Chat
React Chat
Responsive React Chat built with Bootstrap 5. Many variants of chatbox UI, mobile app, messages box, chat window, chatbot UI, group chat, chat widget, web chat & moreBasic example
Simple chatbox template for Bootstrap, you can use it as a popup chat window on your website or even create your own chatbot based on this UI.
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardHeader,
MDBCardBody,
MDBIcon,
MDBTextArea,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer className="py-5">
<MDBRow className="d-flex justify-content-center">
<MDBCol md="8" lg="6" xl="4">
<MDBCard id="chat1" style={{ borderRadius: "15px" }}>
<MDBCardHeader
className="d-flex justify-content-between align-items-center p-3 bg-info text-white border-bottom-0"
style={{
borderTopLeftRadius: "15px",
borderTopRightRadius: "15px",
}}
>
<MDBIcon fas icon="angle-left" />
<p className="mb-0 fw-bold">Live chat</p>
<MDBIcon fas icon="times" />
</MDBCardHeader>
<MDBCardBody>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div
className="p-3 ms-3"
style={{
borderRadius: "15px",
backgroundColor: "rgba(57, 192, 237,.2)",
}}
>
<p className="small mb-0">
Hello and thank you for visiting MDBootstrap. Please click
the video below.
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end mb-4">
<div
className="p-3 me-3 border"
style={{ borderRadius: "15px", backgroundColor: "#fbfbfb" }}
>
<p className="small mb-0">
Thank you, I really like your product.
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div className="ms-3" style={{ borderRadius: "15px" }}>
<div className="bg-image">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/screenshot1.webp"
style={{ borderRadius: "15px" }}
alt="video"
/>
<a href="#!">
<div className="mask"></div>
</a>
</div>
</div>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div
className="p-3 ms-3"
style={{
borderRadius: "15px",
backgroundColor: "rgba(57, 192, 237,.2)",
}}
>
<p className="small mb-0">...</p>
</div>
</div>
<MDBTextArea
className="form-outline"
label="Type your message"
id="textAreaExample"
rows={4}
/>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
#chat1 .form-outline ~ .form-notch div {
pointer-events: none;
border: 1px solid;
border-color: #eee;
box-sizing: border-box;
background: transparent;
}
#chat1 .form-outline ~ .form-notch .form-notch-leading {
left: 0;
top: 0;
height: 100%;
border-right: none;
border-radius: 0.65rem 0 0 0.65rem;
}
#chat1 .form-outline ~ .form-notch .form-notch-middle {
flex: 0 0 auto;
max-width: calc(100% - 1rem);
height: 100%;
border-right: none;
border-left: none;
}
#chat1 .form-outline ~ .form-notch .form-notch-trailing {
flex-grow: 1;
height: 100%;
border-left: none;
border-radius: 0 0.65rem 0.65rem 0;
}
#chat1 .form-outline:focus ~ .form-notch .form-notch-leading {
border-top: 0.125rem solid #39c0ed;
border-bottom: 0.125rem solid #39c0ed;
border-left: 0.125rem solid #39c0ed;
}
#chat1 .form-outline:focus ~ .form-notch .form-notch-leading,
#chat1 .form-outline.active ~ .form-notch .form-notch-leading {
border-right: none;
transition: all 0.2s linear;
}
#chat1 .form-outline:focus ~ .form-notch .form-notch-middle {
border-bottom: 0.125rem solid;
border-color: #39c0ed;
}
#chat1 .form-outline:focus ~ .form-notch .form-notch-middle,
#chat1 .form-outline.active ~ .form-notch .form-notch-middle {
border-top: none;
border-right: none;
border-left: none;
transition: all 0.2s linear;
}
#chat1 .form-outline .form-control:focus ~ .form-notch .form-notch-trailing {
border-top: 0.125rem solid #39c0ed;
border-bottom: 0.125rem solid #39c0ed;
border-right: 0.125rem solid #39c0ed;
}
#chat1 .form-outline .form-control:focus ~ .form-notch .form-notch-trailing,
#chat1 .form-outline .form-control.active ~ .form-notch .form-notch-trailing {
border-left: none;
transition: all 0.2s linear;
}
#chat1 .form-outline .form-control:focus ~ .form-label {
color: #39c0ed;
}
#chat1 .form-outline .form-control ~ .form-label {
color: #bfbfbf;
}
Simple Chat Application
This chat application theme also includes an emoji and file input buttons. Check out our plugin to implement an advanced file upload functionality or use a basic file input form to make it work on production.
Chat
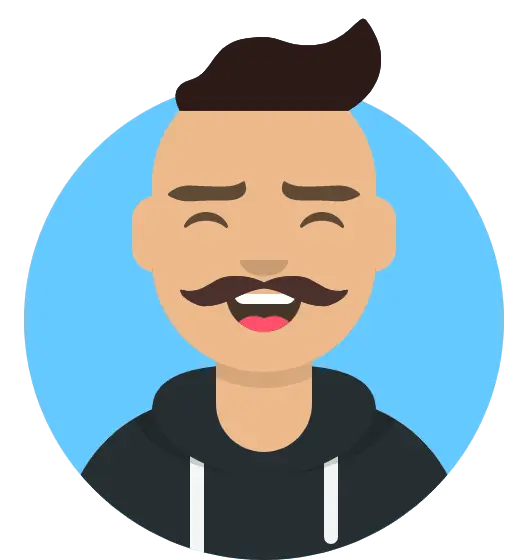
Hi
How are you ...???
What are you doing tomorrow? Can we come up a bar?
23:58
Today
Hiii, I'm good.
How are you doing?
Long time no see! Tomorrow office. will be free on sunday.
00:06
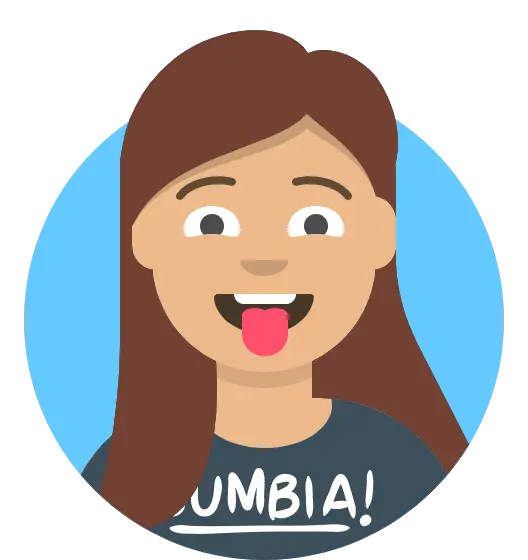
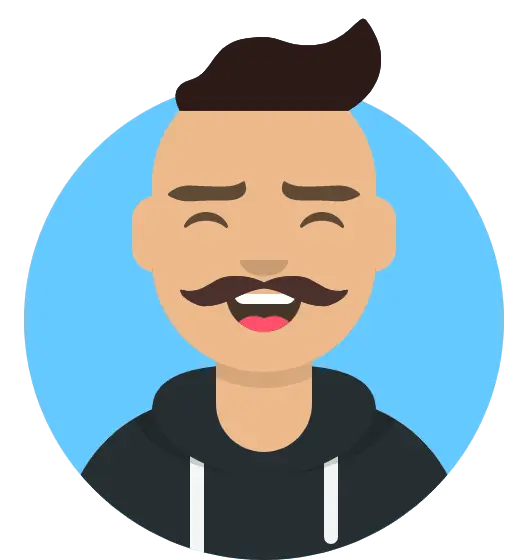
Okay
We will go on Sunday?
