: sm="9"
<MDBRow>
<MDBCol col="8" sm="6" class="col-example">
Level 2: col="8" sm="6"
</MDBCol>
<MDBCol col="4" sm="6" class="col-example">
Level 2: col="4" sm="6"
</MDBCol>
</MDBRow>
</MDBCol>
</MDBRow>
</MDBContainer>
</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="gridSystemModal = false"> Close </MDBBtn>
<MDBBtn color="primary"> Save changes </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBRow,
MDBCol,
MDBContainer
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBRow,
MDBCol,
MDBContainer
},
setup() {
const gridSystemModal = ref(false);
return {
gridSystemModal,
};
},
};
</script>
<script setup lang="ts">
import {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn,
MDBRow,
MDBCol,
MDBContainer
} from 'mdb-vue-ui-kit';
import { ref } from 'vue';
const gridSystemModal = ref(false);
</script>
Varying modal content
Have a bunch of buttons that all trigger the same modal with slightly different contents? Use
Vue
refs
to vary the contents of the modal depending on which button was clicked.
Below is a live demo followed by example Vue template and script.
<template>
<MDBBtn
color="primary"
aria-controls="varyingExampleModal"
@click="
() => {
modalContent = '@mdo';
varyingExampleModal = true;
}
"
>
Open modal for @mdo
</MDBBtn>
<MDBBtn
color="primary"
aria-controls="varyingExampleModal"
@click="
() => {
modalContent = '@fat';
varyingExampleModal = true;
}
"
>
Open modal for @fat
</MDBBtn>
<MDBBtn
color="primary"
aria-controls="varyingExampleModal"
@click="
() => {
modalContent = '@getbootstrap';
varyingExampleModal = true;
}
"
>
Open modal for @getbootstrap
</MDBBtn>
<MDBModal
id="varyingExampleModal"
tabindex="-1"
labelledby="varyingExampleModalLabel"
v-model="varyingExampleModal"
>
<MDBModalHeader>
<MDBModalTitle id="varyingExampleModalLabel"> New message </MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>
<form>
<div class="mb-3">
<label for="recipient-name" class="col-form-label">Recipient: </label>
<input
type="text"
class="form-control"
id="recipient-name"
:value="modalContent"
/>
</div>
<div class="mb-3">
<label for="message-text" class="col-form-label">Message:</label>
<textarea class="form-control" id="message-text"></textarea>
</div>
</form>
</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="secondary" @click="varyingExampleModal = false"> Close </MDBBtn>
<MDBBtn color="primary"> Send message </MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import { MDBModal, MDBModalHeader, MDBModalTitle, MDBModalBody, MDBModalFooter, MDBBtn } from "mdb-vue-ui-kit";
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn
},
setup() {
const varyingExampleModal = ref(false);
const modalContent = ref('');
return {
varyingExampleModal,
modalContent,
}
}
};
</script>
<script setup lang="ts">
import { MDBModal, MDBModalHeader, MDBModalTitle, MDBModalBody, MDBModalFooter, MDBBtn } from "mdb-vue-ui-kit";
import { ref } from 'vue';
const varyingExampleModal = ref(false);
const modalContent = ref('');
</script>
Toggle between modals
Toggle between multiple modals with some clever placement of the
data-mdb-target
and data-mdb-toggle
attributes. For example, you
could toggle a password reset modal from within an already open sign in modal.
Please note multiple modals cannot be open at the same time—this method
simply toggles between two separate modals.
Below is a live demo followed by example HTML and JavaScript. For more information, read the
modal API/events doc for details on relatedTarget
.
