Card deck
React Bootstrap 5 Card Deck
Responsive Card Deck built with Bootstrap 5, React 18 and Material Design 2.0. Bootstrap card-deck with multiple rows and standard breakpoints.Card layout
In addition to styling the content within cards, Bootstrap includes a few options for laying out series of cards.
Card groups
Use card groups to render cards as a single, attached element with equal width and height
columns. Card groups start off stacked and use
display: flex;
to become attached with uniform dimensions starting at the
sm
breakpoint.
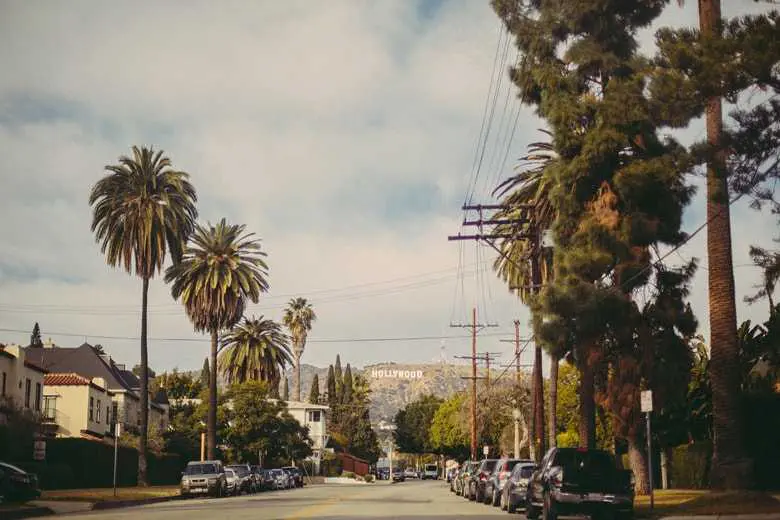
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
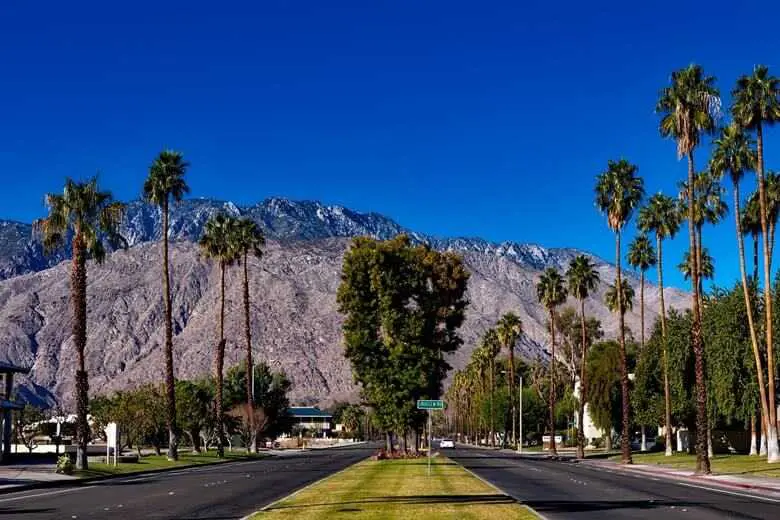
Card title
This card has supporting text below as a natural lead-in to additional content.
