Profiles
React Bootstrap 5 Profile page & profile cards
Responsive profile pages and cards built with React Bootstrap 5. User profile card, profile picture, followers, avatars, comments, social stats, edit profile form.Basic profile card
A simple profile card template with chat option, square avatar and stat counters.
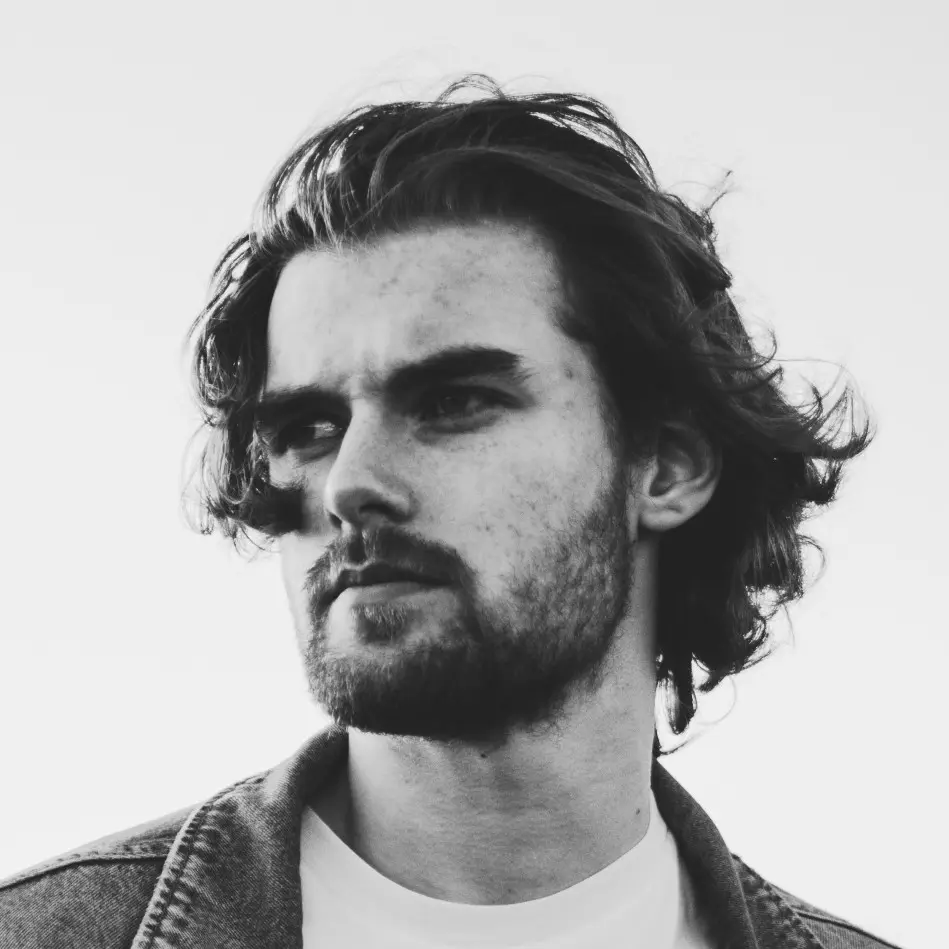
Danny McLoan
Senior Journalist
Articles
41
Followers
976
Rating
8.5
import React from 'react';
import { MDBCol, MDBContainer, MDBRow, MDBCard, MDBCardTitle, MDBCardText, MDBCardBody, MDBCardImage, MDBBtn } from 'mdb-react-ui-kit';
export default function Basic() {
return (
<div className="vh-100" style={{ backgroundColor: '#9de2ff' }}>
<MDBContainer>
<MDBRow className="justify-content-center">
<MDBCol md="9" lg="7" xl="5" className="mt-5">
<MDBCard style={{ borderRadius: '15px' }}>
<MDBCardBody className="p-4">
<div className="d-flex text-black">
<div className="flex-shrink-0">
<MDBCardImage
style={{ width: '180px', borderRadius: '10px' }}
src='https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-profiles/avatar-1.webp'
alt='Generic placeholder image'
fluid />
</div>
<div className="flex-grow-1 ms-3">
<MDBCardTitle>Danny McLoan</MDBCardTitle>
<MDBCardText>Senior Journalist</MDBCardText>
<div className="d-flex justify-content-start rounded-3 p-2 mb-2"
style={{ backgroundColor: '#efefef' }}>
<div>
<p className="small text-muted mb-1">Articles</p>
<p className="mb-0">41</p>
</div>
<div className="px-3">