<template>
<MDBBtn color="primary" @click="exampleModalToggle1 = true">Open first modal</MDBBtn>
<MDBModal id="exampleModalToggle1" tabindex="-1" labelledby="exampleModalToggle1Label" v-model="exampleModalToggle1">
<MDBModalHeader>
<MDBModalTitle id="exampleModalToggle1Label">
Modal 1</MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>Show a second modal and hide this one with the button
below.</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="primary" @click="openSecondModal">Open second modal</MDBBtn>
</MDBModalFooter>
</MDBModal>
<MDBModal id="exampleModalToggle2" tabindex="-1" labelledby="exampleModalToggle2Label" v-model="exampleModalToggle2">
<MDBModalHeader>
<MDBModalTitle id="exampleModalToggle2Label">
Modal 1</MDBModalTitle>
</MDBModalHeader>
<MDBModalBody>Hide this modal and show the first with the button
below.</MDBModalBody>
<MDBModalFooter>
<MDBBtn color="primary" @click="openFirstModal">Back to first</MDBBtn>
</MDBModalFooter>
</MDBModal>
</template>
<script>
import { MDBModal, MDBModalHeader, MDBModalTitle, MDBModalBody, MDBModalFooter, MDBBtn } from "mdb-vue-ui-kit";
import { ref } from 'vue';
export default {
components: {
MDBModal,
MDBModalHeader,
MDBModalTitle,
MDBModalBody,
MDBModalFooter,
MDBBtn
},
setup() {
const exampleModalToggle1 = ref(false);
const exampleModalToggle2 = ref(false);
const openSecondModal = () => {
exampleModalToggle1.value = false;
setTimeout(() => {
exampleModalToggle2.value = true;
}, 200);
};
const openFirstModal = () => {
exampleModalToggle2.value = false;
setTimeout(() => {
exampleModalToggle1.value = true;
}, 200);
};
return {
exampleModalToggle1,
exampleModalToggle2,
openSecondModal,
openFirstModal
}
}
};
</script>
<script setup lang="ts">
import { MDBModal, MDBModalHeader, MDBModalTitle, MDBModalBody, MDBModalFooter, MDBBtn } from "mdb-vue-ui-kit";
import { ref } from 'vue';
const exampleModalToggle1 = ref(false);
const exampleModalToggle2 = ref(false);
const openSecondModal = () => {
exampleModalToggle1.value = false;
setTimeout(() => {
exampleModalToggle2.value = true;
}, 200);
};
const openFirstModal = () => {
exampleModalToggle2.value = false;
setTimeout(() => {
exampleModalToggle1.value = true;
}, 200);
};
</script>
Change animation
The $modal-fade-transform
variable determines the transform state of
modal dialog
content before the modal fade-in animation, the
$modal-show-transform
variable determines the transform of
.modal-dialog
at the end of the modal fade-in animation.
If you want for example a zoom-in animation, you can set
$modal-fade-transform: scale(.8)
.
Remove animation
For modals that simply appear rather than fade in to view, add the
:animation="false"
property to your modal markup.
<template>
<MDBModal tabindex="-1" labelledby="..." v-model="..." :animation="false" />
</template>
Accessibility
Be sure to add labelledby="..."
>, referencing the modal title, to
MDBModal
>. Additionally, you may give a description of your modal dialog with
aria-describedby
> on MDBModal
>. Note that you don’t need to add
role="dialog"
> since we already add it inside component.
Embedding YouTube videos
Embedding YouTube videos in modals requires additional JavaScript not in Bootstrap to automatically stop playback and more. See this helpful Stack Overflow post for more information.
Optional sizes
Modals have three optional sizes, available via modifier properties to be placed on a
MDBModal
. These sizes kick in at certain breakpoints to avoid horizontal
scrollbars on narrower viewports.
Size | Property | Modal max-width |
---|---|---|
Small | size="sm" |
300px |
Default | None | 500px |
Large | size="lg" |
800px |
Extra large | size="xl" |
1140px |
<template>
<MDBModal size="xl">...</MDBModal>
<MDBModal size="lg">...</MDBModal>
<MDBModal size="sm">...</MDBModal>
</template>
Fullscreen Modal
Another override is the option to pop up a modal that covers the user viewport, available via
fullscreen
properties that are placed on a MDBModal
.
Class | Availability |
---|---|
fullscreen |
Always |
fullscreen="sm-down" |
Below 576px |
fullscreen="md-down" |
Below 768px |
fullscreen="lg-down" |
Below 992px |
fullscreen="xl-down" |
Below 1200px |
fullscreen="xxl-down" |
Below 1400px |
<!-- Full screen modal -->
<template>
<MDBModal fullscreen>...</MDBModal>
<MDBModal fullscreen="sm-down">...</MDBModal>
<MDBModal fullscreen="md-down">...</MDBModal>
<MDBModal fullscreen="lg-down">...</MDBModal>
<MDBModal fullscreen="xl-down">...</MDBModal>
<MDBModal fullscreen="xxl-down">...</MDBModal>
</template>
Non-Invasive Modal
This type of modal does not block any interaction on the page.
Simply add property nonInvasive
to MDBModal
.
