Sticky
React Bootstrap 5 Sticky
Sticky element can be locked in a particular area of the page. It is often used in navigation menus.
Note: Read the API tab to find all available options and advanced customization
Basic example
To start use sticky just create MDBSticky
component or useStickyRef
hook
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
boundary
tag='a'
className='btn btn-outline-primary bg-white'
href='https://mdbootstrap.com/docs/jquery/getting-started/download/'
>
Download MDB
</MDBSticky>
);
}
import React from 'react';
import { useStickyRef } from 'mdb-react-ui-kit';
export default function App() {
const basicExampleRef = useStickyRef({ boundary: true })
return (
<a
ref={basicExampleRef}
className='btn btn-outline-primary bg-white'
href='https://mdbootstrap.com/docs/jquery/getting-started/download/'
>
Download MDB
</a>
);
}
Sticky bottom
You can pin the element to the bottom by passing
position="bottom"
as a prop
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
boundary
position='bottom'
direction='both'
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
import React from 'react';
import { useStickyRef } from 'mdb-react-ui-kit';
export default function App() {
const stickyBottomExampleRef = useStickyRef({
boundary: true,
position: 'bottom',
direction: 'both'
})
return (
<img
ref={stickyBottomExampleRef}
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
Boundary
Set boundary
so that sticky only works inside the parent
element. Remember to set the correct parent height.
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
boundary
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
import React from 'react';
import { useStickyRef } from 'mdb-react-ui-kit';
export default function App() {
const boundaryExampleRef = useStickyRef({ boundary: true });
return (
<img
ref={boundaryExampleRef}
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
Outer element as a boundary
You can specify an element reference to be the sticky boundary by passing
boundary={elementReference}
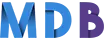
import React, { useRef } from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
const boundaryRef = useRef(null);
return (
<>
<div>
<MDBSticky
boundary={boundaryRef}
direction='both'
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
</div>
<div ref={boundaryRef} className='mt-auto' style={{ height: '5rem' }}>
Stop here
</div>
</>
);
}
import React, { useRef } from 'react';
import { useStickyRef } from 'mdb-react-ui-kit';
export default function App() {
const boundaryRef = useRef(null);
const outerBoundaryExampleRef = useStickyRef({ boundary: boundaryRef, direction: 'both' });
return (
<>
<div>
<img
ref={outerBoundaryExampleRef}
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
</div>
<div id='sticky-stop' className='mt-auto' style={{ height: '5rem' }}>
Stop here
</div>
</>
);
}
Direction
Default scrolling direction is down
. You can change it by setting
direction="top"
or
direction="both"
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<>
<MDBSticky boundary tag='a' className='btn btn btn-primary' direction='up'>
Up
</MDBSticky>
<MDBSticky boundary tag='a' className='btn btn btn-primary' direction='down'>
Down
</MDBSticky>
<MDBSticky boundary tag='a' className='btn btn btn-primary' direction='both'>
Both
</MDBSticky>
</>
);
}
import React from 'react';
import { useStickyRef } from 'mdb-react-ui-kit';
export default function App() {
const directionUpExampleRef = useStickyRef({ boundary: true, direction: 'up' });
const directionDownExampleRef = useStickyRef({ boundary: true, direction: 'down' });
const directionBothExampleRef = useStickyRef({ boundary: true, direction: 'both' });
return (
<>
<a ref={directionUpExampleRef} className='btn btn btn-primary' >
Up
</a>
<a ref={directionDownExampleRef} className='btn btn btn-primary' >
Down
</a>
<a ref={directionBothExampleRef} className='btn btn btn-primary' >
Both
</a>
</>
);
}
Animation
You can add an animation that will run when the sticky starts and when the sticky element is
hidden. Just specify the CSS class of the animation using the
animationSticky
and
animationUnsticky
properties.
Remember that not every animation will be appropriate. We suggest using the animations used in the example below.
