Position
React Bootstrap 5 Position component
Use these helpers for quickly configuring the position of an element.
Basic examples
Fixed top
Position an element at the top of the viewport, from edge to edge. Be sure you understand the ramifications of fixed position in your project; you may need to add additional CSS.
import React from 'react';
export default function App() {
return (
<div className="fixed-top">...</div>
);
}
Fixed bottom
Position an element at the bottom of the viewport, from edge to edge. Be sure you understand the ramifications of fixed position in your project; you may need to add additional CSS.
import React from 'react';
export default function App() {
return (
<div className="fixed-bottom">...</div>
);
}
Sticky top
Position an element at the top of the viewport, from edge to edge, but only after you scroll
past it. The .sticky-top
utility uses CSS’s position: sticky
, which
isn’t fully supported in all browsers.
import React from 'react';
export default function App() {
return (
<div className="sticky-top">...</div>
);
}
Responsive sticky top
Responsive variations also exist for .sticky-top
utility.
import React from 'react';
export default function App() {
return (
<>
<div className="sticky-sm-top">Stick to the top on viewports sized SM (small) or wider</div>
<div className="sticky-md-top">Stick to the top on viewports sized MD (medium) or wider</div>
<div className="sticky-lg-top">Stick to the top on viewports sized LG (large) or wider</div>
<div className="sticky-xl-top">Stick to the top on viewports sized XL (extra-large) or wider</div>
</>
);
}
Position values
Quick positioning classes are available, though they are not responsive.
import React from 'react';
export default function App() {
return (
<>
<div className="position-static">...</div>
<div className="position-relative">...</div>
<div className="position-absolute">...</div>
<div className="position-fixed">...</div>
<div className="position-sticky">...</div>
</>
);
}
Arrange elements
Arrange elements easily with the edge positioning utilities. The format is
{property}-{position}
.
Where property is one of:
top
- for the verticaltop
positionstart
- for the horizontalleft
position (in LTR)bottom
- for the verticalbottom
positionend
- for the horizontalright
position (in LTR)
Where position is one of:
0
- for0
edge position50
- for50%
edge position100
- for100%
edge position
You can add more position values by adding entries to the
$position-values
Sass map variable.
import React from 'react';
export default function App() {
return (
<div className="position-relative position-relative-example">
<div className="position-absolute top-0 start-0"></div>
<div className="position-absolute top-0 end-0"></div>
<div className="position-absolute top-50 start-50"></div>
<div className="position-absolute bottom-50 end-50"></div>
<div className="position-absolute bottom-0 start-0"></div>
<div className="position-absolute bottom-0 end-0"></div>
</div>
);
}
.position-relative-example {
height: 200px;
width: 100%;
background-color: #f5f5f5;
}
.position-relative-example div {
width: 2em;
height: 2em;
background-color: #343a40;
border-radius: 0.25rem;
}
Center elements
In addition, you can also center the elements with the transform utility class
.translate-middle
.
This class applies the transformations
translateX(-50%)
and translateY(-50%)
to the element which, in
combination with the edge positioning utilities, allows you to absolute center an element.
import React from 'react';
export default function App() {
return (
<div className="position-relative position-relative-example">
<div className="position-absolute top-0 start-0 translate-middle"></div>
<div className="position-absolute top-0 start-50 translate-middle"></div>
<div className="position-absolute top-0 start-100 translate-middle"></div>
<div className="position-absolute top-50 start-0 translate-middle"></div>
<div className="position-absolute top-50 start-50 translate-middle"></div>
<div className="position-absolute top-50 start-100 translate-middle"></div>
<div className="position-absolute top-100 start-0 translate-middle"></div>
<div className="position-absolute top-100 start-50 translate-middle"></div>
<div className="position-absolute top-100 start-100 translate-middle"></div>
</div>
);
}
.position-relative-example {
height: 200px;
width: 100%;
background-color: #f5f5f5;
}
.position-relative-example div {
width: 2em;
height: 2em;
background-color: #343a40;
border-radius: 0.25rem;
}
Additional examples
Here are some real life examples of these classes:
import React from 'react';
export default function App() {
return (
<>
<MDBBtn color="primary" className="position-relative"
>Mails
<span
className="position-absolute top-0 start-100 translate-middle badge rounded-pill bg-secondary px-1"
>
+99
<span className="visually-hidden">unread messages</span>
</span>
</MDBBtn>
<MDBBtn color="dark" className="position-relative">
Marker
<svg
width="1em"
height="1em"
viewBox="0 0 16 16"
className="position-absolute top-100 start-50 translate-middle bi bi-caret-down-fill"
fill="#343a40"
xmlns="http://www.w3.org/2000/svg"
>
<path
d="M7.247 11.14L2.451 5.658C1.885 5.013 2.345 4 3.204 4h9.592a1 1 0 0 1 .753 1.659l-4.796 5.48a1 1 0 0 1-1.506 0z"
/>
</svg>
</MDBBtn>
<MDBBtn color="primary" className="position-relative">
Alerts
<span
className="position-absolute top-0 start-100 translate-middle badge border border-light rounded-circle bg-danger p-2"
>
<span className="visually-hidden">unread messages</span>
</span>
</MDBBtn>
</>
);
}
You can use these classes with existing components to create new ones. Remember that you can
extend its functionality by adding entries to the $position-values
variable.