Last updated 3 mins ago
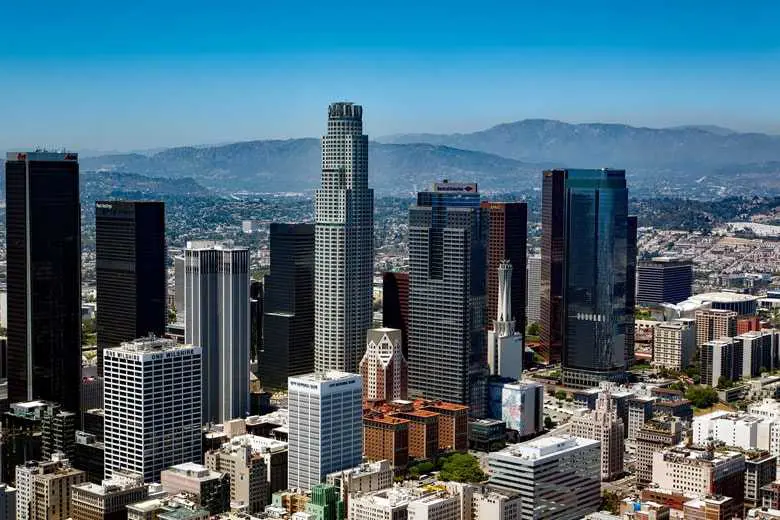
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
Last updated 3 mins ago
import React from 'react';
import {
MDBCard,
MDBCardImage,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBCardGroup
} from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCardGroup>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/041.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/042.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/043.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
card has even longer content than the first to show that equal height action.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCardGroup>
);
}
When using card groups with footers, their content will automatically line up.
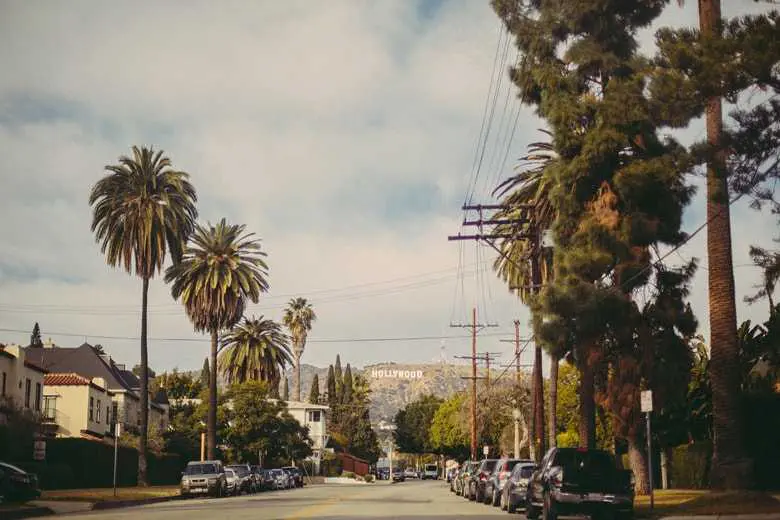
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
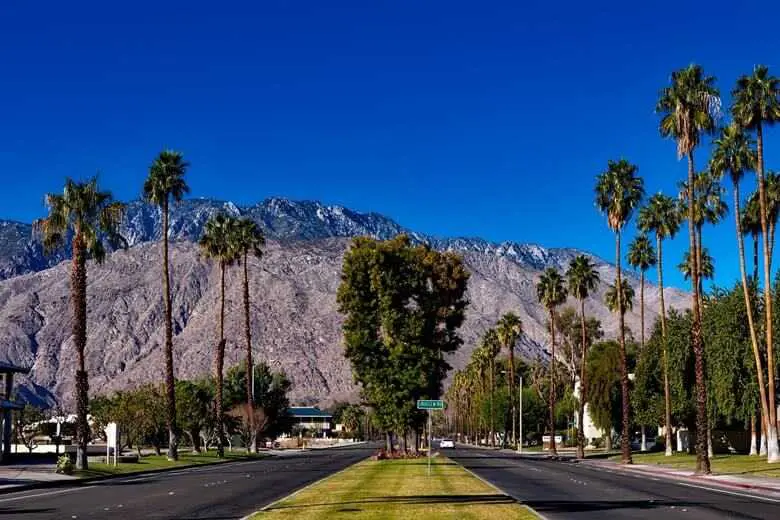
Card title
This card has supporting text below as a natural lead-in to additional content.