<p className="small text-muted mb-1">Followers</p>
<p className="mb-0">976</p>
</div>
<div>
<p className="small text-muted mb-1">Rating</p>
<p className="mb-0">8.5</p>
</div>
</div>
<div className="d-flex pt-1">
<MDBBtn outline className="me-1 flex-grow-1">Chat</MDBBtn>
<MDBBtn className="flex-grow-1">Follow</MDBBtn>
</div>
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</div>
);
}
eCommerce profile card
A eCommerce profile card with a star rating number of comments / testimonial reviews and a "Book now" call to action button. The CTA in this case could be integrated with a calendar schedule.
Exquisite hand henna tattoo
3 hrs
$90
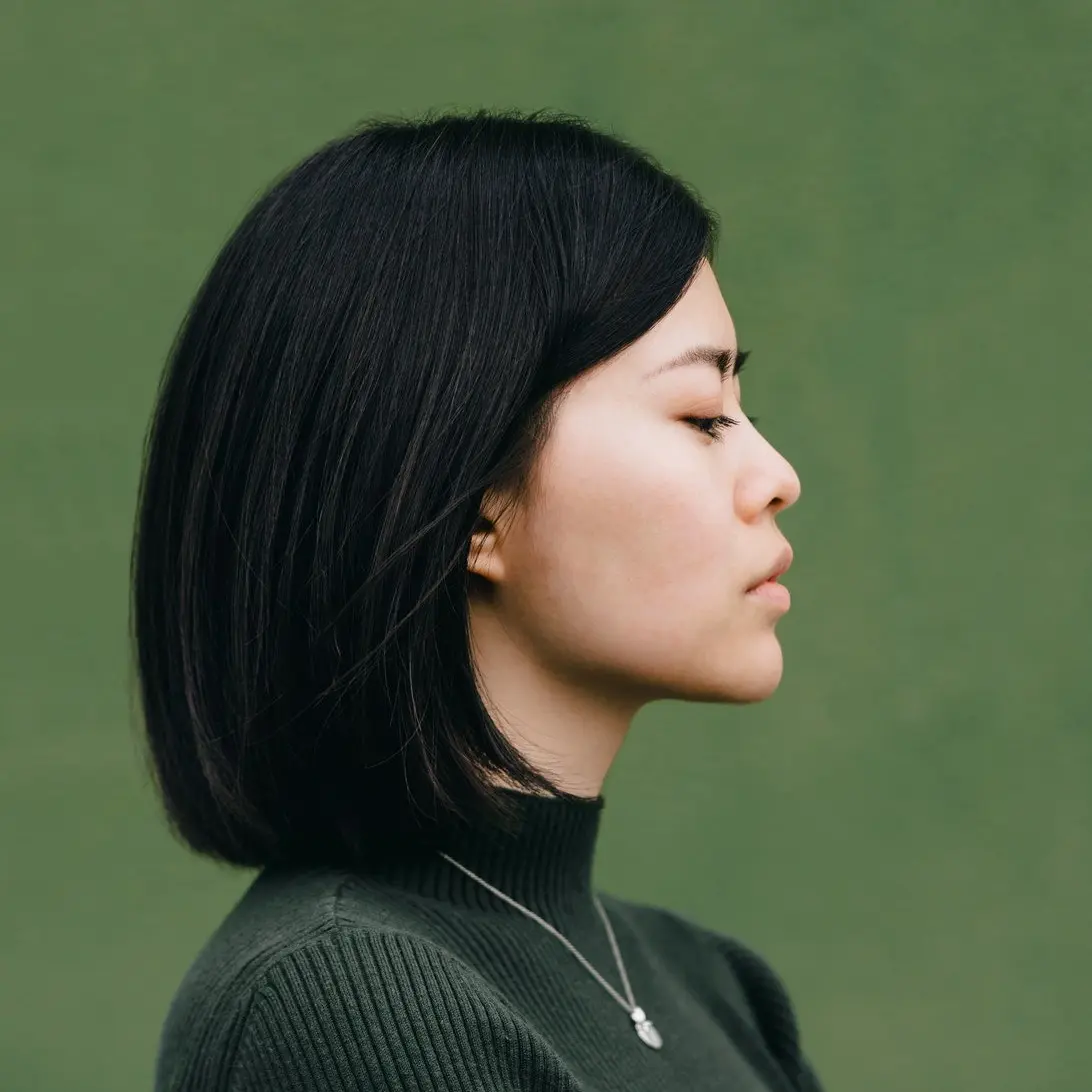
@sheisme
52 comments
import React from 'react';
import { MDBCol, MDBContainer, MDBRow, MDBCard, MDBCardText, MDBCardBody, MDBCardImage, MDBBtn, MDBTypography, MDBIcon } from 'mdb-react-ui-kit';
export default function ECommerce() {
return (
<div className="vh-100" style={{ backgroundColor: '#eee' }}>
<MDBContainer>
<MDBRow className="justify-content-center">
<MDBCol md="9" lg="7" xl="5" className="mt-5">
<MDBCard style={{ borderRadius: '15px', backgroundColor: '#93e2bb' }}>
<MDBCardBody className="p-4 text-black">
<div>
<MDBTypography tag='h6'>Exquisite hand henna tattoo</MDBTypography>
<div className="d-flex align-items-center justify-content-between mb-3">
<p className="small mb-0"><MDBIcon far icon="clock me-2" />3 hrs</p>
<p className="fw-bold mb-0">$90</p>
</div>
