Product Cards
React Bootstrap 5 Product Cards
Responsive React Product Cards built with the latest Bootstrap 5. Card grid usage, eCommerce product cards, product card listing deck, card with reviews and more.Basic example
Basic example of a product card.
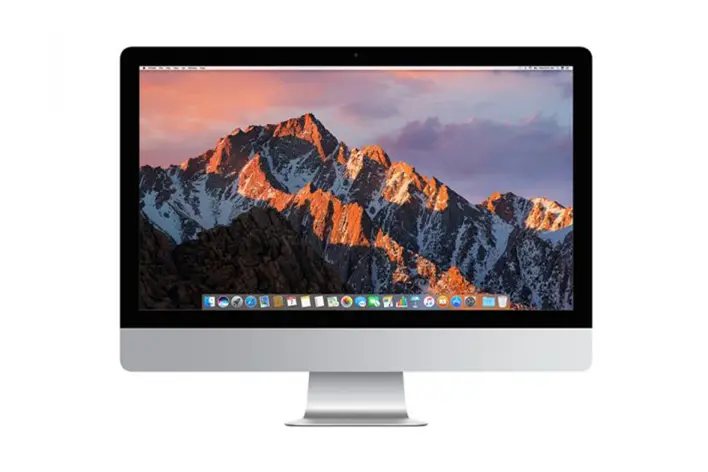
Believing is seeing
Apple pro display XDR
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCardTitle,
MDBIcon,
} from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer fluid className="my-5">
<MDBRow className="justify-content-center">
<MDBCol md="6">
<MDBCard className="text-black">
<MDBIcon fab icon="apple" size="lg" className="px-3 pt-3 pb-2" />
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/3.webp"
position="top"
alt="Apple Computer"
/>
<MDBCardBody>
<div className="text-center">
<MDBCardTitle>Believing is seeing</MDBCardTitle>
<p className="text-muted mb-4">Apple pro display XDR</p>
</div>
<div>
<div className="d-flex justify-content-between">
<span>Pro Display XDR</span>
<span>$5,999</span>
</div>
<div className="d-flex justify-content-between">
<span>Pro stand</span>
<span>$999</span>
</div>
<div className="d-flex justify-content-between">
<span>Vesa Mount Adapter</span>
<span>$199</span>
</div>
</div>
<div className="d-flex justify-content-between total font-weight-bold mt-4">
<span>Total</span>
<span>$7,197.00</span>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}
Product comparison template
Examples of cards with product comparisons, great alternative to a comparison table pricing.
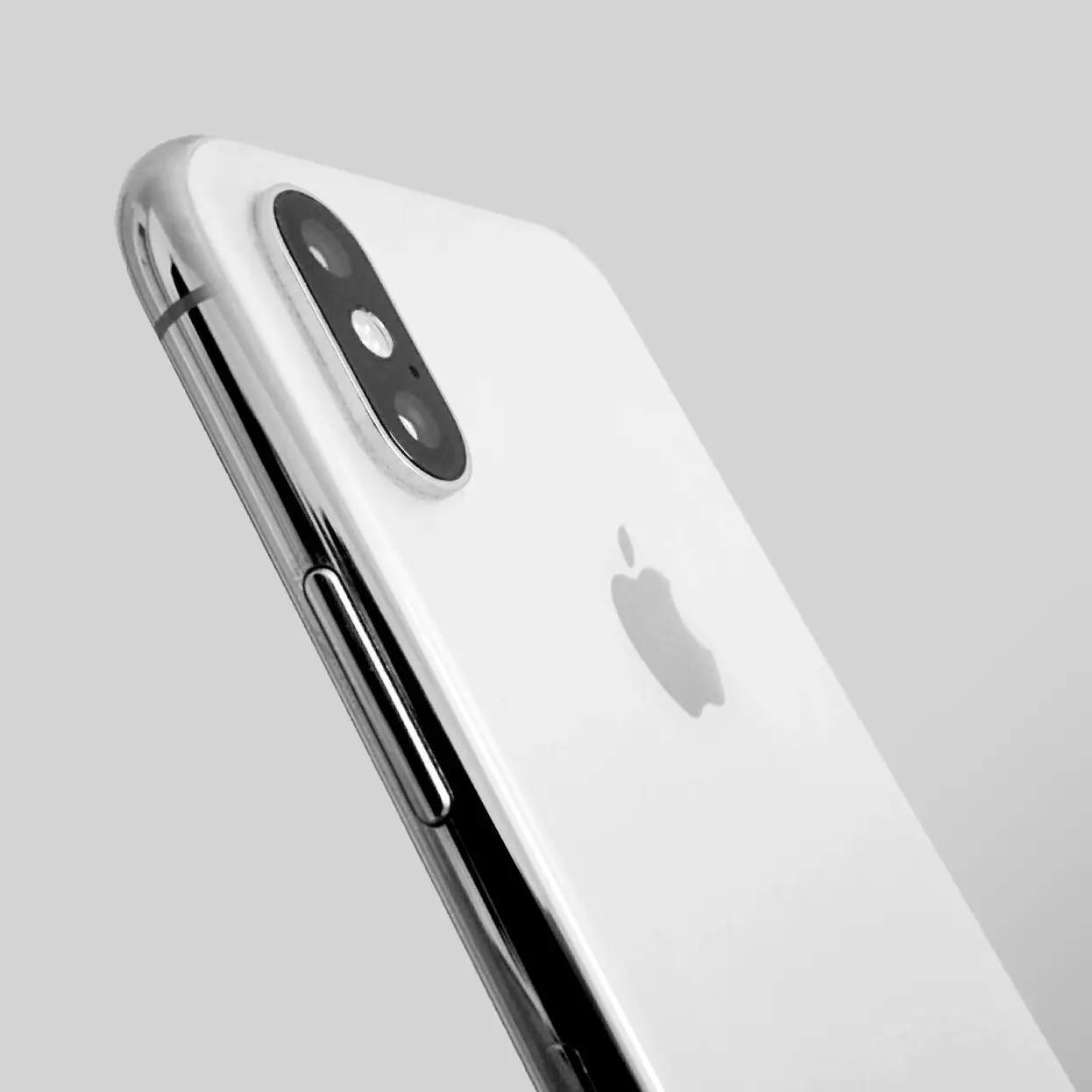
iPhone X
Starting at $399
Quick Look
- Wide
- Telephoto
Capacity
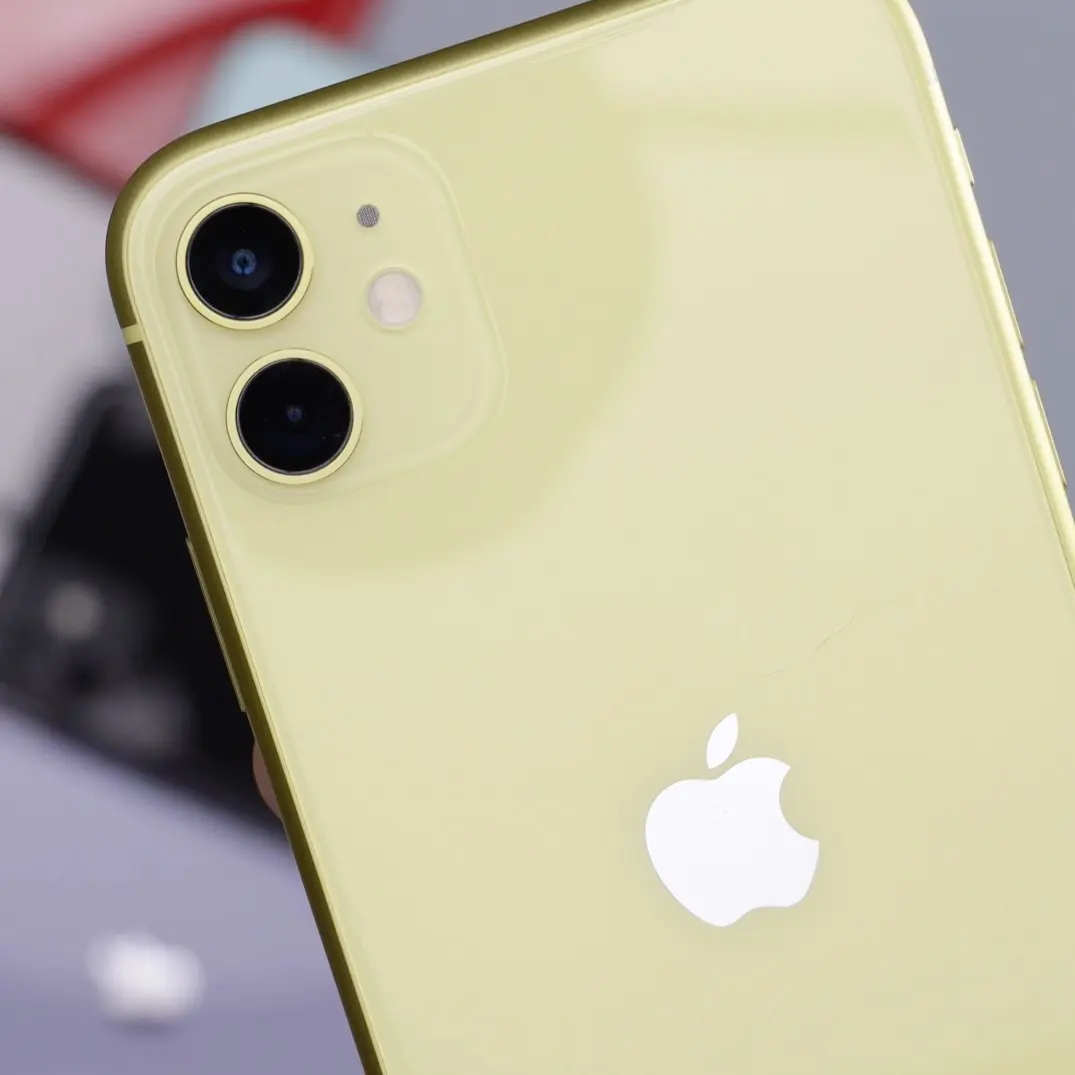
iPhone 11
Starting at $499
Quick Look
- Wide
Capacity
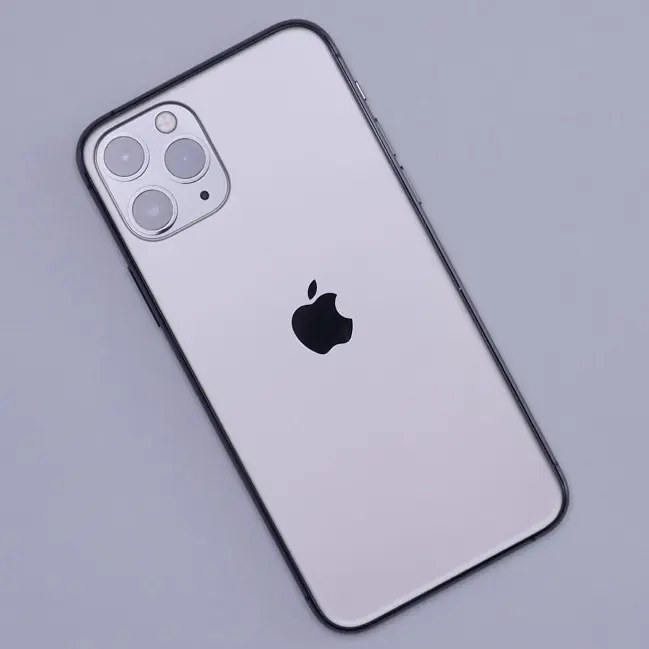
iPhone 11 Pro
