Address Form
React Bootstrap 5 Address Form component
Responsive address form built with React Bootstrap 5. Address form templates include basic examples, email validation, use of cards, use of photos & more.
Basic examples
Simple example of an address form on checkout. Visit the validation documentation in order to learn how to add email and phone number validation to specific input fields.
Billing details
Summary
- Products $53.98
- Shipping Gratis
-
Total amount$53.98
(including VAT)
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardHeader, MDBCheckbox, MDBCol, MDBInput, MDBListGroup, MDBListGroupItem, MDBRow, MDBTextArea, MDBTypography } from 'mdb-react-ui-kit';
export default function Basic() {
return (
<div className="mx-auto mt-5" style={{ maxWidth: '900px' }}>
<MDBRow>
<MDBCol md="8" className="mb-4">
<MDBCard className="mb-4">
<MDBCardHeader className="py-3">
<MDBTypography tag="h5" className="mb-0">Biling details</MDBTypography>
</MDBCardHeader>
<MDBCardBody>
<form>
<MDBRow className="mb-4">
<MDBCol>
<MDBInput label='First name' type='text' />
</MDBCol>
<MDBCol>
<MDBInput label='Last name' type='text' />
</MDBCol>
</MDBRow>
<MDBInput label='Company name' type='text' className="mb-4" />
<MDBInput label='Address' type='text' className="mb-4" />
<MDBInput label='Email' type='text' className="mb-4" />
<MDBInput label='Phone' type='text' className="mb-4" />
<MDBTextArea label='Additional information' rows={4} className="mb-4" />
<div className="d-flex justify-content-center">
<MDBCheckbox name='flexCheck' value='' id='flexCheckChecked' label='Create an account?' defaultChecked />
</div>
</form>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4" className="mb-4">
<MDBCard className="mb-4">
<MDBCardHeader className="py-3">
<MDBTypography tag="h5" className="mb-0">Summary</MDBTypography>
</MDBCardHeader>
<MDBCardBody>
<MDBListGroup flush>
<MDBListGroupItem className="d-flex justify-content-between align-items-center border-0 px-0 pb-0">
Products
<span>$53.98</span>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center px-0">
Shipping
<span>Gratis</span>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center border-0 px-0 mb-3">
<div>
<strong>Total amount</strong>
<strong>
<p className="mb-0">(including VAT)</p>
</strong>
</div>
<span><strong>$53.98</strong></span>
</MDBListGroupItem>
</MDBListGroup>
<MDBBtn size="lg" block>
Make purchase
</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</div>
);
}
Delivery address form card
You can embed an address form inside a Card. This example uses a background generated with our Gradient Generator and an irregular shaped card in order to make it more visually interesting.
We've also added animation on scroll to make the design more dynamic and highlight the speed of delivery.
Welcome
You are 30 seconds away from compleating your order!
