Quotes
React Bootstrap 5 Quote component
Responsive React Quotes built with Bootstrap 5. HTML & CSS. Quote boxes with quote symbol, blockquotes, quote carousel slider, various quote styles with HTML & CSS.Quote carousel
A beautiful quotes slider with author image and a quotation icon.
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBRow,
MDBCarousel,
MDBCarouselInner,
MDBCarouselItem,
MDBTypography,
MDBIcon,
} from "mdb-react-ui-kit";
export default function QuoteCarosuel() {
return (
<section className="vh-100 gradient-custom">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol xl="10">
<MDBCard>
<MDBCardBody className="py-5">
<MDBCarousel showControls showIndicators dark>
<MDBCarouselInner>
<MDBCarouselItem className="active" tag="div">
<MDBRow className="justify-content-center">
<MDBCol md="8" lg="9" xl="8">
<div className="d-flex">
<div className="flex-shrink-0">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(1).webp"
className="rounded-circle mb-4 mb-lg-0 shadow-2"
alt="woman avatar"
width="90"
height="90"
/>
</div>
<div className="flex-grow-1 ms-4 ps-3">
<figure>
<MDBTypography blockquote className="mb-4">
<p>
<MDBIcon
fas
icon="quote-left fa-lg text-warning me-2"
/>
<span className="font-italic">
Lorem ipsum dolor sit amet consectetur
adipisicing elit. Pariatur sint nesciunt
ad itaque aperiam expedita officiis
incidunt minus facere, molestias quisquam
impedit inventore.
</span>
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Miranda Smith in{" "}
<cite title="Source Title">The Guardian</cite>
</figcaption>
</figure>
</div>
</div>
</MDBCol>
</MDBRow>
</MDBCarouselItem>
<MDBCarouselItem tag="div">
<MDBRow className="justify-content-center">
<MDBCol md="8" lg="9" xl="8">
<div className="d-flex">
<div className="flex-shrink-0">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(2).webp"
className="rounded-circle mb-4 mb-lg-0 shadow-2"
alt="woman avatar"
width="90"
height="90"
/>
</div>
<div className="flex-grow-1 ms-4 ps-3">
<figure>
<MDBTypography blockquote className="mb-4">
<p>
<MDBIcon
fas
icon="quote-left fa-lg text-warning me-2"
/>
<span className="font-italic">
Sed ut perspiciatis unde omnis iste natus
error sit voluptatem accusantium
doloremque laudantium, totam rem aperiam,
eaque ipsa quae ab illo inventore
veritatis.
</span>
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Annie Hall{" "}
<cite title="Source Title">
New York Times
</cite>
</figcaption>
</figure>
</div>
</div>
</MDBCol>
</MDBRow>
</MDBCarouselItem>
<MDBCarouselItem tag="div">
<MDBRow className="justify-content-center">
<MDBCol md="8" lg="9" xl="8">
<div className="d-flex">
<div className="flex-shrink-0">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(9).webp"
className="rounded-circle mb-4 mb-lg-0 shadow-2"
alt="woman avatar"
width="90"
height="90"
/>
</div>
<div className="flex-grow-1 ms-4 ps-3">
<figure>
<MDBTypography blockquote className="mb-4">
<p>
<MDBIcon
fas
icon="quote-left fa-lg text-warning me-2"
/>
<span className="font-italic">
At vero eos et accusamus et iusto odio
dignissimos qui blanditiis praesentium
voluptatum deleniti atque corrupti quos
dolores et quas molestias excepturi sint
amet dolore.
</span>
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Jason More in{" "}
<cite title="Source Title">
Smash Magazine
</cite>
</figcaption>
</figure>
</div>
</div>
</MDBCol>
</MDBRow>
</MDBCarouselItem>
</MDBCarouselInner>
</MDBCarousel>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
.gradient-custom {
/* fallback for old browsers */
background: #f6d365;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(246, 211, 101, 1), rgba(253, 160, 133, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(246, 211, 101, 1), rgba(253, 160, 133, 1))
}
Blockquotes
A simple block quotes with author name and a colorful border.