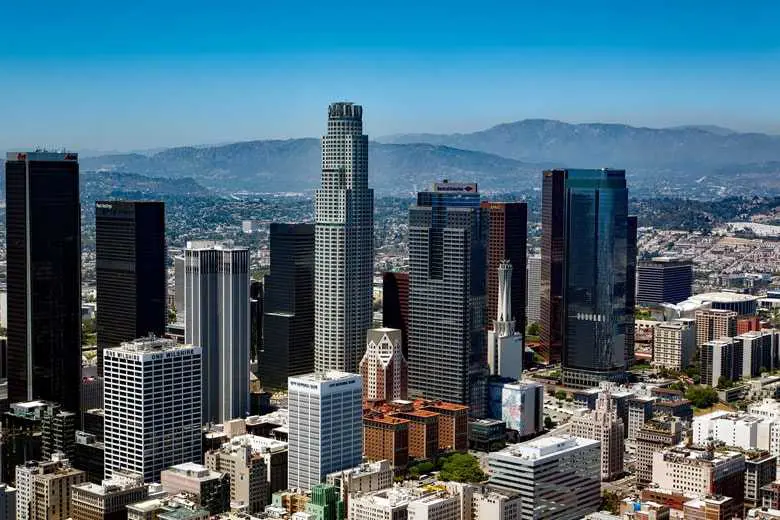
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
import React from 'react';
import {
MDBCard,
MDBCardImage,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBCardFooter,
MDBCardGroup
} from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCardGroup>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/041.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/042.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/043.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
card has even longer content than the first to show that equal height action.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCardGroup>
);
}
Grid cards
Use the Bootstrap grid system and its
.row-cols
classes to control how many grid columns (wrapped around your cards)
you show per row. For example, here’s .row-cols-1