00:07
That's awesome!
I will meet you Sandon Square sharp at 10 AM
Is that okay?
00:09
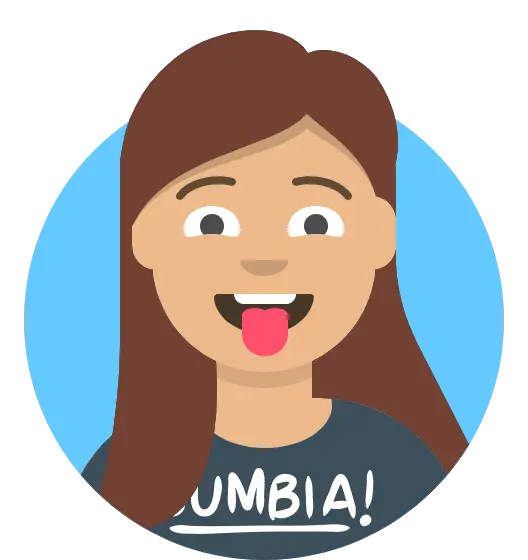
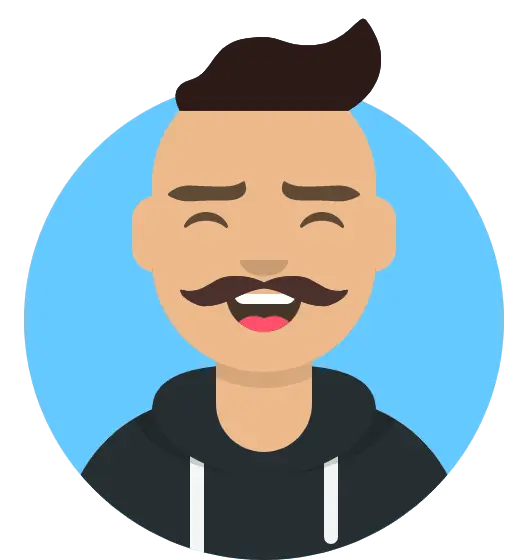
Okay i will meet you on Sandon Square
00:11
Do you have pictures of Matley Marriage?
00:11
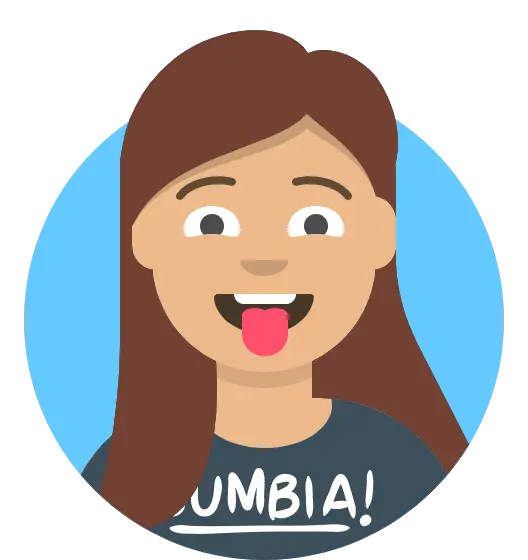
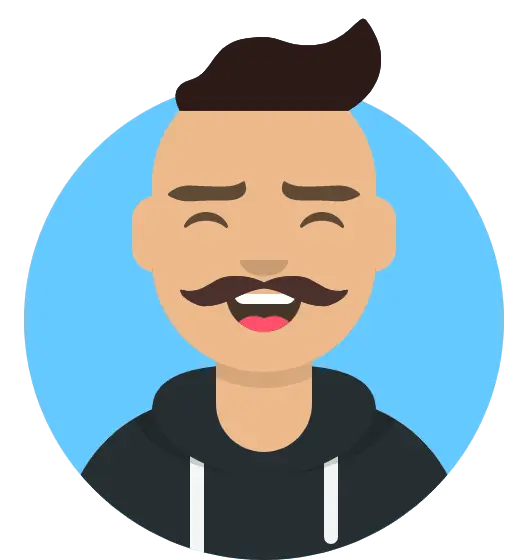
Sorry I don't have. i changed my phone.
00:13
Okay then see you on sunday!!
00:15
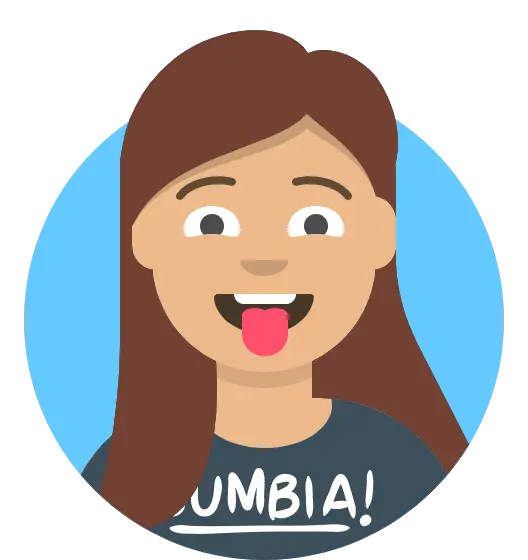
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardHeader,
MDBCardBody,
MDBCardFooter,
MDBIcon,
MDBBtn,
MDBScrollbar,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer fluid className="py-5" style={{ backgroundColor: "#eee" }}>
<MDBRow className="d-flex justify-content-center">
<MDBCol md="10" lg="8" xl="6">
<MDBCard id="chat2" style={{ borderRadius: "15px" }}>
<MDBCardHeader className="d-flex justify-content-between align-items-center p-3">
<h5 className="mb-0">Chat</h5>
<MDBBtn color="primary" size="sm" rippleColor="dark">
Let's Chat App
</MDBBtn>
</MDBCardHeader>
<MDBScrollbar
suppressScrollX
style={{ position: "relative", height: "400px" }}
>
<MDBCardBody>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Hi
</p>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
How are you ...???
</p>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
What are you doing tomorrow? Can we come up a bar?
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
23:58
</p>
</div>
</div>
<div className="divider d-flex align-items-center mb-4">
<p
className="text-center mx-3 mb-0"
style={{ color: "#a2aab7" }}
>
Today
</p>
</div>
<div className="d-flex flex-row justify-content-end mb-4 pt-1">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Hiii, I'm good.
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
How are you doing?
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Long time no see! Tomorrow office. will be free on sunday.
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:06
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava4-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Okay
</p>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
We will go on Sunday?
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
00:07
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end mb-4">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
That's awesome!
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
I will meet you Sandon Square sharp at 10 AM
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Is that okay?
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:09
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava4-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Okay i will meet you on Sandon Square
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
00:11
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end mb-4">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Do you have pictures of Matley Marriage?
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:11
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava4-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Sorry I don't have. i changed my phone.
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
00:13
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Okay then see you on sunday!!