Age is an issue of mind over matter. If you don't mind, it doesn't matter.
All you need in this life is ignorance and confidence, and then success is sure.
import { MDBCol, MDBContainer, MDBRow, MDBTypography } from "mdb-react-ui-kit";
import React from "react";
export default function Blockquote() {
return (
<section className="vh-100" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="align-items-center h-100">
<MDBCol lg="6" className="mb-4 mb-lg-4">
<figure
className="bg-white p-3 rounded"
style={{ borderLeft: ".25rem solid #a34e78" }}
>
<MDBTypography blockquote className="pb-2">
<p>
Age is an issue of mind over matter. If you don't mind, it
doesn't matter.
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Mark Twain
</figcaption>
</figure>
</MDBCol>
<MDBCol lg="6" className="mb-4 mb-lg-4">
<figure
className="bg-white p-3 rounded"
style={{ borderLeft: ".25rem solid #f68e9d" }}
>
<MDBTypography blockquote className="pb-2">
<p>
All you need in this life is ignorance and confidence, and
then success is sure.
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Mark Twain
</figcaption>
</figure>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Quote block with image
A simple quote card with centered image and quotation mark icons.
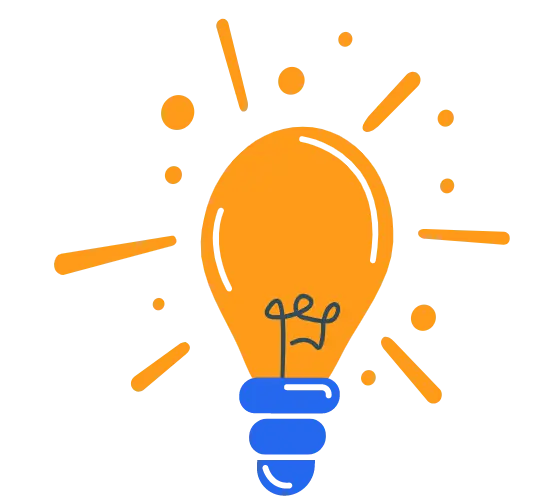
Many of life's failures are people who did not realize how close they were to success when they gave up.
import {
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
import React from "react";
export default function BlockquoteWithImage() {
return (
<section className="vh-100" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="9" xl="7">
<MDBCard style={{ borderRadius: "15px" }}>
<MDBCardBody className="p-5">
<div className="text-center mb-4 pb-2">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-quotes/bulb.webp"
alt="Bulb"
width="100"
/>
</div>
<figure className="mb-0 text-center">
<MDBTypography blockquote>
<p className="pb-3">
<MDBIcon fas icon="quote-left text-primary" size="xs" />
<span className="lead font-italic">
Many of life's failures are people who did not realize
how close they were to success when they gave up.
</span>
<MDBIcon fas icon="quote-right text-primary" size="xs" />
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Thomas Edison
</figcaption>
</figure>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Quote block with icon
A simple quotebox with a stylized quote-left
icon.
"Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo @consequat."
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function BlockquoteWithIcon() {
return (
<section className="vh-100" style={{ backgroundColor: "#f5f7fa" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol md="9" lg="7" xl="5">
<MDBCard>
<MDBCardBody>
<MDBTypography
blockquote
className="blockquote-custom bg-white px-3 pt-4"
>
<div className="blockquote-custom-icon bg-info shadow-1-strong">
<MDBIcon fas icon="quote-left text-white" />
</div>
<p className="mb-0 mt-2 font-italic">
"Lorem ipsum dolor sit amet, consectetur adipiscing elit,
sed do eiusmod tempor incididunt ut labore et dolore magna
aliqua. Ut enim ad minim veniam, quis nostrud exercitation
ullamco laboris nisi ut aliquip ex ea commodo
<a href="#" className="text-info">
@consequat
</a>
."