laying out the cards on one
column, and .row-cols-md-2
splitting four cards to equal width across multiple
rows, from the medium breakpoint up.
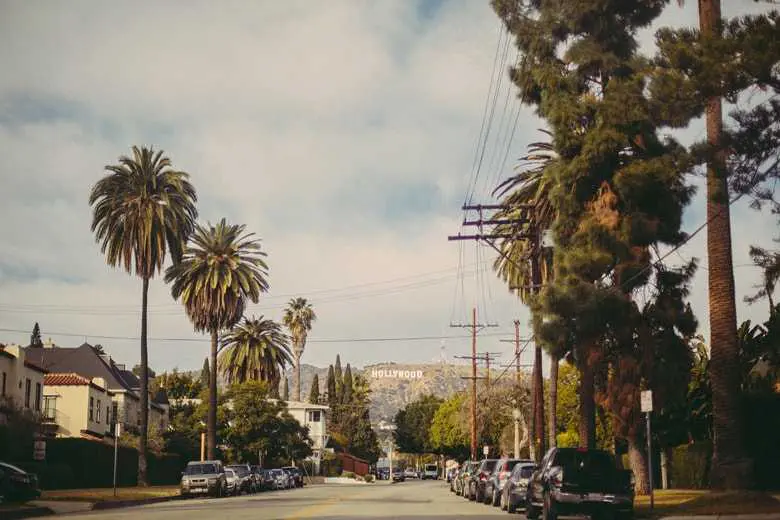
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
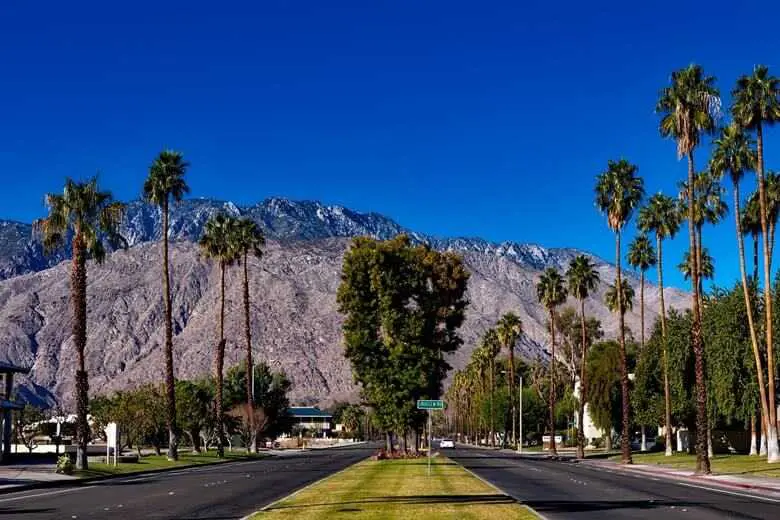
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
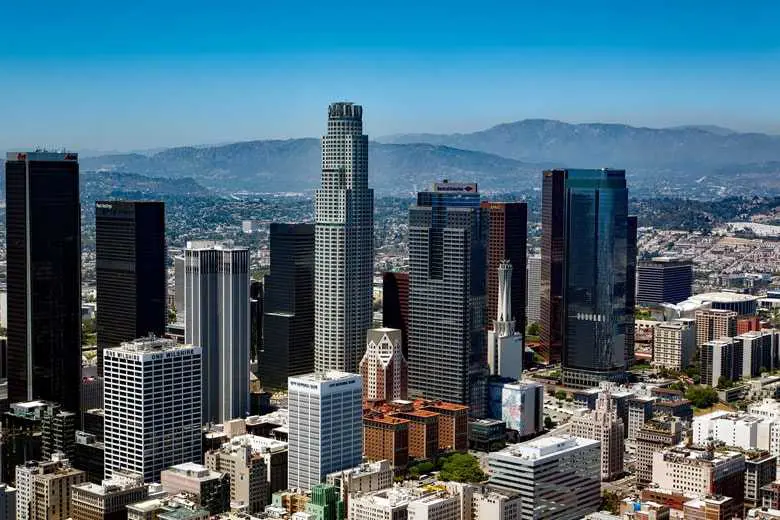
Card title
This is a longer card with supporting text below as a natural lead-in to additional content.
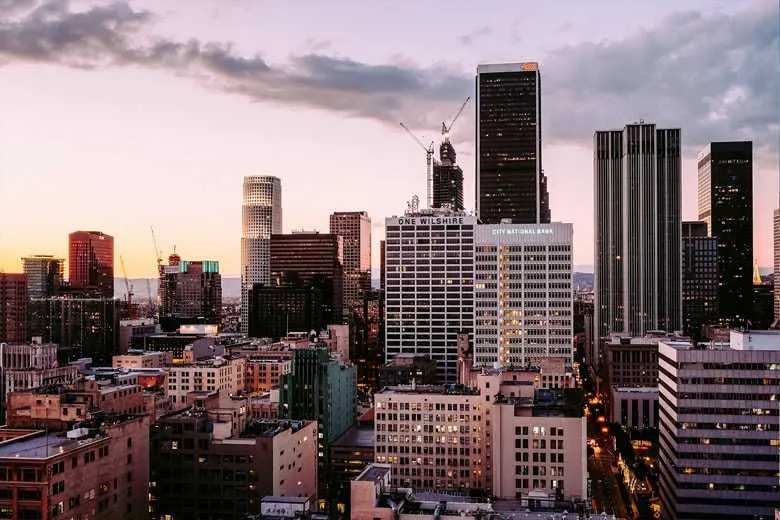
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import React from 'react';
import {
MDBCard,
MDBCardImage,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBRow,
MDBCol
} from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-2 g-4'>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
Change it to .row-cols-3
and you’ll see the fourth card wrap.