Delivery Details
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCol, MDBIcon, MDBInput, MDBRow, MDBTypography } from 'mdb-react-ui-kit';
export default function Delivery() {
return (
<>
<div className="mx-auto gradient-custom mt-5" style={{ maxWidth: '800px', height: '400px' }}>
<MDBRow className="pt-3 mx-3">
<MDBCol md="3">
<div className="text-center" style={{ marginTop: '50px', marginLeft: '10px' }}>
<MDBIcon fas icon="shipping-fast text-white" size="3x" />
<MDBTypography tag="h3" className="text-white">Welcome</MDBTypography>
<p className="white-text">You are 30 seconds away from compleating your order!</p>
</div>
<div className="text-center">
<MDBBtn color="white" rounded className="back-button">Go back</MDBBtn>
</div>
</MDBCol>
<MDBCol md="9" className="justify-content-center">
<MDBCard className="card-custom pb-4">
<MDBCardBody className="mt-0 mx-5">
<div className="text-center mb-3 pb-2 mt-3">
<MDBTypography tag="h4" style={{ color: '#495057' }} >Delivery Details</MDBTypography>
</div>
<form className="mb-0">
<MDBRow className="mb-4">
<MDBCol>
<MDBInput label='First name' type='text' />
</MDBCol>
<MDBCol>
<MDBInput label='Last name' type='text' />
</MDBCol>
</MDBRow>
<MDBRow className="mb-4">
<MDBCol>
<MDBInput label='City' type='text' />
</MDBCol>
<MDBCol>
<MDBInput label='Zip' type='text' />
</MDBCol>
</MDBRow>
<MDBRow className="mb-4">
<MDBCol>
<MDBInput label='Address' type='text' />
</MDBCol>
<MDBCol>
<MDBInput label='Email' type='text' />
</MDBCol>
</MDBRow>
<div className="float-end">
<MDBBtn rounded style={{backgroundColor: '#0062CC'}}>Place order</MDBBtn>
</div>
</form>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</div>
</>
);
}
.gradient-custom {
background: -webkit-linear-gradient(left, #3931af, #00c6ff);
}
.card-custom {
border-bottom-left-radius: 10% 50%;
border-top-left-radius: 10% 50%;
background-color: #f8f9fa ;
}
.input-custom {
background-color: white ;
}
.white-text {
color: hsl(52, 0%, 98%);
font-weight: 100 ;
font-size: 14px;
}
.back-button {
background-color: hsl(52, 0%, 98%);
font-weight: 700;
color: black ;
margin-top: 50px ;
}
Advanced address form
With the use of MDB React components you can create a variety of simple & complex address forms.
Adding a Select menu with a country of delivery is one of the most common use cases. If you are planning to include the country list in your address form, you should also take a look at the list of Flags that can be easily included as icons.
Delivery Country:
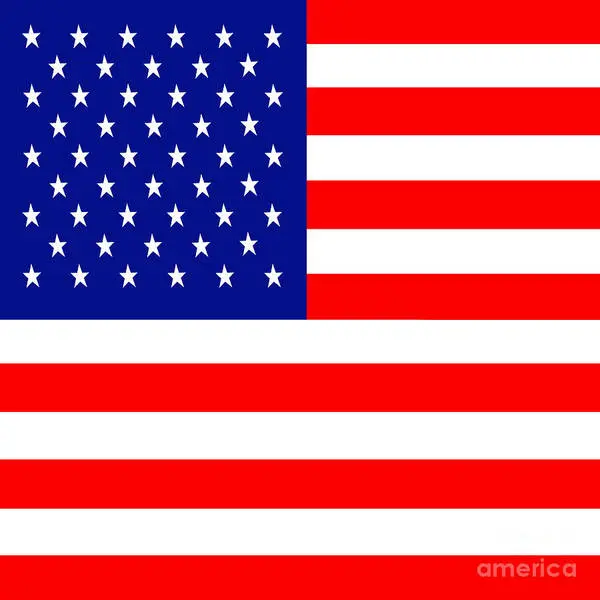
Email address
your-email@gmail.com
1 item Edit
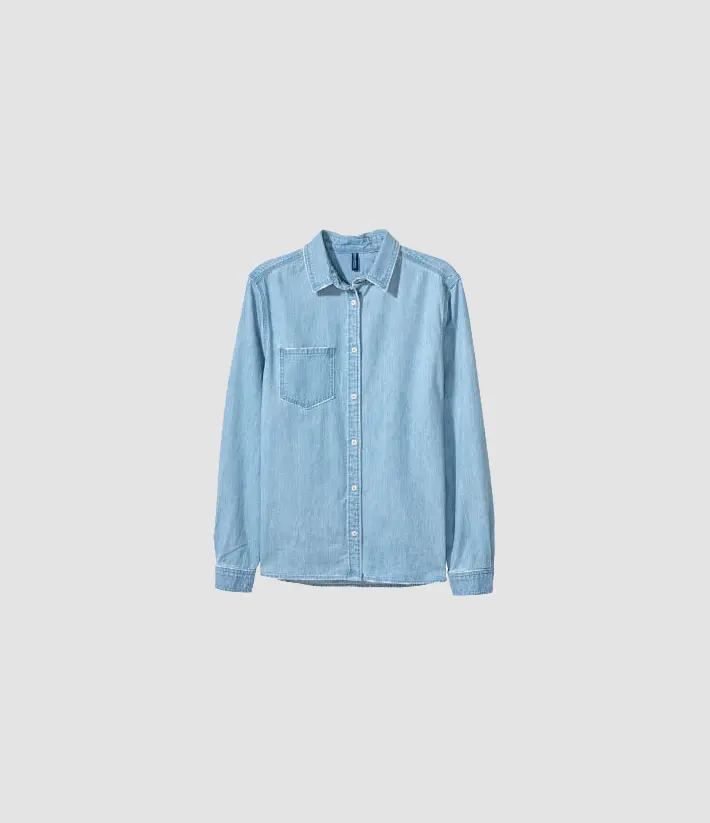
Denim jacket
Black UK 8Qty:1
Delivery address