</div>
<div className="d-flex align-items-center mb-4">
<div className="flex-shrink-0">
<MDBCardImage
style={{ width: '70px' }}
className="img-fluid rounded-circle border border-dark border-3"
src='https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-profiles/avatar-2.webp'
alt='Generic placeholder image'
fluid />
</div>
<div className="flex-grow-1 ms-3">
<div className="d-flex flex-row align-items-center mb-2">
<p className="mb-0 me-2">@sheisme</p>
<ul className="mb-0 list-unstyled d-flex flex-row" style={{ color: '#1B7B2C' }}>
<li>
<MDBIcon fas icon="star fa-xs" />
</li>
<li>
<MDBIcon fas icon="star fa-xs" />
</li>
<li>
<MDBIcon fas icon="star fa-xs" />
</li>
<li>
<MDBIcon fas icon="star fa-xs" />
</li>
<li>
<MDBIcon fas icon="star fa-xs" />
</li>
</ul>
</div>
<div>
<MDBBtn outline color="dark" rounded size="sm">+ Follow</MDBBtn>
<MDBBtn outline color="dark" rounded size="sm" className="mx-1">See profile</MDBBtn>
<MDBBtn outline color="dark" floating size="sm"><MDBIcon fas icon="comment" /></MDBBtn>
</div>
</div>
</div>
<hr />
<MDBCardText>52 comments</MDBCardText>
<MDBBtn color="success" rounded block size="lg">
<MDBIcon far icon="clock me-2" /> Book now
</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</div>
);
}
User profile page template
A full user profile layout, with a circle profile picture in the header, projects section with social icons, a detailed address section and experience cards using progress bars to indicate skill level.