</p>
<footer className="blockquote-footer pt-4 mt-4 border-top">
Someone famous in
<cite title="Source Title">Source Title</cite>
</footer>
</MDBTypography>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
.blockquote-custom {
position: relative;
font-size: 1.1rem;
}
.blockquote-custom-icon {
width: 50px;
height: 50px;
border-radius: 50%;
display: flex;
align-items: center;
justify-content: center;
position: absolute;
top: -40px;
left: 19px;
}
Square quoteboxes
I have not failed. I've just found 10,000 ways that won't work.
Be less curious about people and more curious about ideas.
import React from "react";
import {
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function Quoteboxes() {
return (
<section className="vh-100 bg-light">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="10" xl="8">
<figure className="note note-primary p-4">
<MDBTypography blockquote>
<p className="pb-2">
"If it's a good idea, go ahead and do it. It's much easier to
apologize than it is to get permission."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Grace Hopper
</figcaption>
</figure>
</MDBCol>
<MDBCol lg="10" xl="8">
<figure className="note note-secondary p-4">
<MDBTypography blockquote>
<p className="pb-2">
"Science and everyday life cannot and should not be
separated."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Rosalind Franklin
</figcaption>
</figure>
</MDBCol>
<MDBCol lg="10" xl="8">
<figure className="note note-success p-4">
<MDBTypography blockquote>
<p className="pb-2">
"Science makes people reach selflessly for truth and
objectivity; it teaches people to accept reality, with wonder
and admiration, not to mention the deep awe and joy that the
natural order of things brings to the true scientist."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Lise Meitner
</figcaption>
</figure>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Quoteboxes
Those quoteboxes are using note
classes, from our
typography.
"If it's a good idea, go ahead and do it. It's much easier to apologize than it is to get permission."
"Science and everyday life cannot and should not be separated."
"Science makes people reach selflessly for truth and objectivity; it teaches people to accept reality, with wonder and admiration, not to mention the deep awe and joy that the natural order of things brings to the true scientist."
import React from "react";
import {
MDBCol,
MDBContainer,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function Quoteboxes() {
return (
<section className="vh-100 bg-light">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="10" xl="8">
<MDBTypography
tag="figure"
note
noteColor="primary"
className="p-4"
>
<MDBTypography blockquote>
<p className="pb-2">
"If it's a good idea, go ahead and do it. It's much easier to
apologize than it is to get permission."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Grace Hopper
</figcaption>
</MDBTypography>
</MDBCol>
<MDBCol lg="10" xl="8">
<MDBTypography
tag="figure"
note
noteColor="secondary"
className="p-4"
>
<MDBTypography blockquote>
<p className="pb-2">
"Science and everyday life cannot and should not be
separated."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Rosalind Franklin
</figcaption>
</MDBTypography>
</MDBCol>
<MDBCol lg="10" xl="8">
<MDBTypography
tag="figure"
note
noteColor="success"
className="p-4"
>
<MDBTypography blockquote>
<p className="pb-2">
"Science makes people reach selflessly for truth and
objectivity; it teaches people to accept reality, with wonder
and admiration, not to mention the deep awe and joy that the
natural order of things brings to the true scientist."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Lise Meitner
</figcaption>
</MDBTypography>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Quotebox with likes counter
A dark quote card with quotation mark icon on top, and a clickable "like quote" icon on bottom.
Genius is one percent inspiration and ninety-nine percent perspiration.
Thomas Edison
876
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function QuoteboxWithLikesCounter() {
return (
<section className="vh-100 bg-light">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol md="12" xl="5">
<MDBCard
className="text-white"
style={{ backgroundColor: "#1f959b", borderRadius: "15px" }}
>
<MDBCardBody className="p-5">
<MDBIcon fas icon="quote-left mb-4" size="2x" />
<p className="lead">
Genius is one percent inspiration and ninety-nine percent
perspiration.
</p>
<hr />
<div className="d-flex justify-content-between">
<p className="mb-0">Thomas Edison</p>
<MDBTypography tag="h6" className="mb-0">
<span
className="badge rounded-pill"
style={{backgroundColor: "rgba(0,0,0, 0.2)"}}
>
876
</span>{" "}
<MDBIcon fas icon="heart ms-2" />
</MDBTypography>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Grey quote
A simple quote card design with default blockquote
class and a subtle yellow border.