Starting at $599
Quick Look
- Wide
- Telephoto
Capacity
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCardTitle,
MDBIcon,
MDBBtn,
} from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer fluid className="my-5">
<MDBRow>
<MDBCol md="4" className="mb-4 mb-lg-0">
<MDBCard className="text-black">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-product-cards/img1.webp"
position="top"
alt="iPhone"
/>
<MDBCardBody>
<div className="text-center mt-1">
<MDBCardTitle className="h4">iPhone X</MDBCardTitle>
<h6 className="text-primary mb-1 pb-3">Starting at $399</h6>
</div>
<div className="text-center">
<div
className="p-3 mx-n3 mb-4"
style={{ backgroundColor: "#eff1f2" }}
>
<h5 className="mb-0">Quick Look</h5>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mt-4 mb-0">5.8″</span>
<span>Super Retina HD display1</span>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mb-0">
<MDBIcon fas icon="camera-retro" />
</span>
<ul className="list-unstyled mb-0">
<li aria-hidden="true">—</li>
<li>Wide</li>
<li>Telephoto</li>
<li aria-hidden="true">—</li>
</ul>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mb-0">2x</span>
<span>Optical zoom range</span>
</div>
<div
className="p-3 mx-n3 mb-4"
style={{ backgroundColor: "#eff1f2" }}
>
<h5 className="mb-0">Capacity</h5>
</div>
<div className="d-flex flex-column mb-4 lead">
<span className="mb-2">64GB</span>
<span className="mb-2">256GB</span>
<span style={{ color: "transparent" }}>0</span>
</div>
</div>
<div className="d-flex flex-row">
<MDBBtn
color="primary"
rippleColor="dark"
className="flex-fill ms-1"
>
Learn more
</MDBBtn>
<MDBBtn color="danger" className="flex-fill ms-2">
Buy now
</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4" className="mb-4 mb-lg-0">