<!-- Non-invasive modal -->
<template>
<MDBModal nonInvasive>...</MDBModal>
</template>
Modal - API
Import
<script>
import {
MDBModal,
MDBModalBody,
MDBModalHeader,
MDBModalTitle,
MDBModalFooter
} from 'mdb-vue-ui-kit';
</script>
Properties
Modal
Name | Type | Default | Description | Example |
---|---|---|---|---|
animation
|
Boolean | true |
Turn modal animation on or off |
<MDBModal :animation="false" />
|
bgSrc
|
String | -- |
Dark style necessitate having a picture background, but the feature is for all the modals, really |
<MDBModal bgSrc="/image/src" />
|
centered
|
Boolean | false |
Center modal vertically and horizonally |
<MDBModal centered />
|
duration
|
Number | 400 |
Sets animation duration in miliseconds |
<MDBModal :duration="500" />
|
focus
|
Boolean | true |
Puts the focus on the modal when initialized. |
<MDBModal :focus="false" />
|
fullscreen
|
Number | 400 |
Makes the modal cover user viewport on a specific breakpoint |
<MDBModal :fullscreen="lg-down" />
|
keyboard
|
Boolean | true |
Closes the modal when escape key is pressed |
<MDBModal :keyboard="false" />
|
labelledby
|
String | -- |
When having modal content with the title add labelledby to make modal
more accessible
|
<MDBModal labelledby="modalExampleTitle">
<MDBModalHeader>
<MDBModalTitle tag="h4" id="modalExampleTitle">
Modal title
</MDBModalTitle>
</MDBModalHeader>
</MDBModal>
|
nonInvasive
|
Boolean | false |
Removes the dark overlay from the background, allows to scroll through page and allows to open another modal while non-invasive modal is open. |
<MDBModal nonInvasive />
|
removeBackdrop
|
Boolean | false |
Removes the dark overlay from the background, along its functionality |
<MDBModal removeBackdrop />
|
scrollable
|
Boolean | false |
Allows to scroll through long contents with a scrollbar |
<MDBModal scrollable />
|
size
|
String | -- |
Sets size of MDBModal component. Use "sm | lg | xl" values |
<MDBModal size="sm" /> |
staticBackdrop
|
Boolean | false |
Will prevent modal close when clicking outside the backdrop |
<MDBModal staticBackdrop />
|
tag
|
String | 'div' |
Defines tag of the MDBModal element |
<MDBModal tag="span" />
|
v-model
|
String | false |
Opens / closes modal component |
<MDBModal v-model="true" />
|
Modal Header
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBModalHeader element |
<MDBModalHeader tag="header" />
|
close
|
Boolean | true |
Turning it to false removes the close "x" icon in the right top corner |
<MDBModalHeader :close="false" />
|
closeWhite
|
Boolean | false |
Makes the closing "x" sign works better with dark variant of the modal |
<MDBModalHeader :closeWhite />
|
color
|
String | -- |
Determines color of the header |
<MDBModalHeader color="secondary" />
|
Modal Title
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'h5' |
Defines tag of the MDBModalTitle element |
<MDBModalTitle tag="h6" />
|
bold
|
Boolean | false |
Makes title font bold |
<MDBModalTitle bold />
|
Events
Bootstrap’s modal class exposes a few events for hooking into modal functionality. All modal
events are fired at the modal itself (i.e. at the
<div class="modal">
).
Event type | Description |
---|---|
show |
This event fires immediately when the modal has been called to show. |
shown |
This event is fired when the modal has been made visible to the user (will wait for CSS transitions to complete). |
hide |
This event is fired immediately when the modal has been called to hide. |
hidden |
This event is fired when the modal has finished being hidden from the user (will wait for CSS transitions to complete). |
<template>
<MDBModal v-model="exampleModal" @show="doSomething">...</MDBModal>
</template>
CSS variables
As part of MDB’s evolving CSS variables approach, modal now use local CSS variables on .modal
for
enhanced real-time customization. Values for the CSS variables are set via Sass, so Sass customization is still
supported, too.
Modal's CSS variables are in different classes which belong to this component. To make it easier to use them, you can find below all of the used CSS variables.
// Variables for modal free
// .modal
--#{$prefix}modal-zindex: #{$zindex-modal};
--#{$prefix}modal-width: #{$modal-md};
--#{$prefix}modal-padding: #{$modal-inner-padding};
--#{$prefix}modal-margin: #{$modal-dialog-margin};
--#{$prefix}modal-color: #{$modal-content-color};
--#{$prefix}modal-bg: #{$modal-content-bg};
--#{$prefix}modal-border-color: #{$modal-content-border-color};
--#{$prefix}modal-border-width: #{$modal-content-border-width};
--#{$prefix}modal-border-radius: #{$modal-content-border-radius};
