Images
React Bootstrap 5 Images component
Documentation and examples for opting images into responsive behavior (so they never become larger than their parent elements) and add lightweight styles to them—all via classes.
Responsive images
Images in MDB are made responsive with .img-fluid
. This applies
max-width: 100%;
and height: auto;
to the image so that it scales
with the parent element.
import React from 'react';
export default function App() {
return (
<img src='https://mdbootstrap.com/img/new/slides/041.webp' className='img-fluid shadow-4' alt='...' />
);
}
Thumbnails
In addition to our
border-radius utilities, you can use
.img-thumbnail
to give an image a rounded 1px border appearance.
import React from 'react';
export default function App() {
return (
<img
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
className='img-thumbnail'
alt='...'
/>
);
}
Shadows
By using our
shadow classes you can add a shadow to
the image. In the example below, we add shadow-2-strong
class.
import React from 'react';
export default function App() {
return (
<img
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
className='img-fluid shadow-2-strong'
alt=''
/>
);
}
By adding .hover-shadow
class to the element you can apply a shadow hover effect.
import React from 'react';
export default function App() {
return (
<img
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
className='img-fluid hover-shadow'
alt=''
/>
);
}
Ripple
You can change the image into a clickable element and apply a
ripple effect to it by wrapping it in
MDBRipple
.
import React from 'react';
import { MDBRipple } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRipple rippleTag='a'>
<img
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
className='img-fluid rounded'
alt='example'
/>
</MDBRipple>
);
}
Masks
You can cover the image with mask to achieve the desired contrast and for example, place text on it.
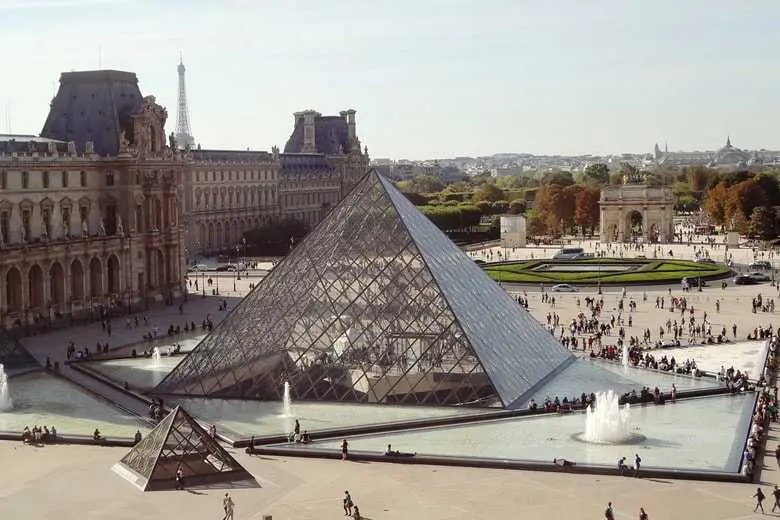
Can you see me?
import React from 'react';
export default function App() {
return (
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' alt='Sample' />
<div className='mask' style={{ backgroundColor: 'rgba(0, 0, 0, 0.6)' }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Can you see me?</p>
</div>
</div>
</div>
);
}
Hover effects
By using .hover-overlay
class you can apply gentle and decorative
hover effects to the image.
import React from 'react';
export default function App() {
return (
<div className='bg-image hover-overlay'>
<img src='https://mdbootstrap.com/img/new/fluid/city/055.webp' className='img-fluid' />
<a href='#!'>
<div className='mask overlay' style={{ backgroundColor: 'rgba(57, 192, 237, 0.2)' }}></div>
</a>
</div>
);
}
Shapes
By using border utilities you can change the shape of the image.