<MDBCard className="text-black">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-product-cards/img2.webp"
position="top"
alt="iPhone"
/>
<MDBCardBody>
<div className="text-center mt-1">
<MDBCardTitle className="h4">iPhone 11</MDBCardTitle>
<h6 className="text-primary mb-1 pb-3">Starting at $499</h6>
</div>
<div className="text-center">
<div
className="p-3 mx-n3 mb-4"
style={{ backgroundColor: "#eff1f2" }}
>
<h5 className="mb-0">Quick Look</h5>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mt-4 mb-0">6.1″</span>
<span>Liquid Retina HD display1</span>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mb-0">
<MDBIcon fas icon="camera-retro" />
</span>
<ul className="list-unstyled mb-0">
<li aria-hidden="true">Ultra Wide</li>
<li>Wide</li>
<li aria-hidden="true">—</li>
<li aria-hidden="true">—</li>
</ul>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mb-0">2x</span>
<span>Optical zoom range</span>
</div>
<div
className="p-3 mx-n3 mb-4"
style={{ backgroundColor: "#eff1f2" }}
>
<h5 className="mb-0">Capacity</h5>
</div>
<div className="d-flex flex-column mb-4 lead">
<span className="mb-2">64GB</span>
<span class="mb-2">128GB</span>
<span>256GB</span>
</div>
</div>
<div className="d-flex flex-row">
<MDBBtn
color="primary"
rippleColor="dark"
className="flex-fill ms-1"
>
Learn more
</MDBBtn>
<MDBBtn color="danger" className="flex-fill ms-2">
Buy now
</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4" className="mb-4 mb-lg-0">
<MDBCard className="text-black">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-product-cards/img3.webp"
position="top"
alt="iPhone"
/>
<MDBCardBody>
<div className="text-center mt-1">
<MDBCardTitle className="h4">iPhone 11 Pro</MDBCardTitle>
<h6 className="text-primary mb-1 pb-3">Starting at $599</h6>
</div>
<div className="text-center">
<div
