Avatar
React Bootstrap 5 Avatar
Responsive React Avatar built with Bootstrap 5. Examples of circle or square avatar, avatar in a card, inside the navbar, testimonial carousel, profile card & more.Basic example
This is a basic example of a circle-shaped avatar. The roundness is
achieved by adding the .rounded-circle
class. You can
experiment with different
border radius
to change the roundness of the image corners. Make sure to check out the
Image documentation
to learn more about image options and responsiveness.
import React from "react";
import { MDBContainer } from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer className="my-5 d-flex justify-content-center">
<img
src="https://mdbcdn.b-cdn.net/img/new/avatars/2.webp"
className="rounded-circle"
alt="Avatar"
/>
</MDBContainer>
);
}
export default App;
With shadows
Adding a shadow to your avatar will make it stand out. You can generate a custom shadow with our generator.
import React from "react";
import { MDBContainer } from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer className="my-5 d-flex justify-content-center">
<img
src="https://mdbcdn.b-cdn.net/img/new/avatars/1.webp"
className="rounded-circle shadow-4"
style={{ width: "150px" }}
alt="Avatar"
/>
</MDBContainer>
);
}
export default App;
Square
The default image shape is rectangular. If you need your avatar to be a square you need to make sure that your source image is of equal width an height, or overwrite them with CSS. You can also make the corners slightly rounded, like in the example below, in order to create a smoother design.
Rounded corners are applied with the .rounded-3
class, you can use different roundness levels from
our Border Radius docs, or
even create a fancy, irregular border shape with our Fancy Shapes Generator.
import React from "react";
import { MDBContainer } from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer className="my-5 d-flex justify-content-center">
<img
src="https://mdbcdn.b-cdn.net/img/new/avatars/5.webp"
className="rounded-3"
style={{ width: "150px" }}
alt="Avatar"
/>
</MDBContainer>
);
}
export default App;
With content
Add additional content to your avatar. The most common use case is adding name and surname along with a text description, but you can also use a variety of other elements like Badges, Buttons, Text Animations, Icons or even Flags that indicate the country of origin.
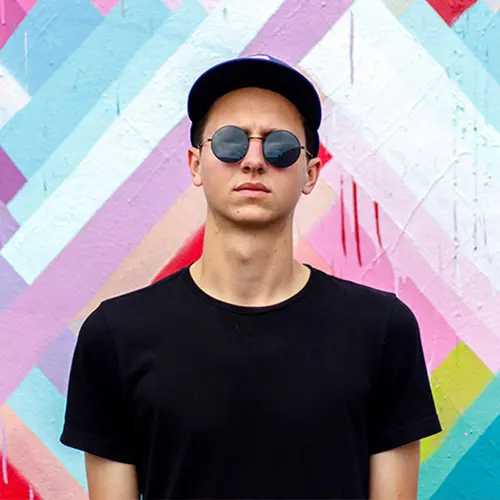
John Doe
Web designer PRO
import React from "react";
import { MDBContainer } from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer className="my-5 d-flex flex-column justify-content-center align-items-center">
<img
src="https://mdbcdn.b-cdn.net/img/new/avatars/8.webp"
className="rounded-circle mb-3"
style={{ width: "150px" }}
alt="Avatar"
/>
<h5 className="mb-2">
<strong>John Doe</strong>
</h5>
<p className="text-muted">
Web designer <span className="badge bg-primary">PRO</span>
</p>
</MDBContainer>
);
}
export default App;
Carousel
Avatars are often used inside Testimonial Sliders and generally in many Review Designs
Besides the avatar itself, the key elements of a good review design are Star Ratings and customer opinions, usually presented with a preceding quotation mark icon.
You can also embed more than one review in a single slide, change the speed of carousel transitions and customize your testimonial slider even further with our Carousel documentation.
import React from "react";
import {
MDBCarousel,
MDBCarouselInner,
MDBCarouselItem,
MDBCarouselElement,
MDBCarouselCaption,
MDBContainer,
MDBRow,
MDBCol,
MDBIcon,
} from "mdb-react-ui-kit";
function App() {
return (
<MDBContainer className="my-5">
<MDBCarousel showControls dark fade>
<MDBCarouselInner>
<MDBCarouselItem className="active text-center">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(10).webp"
alt="avatar"
className="rounded-circle shadow-1-strong mb-4"
style={{ width: "150px" }}
/>
<MDBRow className="d-flex justify-content-center">
<MDBCol lg="8">
<h5 className="mb-3">Maria Kate</h5>
<p>Photographer</p>
<p className="text-muted">
<MDBIcon fas icon="quote-left" className="pe-2" />
Lorem ipsum dolor sit amet consectetur adipisicing elit. Minus
et deleniti nesciunt sint eligendi reprehenderit reiciendis,
quibusdam illo, beatae quia fugit consequatur laudantium velit
magnam error. Consectetur distinctio fugit doloremque.