import React from 'react';
export default function App() {
return (
<div className="position-relative" style={{ width: "100%" }}>
<div className="progress" style={{ height: "1px" }}>
<div
className="progress-bar"
role="progressbar"
style={{ width: "50%" }}
aria-valuenow="25"
aria-valuemin="0"
aria-valuemax="100"
></div>
</div>
<MDBBtn
color="primary"
size="sm"
className="position-absolute top-0 start-0 translate-middle rounded-pill"
>
1
</MDBBtn>
<MDBBtn
color="primary"
size="sm"
className="position-absolute top-0 start-50 translate-middle rounded-pill"
>
2
</MDBBtn>
<MDBBtn
color="secondary"
size="sm"
className="position-absolute top-0 start-100 translate-middle rounded-pill"
>
3
</MDBBtn>
</div>
);
}
Object Fit
It's an utility for controlling how the content of the replaced element is resized.
Resize the content of an item to cover its container with .object-cover
.
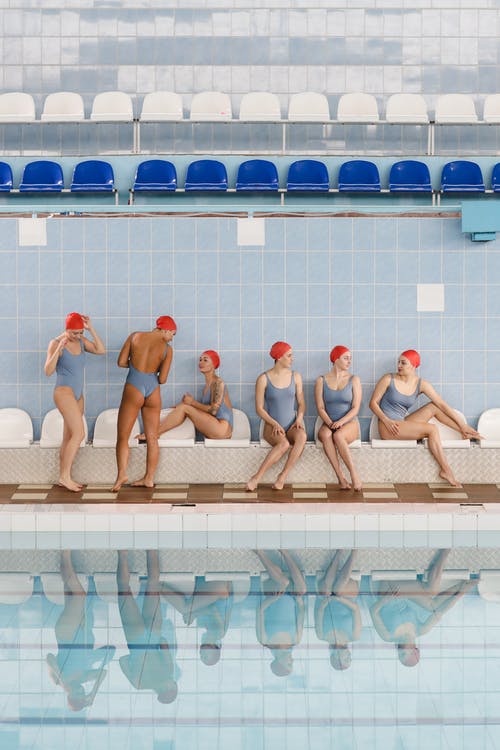
object-fit: cover;
import React from 'react';
export default function App() {
return (
<section className='border p-4 d-flex mb-4'>
<div className="row">
<div className='col-md-6'>
<div>
<img
src='https://mdbootstrap.com/img/new/blog/2.jpeg'
className='object-cover w-100'
style={{ height: '200px' }}
alt
/>
<p className='pt-3 mb-0 text-center'>object-fit: cover;</p>
</div>
</div>
</div>
</section>
);
}
Object Position
These are tools for controlling how the content of the replaced item should be placed in its container.
To specify how the contents of the replaced element should be placed in its container, use classes
.object-top
, .object-center
or .object-bottom
.