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:15
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava4-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
</MDBCardBody>
</MDBScrollbar>
<MDBCardFooter className="text-muted d-flex justify-content-start align-items-center p-3">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3-bg.webp"
alt="avatar 3"
style={{ width: "45px", height: "100%" }}
/>
<input
type="text"
class="form-control form-control-lg"
id="exampleFormControlInput1"
placeholder="Type message"
></input>
<a className="ms-1 text-muted" href="#!">
<MDBIcon fas icon="paperclip" />
</a>
<a className="ms-3 text-muted" href="#!">
<MDBIcon fas icon="smile" />
</a>
<a className="ms-3" href="#!">
<MDBIcon fas icon="paper-plane" />
</a>
</MDBCardFooter>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
#chat2 .form-control {
border-color: transparent;
}
#chat2 .form-control:focus {
border-color: transparent;
box-shadow: inset 0px 0px 0px 1px transparent;
}
.divider:after,
.divider:before {
content: "";
flex: 1;
height: 1px;
background: #eee;
}
Chat window
A bigger chat window would be a perfect fit for chat rooms and group chat apps.
Member
-
John Doe
Hello, Are you there?
Just now
1 -
Danny Smith
Lorem ipsum dolor sit.
5 mins ago
-
Alex Steward
Lorem ipsum dolor sit.
Yesterday
-
Ashley Olsen
Lorem ipsum dolor sit.
Yesterday
-
Kate Moss
Lorem ipsum dolor sit.
Yesterday
-
Lara Croft
Lorem ipsum dolor sit.
Yesterday
-
Brad Pitt
Lorem ipsum dolor sit.
5 mins ago
-
Brad Pitt
12 mins ago
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
-
Lara Croft
13 mins ago
Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium.
-
Brad Pitt
10 mins ago
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
-
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBIcon,
MDBBtn,
MDBTypography,
MDBTextArea,
MDBCardHeader,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer fluid className="py-5" style={{ backgroundColor: "#eee" }}>
<MDBRow>
<MDBCol md="6" lg="5" xl="4" className="mb-4 mb-md-0">
<h5 className="font-weight-bold mb-3 text-center text-lg-start">
Member
</h5>
<MDBCard>
<MDBCardBody>
<MDBTypography listUnStyled className="mb-0">
<li
className="p-2 border-bottom"
style={{ backgroundColor: "#eee" }}
>
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-8.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">John Doe</p>
<p className="small text-muted">
Hello, Are you there?
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Just now</p>
<span className="badge bg-danger float-end">1</span>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-1.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Danny Smith</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">5 mins ago</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-2.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Alex Steward</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-3.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Ashley Olsen</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-4.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Kate Moss</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-5.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Lara Croft</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2">
<a href="#!" className="d-flex justify-content-between">
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Brad Pitt</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">5 mins ago</p>
<span className="text-muted float-end">
<MDBIcon fas icon="check" />
</span>
</div>
</a>
</li>
</MDBTypography>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6" lg="7" xl="8">
<MDBTypography listUnStyled>
<li className="d-flex justify-content-between mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="avatar"
className="rounded-circle d-flex align-self-start me-3 shadow-1-strong"
width="60"
/>
<MDBCard>
<MDBCardHeader className="d-flex justify-content-between p-3">
<p className="fw-bold mb-0">Brad Pitt</p>
<p className="text-muted small mb-0">
<MDBIcon far icon="clock" /> 12 mins ago
</p>
</MDBCardHeader>
<MDBCardBody>
<p className="mb-0">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed
do eiusmod tempor incididunt ut labore et dolore magna
aliqua.
</p>
</MDBCardBody>
</MDBCard>
</li>
<li class="d-flex justify-content-between mb-4">
<MDBCard className="w-100">
<MDBCardHeader className="d-flex justify-content-between p-3">
<p class="fw-bold mb-0">Lara Croft</p>
<p class="text-muted small mb-0">
<MDBIcon far icon="clock" /> 13 mins ago
</p>
</MDBCardHeader>
<MDBCardBody>
<p className="mb-0">
Sed ut perspiciatis unde omnis iste natus error sit
voluptatem accusantium doloremque laudantium.
</p>
</MDBCardBody>
</MDBCard>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-5.webp"
alt="avatar"
className="rounded-circle d-flex align-self-start ms-3 shadow-1-strong"
width="60"
/>
</li>
<li className="d-flex justify-content-between mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="avatar"
className="rounded-circle d-flex align-self-start me-3 shadow-1-strong"
width="60"
/>
<MDBCard>
<MDBCardHeader className="d-flex justify-content-between p-3">
<p className="fw-bold mb-0">Brad Pitt</p>
<p className="text-muted small mb-0">
<MDBIcon far icon="clock" /> 10 mins ago
</p>
</MDBCardHeader>
<MDBCardBody>
<p className="mb-0">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed
do eiusmod tempor incididunt ut labore et dolore magna
aliqua.
</p>
</MDBCardBody>
</MDBCard>
</li>
<li className="bg-white mb-3">
<MDBTextArea label="Message" id="textAreaExample" rows={4} />
</li>
<MDBBtn color="info" rounded className="float-end">
Send
</MDBBtn>
</MDBTypography>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
Awesome Chat Messages Box
You can also experiment with details of your chat design. For example you could use a more detailed send time & date format or add unread messages notifications with Badges.
Chat messages
Timona Siera
23 Jan 2:00 pm
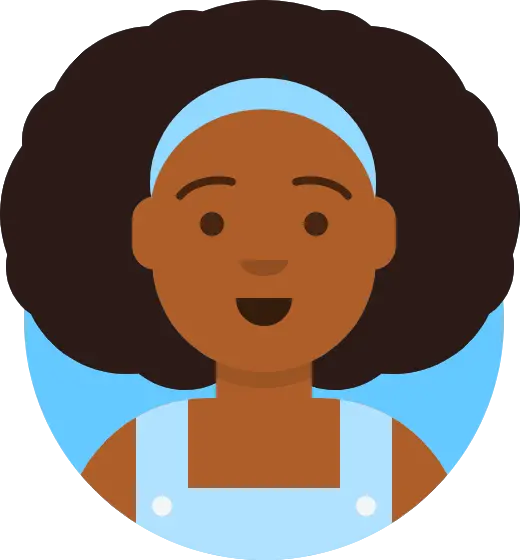
For what reason would it be advisable for me to think about business content?