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
id='MDB-logo'
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
animationSticky='slide-in-down'
animationUnsticky='slide-up'
boundary
/>
);
}
import React from 'react';
import { useStickyRef } from 'mdb-react-ui-kit';
export default function App() {
const animationExampleRef = useStickyRef({
boundary: true,
animationSticky: 'slide-in-down',
animationUnsticky: 'slide-up',
});
return (
<img
ref={animationExampleRef}
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
Sticky - API
Import
import { MDBSticky } from 'mdb-react-ui-kit';
import { useStickyRef } from 'mdb-react-ui-kit';
Properties
MDBSticky
Name | Type | Default | Description | Example |
---|---|---|---|---|
animationSticky
|
'fade-in' | 'fade-in-down' | 'fade-in-left' | 'fade-in-right' | 'fade-in-up' | 'fade-out' | 'fade-out-down' | 'fade-out-left' | 'fade-out-right' | 'fade-out-up' | 'slide-in-down' | 'slide-in-left' | 'slide-in-right' | 'slide-in-up' | 'slide-out-down' | 'slide-out-left' | 'slide-out-right' | 'slide-out-up' | 'slide-down' | 'slide-left' | 'slide-right' | 'slide-up' | 'zoom-in' | 'zoom-out' | 'tada' | 'pulse' | 'drop-in' | 'drop-out' | 'fly-in' | 'fly-in-up' | 'fly-in-down' | 'fly-in-left' | 'fly-in-right' | 'fly-out' | 'fly-out-up' | 'fly-out-down' | 'fly-out-left' | 'fly-out-right' | 'browse-in' | 'browse-out' | 'browse-out-left' | 'browse-out-right' | 'jiggle' | 'flash' | 'shake' | 'glow' | '' |
Set sticky animation |
<MDBSticky animationSticky="fade-in" />
|
animationUnsticky
|
'fade-in' | 'fade-in-down' | 'fade-in-left' | 'fade-in-right' | 'fade-in-up' | 'fade-out' | 'fade-out-down' | 'fade-out-left' | 'fade-out-right' | 'fade-out-up' | 'slide-in-down' | 'slide-in-left' | 'slide-in-right' | 'slide-in-up' | 'slide-out-down' | 'slide-out-left' | 'slide-out-right' | 'slide-out-up' | 'slide-down' | 'slide-left' | 'slide-right' | 'slide-up' | 'zoom-in' | 'zoom-out' | 'tada' | 'pulse' | 'drop-in' | 'drop-out' | 'fly-in' | 'fly-in-up' | 'fly-in-down' | 'fly-in-left' | 'fly-in-right' | 'fly-out' | 'fly-out-up' | 'fly-out-down' | 'fly-out-left' | 'fly-out-right' | 'browse-in' | 'browse-out' | 'browse-out-left' | 'browse-out-right' | 'jiggle' | 'flash' | 'shake' | 'glow' | '' |
Set unsticky animation |
<MDBSticky animationUnsticky="fade-out" />
|
boundary
|
boolean | false |
Set to true to stop sticky on the end of the parent |
<MDBSticky boundary />
|
delay
|
number | 0 |
Set the number of pixels beyond which the item will be pinned |
<MDBSticky delay={100} />
|
direction
|
'up' | 'down' | 'both' | 'down' |
Set the scrolling direction for which the element is to be stikcky |
<MDBSticky direction='up' />
|
offset
|
Number | 0 |
Set the distance from the top edge of the element for which the element is sticky |
<MDBSticky offset={100} />
|
position
|
'top' | 'bottom' | 'top' |
Set the edge of the screen the item should stick to |
<MDBSticky position="down" />
|
stickyRef
|
React.RefObject | '' |
Sets a reference for the MDBSticky element. |
<MDBSticky stickyRef={exampleRef} />
|
useStickyRef
Name | Type | Default | Description | Example |
---|---|---|---|---|
animationSticky
|
'fade-in' | 'fade-in-down' | 'fade-in-left' | 'fade-in-right' | 'fade-in-up' | 'fade-out' | 'fade-out-down' | 'fade-out-left' | 'fade-out-right' | 'fade-out-up' | 'slide-in-down' | 'slide-in-left' | 'slide-in-right' | 'slide-in-up' | 'slide-out-down' | 'slide-out-left' | 'slide-out-right' | 'slide-out-up' | 'slide-down' | 'slide-left' | 'slide-right' | 'slide-up' | 'zoom-in' | 'zoom-out' | 'tada' | 'pulse' | 'drop-in' | 'drop-out' | 'fly-in' | 'fly-in-up' | 'fly-in-down' | 'fly-in-left' | 'fly-in-right' | 'fly-out' | 'fly-out-up' | 'fly-out-down' | 'fly-out-left' | 'fly-out-right' | 'browse-in' | 'browse-out' | 'browse-out-left' | 'browse-out-right' | 'jiggle' | 'flash' | 'shake' | 'glow' | '' |
Set sticky animation |
useStickyRef({ animationSticky: 'fade-in' })
|
animationUnsticky
|
'fade-in' | 'fade-in-down' | 'fade-in-left' | 'fade-in-right' | 'fade-in-up' | 'fade-out' | 'fade-out-down' | 'fade-out-left' | 'fade-out-right' | 'fade-out-up' | 'slide-in-down' | 'slide-in-left' | 'slide-in-right' | 'slide-in-up' | 'slide-out-down' | 'slide-out-left' | 'slide-out-right' | 'slide-out-up' | 'slide-down' | 'slide-left' | 'slide-right' | 'slide-up' | 'zoom-in' | 'zoom-out' | 'tada' | 'pulse' | 'drop-in' | 'drop-out' | 'fly-in' | 'fly-in-up' | 'fly-in-down' | 'fly-in-left' | 'fly-in-right' | 'fly-out' | 'fly-out-up' | 'fly-out-down' | 'fly-out-left' | 'fly-out-right' | 'browse-in' | 'browse-out' | 'browse-out-left' | 'browse-out-right' | 'jiggle' | 'flash' | 'shake' | 'glow' | '' |
Set unsticky animation |
useStickyRef({ animationUnsticky: 'fade-out' })
|
boundary
|
boolean | false |
Set to true to stop sticky on the end of the parent |
useStickyRef({ boundary: true })
|
delay
|
number | 0 |
Set the number of pixels beyond which the item will be pinned |
useStickyRef({ delay: 100 })
|
direction
|
'up' | 'down' | 'both' | 'down' |
Set the scrolling direction for which the element is to be stikcky |
useStickyRef({ direction: 'up' })
|
offset
|
Number | 0 |
Set the distance from the top edge of the element for which the element is sticky |
useStickyRef({ offset: 100 })
|
position
|
'top' | 'bottom' | 'top' |
Set the edge of the screen the item should stick to |
useStickyRef({ position: 'down' })
|
Events
MDBSticky
Name | Type | Description |
---|---|---|
onActive
|
() => any | This event fires immediately when the element is active |
onInactive
|
() => any | This event fires immediately when the element is inactive |