className="p-3 mx-n3 mb-4"
style={{ backgroundColor: "#eff1f2" }}
>
<h5 className="mb-0">Quick Look</h5>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mt-4 mb-0">5.8″</span>
<span>Super Retina HD display1</span>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mb-0">
<MDBIcon fas icon="camera-retro" />
</span>
<ul className="list-unstyled mb-0">
<li aria-hidden="true">Ultra Wide</li>
<li>Wide</li>
<li>Telephoto</li>
<li aria-hidden="true">—</li>
</ul>
</div>
<div className="d-flex flex-column mb-4">
<span className="h1 mb-0">4x</span>
<span>Optical zoom range</span>
</div>
<div
className="p-3 mx-n3 mb-4"
style={{ backgroundColor: "#eff1f2" }}
>
<h5 className="mb-0">Capacity</h5>
</div>
<div className="d-flex flex-column mb-4 lead">
<span class="mb-2">64GB</span>
<span class="mb-2">256GB</span>
<span>512GB</span>
</div>
</div>
<div className="d-flex flex-row">
<MDBBtn
color="primary"
rippleColor="dark"
className="flex-fill ms-1"
>
Learn more
</MDBBtn>
<MDBBtn color="danger" className="flex-fill ms-2">
Buy now
</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}
Ecommerce category product list page
Product listing cards with star ratings and the number of reviews
Quant trident shirts
There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form, by injected humour, or randomised words which don't look even slightly believable.
$13.99
Free shipping
Quant olap shirts
There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form, by injected humour, or randomised words which don't look even slightly believable.
$14.99
Free shipping
Quant ruybi shirts
There are many variations of passages of Lorem Ipsum available, but the majority have suffered alteration in some form, by injected humour, or randomised words which don't look even slightly believable.