import React from 'react';
import { MDBAccordion, MDBAccordionItem, MDBBtn, MDBCard, MDBCardBody, MDBCardFooter, MDBCardHeader, MDBCardImage, MDBCheckbox, MDBCol, MDBContainer, MDBInput, MDBListGroup, MDBListGroupItem, MDBRow, MDBTextArea, MDBTypography } from 'mdb-react-ui-kit';
export default function Advanced() {
return (
<MDBContainer className="my-5 py-5" style={{maxWidth: '1100px'}}>
<section>
<MDBRow>
<MDBCol md="8">
<MDBCard className="mb-4">
<MDBCardBody>
<p className="text-uppercase h4 text-font">Delivery Country:</p>
<MDBRow>
<MDBCol md="1">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Others/extended-example/usa2.webp"
className="rounded-circle me-2"
style={{ width: '35px' }}
alt="USA" />
</MDBCol>
<MDBCol md="8">
{/* PRO NEEDED */}
<select className="custom-select">
<option value="1">United States</option>
<option value="2">Spain</option>
<option value="3">Poland</option>
<option value="4">Italy</option>
<option value="5">Greece</option>
<option value="6">Germany</option>
<option value="7">Croatia</option>
<option value="8">Sweden</option>
</select>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBAccordion className="card mb-4">
<MDBAccordionItem collapseId={1} className="border-0" headerTitle='Promo/Student Code or Vouchers'>
<MDBInput label='Enter code' type='text' />
</MDBAccordionItem>
</MDBAccordion>
<MDBCard className="mb-4">
<MDBCardBody>
<p className="text-uppercase fw-bold mb-3 text-font">Email address</p>
<MDBRow>
<MDBCol md="4">
<p>your-email@gmail.com</p>
</MDBCol>
<MDBCol md="7">
<MDBBtn outline color="dark" className="float-end button-color">Change</MDBBtn>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4" className="mb-4 position-statics">
<MDBCard className="mb-4">
<MDBCardHeader className="py-3">
<MDBTypography tag="h5" className="mb-0 text-font">
1 item <span className="float-end mt-1" style={{ fontSize: '13px' }}>Edit</span>
</MDBTypography>
</MDBCardHeader>
<MDBCardBody>
<MDBRow>
<MDBCol md="4">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Vertical/12a.webp"
className="rounded-3" style={{ width: '100px' }} alt="Blue Jeans Jacket" />
</MDBCol>
<MDBCol md="6" className="ms-3">
<span className="mb-0 text-price">$35.00</span>
<p className="mb-0 text-descriptions">Denim jacket </p>
<span className="text-descriptions fw-bold">Black</span> <span
className="text-descriptions fw-bold">UK 8</span>
<p className="text-descriptions mt-0">
Qty:<span className="text-descriptions fw-bold">1</span>
</p>
</MDBCol>
</MDBRow>
</MDBCardBody>
<MDBCardFooter className="mt-4">
<MDBListGroup flush>
<MDBListGroupItem className="d-flex justify-content-between align-items-center border-0 px-0 pb-0 text-muted">