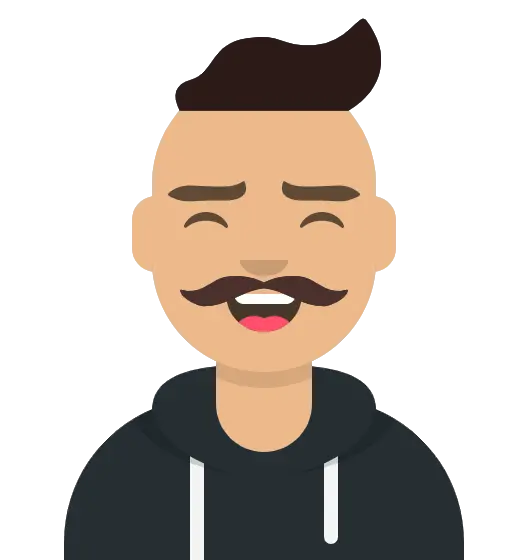
John Smith
Full Stack Developer
Bay Area, San Francisco, CA
-
https://mdbootstrap.com
-
mdbootstrap
-
@mdbootstrap
-
mdbootstrap
-
mdbootstrap
Full Name
Johnatan Smith
example@example.com
Phone
(097) 234-5678
Mobile
(098) 765-4321
Address
Bay Area, San Francisco, CA
assigment Project Status
Web Design
Website Markup
One Page
Mobile Template
Backend API
assigment Project Status
Web Design
Website Markup
One Page
Mobile Template
Backend API
import React from 'react';
import {
MDBCol,
MDBContainer,
MDBRow,
MDBCard,
MDBCardText,
MDBCardBody,
MDBCardImage,
MDBBtn,
MDBBreadcrumb,
MDBBreadcrumbItem,
MDBProgress,
MDBProgressBar,
MDBIcon,
MDBListGroup,
MDBListGroupItem
} from 'mdb-react-ui-kit';
export default function ProfilePage() {
return (
<section style={{ backgroundColor: '#eee' }}>
<MDBContainer className="py-5">
<MDBRow>
<MDBCol>
<MDBBreadcrumb className="bg-light rounded-3 p-3 mb-4">
<MDBBreadcrumbItem>
<a href='#'>Home</a>
</MDBBreadcrumbItem>
<MDBBreadcrumbItem>
<a href="#">User</a>
</MDBBreadcrumbItem>
<MDBBreadcrumbItem active>User Profile</MDBBreadcrumbItem>
</MDBBreadcrumb>
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol lg="4">
<MDBCard className="mb-4">
<MDBCardBody className="text-center">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3.webp"
alt="avatar"
className="rounded-circle"
style={{ width: '150px' }}
fluid />
<p className="text-muted mb-1">Full Stack Developer</p>
<p className="text-muted mb-4">Bay Area, San Francisco, CA</p>
<div className="d-flex justify-content-center mb-2">
<MDBBtn>Follow</MDBBtn>
<MDBBtn outline className="ms-1">Message</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-4 mb-lg-0">
<MDBCardBody className="p-0">
<MDBListGroup flush className="rounded-3">
<MDBListGroupItem className="d-flex justify-content-between align-items-center p-3">
<MDBIcon fas icon="globe fa-lg text-warning" />
<MDBCardText>https://mdbootstrap.com</MDBCardText>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center p-3">
<MDBIcon fab icon="github fa-lg" style={{ color: '#333333' }} />
<MDBCardText>mdbootstrap</MDBCardText>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center p-3">
<MDBIcon fab icon="twitter fa-lg" style={{ color: '#55acee' }} />
<MDBCardText>@mdbootstrap</MDBCardText>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center p-3">
<MDBIcon fab icon="instagram fa-lg" style={{ color: '#ac2bac' }} />
<MDBCardText>mdbootstrap</MDBCardText>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center p-3">
<MDBIcon fab icon="facebook fa-lg" style={{ color: '#3b5998' }} />
<MDBCardText>mdbootstrap</MDBCardText>
</MDBListGroupItem>
</MDBListGroup>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol lg="8">
<MDBCard className="mb-4">
<MDBCardBody>
<MDBRow>
<MDBCol sm="3">
<MDBCardText>Full Name</MDBCardText>
</MDBCol>
<MDBCol sm="9">