</p>
</MDBCol>
</MDBRow>
<ul className="list-unstyled d-flex justify-content-center text-warning mb-0">
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon far icon="star" size="sm" />
</li>
</ul>
</MDBCarouselItem>
<MDBCarouselItem className="text-center">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(32).webp"
alt="avatar"
className="rounded-circle shadow-1-strong mb-4"
style={{ width: "150px" }}
/>
<MDBRow className="d-flex justify-content-center">
<MDBCol lg="8">
<h5 className="mb-3">John Doe</h5>
<p>Web Developer</p>
<p className="text-muted">
<MDBIcon fas icon="quote-left" className="pe-2" />
Lorem ipsum dolor sit amet consectetur adipisicing elit. Minus
et deleniti nesciunt sint eligendi reprehenderit reiciendis.
</p>
</MDBCol>
</MDBRow>
<ul className="list-unstyled d-flex justify-content-center text-warning mb-0">
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon far icon="star" size="sm" />
</li>
</ul>
</MDBCarouselItem>
<MDBCarouselItem className="text-center">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(1).webp"
alt="avatar"
className="rounded-circle shadow-1-strong mb-4"
style={{ width: "150px" }}
/>
<MDBRow className="d-flex justify-content-center">
<MDBCol lg="8">
<h5 className="mb-3">Anna Deynah</h5>
<p>Web Developer</p>
<p className="text-muted">
<MDBIcon fas icon="quote-left" className="pe-2" />
Lorem ipsum dolor sit amet consectetur adipisicing elit. Minus
et deleniti nesciunt sint eligendi reprehenderit reiciendis,
quibusdam illo, beatae quia fugit consequatur laudantium velit
magnam error. Consectetur distinctio fugit doloremque.
</p>
</MDBCol>
</MDBRow>
<ul className="list-unstyled d-flex justify-content-center text-warning mb-0">
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon fas icon="star" size="sm" />
</li>
<li>
<MDBIcon far icon="star" size="sm" />
</li>
</ul>
</MDBCarouselItem>
</MDBCarouselInner>
</MDBCarousel>
</MDBContainer>
);
}
export default App;
Testimonials
Customer reviews with avatars, can also work great within simple Cards.
Testimonials
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Fugit, error amet numquam iure provident voluptate esse quasi, veritatis totam voluptas nostrum quisquam eum porro a pariatur veniam.
.webp)
Maria Smantha
Lorem ipsum dolor sit amet eos adipisci, consectetur adipisicing elit.
.webp)
Lisa Cudrow
Neque cupiditate assumenda in maiores repudi mollitia architecto.
.webp)
John Smith
Delectus impedit saepe officiis ab aliquam repellat rem unde ducimus.
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBIcon,
} from "mdb-react-ui-kit";
import "./testimonials.css";
function App() {
return (
<MDBContainer className="my-5">
<MDBRow className="d-flex justify-content-center">
<MDBCol md="10" xl="8" className="text-center">
<h3 className="mb-4">Testimonials</h3>
<p classNAme="mb-4 pb-2 mb-md-5 pb-md-0">
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Fugit,
error amet numquam iure provident voluptate esse quasi, veritatis
totam voluptas nostrum quisquam eum porro a pariatur veniam.
</p>
</MDBCol>
</MDBRow>
<MDBRow className="text-center">
<MDBCol md="4" className="mb-5 mb-md-0">
<MDBCard className="testimonial-card">
<div
className="card-up"
style={{ backgroundColor: "#9d789b" }}
></div>
<div className="avatar mx-auto bg-white">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(1).webp"
className="rounded-circle img-fluid"
/>
</div>
<MDBCardBody>
<h4 className="mb-4">Maria Smantha</h4>
<hr />
<p className="dark-grey-text mt-4">
<MDBIcon fas icon="quote-left" className="pe-2" />
Lorem ipsum dolor sit amet eos adipisci, consectetur adipisicing
elit.
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4" className="mb-5 mb-md-0">
<MDBCard className="testimonial-card">
<div
className="card-up"
style={{ backgroundColor: "#7a81a8" }}
></div>
<div className="avatar mx-auto bg-white">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(2).webp"
className="rounded-circle img-fluid"
/>
</div>
<MDBCardBody>
<h4 className="mb-4">Lisa Cudrow</h4>
<hr />
<p className="dark-grey-text mt-4">
<MDBIcon fas icon="quote-left" className="pe-2" />
Neque cupiditate assumenda in maiores repudi mollitia
architecto.
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4" className="mb-5 mb-md-0">
<MDBCard className="testimonial-card">
<div
className="card-up"
style={{ backgroundColor: "#6d5b98" }}
></div>
<div className="avatar mx-auto bg-white">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(9).webp"
className="rounded-circle img-fluid"
/>
</div>
<MDBCardBody>
<h4 className="mb-4">John Smith</h4>
<hr />
<p className="dark-grey-text mt-4">
<MDBIcon fas icon="quote-left" className="pe-2" />
Delectus impedit saepe officiis ab aliquam repellat rem unde
ducimus.