.rounded
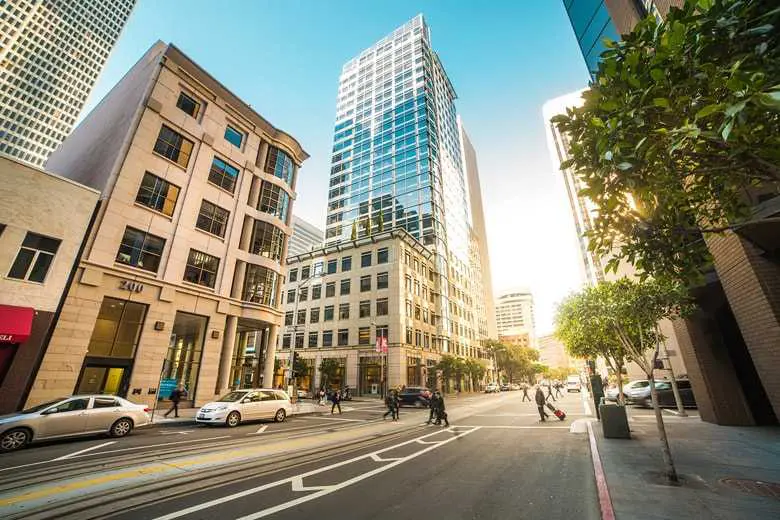
.rounded-circle
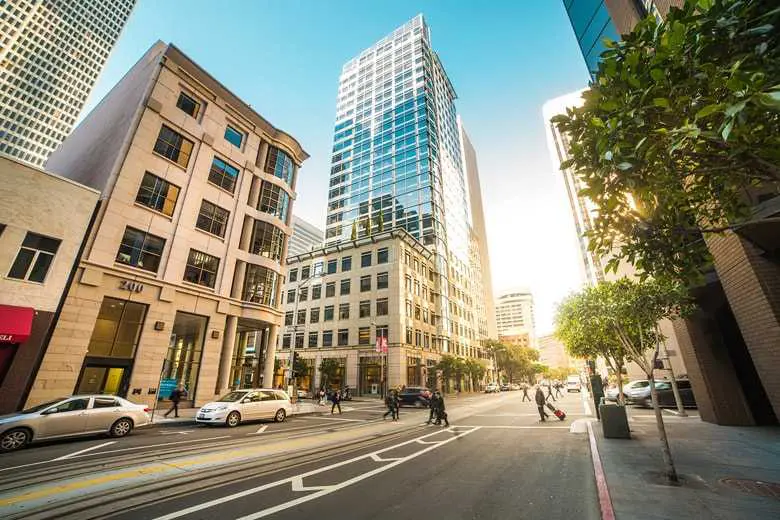
.rounded-pill
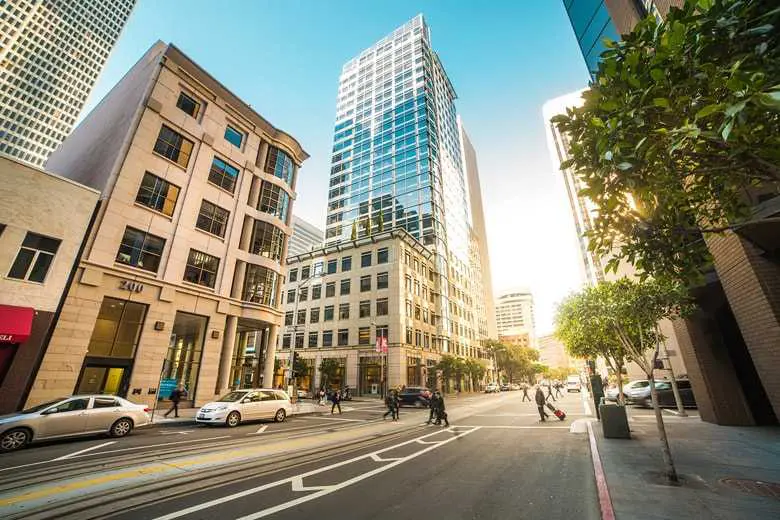
import React from 'react';
import { MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow>
<MDBCol lg='4' md='12' className='mb-4'>
<img src='https://mdbootstrap.com/img/new/standard/city/047.webp' className='img-fluid rounded' alt='' />
</MDBCol>
<MDBCol lg='4' md='6' className='mb-4'>
<img
src='https://mdbootstrap.com/img/new/standard/city/047.webp'
className='img-fluid rounded-circle'
alt=''
/>
</MDBCol>
<MDBCol lg='4' md='6' className='mb-4'>
<img
src='https://mdbootstrap.com/img/new/standard/city/047.webp'
className='img-fluid rounded-pill'
alt=''
/>
</MDBCol>
</MDBRow>
);
}
Images - API
CSS variables
As part of MDB’s evolving CSS variables approach, image now use local CSS variables for enhanced real-time customization. Values for the CSS variables are set via Sass, so Sass customization is still supported, too.
Images CSS variables are in different classes which belong to this component. To make it easier to use them, you can find below all of the used CSS variables.
// .hover-overlay
--#{$prefix}image-hover-transition: #{$image-hover-overlay-transition};
// .hover-zoom
--#{$prefix}image-hover-zoom-transition: #{$image-hover-zoom-transition};
--#{$prefix}image-hover-zoom-transform: #{$image-hover-zoom-transform};
// .hover-shadow
--#{$prefix}image-hover-shadow-transition: #{$image-hover-shadow-transition};
// .hover-shadow-soft
--#{$prefix}image-hover-shadow-box-shadow-soft: #{$image-hover-shadow-box-shadow-soft};
SCSS variables
$thumbnail-padding: .25rem;
$thumbnail-bg: $body-bg;
$thumbnail-border-width: $border-width;
$thumbnail-border-color: var(--#{$prefix}border-color);
$thumbnail-border-radius: $border-radius;
$thumbnail-box-shadow: $box-shadow-sm;
$image-hover-overlay-transition: all 0.3s ease-in-out;
$image-hover-zoom-transition: all 0.3s linear;
$image-hover-zoom-transform: scale(1.1);
$image-hover-shadow-transition: $image-hover-overlay-transition;
$image-hover-shadow-box-shadow: $box-shadow-4-strong;
$image-hover-shadow-box-shadow-soft: $box-shadow-5;