<MDBCardText className="text-muted">Johnatan Smith</MDBCardText>
</MDBCol>
</MDBRow>
<hr />
<MDBRow>
<MDBCol sm="3">
<MDBCardText>Email</MDBCardText>
</MDBCol>
<MDBCol sm="9">
<MDBCardText className="text-muted">example@example.com</MDBCardText>
</MDBCol>
</MDBRow>
<hr />
<MDBRow>
<MDBCol sm="3">
<MDBCardText>Phone</MDBCardText>
</MDBCol>
<MDBCol sm="9">
<MDBCardText className="text-muted">(097) 234-5678</MDBCardText>
</MDBCol>
</MDBRow>
<hr />
<MDBRow>
<MDBCol sm="3">
<MDBCardText>Mobile</MDBCardText>
</MDBCol>
<MDBCol sm="9">
<MDBCardText className="text-muted">(098) 765-4321</MDBCardText>
</MDBCol>
</MDBRow>
<hr />
<MDBRow>
<MDBCol sm="3">
<MDBCardText>Address</MDBCardText>
</MDBCol>
<MDBCol sm="9">
<MDBCardText className="text-muted">Bay Area, San Francisco, CA</MDBCardText>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBRow>
<MDBCol md="6">
<MDBCard className="mb-4 mb-md-0">
<MDBCardBody>
<MDBCardText className="mb-4"><span className="text-primary font-italic me-1">assigment</span> Project Status</MDBCardText>
<MDBCardText className="mb-1" style={{ fontSize: '.77rem' }}>Web Design</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={80} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>Website Markup</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={72} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>One Page</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={89} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>Mobile Template</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={55} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>Backend API</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={66} valuemin={0} valuemax={100} />
</MDBProgress>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6">
<MDBCard className="mb-4 mb-md-0">
<MDBCardBody>
<MDBCardText className="mb-4"><span className="text-primary font-italic me-1">assigment</span> Project Status</MDBCardText>
<MDBCardText className="mb-1" style={{ fontSize: '.77rem' }}>Web Design</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={80} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>Website Markup</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={72} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>One Page</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={89} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>Mobile Template</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={55} valuemin={0} valuemax={100} />
</MDBProgress>
<MDBCardText className="mt-4 mb-1" style={{ fontSize: '.77rem' }}>Backend API</MDBCardText>
<MDBProgress className="rounded">
<MDBProgressBar width={66} valuemin={0} valuemax={100} />
</MDBProgress>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Project cards with attending users
The list of attendance in this example is indicated by profile pictures of users that joined the event.
