Material Design
for Bootstrap 5 & Angular 17
- 700+ UI components & templates
- Super simple, 1 minute installation
- Easy theming and customization
- MIT license - free for personal & commercial use
Trusted by 3,000,000+ developers and designers
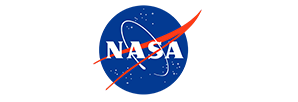
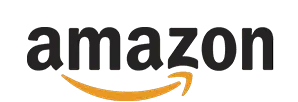
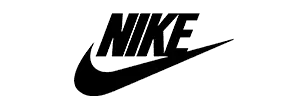
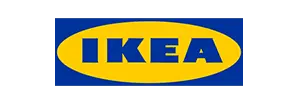
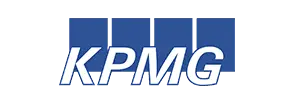
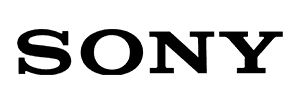
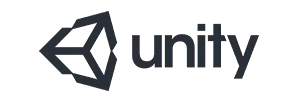
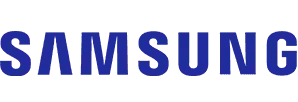
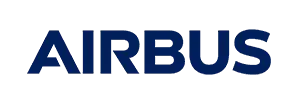
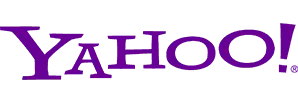
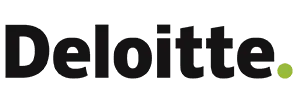
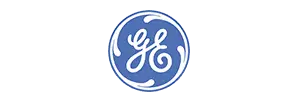