$17.99
Free shipping
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBIcon,
MDBRipple,
MDBBtn,
} from "mdb-react-ui-kit";
import "./ecommerce-category-product.css";
function App() {
return (
<MDBContainer fluid>
<MDBRow className="justify-content-center mb-0">
<MDBCol md="12" xl="10">
<MDBCard className="shadow-0 border rounded-3 mt-5 mb-3">
<MDBCardBody>
<MDBRow>
<MDBCol md="12" lg="3" className="mb-4 mb-lg-0">
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom hover-overlay"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/img%20(4).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6">
<h5>Quant trident shirts</h5>
<div className="d-flex flex-row">
<div className="text-danger mb-1 me-2">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
</div>
<span>310</span>
</div>
<div className="mt-1 mb-0 text-muted small">
<span>100% cotton</span>
<span className="text-primary"> • </span>
<span>Light weight</span>
<span className="text-primary"> • </span>
<span>
Best finish
<br />
</span>
</div>
<div className="mb-2 text-muted small">
<span>Unique design</span>
<span className="text-primary"> • </span>
<span>For men</span>
<span className="text-primary"> • </span>
<span>
Casual
<br />
</span>
</div>
<p className="text-truncate mb-4 mb-md-0">
There are many variations of passages of Lorem Ipsum
available, but the majority have suffered alteration in some
form, by injected humour, or randomised words which don't
look even slightly believable.
</p>
</MDBCol>
<MDBCol
md="6"
lg="3"
className="border-sm-start-none border-start"
>
<div className="d-flex flex-row align-items-center mb-1">
<h4 className="mb-1 me-1">$13.99</h4>
<span className="text-danger">
<s>$20.99</s>
</span>
</div>
<h6 className="text-success">Free shipping</h6>
<div className="d-flex flex-column mt-4">
<MDBBtn color="primary" size="sm">
Details
</MDBBtn>
<MDBBtn outline color="primary" size="sm" className="mt-2">
Add to wish list
</MDBBtn>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
<MDBRow className="justify-content-center mb-3">
<MDBCol md="12" xl="10">
<MDBCard className="shadow-0 border rounded-3">
<MDBCardBody>
<MDBRow>
<MDBCol md="12" lg="3" className="mb-4 mb-lg-0">
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom hover-overlay"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(4).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6">
<h5>Quant olap shirts</h5>
<div className="d-flex flex-row">
<div className="text-danger mb-1 me-2">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
</div>
<span>289</span>
</div>
<div className="mt-1 mb-0 text-muted small">
<span>100% cotton</span>
<span className="text-primary"> • </span>
<span>Light weight</span>
<span classNAme="text-primary"> • </span>
<span>
Best finish
<br />
</span>
</div>
<div className="mb-2 text-muted small">
<span>Unique design</span>
<span className="text-primary"> • </span>
<span>For men</span>
<span className="text-primary"> • </span>
<span>
Casual
<br />
</span>
</div>
<p className="text-truncate mb-4 mb-md-0">
There are many variations of passages of Lorem Ipsum
available, but the majority have suffered alteration in some
form, by injected humour, or randomised words which don't
look even slightly believable.
</p>
</MDBCol>
<MDBCol
md="6"
lg="3"
className="border-sm-start-none border-start"
>
<div className="d-flex flex-row align-items-center mb-1">
<h4 className="mb-1 me-1">$14.99</h4>
<span className="text-danger">
<s>$21.99</s>
</span>
</div>
<h6 className="text-success">Free shipping</h6>
<div className="d-flex flex-column mt-4">
<MDBBtn color="primary" size="sm">
Details
</MDBBtn>
<MDBBtn outline color="primary" size="sm" className="mt-2">
Add to wish list
</MDBBtn>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
<MDBRow className="justify-content-center mb-3">
<MDBCol md="12" xl="10">
<MDBCard className="shadow-0 border rounded-3">
<MDBCardBody>
<MDBRow>
<MDBCol md="12" lg="3" className="mb-4 mb-lg-0">
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom hover-overlay"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(5).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6">
<h5>Quant ruybi shirts</h5>
<div className="d-flex flex-row">
<div className="text-danger mb-1 me-2">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
</div>
<span>145</span>
</div>
<div className="mt-1 mb-0 text-muted small">
<span>100% cotton</span>
<span className="text-primary"> • </span>
<span>Light weight</span>
<span className="text-primary"> • </span>
<span>
Best finish
<br />
</span>
</div>
<div className="mb-2 text-muted small">
<span>Unique design</span>
<span className="text-primary"> • </span>
<span>For women</span>
<span className="text-primary"> • </span>
<span>
Casual
<br />
</span>
</div>
<p className="text-truncate mb-4 mb-md-0">
There are many variations of passages of Lorem Ipsum
available, but the majority have suffered alteration in some
form, by injected humour, or randomised words which don't
look even slightly believable.