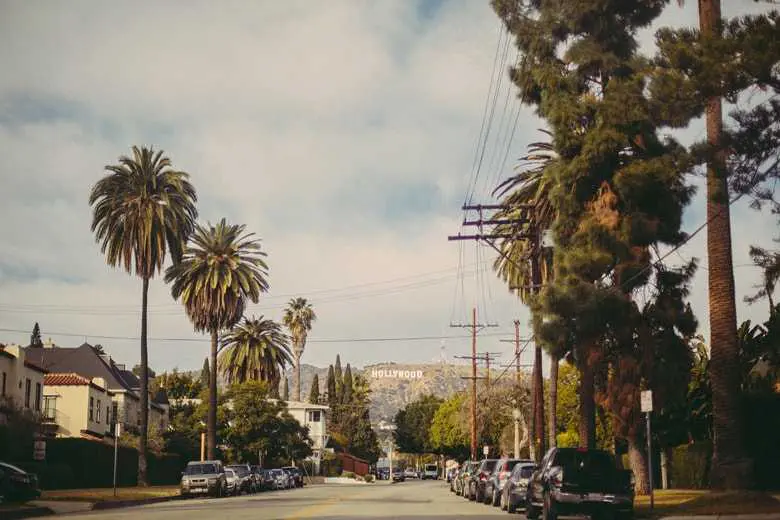
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
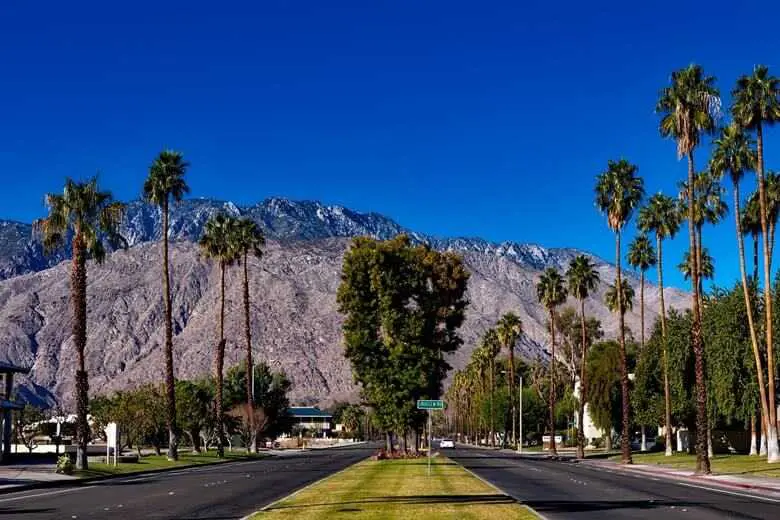
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
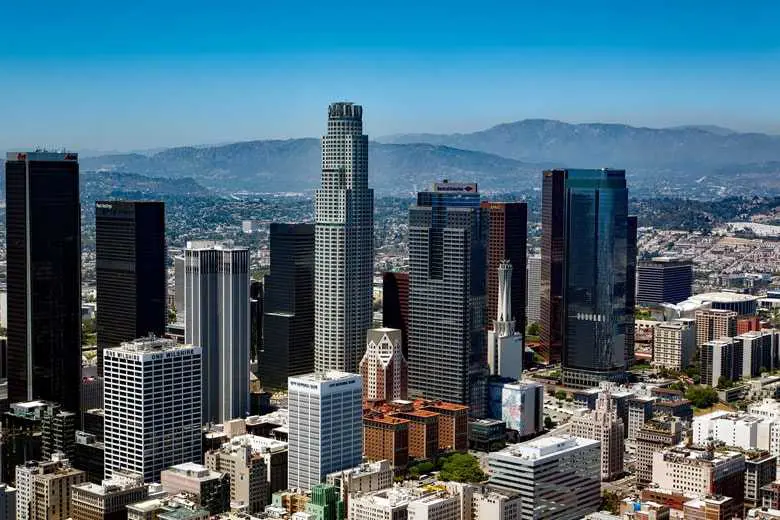
Card title
This is a longer card with supporting text below as a natural lead-in to additional content.