--#{$prefix}modal-box-shadow: #{$modal-content-box-shadow-xs};
--#{$prefix}modal-inner-border-radius: #{$modal-content-inner-border-radius};
--#{$prefix}modal-header-padding-x: #{$modal-header-padding-x};
--#{$prefix}modal-header-padding-y: #{$modal-header-padding-y};
--#{$prefix}modal-header-padding: #{$modal-header-padding}; // Todo in v6: Split this padding into x and y
--#{$prefix}modal-header-border-color: #{$modal-header-border-color};
--#{$prefix}modal-header-border-width: #{$modal-header-border-width};
--#{$prefix}modal-title-line-height: #{$modal-title-line-height};
--#{$prefix}modal-footer-gap: #{$modal-footer-margin-between};
--#{$prefix}modal-footer-bg: #{$modal-footer-bg};
--#{$prefix}modal-footer-border-color: #{$modal-footer-border-color};
--#{$prefix}modal-footer-border-width: #{$modal-footer-border-width};
// .modal-backdrop
--#{$prefix}backdrop-zindex: #{$zindex-modal-backdrop};
--#{$prefix}backdrop-bg: #{$modal-backdrop-bg};
--#{$prefix}backdrop-opacity: #{$modal-backdrop-opacity};
// .modal
--#{$prefix}modal-margin: #{$modal-dialog-margin-y-sm-up};
--#{$prefix}modal-box-shadow: #{$modal-content-box-shadow-sm-up};
// .modal-sm
--#{$prefix}modal-width: #{$modal-sm};
// .modal-lg,
// .modal-xl
--#{$prefix}modal-width: #{$modal-lg};
// .modal-xl
--#{$prefix}modal-width: #{$modal-xl};
// .modal-content
--#{$prefix}modal-box-shadow: #{$modal-box-shadow};
// Variables for modal pro
// .modal
--#{$prefix}modal-top-left-top: #{$modal-top-left-top};
--#{$prefix}modal-top-left-left: #{$modal-top-left-left};
--#{$prefix}modal-top-right-top: #{$modal-top-right-top};
--#{$prefix}modal-top-right-right: #{$modal-top-right-right};
--#{$prefix}modal-bottom-left-bottom: #{$modal-bottom-left-bottom};
--#{$prefix}modal-bottom-left-left: #{$modal-bottom-left-left};
--#{$prefix}modal-bottom-right-bottom: #{$modal-bottom-right-bottom};
--#{$prefix}modal-bottom-right-right: #{$modal-bottom-right-right};
--#{$prefix}modal-fade-top-transform: #{$modal-fade-top-transform};
--#{$prefix}modal-fade-right-transform: #{$modal-fade-right-transform};
--#{$prefix}modal-fade-bottom-transform: #{$modal-fade-bottom-transform};
--#{$prefix}modal-fade-left-transform: #{$modal-fade-left-transform};
--#{$prefix}modal-side-right: #{$modal-side-right};
--#{$prefix}modal-side-bottom: #{$modal-side-bottom};
--#{$prefix}modal-non-invasive-box-shadow: #{$modal-non-invasive-box-shadow};
--#{$prefix}modal-non-invasive-box-shadow-top: #{$modal-non-invasive-box-shadow-top};
SCSS variables
// Variables for modal free
$modal-inner-padding: $spacer;
$modal-footer-margin-between: 0.5rem;
$modal-dialog-margin: 0.5rem;
$modal-dialog-margin-y-sm-up: 1.75rem;
$modal-title-line-height: $line-height-base;
$modal-content-color: null;
$modal-content-bg: $white;
$modal-content-border-color: var(--#{$prefix}border-color-translucent);
$modal-content-border-width: $border-width;
$modal-content-inner-border-radius: subtract(
$modal-content-border-radius,
$modal-content-border-width
);
$modal-content-box-shadow-xs: $box-shadow-sm;
$modal-content-box-shadow-sm-up: $box-shadow;
$modal-backdrop-bg: $black;
$modal-backdrop-opacity: 0.5;
$modal-header-padding-y: $modal-inner-padding;
$modal-header-padding-x: $modal-inner-padding;
$modal-header-padding: $modal-header-padding-y $modal-header-padding-x; // Keep this for backwards compatibility
$modal-footer-bg: null;
$modal-sm: 300px;
$modal-md: 500px;
$modal-lg: 800px;
$modal-xl: 1140px;
$modal-fade-transform: translate(0, -50px);
$modal-show-transform: none;
$modal-transition: transform 0.3s ease-out;
$modal-scale-transform: scale(1.02);
$modal-box-shadow: $box-shadow-4;
$modal-content-border-radius: $border-radius-lg;
$modal-header-border-color: $border-color-alternate;
$modal-footer-border-color: $border-color-alternate;
$modal-header-border-width: $border-width-alternate;
$modal-footer-border-width: $border-width-alternate;
// Variables for modal pro
$modal-top-left-top: 10px;
$modal-top-left-left: 10px;
$modal-top-right-top: 10px;
$modal-top-right-right: 10px;
$modal-bottom-left-bottom: 10px;
$modal-bottom-left-left: 10px;
$modal-bottom-right-bottom: 10px;
$modal-bottom-right-right: 10px;
$modal-fade-top-transform: translate3d(0, -25%, 0);
$modal-fade-right-transform: translate3d(25%, 0, 0);
$modal-fade-bottom-transform: translate3d(0, 25%, 0);
$modal-fade-left-transform: translate3d(-25%, 0, 0);
$modal-side-right: 10px;
$modal-side-bottom: 10px;
$modal-non-invasive-box-shadow: $box-shadow-3;
$modal-non-invasive-box-shadow-top: $box-shadow-3-top;