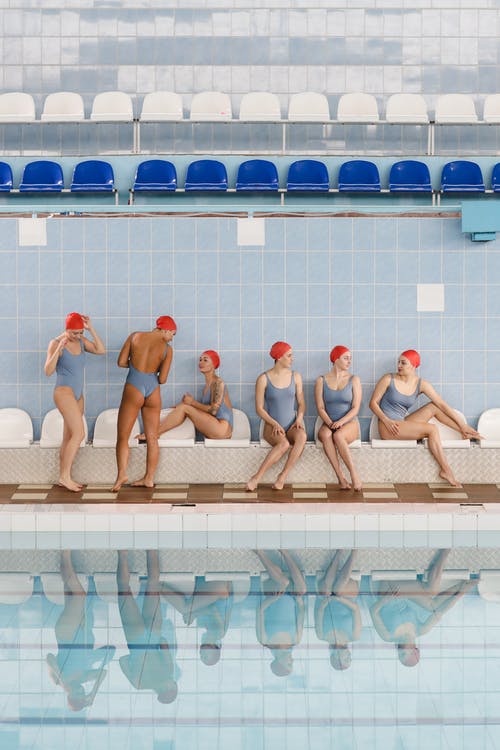
object-position: top;
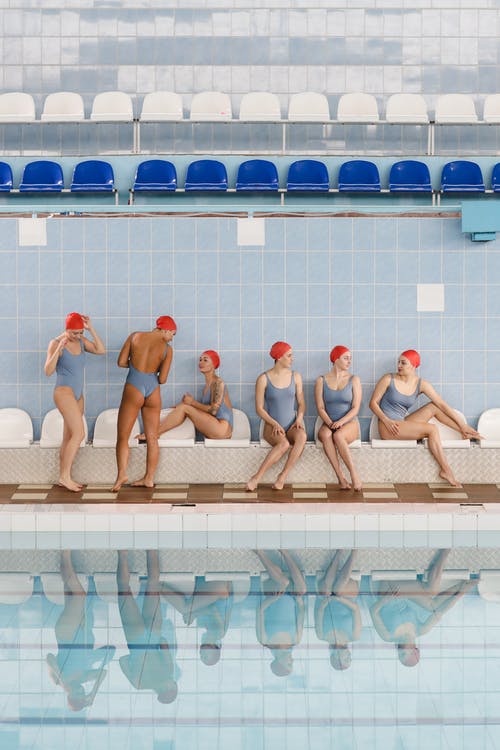
object-fit: center;
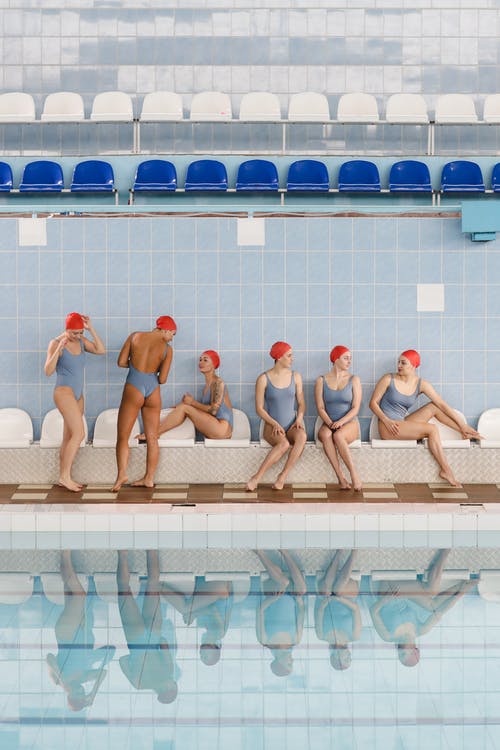
object-fit: bottom;
import React from 'react';
export default function App() {
return (
<section className=' p-4'>
<div class='row'>
<div className='col-md-4 px-2'>
<div>
<img
src='https://mdbootstrap.com/img/new/blog/2.jpeg'
className='object-cover w-100 object-top'
style={{ height: '250px' }}
alt
/>
<p className='pt-3 mb-0 text-center'>object-position: top;</p>
</div>
</div>
<div className='col-md-4 px-2'>
<div>
<img
src='https://mdbootstrap.com/img/new/blog/2.jpeg'
className='object-cover w-100 object-center'
style={{ height: '250px' }}
alt
/>
<p className='pt-3 mb-0 text-center'>object-fit: center;</p>
</div>
</div>
<div className='col-md-4 px-2'>
<div>
<img
src='https://mdbootstrap.com/img/new/blog/2.jpeg'
className='object-cover w-100 object-bottom'
style={{ height: '250px' }}
alt
/>
<p className='pt-3 mb-0 text-center'>object-fit: bottom;</p>
</div>
</div>
</div>
</section>
);
}