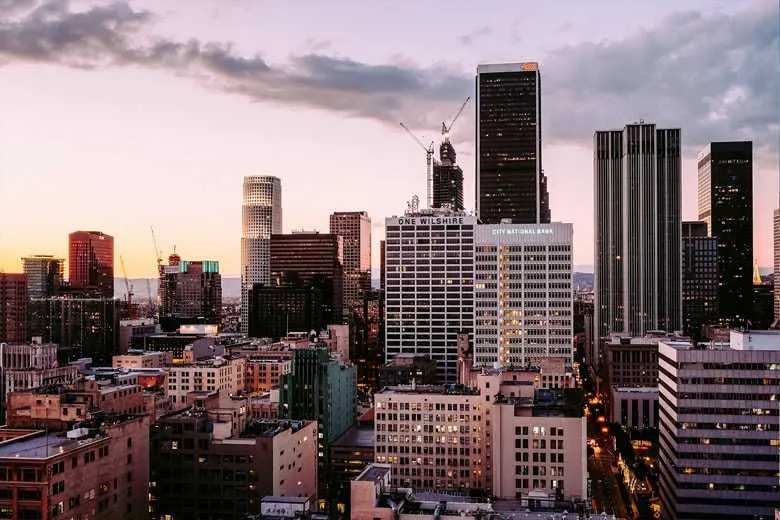
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import React from 'react';
import {
MDBCard,
MDBCardImage,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBRow,
MDBCol
} from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-3 g-4'>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
When you need equal height, add .h-100
to the cards. If you want equal heights
by default, you can set $card-height: 100%
in Sass.
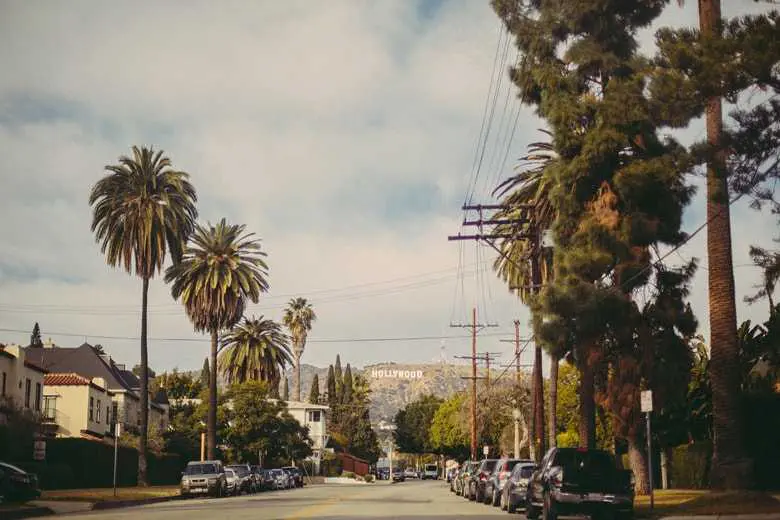
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
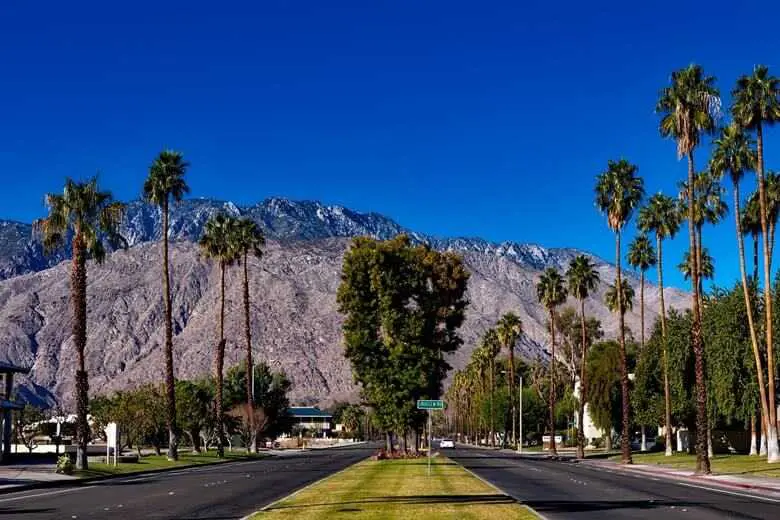
Card title
This is a short card.