Subtotal
<span>$35.00</span>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center px-0 fw-bold text-uppercase">
Total to pay
<span>$35.00</span>
</MDBListGroupItem>
</MDBListGroup>
</MDBCardFooter>
</MDBCard>
</MDBCol>
<MDBCol md="8" className="mb-4">
<MDBCard className="mb-4">
<MDBCardHeader className="py-3">
<MDBTypography tag="h5" className="mb-0 text-font text-uppercase">Delivery address</MDBTypography>
</MDBCardHeader>
<MDBCardBody>
<form>
<MDBRow className="mb-4">
<MDBCol>
<MDBInput label='First name' type='text' />
</MDBCol>
<MDBCol>
<MDBInput label='Last name' type='text' />
</MDBCol>
</MDBRow>
<MDBInput label='Company name' type='text' className="mb-4" />
<MDBInput label='Address' type='text' className="mb-4" />
<MDBInput label='Email' type='text' className="mb-4" />
<MDBInput label='Phone' type='text' className="mb-4" />
<MDBTextArea label='Additional information' rows={4} className="mb-4" />
<div className="d-flex justify-content-center">
<MDBCheckbox name='flexCheck' value='' id='flexCheckChecked' label='Create an account?' defaultChecked />
</div>
</form>
</MDBCardBody>
</MDBCard>
<div className="text-center">
<MDBBtn className="button-order col-md-10">Place order</MDBBtn>
</div>
</MDBCol>
</MDBRow>
</section>
</MDBContainer>
);
}
.text-font{
font-family: futura-pt,Tahoma,Geneva,Verdana,Arial,sans-serif;
font-weight: 700;
letter-spacing: .156rem;
font-size: 1.125rem;
}
.text-price{
padding: 0 .625rem;
font-family: futura-pt,Tahoma,Geneva,Verdana,Arial,sans-serif;
font-style: normal;
font-size: .75rem;
font-weight: 700;
line-height: .813rem;
letter-spacing: 1.6px;
}
.text-descriptions{
font-family: futura-pt,Tahoma,Geneva,Verdana,Arial,sans-serif;
font-style: normal;
font-size: .75rem;
font-weight: 400;
line-height: 1.125rem;
margin: .313rem 0 .938rem;
padding: 0 .625rem;
}
.button-color{
color: #4e4e4e ;
border-color: #4e4e4e ;
}
.button-order{
font-family: futura-pt,Tahoma,Geneva,Verdana,Arial,sans-serif;
font-style: normal;
font-size: .75rem;
font-weight: 700;
background-color: hsl(90, 40%, 50%);
color: white;
}
Address form with image
Adding an Image to your address form will make it more visually appealing.
In the example below we've used a Background Image to add some additional text on top. We've covered the image with Mask Overlay to ensure high contrast - text on top of an image is usually easier to read when you apply a mask. For white text in the example, a dark mask is perfect but you can customize your mask by tweaking its color, transparency and blur effect with our Mask Generator.
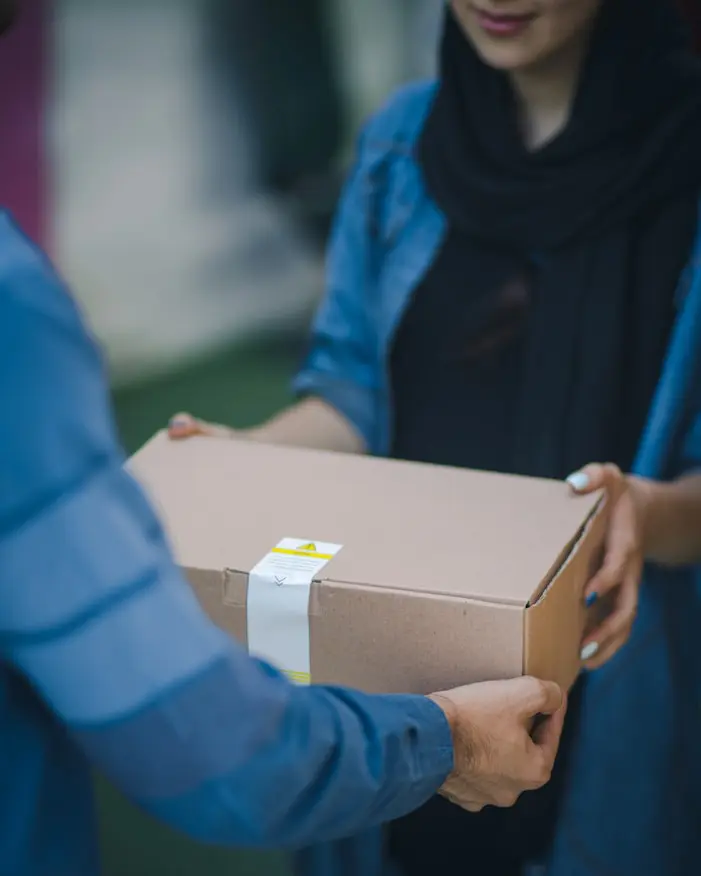
Lorem ipsum delivery
Everything at your doorstep.