</p>
</MDBCol>
<MDBCol
md="6"
lg="3"
className="border-sm-start-none border-start"
>
<div className="d-flex flex-row align-items-center mb-1">
<h4 className="mb-1 me-1">$17.99</h4>
<span className="text-danger">
<s>$25.99</s>
</span>
</div>
<h6 className="text-success">Free shipping</h6>
<div className="d-flex flex-column mt-4">
<MDBBtn color="primary" size="sm">
Details
</MDBBtn>
<MDBBtn outline color="primary" size="sm" className="mt-2">
Add to wish list
</MDBBtn>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}
@media (max-width: 767.98px) {
.border-sm-start-none {
border-left: none !important;
}
}
Ecommerce product listing
Simple product listing card deck with number of discounted offers and discount value.
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBIcon,
} from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer fluid className="my-5">
<MDBRow>
<MDBCol md="12" lg="4" className="mb-4 mb-lg-0">
<MDBCard>
<div className="d-flex justify-content-between p-3">
<p className="lead mb-0">Today's Combo Offer</p>
<div
className="bg-info rounded-circle d-flex align-items-center justify-content-center shadow-1-strong"
style={{ width: "35px", height: "35px" }}
>
<p className="text-white mb-0 small">x4</p>
</div>
</div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/4.webp"
position="top"
alt="Laptop"
/>
<MDBCardBody>
<div className="d-flex justify-content-between">
<p className="small">
<a href="#!" className="text-muted">
Laptops
</a>
</p>
<p className="small text-danger">
<s>$1099</s>
</p>
</div>
<div className="d-flex justify-content-between mb-3">
<h5 className="mb-0">HP Notebook</h5>
<h5 className="text-dark mb-0">$999</h5>
</div>
<div class="d-flex justify-content-between mb-2">
<p class="text-muted mb-0">
Available: <span class="fw-bold">6</span>
</p>
<div class="ms-auto text-warning">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="12" lg="4" className="mb-4 mb-lg-0">
<MDBCard>
<div className="d-flex justify-content-between p-3">
<p className="lead mb-0">Today's Combo Offer</p>
<div
className="bg-info rounded-circle d-flex align-items-center justify-content-center shadow-1-strong"
style={{ width: "35px", height: "35px" }}
>
<p className="text-white mb-0 small">x2</p>
</div>
</div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/7.webp"
position="top"
alt="Laptop"
/>
<MDBCardBody>
<div className="d-flex justify-content-between">
<p className="small">
<a href="#!" className="text-muted">
Laptops
</a>
</p>
<p className="small text-danger">
<s>$1199</s>
</p>
</div>
<div className="d-flex justify-content-between mb-3">
<h5 className="mb-0">HP Envy</h5>
<h5 className="text-dark mb-0">$1099</h5>
</div>
<div class="d-flex justify-content-between mb-2">
<p class="text-muted mb-0">
Available: <span class="fw-bold">7</span>
</p>
<div class="ms-auto text-warning">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon far icon="star" />
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="12" lg="4" className="mb-4 mb-lg-0">
<MDBCard>
<div className="d-flex justify-content-between p-3">
<p className="lead mb-0">Today's Combo Offer</p>
<div
className="bg-info rounded-circle d-flex align-items-center justify-content-center shadow-1-strong"
style={{ width: "35px", height: "35px" }}
>
<p className="text-white mb-0 small">x3</p>
</div>
</div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/5.webp"
position="top"
alt="Gaming Laptop"
/>
<MDBCardBody>
<div className="d-flex justify-content-between">
<p className="small">
<a href="#!" className="text-muted">
Laptops
</a>
</p>
<p className="small text-danger">
<s>$1399</s>
</p>
</div>
<div className="d-flex justify-content-between mb-3">
<h5 className="mb-0">Toshiba B77</h5>
<h5 className="text-dark mb-0">$1299</h5>
</div>
<div class="d-flex justify-content-between mb-2">
<p class="text-muted mb-0">
Available: <span class="fw-bold">5</span>
</p>
<div class="ms-auto text-warning">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star-half-alt" />
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}
Quick buy product card
Simple product card with "Buy now" CTA button. Ideal as an upselling card for shopping carts.