23 Jan 2:05 pm
Johny Bullock
Thank you for your believe in our supports
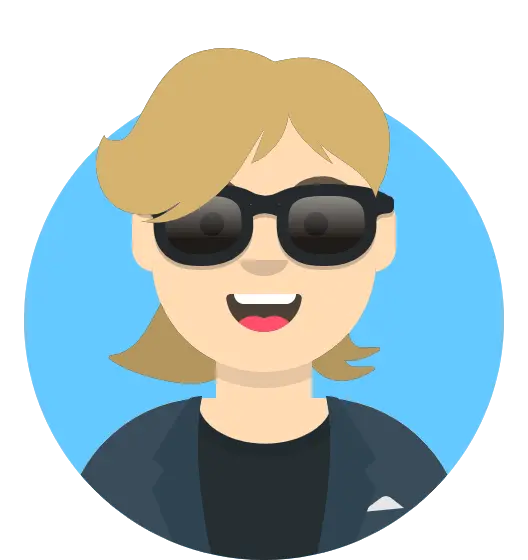
Timona Siera
23 Jan 5:37 pm
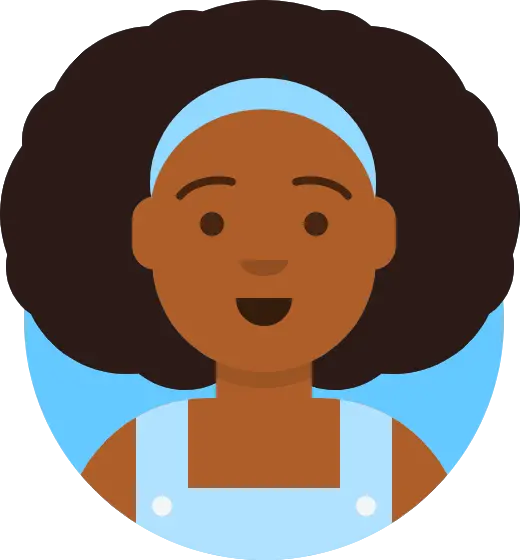
Lorem ipsum dolor sit amet consectetur adipisicing elit similique quae consequatur
23 Jan 6:10 pm
Johny Bullock
Dolorum quasi voluptates quas amet in repellendus perspiciatis fugiat
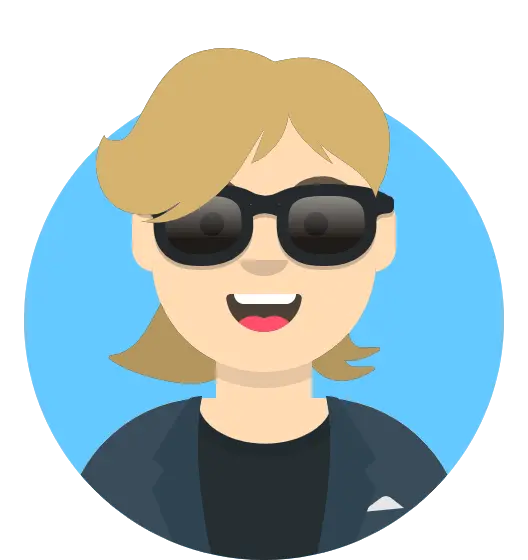
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardHeader,
MDBCardBody,
MDBIcon,
MDBBtn,
MDBScrollbar,
MDBCardFooter,
MDBInputGroup,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer fluid className="py-5" style={{ backgroundColor: "#eee" }}>
<MDBRow className="d-flex justify-content-center">
<MDBCol md="8" lg="6" xl="4">
<MDBCard>
<MDBCardHeader
className="d-flex justify-content-between align-items-center p-3"
style={{ borderTop: "4px solid #ffa900" }}
>
<h5 className="mb-0">Chat messages</h5>
<div className="d-flex flex-row align-items-center">
<span className="badge bg-warning me-3">20</span>
<MDBIcon
fas
icon="minus"
size="xs"
className="me-3 text-muted"
/>
<MDBIcon
fas
icon="comments"
size="xs"
className="me-3 text-muted"
/>
<MDBIcon
fas
icon="times"
size="xs"
className="me-3 text-muted"
/>
</div>
</MDBCardHeader>
<MDBScrollbar
suppressScrollX
style={{ position: "relative", height: "400px" }}
>
<MDBCardBody>
<div className="d-flex justify-content-between">
<p className="small mb-1">Timona Siera</p>
<p className="small mb-1 text-muted">23 Jan 2:00 pm</p>
</div>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-3 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
For what reason would it be advisable for me to think
about business content?
</p>
</div>
</div>
<div className="d-flex justify-content-between">
<p className="small mb-1 text-muted">23 Jan 2:05 pm</p>
<p className="small mb-1">Johny Bullock</p>
</div>
<div className="d-flex flex-row justify-content-end mb-4 pt-1">
<div>
<p className="small p-2 me-3 mb-3 text-white rounded-3 bg-warning">
Thank you for your believe in our supports
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex justify-content-between">
<p className="small mb-1">Timona Siera</p>
<p className="small mb-1 text-muted">23 Jan 5:37 pm</p>
</div>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-3 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Lorem ipsum dolor sit amet consectetur adipisicing elit
similique quae consequatur
</p>
</div>
</div>
<div className="d-flex justify-content-between">
<p className="small mb-1 text-muted">23 Jan 6:10 pm</p>
<p className="small mb-1">Johny Bullock</p>
</div>
<div className="d-flex flex-row justify-content-end mb-4 pt-1">
<div>
<p className="small p-2 me-3 mb-3 text-white rounded-3 bg-warning">
Dolorum quasi voluptates quas amet in repellendus
perspiciatis fugiat
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
</MDBCardBody>
</MDBScrollbar>
<MDBCardFooter className="text-muted d-flex justify-content-start align-items-center p-3">
<MDBInputGroup className="mb-0">
<input
className="form-control"
placeholder="Type message"
type="text"
/>
<MDBBtn color="warning" style={{ paddingTop: ".55rem" }}>
Button
</MDBBtn>
</MDBInputGroup>
</MDBCardFooter>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
Chat window with gradient background
Customize your chat with different backgrounds and styles. In the example below we've used our Gradient Generator for creating a colorful background and Mask Generator to make the chat bubbles resemble glass panels with glassmorphism effect.
Member
-
John Doe
Hello, Are you there?
Just now
1 -
Danny Smith
Lorem ipsum dolor sit.
5 mins ago
-
Alex Steward
Lorem ipsum dolor sit.
Yesterday
-
Ashley Olsen
Lorem ipsum dolor sit.
Yesterday
-
Kate Moss
Lorem ipsum dolor sit.
Yesterday
-
Lara Croft
Lorem ipsum dolor sit.
Yesterday
-
Brad Pitt
Lorem ipsum dolor sit.
5 mins ago
-
Brad Pitt
12 mins ago
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
-
Lara Croft
13 mins ago
Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium.
-
Brad Pitt
10 mins ago
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
-
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBIcon,
MDBBtn,
MDBTypography,
MDBTextArea,
MDBCardHeader,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer fluid className="py-5 gradient-custom">
<MDBRow>
<MDBCol md="6" lg="5" xl="4" className="mb-4 mb-md-0">
<h5 className="font-weight-bold mb-3 text-center text-white">
Member
</h5>
<MDBCard className="mask-custom">
<MDBCardBody>
<MDBTypography listUnStyled className="mb-0">
<li
className="p-2 border-bottom"
style={{
borderBottom: "1px solid rgba(255,255,255,.3) !important",
}}
>
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-8.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">John Doe</p>
<p className="small text-white">
Hello, Are you there?
</p>
</div>
</div>
<div className="pt-1">
<p className="small mb-1 text-white">Just now</p>
<span className="badge bg-danger float-end">1</span>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-1.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Danny Smith</p>
<p className="small text-white">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-whites mb-1">5 mins ago</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-2.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Alex Steward</p>
<p className="small text-white">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-white mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-3.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Ashley Olsen</p>
<p className="small text-white">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-white mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-4.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Kate Moss</p>
<p className="small text-white">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-white mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-5.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Lara Croft</p>
<p className="small text-white">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-white mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2">
<a
href="#!"
className="d-flex justify-content-between link-light"
>
<div className="d-flex flex-row">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="avatar"
className="rounded-circle d-flex align-self-center me-3 shadow-1-strong"
width="60"
/>
<div className="pt-1">
<p className="fw-bold mb-0">Brad Pitt</p>
<p className="small text-white">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-white mb-1">5 mins ago</p>
<span className="text-white float-end">
<MDBIcon fas icon="check" />
</span>
</div>
</a>
</li>
</MDBTypography>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="6" lg="7" xl="8">
<MDBTypography listUnStyled className="text-white">
<li className="d-flex justify-content-between mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="avatar"
className="rounded-circle d-flex align-self-start me-3 shadow-1-strong"
width="60"
/>
<MDBCard className="mask-custom">
<MDBCardHeader
className="d-flex justify-content-between p-3"
style={{ borderBottom: "1px solid rgba(255,255,255,.3)" }}
>
<p className="fw-bold mb-0">Brad Pitt</p>
<p className="text-light small mb-0">
<MDBIcon far icon="clock" /> 12 mins ago
</p>
</MDBCardHeader>
<MDBCardBody>
<p className="mb-0">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed
do eiusmod tempor incididunt ut labore et dolore magna
aliqua.