import React from 'react';
import {
MDBCol,
MDBContainer,
MDBRow,
MDBCard,
MDBCardText,
MDBCardBody,
MDBCardImage,
MDBBtn,
MDBTypography,
MDBIcon
} from 'mdb-react-ui-kit';
export default function AttendingUsers() {
return (
<section className="vh-100" style={{ backgroundColor: '#5f59f7' }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol xl="10">
<MDBCard className="mb-5" style={{ borderRadius: '15px' }}>
<MDBCardBody className="p-4">
<MDBTypography tag='h3'>Program Title</MDBTypography>
<MDBCardText className="small">
<MDBIcon far icon="star" size="lg" />
<span className="mx-2">|</span> Created by <strong>MDBootstrap</strong> on 11 April , 2021
</MDBCardText>
<hr className="my-4" />
<div className="d-flex justify-content-start align-items-center">
<MDBCardText className="text-uppercase mb-0">
<MDBIcon fas icon="cog me-2" /> <span className="text-muted small">settings</span>
</MDBCardText>
<MDBCardText className="text-uppercase mb-0">
<MDBIcon fas icon="link ms-4 me-2" /> <span className="text-muted small">program link</span>
</MDBCardText>
<MDBCardText className="text-uppercase mb-0">
<MDBIcon fas icon="ellipsis-h ms-4 me-2" /> <span className="text-muted small">program link</span> <span className="ms-3 me-4">|</span>
</MDBCardText>
<a href="#!">
<MDBCardImage
width="35"
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-2.webp"
alt="avatar"
className="rounded-circle me-3"
fluid />
</a>
<MDBBtn outline color="dark" floating size="sm">
<MDBIcon fas icon="plus" />
</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-5" style={{ borderRadius: '15px' }}>
<MDBCardBody className="p-4">
<MDBTypography tag='h3'>Company Culture</MDBTypography>
<MDBCardText className="small">
<MDBIcon fas icon="star text-warning" size="lg" />
<span className="mx-2">|</span> Public <span className="mx-2">|</span> Updated by <strong>MDBootstrap</strong> on 11 April , 2021
</MDBCardText>
<hr className="my-4" />
<div className="d-flex justify-content-start align-items-center">
<MDBCardText className="text-uppercase mb-0">
<MDBIcon fas icon="cog me-2" /> <span className="text-muted small">settings</span>
</MDBCardText>
<MDBCardText className="text-uppercase mb-0">
<MDBIcon fas icon="link ms-4 me-2" /> <span className="text-muted small">program link</span>
</MDBCardText>
<MDBCardText className="text-uppercase mb-0">
<MDBIcon fas icon="ellipsis-h ms-4 me-2" /> <span className="text-muted small">program link</span> <span className="ms-3 me-4">|</span>
</MDBCardText>
<a href="#!">
<MDBCardImage width="35" src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-2.webp" alt="avatar" className="rounded-circle me-1" fluid />
<MDBCardImage width="35" src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-3.webp" alt="avatar" className="rounded-circle me-1" fluid />
<MDBCardImage width="35" src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-4.webp" alt="avatar" className="rounded-circle me-1" fluid />
<MDBCardImage width="35" src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-5.webp" alt="avatar" className="rounded-circle me-3" fluid />
</a>
<MDBBtn outline color="dark" floating size="sm">
<MDBIcon fas icon="plus" />
</MDBBtn>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Personal profile with edit icon
Responsive profile card design with a clickable update option for the user. Check out the inputs documentation, to integrate & make this template interactive.
import React from 'react';
import { MDBCol, MDBContainer, MDBRow, MDBCard, MDBCardText, MDBCardBody, MDBCardImage, MDBTypography, MDBIcon } from 'mdb-react-ui-kit';
export default function PersonalProfile() {
return (
<section className="vh-100" style={{ backgroundColor: '#f4f5f7' }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="6" className="mb-4 mb-lg-0">
<MDBCard className="mb-3" style={{ borderRadius: '.5rem' }}>
<MDBRow className="g-0">
<MDBCol md="4" className="gradient-custom text-center text-white"
style={{ borderTopLeftRadius: '.5rem', borderBottomLeftRadius: '.5rem' }}>
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="Avatar" className="my-5" style={{ width: '80px' }} fluid />
<MDBTypography tag="h5">Marie Horwitz</MDBTypography>
<MDBCardText>Web Designer</MDBCardText>
<MDBIcon far icon="edit mb-5" />
</MDBCol>
<MDBCol md="8">
<MDBCardBody className="p-4">
<MDBTypography tag="h6">Information</MDBTypography>
<hr className="mt-0 mb-4" />
<MDBRow className="pt-1">
<MDBCol size="6" className="mb-3">
<MDBTypography tag="h6">Email</MDBTypography>
<MDBCardText className="text-muted">info@example.com</MDBCardText>
</MDBCol>
<MDBCol size="6" className="mb-3">
<MDBTypography tag="h6">Phone</MDBTypography>
