Weather
React Bootstrap 5 Weather templates & widgets
Responsive React Weather templates built with the latest Bootstrap 5. Examples of weather dashboards with icons, cards, real data from API, interactive animated backgrounds & moreBasic weather widget
Simple weather card with temperature, wind & humidity metrics.
Warsaw
15:07
13°C
Stormy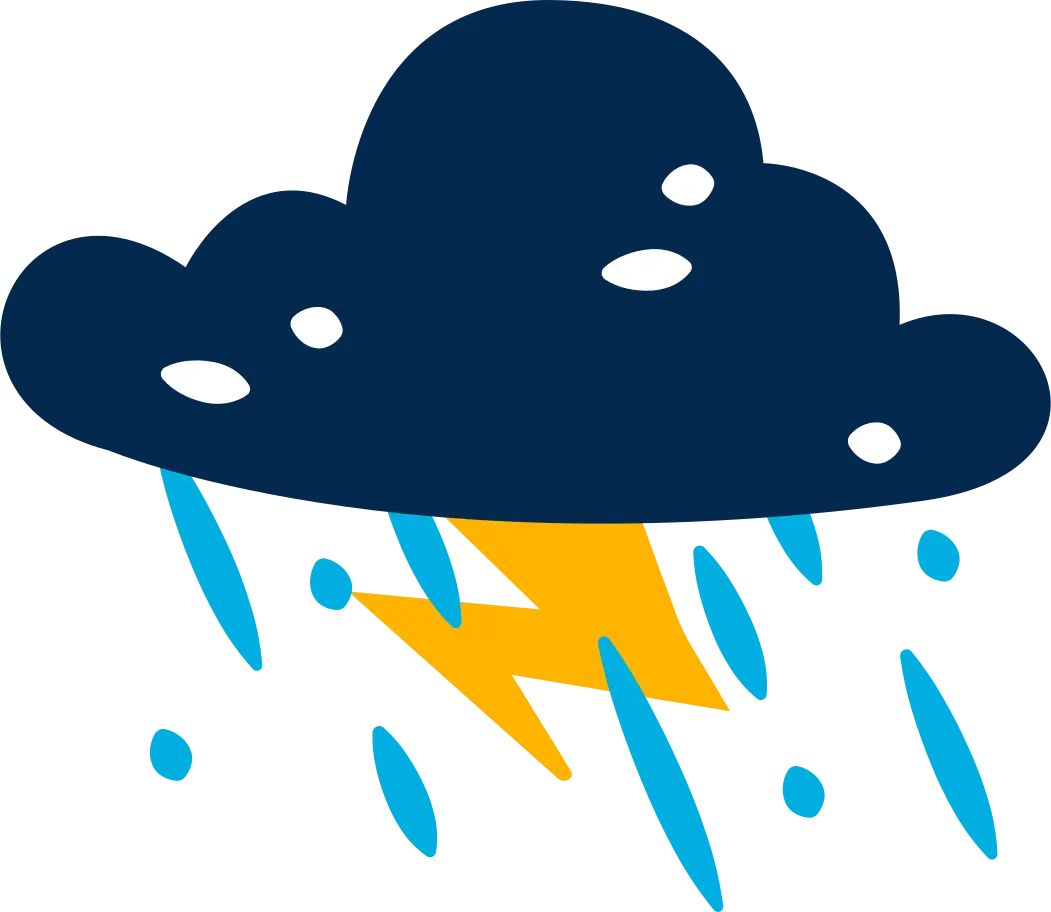
Weather App
A simple application design for checking the weather in a given city.
Weather dashboard with API
A simple dashboard displaying current & future weather with data from free API setup using JavaScript.
Weather card
Minimalistic weather cards with background image reflecting temperature and clouds & sun icons.
Current Weather info card
An illustrated card with current weather information.
Weather report
A minimalistic weather report dashboard with white background and black borders and a simple table for weather data.
Toronto
21.02.2021
Rain map
-4°C
10:00
Sunday
Cloudy
Sun
-4°C
Mon
-4°C
Tue
-4°C
Wed
-4°C
Thu
-4°C
Fri
-4°C
Sat
-4°C
For a month
11:00 -4°
12:00 -4°
13:00 -5°
14:00 -7°
15:00 -6°
16:00 -4°
17:00 -3°
Weather card UI
Beautiful weather widget styling, with basic weather info.