</p>
</MDBCardBody>
</MDBCard>
</li>
<li class="d-flex justify-content-between mb-4">
<MDBCard className="w-100 mask-custom">
<MDBCardHeader
className="d-flex justify-content-between p-3"
style={{ borderBottom: "1px solid rgba(255,255,255,.3)" }}
>
<p class="fw-bold mb-0">Lara Croft</p>
<p class="text-light small mb-0">
<MDBIcon far icon="clock" /> 13 mins ago
</p>
</MDBCardHeader>
<MDBCardBody>
<p className="mb-0">
Sed ut perspiciatis unde omnis iste natus error sit
voluptatem accusantium doloremque laudantium.
</p>
</MDBCardBody>
</MDBCard>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-5.webp"
alt="avatar"
className="rounded-circle d-flex align-self-start ms-3 shadow-1-strong"
width="60"
/>
</li>
<li className="d-flex justify-content-between mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
alt="avatar"
className="rounded-circle d-flex align-self-start me-3 shadow-1-strong"
width="60"
/>
<MDBCard className="mask-custom">
<MDBCardHeader
className="d-flex justify-content-between p-3"
style={{ borderBottom: "1px solid rgba(255,255,255,.3)" }}
>
<p className="fw-bold mb-0">Brad Pitt</p>
<p className="text-light small mb-0">
<MDBIcon far icon="clock" /> 10 mins ago
</p>
</MDBCardHeader>
<MDBCardBody>
<p className="mb-0">
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed
do eiusmod tempor incididunt ut labore et dolore magna
aliqua.
</p>
</MDBCardBody>
</MDBCard>
</li>
<li className="mb-3">
<MDBTextArea label="Message" id="textAreaExample" rows={4} />
</li>
<MDBBtn color="light" size="lg" rounded className="float-end">
Send
</MDBBtn>
</MDBTypography>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
.gradient-custom {
/* fallback for old browsers */
background: #fccb90;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(
to bottom right,
rgba(252, 203, 144, 1),
rgba(213, 126, 235, 1)
);
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(
to bottom right,
rgba(252, 203, 144, 1),
rgba(213, 126, 235, 1)
);
}
.mask-custom {
background: rgba(24, 24, 16, 0.2);
border-radius: 2em;
backdrop-filter: blur(15px);
border: 2px solid rgba(255, 255, 255, 0.05);
background-clip: padding-box;
box-shadow: 10px 10px 10px rgba(46, 54, 68, 0.03);
}
Chatroom template with scrollbar
If the recipients list in your chatroom app gets too long to display, you should consider adding a Scrollbar to it.
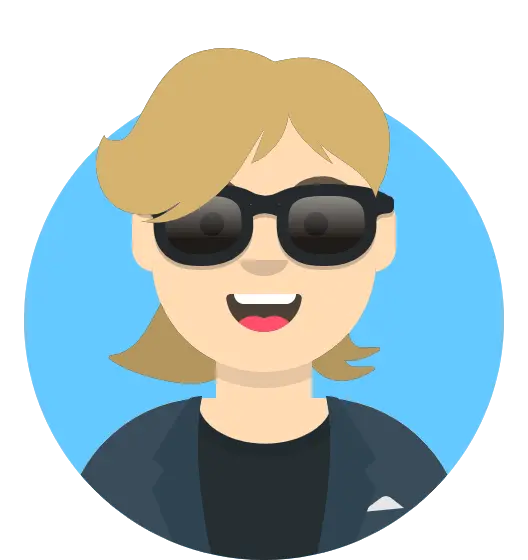
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
12:00 PM | Aug 13
Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat.
12:00 PM | Aug 13
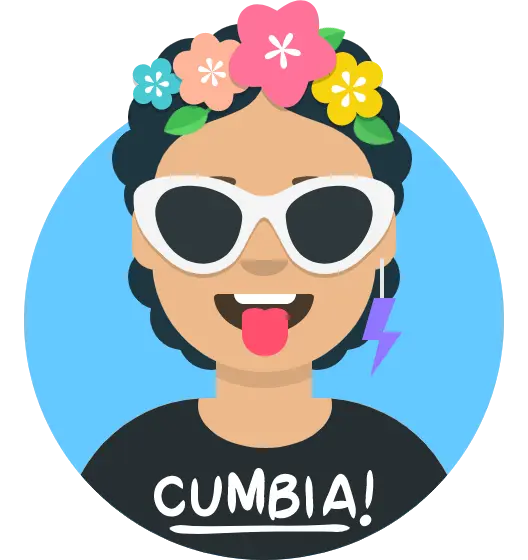
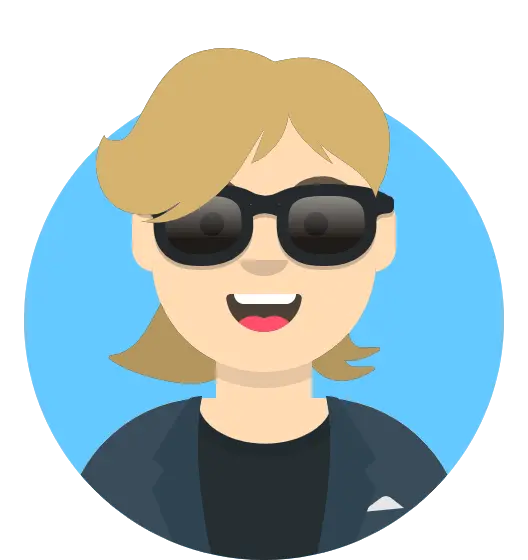
Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur.
12:00 PM | Aug 13
Excepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt mollit anim id est laborum.
12:00 PM | Aug 13
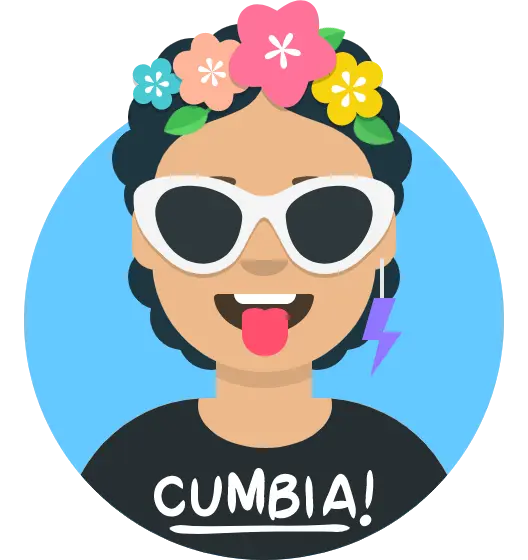
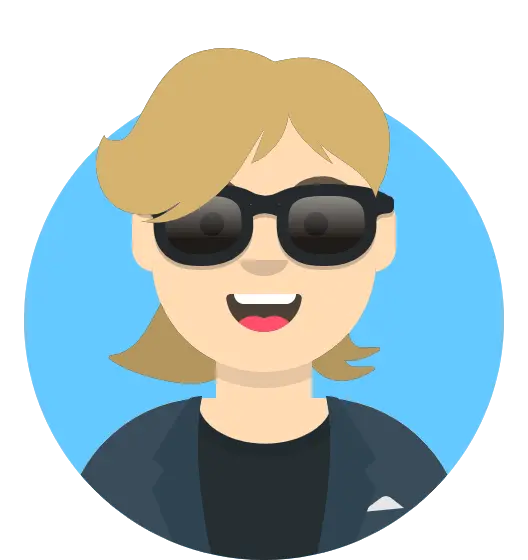
Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo.