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBIcon,
MDBBtn,
MDBRipple,
} from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer fluid className="my-5">
<MDBRow className="justify-content-center">
<MDBCol md="8" lg="6" xl="4">
<MDBCard style={{ borderRadius: "15px" }}>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-overlay"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/12.webp"
fluid
className="w-100"
style={{
borderTopLeftRadius: "15px",
borderTopRightRadius: "15px",
}}
/>
<a href="#!">
<div className="mask"></div>
</a>
</MDBRipple>
<MDBCardBody className="pb-0">
<div className="d-flex justify-content-between">
<div>
<p>
<a href="#!" className="text-dark">
Dell Xtreme 270
</a>
</p>
<p className="small text-muted">Laptops</p>
</div>
<div>
<div className="d-flex flex-row justify-content-end mt-1 mb-4 text-danger">
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
<MDBIcon fas icon="star" />
</div>
<p className="small text-muted">Rated 4.0/5</p>
</div>
</div>
</MDBCardBody>
<hr class="my-0" />
<MDBCardBody className="pb-0">
<div className="d-flex justify-content-between">
<p>
<a href="#!" className="text-dark">
$3,999
</a>
</p>
<p className="text-dark">#### 8787</p>
</div>
<p className="small text-muted">VISA Platinum</p>
</MDBCardBody>
<hr class="my-0" />
<MDBCardBody className="pb-0">
<div className="d-flex justify-content-between align-items-center pb-2 mb-4">
<a href="#!" className="text-dark fw-bold">
Cancel
</a>
<MDBBtn color="primary">Buy now</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}
Bestsellers listing
Bestseller cards with zoom effect on hover and category badges. Great for creating an impressive product gallery.
Bestsellers
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBIcon,
MDBBtn,
MDBRipple,
} from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer fluid className="my-5 text-center">
<h4 className="mt-4 mb-5">
<strong>Bestsellers</strong>
</h4>
<MDBRow>
<MDBCol md="12" lg="4" className="mb-4">
<MDBCard>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/belt.webp"
fluid
className="w-100"
/>
<a href="#!">
<div className="mask">
<div className="d-flex justify-content-start align-items-end h-100">
<h5>
<span className="badge bg-primary ms-2">New</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
<MDBCardBody>
<a href="#!" className="text-reset">
<h5 className="card-title mb-3">Product name</h5>
</a>
<a href="#!" className="text-reset">
<p>Category</p>
</a>
<h6 className="mb-3">$61.99</h6>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBCard>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/img%20(4).webp"
fluid
className="w-100"
/>
<a href="#!">
<div className="mask">
<div className="d-flex justify-content-start align-items-end h-100">
<h5>
<span className="badge bg-success ms-2">Eco</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
<MDBCardBody>
<a href="#!" className="text-reset">
<h5 className="card-title mb-3">Product name</h5>
</a>
<a href="#!" className="text-reset">
<p>Category</p>
</a>
<h6 className="mb-3">$61.99</h6>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBCard>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/shoes%20(3).webp"
fluid
className="w-100"
/>
<a href="#!">
<div className="mask">
<div className="d-flex justify-content-start align-items-end h-100">
<h5>
<span className="badge bg-danger ms-2">-10%</span>
</h5>
</div>
</div>
<div class="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
<MDBCardBody>
<a href="#!" className="text-reset">
<h5 className="card-title mb-3">Product name</h5>
</a>
<a href="#!" className="text-reset">
<p>Category</p>
</a>
<h6 className="mb-3">
<s>$61.99</s>
<strong className="ms-2 text-danger">$50.99</strong>
</h6>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="12" lg="4" className="mb-4">
<MDBCard>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/img%20(23).webp"
fluid
className="w-100"
/>
<a href="#!">
<div className="mask">
<div className="d-flex justify-content-start align-items-end h-100">
<h5>
<span className="badge bg-success ms-2">Eco</span>
<span className="badge bg-danger ms-2">-10%</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
<MDBCardBody>
<a href="#!" className="text-reset">
<h5 className="card-title mb-3">Product name</h5>
</a>
<a href="#!" className="text-reset">
<p>Category</p>
</a>
<h6 className="mb-3">
<s>$61.99</s>
<strong className="ms-2 text-danger">$50.99</strong>