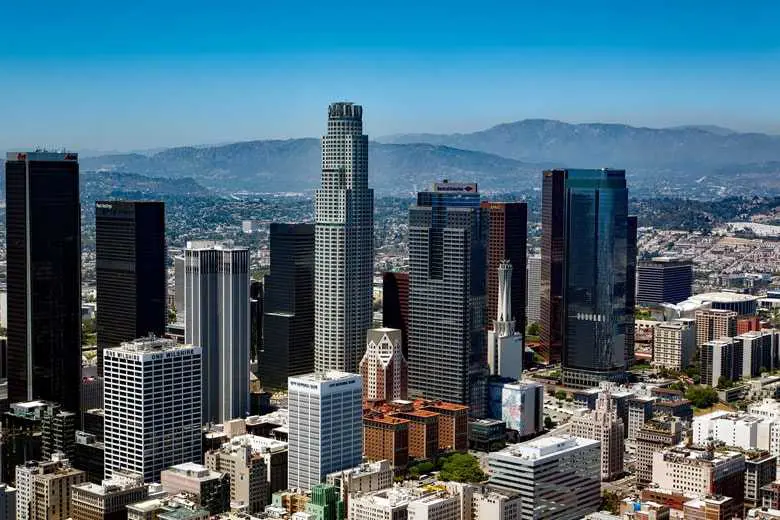
Card title
This is a longer card with supporting text below as a natural lead-in to additional content.
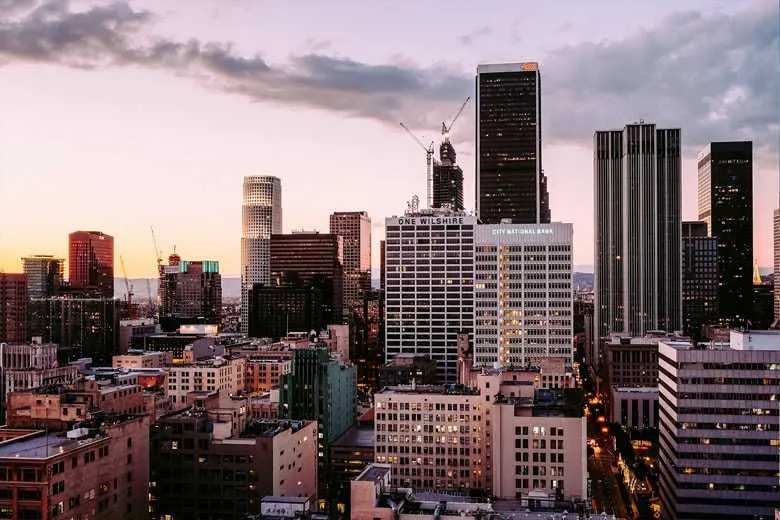
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import React from 'react';
import {
MDBCard,
MDBCardImage,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBRow,
MDBCol
} from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-3 g-4'>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>This is a short card.</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
Just like with card groups, card footers will automatically line up.