12:00 PM | Aug 13
Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt.
12:00 PM | Aug 13
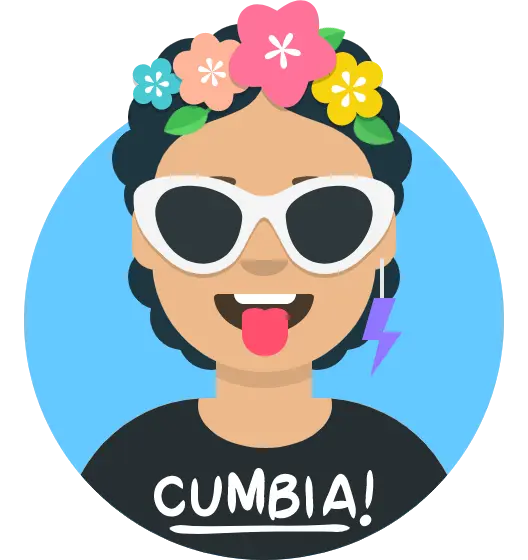
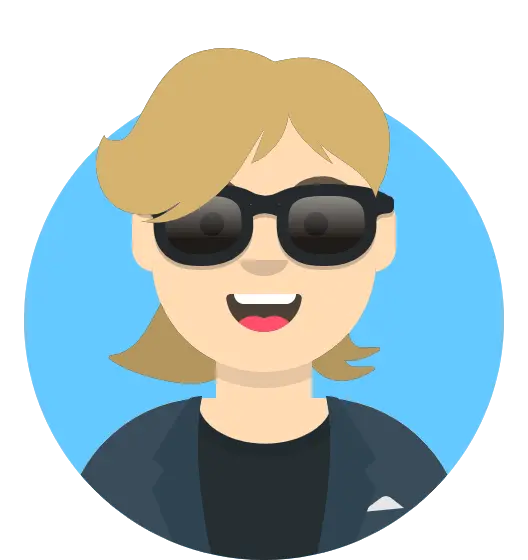
Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem.
12:00 PM | Aug 13
Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur?
12:00 PM | Aug 13
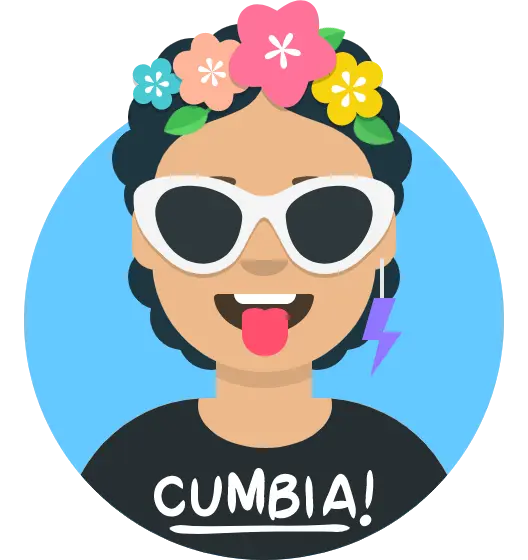
import React from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBIcon,
MDBTypography,
MDBInputGroup,
MDBScrollbar,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer fluid className="py-5" style={{ backgroundColor: "#CDC4F9" }}>
<MDBRow>
<MDBCol md="12">
<MDBCard id="chat3" style={{ borderRadius: "15px" }}>
<MDBCardBody>
<MDBRow>
<MDBCol md="6" lg="5" xl="4" className="mb-4 mb-md-0">
<div className="p-3">
<MDBInputGroup className="rounded mb-3">
<input
className="form-control rounded"
placeholder="Search"
type="search"
/>
<span
className="input-group-text border-0"
id="search-addon"
>
<MDBIcon fas icon="search" />
</span>
</MDBInputGroup>
<MDBScrollbar
suppressScrollX
style={{ position: "relative", height: "400px" }}
>
<MDBTypography listUnStyled className="mb-0">
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between"
>
<div className="d-flex flex-row">
<div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar"
className="d-flex align-self-center me-3"
width="60"
/>
<span className="badge bg-success badge-dot"></span>
</div>
<div className="pt-1">
<p className="fw-bold mb-0">Marie Horwitz</p>
<p className="small text-muted">
Hello, Are you there?
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Just now</p>
<span className="badge bg-danger rounded-pill float-end">
3
</span>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between"
>
<div class="d-flex flex-row">
<div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
alt="avatar"
className="d-flex align-self-center me-3"
width="60"
/>
<span className="badge bg-warning badge-dot"></span>
</div>
<div className="pt-1">
<p className="fw-bold mb-0">Alexa Chung</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">
5 mins ago
</p>
<span className="badge bg-danger rounded-pill float-end">
2
</span>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between"
>
<div className="d-flex flex-row">
<div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava3-bg.webp"
alt="avatar"
className="d-flex align-self-center me-3"
width="60"
/>
<span className="badge bg-success badge-dot"></span>
</div>
<div className="pt-1">
<p className="fw-bold mb-0">Danny McChain</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between"
>
<div className="d-flex flex-row">
<div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava4-bg.webp"
alt="avatar"
className="d-flex align-self-center me-3"
width="60"
/>
<span className="badge bg-danger badge-dot"></span>
</div>
<div className="pt-1">
<p className="fw-bold mb-0">Ashley Olsen</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2 border-bottom">
<a
href="#!"
className="d-flex justify-content-between"
>
<div className="d-flex flex-row">
<div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar"
className="d-flex align-self-center me-3"
width="60"
/>
<span className="badge bg-warning badge-dot"></span>
</div>
<div className="pt-1">
<p className="fw-bold mb-0">Kate Moss</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
<li className="p-2">
<a
href="#!"
className="d-flex justify-content-between"
>
<div class="d-flex flex-row">
<div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar"
className="d-flex align-self-center me-3"
width="60"
/>
<span className="badge bg-success badge-dot"></span>
</div>
<div className="pt-1">
<p className="fw-bold mb-0">Ben Smith</p>
<p className="small text-muted">
Lorem ipsum dolor sit.
</p>
</div>
</div>
<div className="pt-1">
<p className="small text-muted mb-1">Yesterday</p>
</div>
</a>
</li>
</MDBTypography>
</MDBScrollbar>
</div>
</MDBCol>
<MDBCol md="6" lg="7" xl="8">
<MDBScrollbar
suppressScrollX
style={{ position: "relative", height: "400px" }}
className="pt-3 pe-3"
>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Lorem ipsum dolor sit amet, consectetur adipiscing
elit, sed do eiusmod tempor incididunt ut labore et
dolore magna aliqua.