Delivery Info
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardImage, MDBCol, MDBContainer, MDBIcon, MDBInput, MDBRow, MDBTypography } from 'mdb-react-ui-kit';
export default function Image() {
return (
<MDBContainer className="py-5" style={{ maxWidth: '1100px' }}>
<MDBRow className="justify-content-center align-items-center">
<MDBCol>
<MDBCard className="my-4 shadow-3">
<MDBRow className="g-0">
<MDBCol md="6" className="d-xl-block bg-image">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Others/extended-example/delivery.webp" alt="Sample photo" fluid />
<div className='mask' style={{ backgroundColor: 'rgba(0, 0, 0, 0.6)' }}>
<div className="justify-content-center align-items-center h-100">
<div className="text-center" style={{ marginTop: '220px' }}>
<MDBIcon fas icon="truck text-white" size="3x" />
<p className="text-white title-style">Lorem ipsum delivery</p>
<p className="text-white mb-0"></p>
<figure className="text-center mb-0">
<blockquote className="blockquote text-white">
<p className="pb-3">
<MDBIcon fas icon="quote-left text-primary" size="xs" style={{color: 'hsl(210, 100%, 50%)'}} />
<span className="lead font-italic">Everything at your doorstep.</span>
<MDBIcon fas icon="quote-right text-primary" size="xs" style={{color: 'hsl(210, 100%, 50%)'}} />
</p>
</blockquote>
</figure>
</div>
</div>
</div>
</MDBCol>
<MDBCol md="6">
<MDBCardBody className="p-md-5 text-black">
<MDBTypography tag="h3" className="mb-4 text-uppercase">Delivery Info</MDBTypography>
<MDBRow>
<MDBCol md="6" className="mb-4">
<MDBInput label='First name' type='text' size="lg" />
</MDBCol>
<MDBCol md="6" className="mb-4">
<MDBInput label='Last name' type='text' size="lg" />
</MDBCol>
</MDBRow>
<MDBInput label='Address' type='text' className="mb-4" size="lg" />
<MDBRow>
<MDBCol md="6" className="mb-4">
<MDBInput label='State' type='text' size="lg" />
</MDBCol>
<MDBCol md="6" className="mb-4">
<MDBInput label='City' type='text' size="lg" />
</MDBCol>
</MDBRow>
<MDBInput label='Zip' type='text' className="mb-4" size="lg" />
<MDBInput label='Email' type='text' className="mb-4" size="lg" />
<div className="d-flex justify-content-end pt-3">
<MDBBtn size="lg" className="ms-2" style={{backgroundColor: 'hsl(210, 100%, 50%)'}}>Place order</MDBBtn>
</div>
</MDBCardBody>
</MDBCol>
</MDBRow>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
.title-style{
font-family: Georgia, 'Times New Roman', Times, serif;
font-weight: 700;
font-size: 20px;
color: hsl(52, 0%, 98%);
}
.title-quote{
font-family: Georgia, 'Times New Roman', Times, serif;
font-weight: 400;
color: hsl(52, 0%, 98%);
}