<MDBCardText className="text-muted">123 456 789</MDBCardText>
</MDBCol>
</MDBRow>
<MDBTypography tag="h6">Information</MDBTypography>
<hr className="mt-0 mb-4" />
<MDBRow className="pt-1">
<MDBCol size="6" className="mb-3">
<MDBTypography tag="h6">Email</MDBTypography>
<MDBCardText className="text-muted">info@example.com</MDBCardText>
</MDBCol>
<MDBCol size="6" className="mb-3">
<MDBTypography tag="h6">Phone</MDBTypography>
<MDBCardText className="text-muted">123 456 789</MDBCardText>
</MDBCol>
</MDBRow>
<div className="d-flex justify-content-start">
<a href="#!"><MDBIcon fab icon="facebook me-3" size="lg" /></a>
<a href="#!"><MDBIcon fab icon="twitter me-3" size="lg" /></a>
<a href="#!"><MDBIcon fab icon="instagram me-3" size="lg" /></a>
</div>
</MDBCardBody>
</MDBCol>
</MDBRow>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
.gradient-custom {
/* fallback for old browsers */
background: #f6d365;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right bottom, rgba(246, 211, 101, 1), rgba(253, 160, 133, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right bottom, rgba(246, 211, 101, 1), rgba(253, 160, 133, 1))
}
Edit button on profile page
An elegant profile page layout, with an edit button, about section and a photo gallery.
import React from 'react';
import { MDBCol, MDBContainer, MDBRow, MDBCard, MDBCardText, MDBCardBody, MDBCardImage, MDBBtn, MDBTypography } from 'mdb-react-ui-kit';
export default function EditButton() {
return (
<div className="gradient-custom-2" style={{ backgroundColor: '#9de2ff' }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="9" xl="7">
<MDBCard>
<div className="rounded-top text-white d-flex flex-row" style={{ backgroundColor: '#000', height: '200px' }}>
<div className="ms-4 mt-5 d-flex flex-column" style={{ width: '150px' }}>
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-profiles/avatar-1.webp"
alt="Generic placeholder image" className="mt-4 mb-2 img-thumbnail" fluid style={{ width: '150px', zIndex: '1' }} />
<MDBBtn outline color="dark" style={{height: '36px', overflow: 'visible'}}>
Edit profile
</MDBBtn>
</div>
<div className="ms-3" style={{ marginTop: '130px' }}>
<MDBTypography tag="h5">Andy Horwitz</MDBTypography>
<MDBCardText>New York</MDBCardText>
</div>
</div>
<div className="p-4 text-black" style={{ backgroundColor: '#f8f9fa' }}>
<div className="d-flex justify-content-end text-center py-1">
<div>
<MDBCardText className="mb-1 h5">253</MDBCardText>
<MDBCardText className="small text-muted mb-0">Photos</MDBCardText>
</div>
<div className="px-3">
<MDBCardText className="mb-1 h5">1026</MDBCardText>
<MDBCardText className="small text-muted mb-0">Followers</MDBCardText>
</div>
<div>
<MDBCardText className="mb-1 h5">478</MDBCardText>
<MDBCardText className="small text-muted mb-0">Following</MDBCardText>
</div>
</div>
</div>
<MDBCardBody className="text-black p-4">
<div className="mb-5">
<p className="lead fw-normal mb-1">About</p>
<div className="p-4" style={{ backgroundColor: '#f8f9fa' }}>
<MDBCardText className="font-italic mb-1">Web Developer</MDBCardText>
<MDBCardText className="font-italic mb-1">Lives in New York</MDBCardText>
<MDBCardText className="font-italic mb-0">Photographer</MDBCardText>
</div>
</div>
<div className="d-flex justify-content-between align-items-center mb-4">
<MDBCardText className="lead fw-normal mb-0">Recent photos</MDBCardText>
<MDBCardText className="mb-0"><a href="#!" className="text-muted">Show all</a></MDBCardText>
</div>
<MDBRow>
<MDBCol className="mb-2">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/Lightbox/Original/img%20(112).webp"
alt="image 1" className="w-100 rounded-3" />
</MDBCol>
<MDBCol className="mb-2">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/Lightbox/Original/img%20(107).webp"
alt="image 1" className="w-100 rounded-3" />
</MDBCol>
</MDBRow>
<MDBRow className="g-2">
<MDBCol className="mb-2">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/Lightbox/Original/img%20(108).webp"
alt="image 1" className="w-100 rounded-3" />
</MDBCol>
<MDBCol className="mb-2">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/Lightbox/Original/img%20(114).webp"
alt="image 1" className="w-100 rounded-3" />
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</div>
);
}
.gradient-custom-2 {
/* fallback for old browsers */
background: #fbc2eb;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(251, 194, 235, 1), rgba(166, 193, 238, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(251, 194, 235, 1), rgba(166, 193, 238, 1))
}
Profile card with statistics
A light design for any profile card, with social buttons and basic stats.