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted float-end">
12:00 PM | Aug 13
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Ut enim ad minim veniam, quis nostrud exercitation
ullamco laboris nisi ut aliquip ex ea commodo
consequat.
</p>
<p className="small me-3 mb-3 rounded-3 text-muted">
12:00 PM | Aug 13
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Duis aute irure dolor in reprehenderit in voluptate
velit esse cillum dolore eu fugiat nulla pariatur.
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted float-end">
12:00 PM | Aug 13
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Excepteur sint occaecat cupidatat non proident, sunt
in culpa qui officia deserunt mollit anim id est
laborum.
</p>
<p className="small me-3 mb-3 rounded-3 text-muted">
12:00 PM | Aug 13
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Sed ut perspiciatis unde omnis iste natus error sit
voluptatem accusantium doloremque laudantium, totam
rem aperiam, eaque ipsa quae ab illo inventore
veritatis et quasi architecto beatae vitae dicta sunt
explicabo.
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted float-end">
12:00 PM | Aug 13
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Nemo enim ipsam voluptatem quia voluptas sit
aspernatur aut odit aut fugit, sed quia consequuntur
magni dolores eos qui ratione voluptatem sequi
nesciunt.
</p>
<p className="small me-3 mb-3 rounded-3 text-muted">
12:00 PM | Aug 13
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Neque porro quisquam est, qui dolorem ipsum quia dolor
sit amet, consectetur, adipisci velit, sed quia non
numquam eius modi tempora incidunt ut labore et dolore
magnam aliquam quaerat voluptatem.
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted float-end">
12:00 PM | Aug 13
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-primary">
Ut enim ad minima veniam, quis nostrum exercitationem
ullam corporis suscipit laboriosam, nisi ut aliquid ex
ea commodi consequatur?
</p>
<p className="small me-3 mb-3 rounded-3 text-muted">
12:00 PM | Aug 13
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava1-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
</MDBScrollbar>
<div className="text-muted d-flex justify-content-start align-items-center pe-3 pt-3 mt-2">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava6-bg.webp"
alt="avatar 3"
style={{ width: "40px", height: "100%" }}
/>
<input
type="text"
className="form-control form-control-lg"
id="exampleFormControlInput2"
placeholder="Type message"
/>
<a className="ms-1 text-muted" href="#!">
<MDBIcon fas icon="paperclip" />
</a>
<a className="ms-3 text-muted" href="#!">
<MDBIcon fas icon="smile" />
</a>
<a className="ms-3" href="#!">
<MDBIcon fas icon="paper-plane" />
</a>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
#chat3 .form-control {
border-color: transparent;
}
#chat3 .form-control:focus {
border-color: transparent;
box-shadow: inset 0px 0px 0px 1px transparent;
}
.badge-dot {
border-radius: 50%;
height: 10px;
width: 10px;
margin-left: 2.9rem;
margin-top: -0.75rem;
}
Collapsible Chat App
You can allow your users to hide the chat box on your website by combining it with the Collapse functionality.
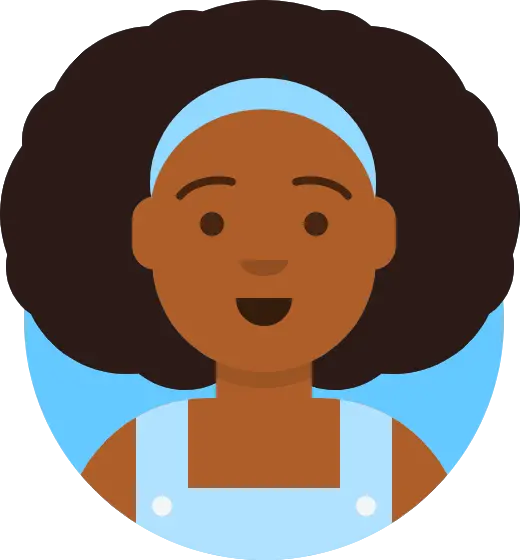
Hi
How are you ...???
What are you doing tomorrow? Can we come up a bar?
23:58
Today
Hiii, I'm good.
How are you doing?
Long time no see! Tomorrow office. will be free on sunday.
00:06
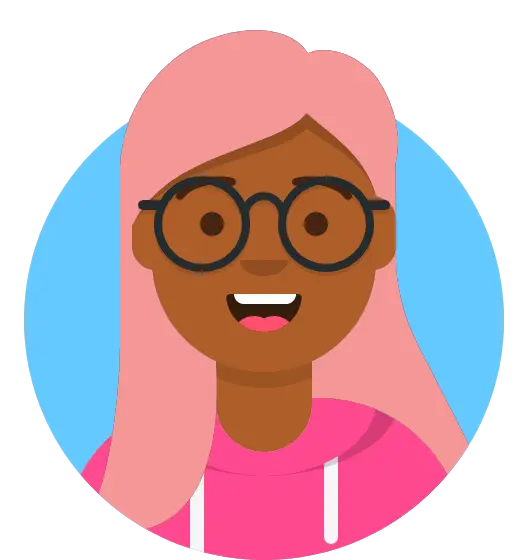
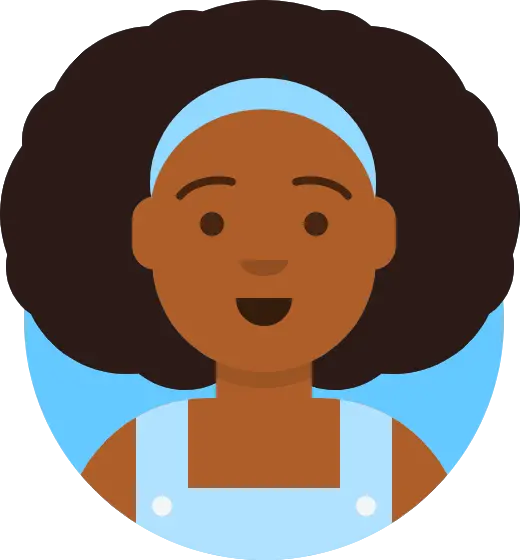
Okay
We will go on Sunday?
00:07
That's awesome!
I will meet you Sandon Square sharp at 10 AM
Is that okay?
00:09
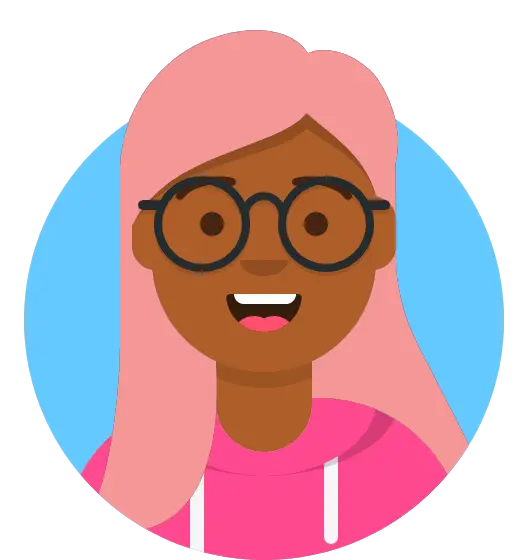
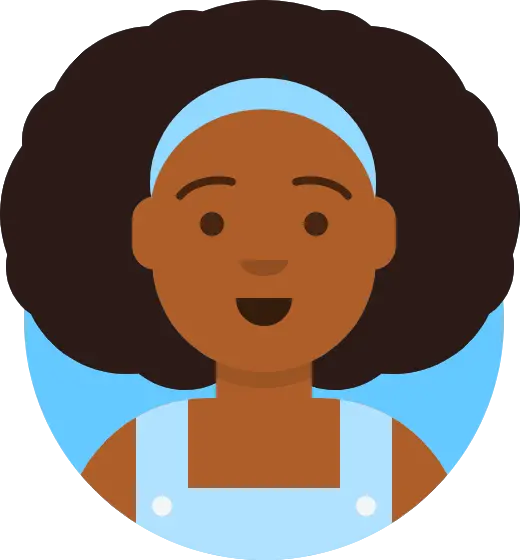
Okay i will meet you on Sandon Square
00:11
Do you have pictures of Matley Marriage?