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
.testimonial-card .card-up {
height: 120px;
overflow: hidden;
border-top-left-radius: 0.25rem;
border-top-right-radius: 0.25rem;
}
.testimonial-card .avatar {
width: 110px;
margin-top: -60px;
overflow: hidden;
border: 3px solid #fff;
border-radius: 50%;
}
Profile
Avatars are often used in user profile cards. The example below was created with a basic Bootstrap Grid.
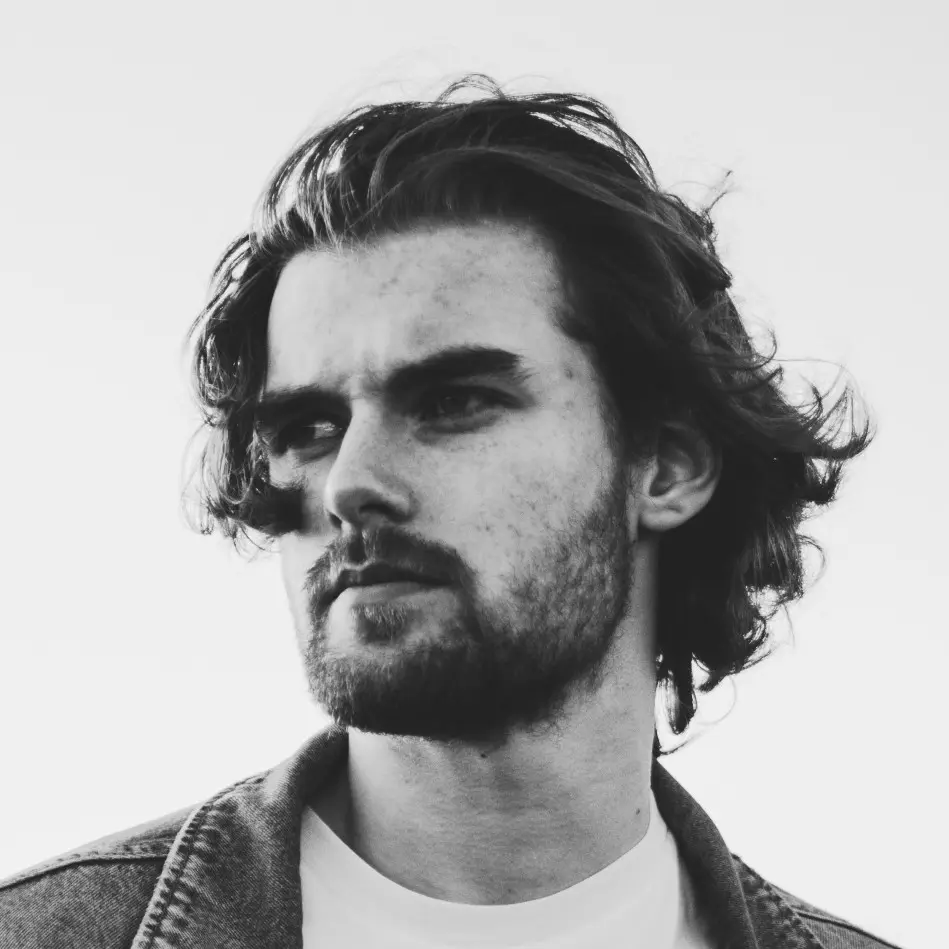
Danny McLoan
Senior Journalist
Articles
41
Followers
976
Rating
8.5
import React from "react";
import {
MDBCol,
MDBContainer,
MDBRow,
MDBCard,
MDBCardTitle,
MDBCardText,
MDBCardBody,
MDBCardImage,
MDBBtn,
} from "mdb-react-ui-kit";
function App() {
return (
<div className="vh-100" style={{ backgroundColor: "#9de2ff" }}>
<MDBContainer>
<MDBRow className="justify-content-center">
<MDBCol md="9" lg="7" xl="5" className="mt-5">
<MDBCard style={{ borderRadius: "15px" }}>
<MDBCardBody className="p-4">
<div className="d-flex text-black">
<div className="flex-shrink-0">
<MDBCardImage
style={{ width: "180px", borderRadius: "10px" }}
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-profiles/avatar-1.webp"
alt="Generic placeholder image"
fluid
/>
</div>
<div className="flex-grow-1 ms-3">
<MDBCardTitle>Danny McLoan</MDBCardTitle>
<MDBCardText>Senior Journalist</MDBCardText>
<div
className="d-flex justify-content-start rounded-3 p-2 mb-2"
style={{ backgroundColor: "#efefef" }}
>
<div>
<p className="small text-muted mb-1">Articles</p>
<p className="mb-0">41</p>
</div>
<div className="px-3">
<p className="small text-muted mb-1">Followers</p>
<p className="mb-0">976</p>
</div>
<div>
<p className="small text-muted mb-1">Rating</p>
<p className="mb-0">8.5</p>
</div>
</div>
<div className="d-flex pt-1">
<MDBBtn outline className="me-1 flex-grow-1">
Chat
</MDBBtn>
<MDBBtn className="flex-grow-1">Follow</MDBBtn>
</div>
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</div>
);
}
export default App;