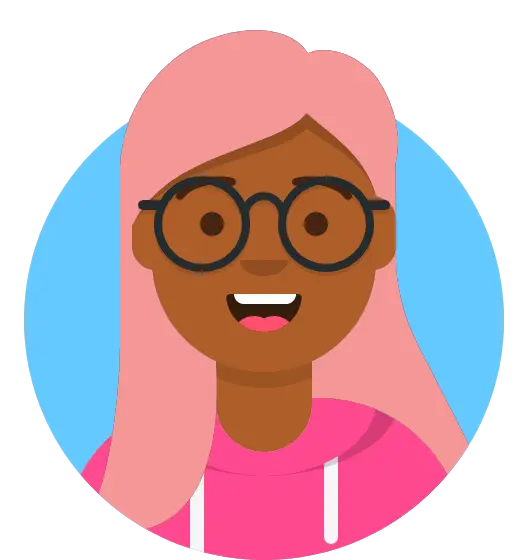
Julie L. Arsenault
@Programmer | mdbootstrap.com
8471
Wallets Balance
8512
Income amounts
4751
Total Transactions
import React from 'react';
import { MDBCol, MDBContainer, MDBRow, MDBCard, MDBCardText, MDBCardBody, MDBCardImage, MDBBtn, MDBTypography, MDBIcon } from 'mdb-react-ui-kit';
export default function ProfileStatistics() {
return (
<div className="vh-100" style={{ backgroundColor: '#eee' }}>
<MDBContainer className="container py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol md="12" xl="4">
<MDBCard style={{ borderRadius: '15px' }}>
<MDBCardBody className="text-center">
<div className="mt-3 mb-4">
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
className="rounded-circle" fluid style={{ width: '100px' }} />
</div>
<MDBTypography tag="h4">Julie L. Arsenault</MDBTypography>
<MDBCardText className="text-muted mb-4">
@Programmer <span className="mx-2">|</span> <a href="#!">mdbootstrap.com</a>
</MDBCardText>
<div className="mb-4 pb-2">
<MDBBtn outline floating>
<MDBIcon fab icon="facebook" size="lg" />
</MDBBtn>
<MDBBtn outline floating className="mx-1">
<MDBIcon fab icon="twitter" size="lg" />
</MDBBtn>
<MDBBtn outline floating>
<MDBIcon fab icon="skype" size="lg" />
</MDBBtn>
</div>
<MDBBtn rounded size="lg">
Message now
</MDBBtn>
<div className="d-flex justify-content-between text-center mt-5 mb-2">
<div>
<MDBCardText className="mb-1 h5">8471</MDBCardText>
<MDBCardText className="small text-muted mb-0">Wallets Balance</MDBCardText>
</div>
<div className="px-3">
<MDBCardText className="mb-1 h5">8512</MDBCardText>
<MDBCardText className="small text-muted mb-0">Followers</MDBCardText>
</div>
<div>
<MDBCardText className="mb-1 h5">4751</MDBCardText>
<MDBCardText className="small text-muted mb-0">Total Transactions</MDBCardText>
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</div>
);
}