00:11
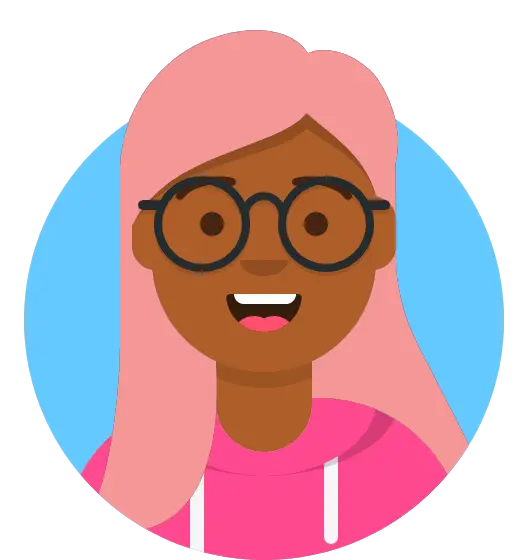
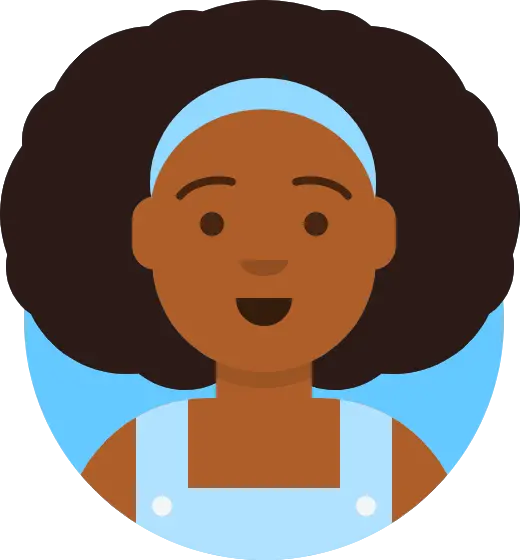
Sorry I don't have. i changed my phone.
00:13
Okay then see you on sunday!!
00:15
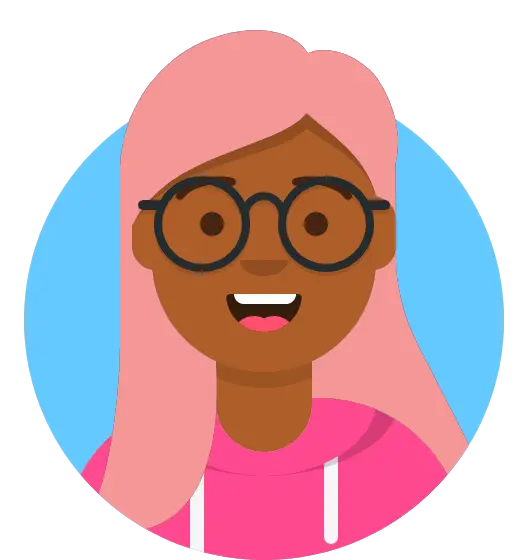
import React, { useState } from "react";
import {
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBIcon,
MDBBtn,
MDBScrollbar,
MDBCardFooter,
MDBCollapse,
} from "mdb-react-ui-kit";
export default function App() {
const [showShow, setShowShow] = useState(false);
const toggleShow = () => setShowShow(!showShow);
return (
<MDBContainer fluid className="py-5">
<MDBRow className="d-flex justify-content-center">
<MDBCol md="8" lg="6" xl="4">
<MDBBtn onClick={toggleShow} color="info" size="lg" block>
<div class="d-flex justify-content-between align-items-center">
<span>Collapsible Chat App</span>
<MDBIcon fas icon="chevron-down" />
</div>
</MDBBtn>
<MDBCollapse show={showShow} className="mt-3">
<MDBCard id="chat4">
<MDBScrollbar
suppressScrollX
style={{ position: "relative", height: "400px" }}
>
<MDBCardBody>
<div className="d-flex flex-row justify-content-start">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Hi
</p>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
How are you ...???
</p>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
What are you doing tomorrow? Can we come up a bar?
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
23:58
</p>
</div>
</div>
<div className="divider d-flex align-items-center mb-4">
<p
className="text-center mx-3 mb-0"
style={{ color: "#a2aab7" }}
>
Today
</p>
</div>
<div className="d-flex flex-row justify-content-end mb-4 pt-1">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
Hiii, I'm good.
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
How are you doing?
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
Long time no see! Tomorrow office. will be free on
sunday.
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:06
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Okay
</p>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
We will go on Sunday?
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
00:07
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end mb-4">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
That's awesome!
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
I will meet you Sandon Square sharp at 10 AM
</p>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
Is that okay?
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:09
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Okay i will meet you on Sandon Square
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
00:11
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end mb-4">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
Do you have pictures of Matley Marriage?
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:11
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
<div className="d-flex flex-row justify-content-start mb-4">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
<div>
<p
className="small p-2 ms-3 mb-1 rounded-3"
style={{ backgroundColor: "#f5f6f7" }}
>
Sorry I don't have. i changed my phone.
</p>
<p className="small ms-3 mb-3 rounded-3 text-muted">
00:13
</p>
</div>
</div>
<div className="d-flex flex-row justify-content-end">
<div>
<p className="small p-2 me-3 mb-1 text-white rounded-3 bg-info">
Okay then see you on sunday!!
</p>
<p className="small me-3 mb-3 rounded-3 text-muted d-flex justify-content-end">
00:15
</p>
</div>
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava2-bg.webp"
alt="avatar 1"
style={{ width: "45px", height: "100%" }}
/>
</div>
</MDBCardBody>
</MDBScrollbar>
<MDBCardFooter className="text-muted d-flex justify-content-start align-items-center p-3">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-chat/ava5-bg.webp"
alt="avatar 3"
style={{ width: "45px", height: "100%" }}
/>
<input
type="text"
className="form-control form-control-lg"
id="exampleFormControlInput3"
placeholder="Type message"
/>
<a className="ms-1 text-muted" href="#!">
<MDBIcon fas icon="paperclip" />
</a>
<a className="ms-3 text-muted" href="#!">
<MDBIcon fas icon="smile" />
</a>
<a className="ms-3 link-info" href="#!">
<MDBIcon fas icon="paper-plane" />
</a>
</MDBCardFooter>
</MDBCard>
</MDBCollapse>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
#chat4 .form-control {
border-color: transparent;
}
#chat4 .form-control:focus {
border-color: transparent;
box-shadow: inset 0px 0px 0px 1px transparent;
}
.divider:after,
.divider:before {
content: "";
flex: 1;
height: 1px;
background: #eee;
}