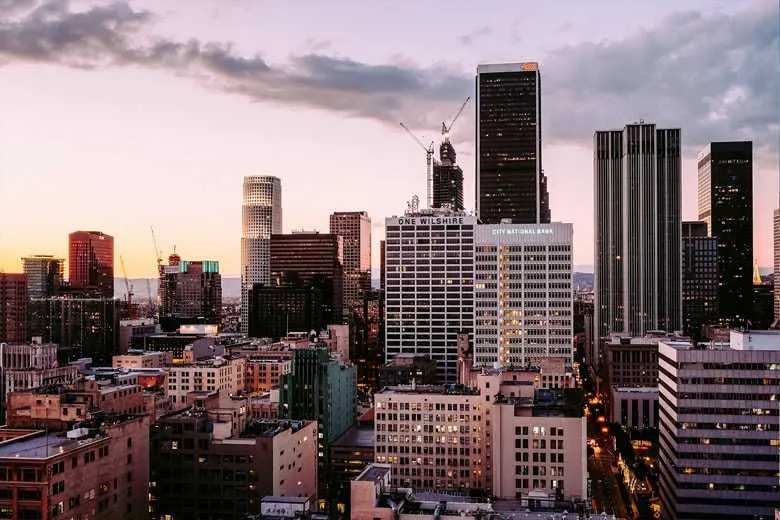
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
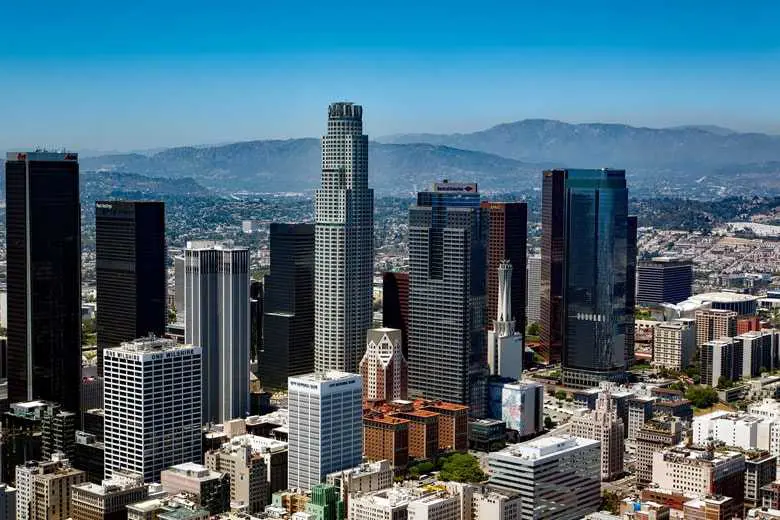
Card title
This card has supporting text below as a natural lead-in to additional content.
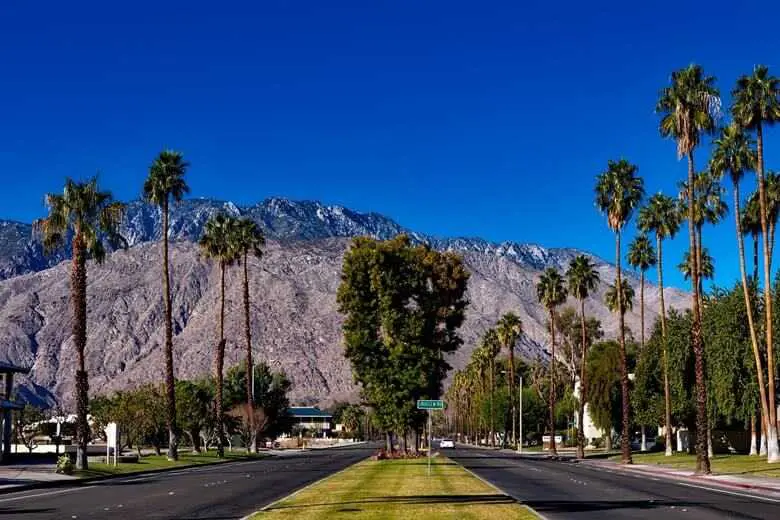
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
import React from 'react';
import {
MDBCard,
MDBCardImage,
MDBCardBody,
MDBCardTitle,
MDBCardText,
MDBCardFooter,
MDBRow,
MDBCol
} from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-3 g-4'>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This card has supporting text below as a natural lead-in to additional content.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
card has even longer content than the first to show that equal height action.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCol>
</MDBRow>
);
}