</h6>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBCard>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/img%20(17).webp"
fluid
className="w-100"
/>
<a href="#!">
<div className="mask">
<div class="d-flex justify-content-start align-items-end h-100"></div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
<MDBCardBody>
<a href="#!" className="text-reset">
<h5 className="card-title mb-3">Product name</h5>
</a>
<a href="#!" className="text-reset">
<p>Category</p>
</a>
<h6 className="mb-3">$61.99</h6>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBCard>
<MDBRipple
rippleColor="light"
rippleTag="div"
className="bg-image rounded hover-zoom"
>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/img%20(30).webp"
fluid
className="w-100"
/>
<a href="#!">
<div className="mask">
<div class="d-flex justify-content-start align-items-end h-100">
<h5>
<span className="badge bg-primary ms-2">New</span>
<span className="badge bg-success ms-2">Eco</span>
<span className="badge bg-danger ms-2">-10%</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
<MDBCardBody>
<a href="#!" className="text-reset">
<h5 className="card-title mb-3">Product name</h5>
</a>
<a href="#!" className="text-reset">
<p>Category</p>
</a>
<h6 className="mb-3">
<s>$61.99</s>
<strong className="ms-2 text-danger">$50.99</strong>
</h6>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}
Product cards listing
Product listing eCommerce section.
import React from "react";
import { MDBContainer, MDBRow, MDBCol, MDBRipple } from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer fluid className="my-5 text-center">
<h4 className="mt-4 mb-5">
<strong>Product Listing</strong>
</h4>
<MDBRow>
<MDBCol md="12" lg="4" className="mb-4">
<MDBRipple
rippleColor="dark"
rippleTag="div"
className="bg-image rounded hover-zoom shadow-1-strong"
>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(1).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(0, 0, 0, 0.3)" }}
>
<div className="d-flex justify-content-start align-items-start h-100">
<h5>
<span className="badge bg-light pt-2 ms-3 mt-3 text-dark">
$123
</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBRipple
rippleColor="dark"
rippleTag="div"
className="bg-image rounded hover-zoom shadow-1-strong"
>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(2).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(0, 0, 0, 0.3)" }}
>
<div className="d-flex justify-content-start align-items-start h-100">
<h5>
<span className="badge bg-light pt-2 ms-3 mt-3 text-dark">
$239
</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBRipple
rippleColor="dark"
rippleTag="div"
className="bg-image rounded hover-zoom shadow-1-strong"
>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(3).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(0, 0, 0, 0.3)" }}
>
<div className="d-flex justify-content-start align-items-start h-100">
<h5>
<span className="badge bg-light pt-2 ms-3 mt-3 text-dark">
$147
</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md="12" lg="4" className="mb-4">
<MDBRipple
rippleColor="dark"
rippleTag="div"
className="bg-image rounded hover-zoom shadow-1-strong"
>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(4).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(0, 0, 0, 0.3)" }}
>
<div className="d-flex justify-content-start align-items-start h-100">
<h5>
<span className="badge bg-light pt-2 ms-3 mt-3 text-dark">
$83
</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBRipple
rippleColor="dark"
rippleTag="div"
className="bg-image rounded hover-zoom shadow-1-strong"
>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(5).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(0, 0, 0, 0.3)" }}
>
<div className="d-flex justify-content-start align-items-start h-100">
<h5>
<span className="badge bg-light pt-2 ms-3 mt-3 text-dark">
$106
</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6" lg="4" className="mb-4">
<MDBRipple
rippleColor="dark"
rippleTag="div"
className="bg-image rounded hover-zoom shadow-1-strong"
>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/new/img(6).webp"
fluid
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(0, 0, 0, 0.3)" }}
>
<div className="d-flex justify-content-start align-items-start h-100">
<h5>
<span className="badge bg-light pt-2 ms-3 mt-3 text-dark">
$58
</span>
</h5>
</div>
</div>
<div className="hover-overlay">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</div>
</a>
</MDBRipple>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #eee;
}