Your time is limited, so don't waste it living someone else's life. Don't be trapped by dogma - which is living with the results of other people's thinking. Don't let the noise of others' opinions drown out your own inner voice. And most important, have the courage to follow your heart and intuition.
import React from "react";
import {
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function Grey() {
return (
<section className="vh-100 bg-light">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol md="12" xl="5">
<figure
className="bg-light p-4"
style={{
borderLeft: ".35rem solid #fcdb5e",
borderTop: "1px solid #eee",
borderRight: "1px solid #eee",
borderBottom: "1px solid #eee",
}}
>
<MDBIcon fas icon="quote-left mb-4" size="2x" style={{color: '#fcdb5e'}} />
<MDBTypography blockquote>
<p className="pb-2">
Your time is limited, so don't waste it living someone else's
life. Don't be trapped by dogma - which is living with the
results of other people's thinking. Don't let the noise of
others' opinions drown out your own inner voice. And most
important, have the courage to follow your heart and
intuition.
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Steve Jobs in <cite title="Source Title">Source Title</cite>
</figcaption>
</figure>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Colorful quote alerts
These quote template are using our default background colors to resemble toast alerts.
"If it's a good idea, go ahead and do it. It's much easier to apologize than it is to get permission."
"Science and everyday life cannot and should not be separated."
"Science makes people reach selflessly for truth and objectivity; it teaches people to accept reality, with wonder and admiration, not to mention the deep awe and joy that the natural order of things brings to the true scientist."
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function ColorfulQuote() {
return (
<section className="vh-100 bg-light">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="10" xl="8">
<MDBCard className="bg-primary text-white rounded-3 mb-3">
<MDBCardBody>
<figure className="mb-0">
<MDBTypography blockquote>
<p className="pb-2">
"If it's a good idea, go ahead and do it. It's much easier
to apologize than it is to get permission."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0 text-white">
Grace Hopper
</figcaption>
</figure>
</MDBCardBody>
</MDBCard>
<MDBCard className="bg-success text-white rounded-3 mb-3">
<MDBCardBody>
<figure className="mb-0">
<MDBTypography blockquote>
<p className="pb-2">
"Science and everyday life cannot and should not be
separated."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0 text-white">
Rosalind Franklin
</figcaption>
</figure>
</MDBCardBody>
</MDBCard>
<MDBCard className="bg-danger text-white rounded-3 mb-3">
<MDBCardBody>
<figure className="mb-0">
<MDBTypography blockquote>
<p className="pb-2">
"Science makes people reach selflessly for truth and
objectivity; it teaches people to accept reality, with
wonder and admiration, not to mention the deep awe and joy
that the natural order of things brings to the true
scientist."
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0 text-white">
Lise Meitner
</figcaption>
</figure>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Quote with avatar
Another subtle quote design with an avatar image and the name of the author.
.webp)
Lorem ipsum dolor sit amet consectetur adipisicing elit. Pariatur sint nesciunt ad itaque aperiam expedita officiis incidunt minus facere molestias inventore.
import {
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
import React from "react";
export default function BlockquoteWithAvatar() {
return (
<section className="vh-100" style={{ backgroundColor: "#CDC4F9" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="10" xl="8">
<MDBCard className="rounded-3">
<MDBCardBody className="p-5">
<div className="d-flex justify-content-center mb-4">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20(28).webp"
className="rounded-circle shadow-1-strong"
width="100"
height="100"
/>
</div>
<figure className="mb-0 text-center">
<MDBTypography blockquote className="mb-4">
<p>
<span className="font-italic">
Lorem ipsum dolor sit amet consectetur adipisicing elit.
Pariatur sint nesciunt ad itaque aperiam expedita
officiis incidunt minus facere molestias inventore.
</span>
</p>
</MDBTypography>
<figcaption className="blockquote-footer mb-0">
Kate Williams in <cite title="Source Title">Vogue</cite>
</figcaption>
</figure>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}