News feed
React Bootstrap 5 News feed
Responsive React News Feed templates built with Bootstrap 5. News article feed, instagram, facebook and twitter-like feed, posts with comments, social section & more.Basic example
Simple newsfeed article, with an image and a "News of the day" label.
Facilis consequatur eligendi
Lorem ipsum dolor sit amet consectetur adipisicing elit. Facilis consequatur eligendi quisquam doloremque vero ex debitis veritatis placeat unde animi laborum sapiente illo possimus, commodi dignissimos obcaecati illum maiores corporis.
import React from "react";
import {
MDBBtn,
MDBCol,
MDBContainer,
MDBRipple,
MDBRow,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer className="py-5">
<MDBRow className="gx-5">
<MDBCol md="6" className="mb-4">
<MDBRipple
className="bg-image hover-overlay ripple shadow-2-strong rounded-5"
rippleTag="div"
rippleColor="light"
>
<img
src="https://mdbcdn.b-cdn.net/img/new/slides/080.webp"
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6" className="mb-4">
<span className="badge bg-danger px-2 py-1 shadow-1-strong mb-3">
News of the day
</span>
<h4>
<strong>Facilis consequatur eligendi</strong>
</h4>
<p className="text-muted">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Facilis
consequatur eligendi quisquam doloremque vero ex debitis veritatis
placeat unde animi laborum sapiente illo possimus, commodi
dignissimos obcaecati illum maiores corporis.
</p>
<MDBBtn>Read More</MDBBtn>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
Blog posts
Article card ideal for a blog post with category icon, and some related articles list.
This is title of the news
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit, iste aliquid. Sed id nihil magni, sint vero provident esse numquam perferendis ducimus dicta adipisci iusto nam temporibus modi animi laboriosam?
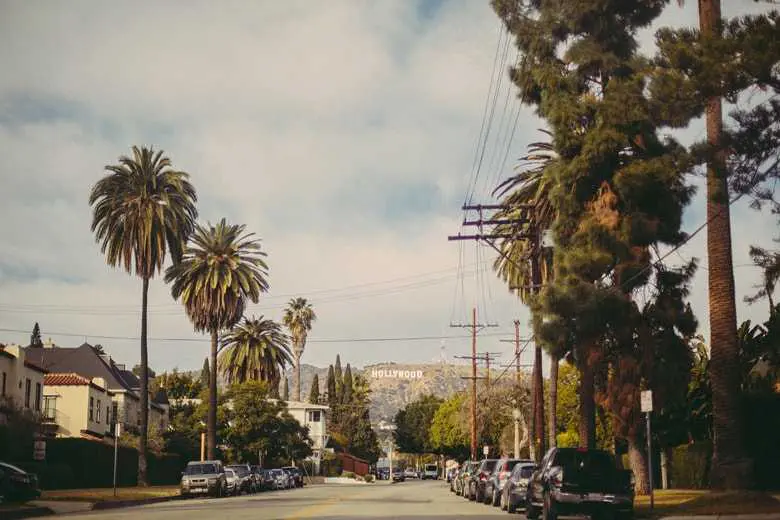
Lorem ipsum dolor sit amet
15.07.2020
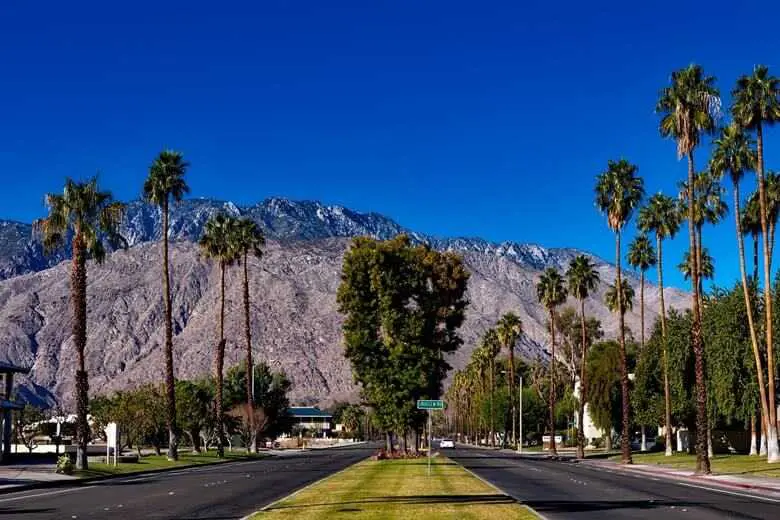
Lorem ipsum dolor sit amet
15.07.2020
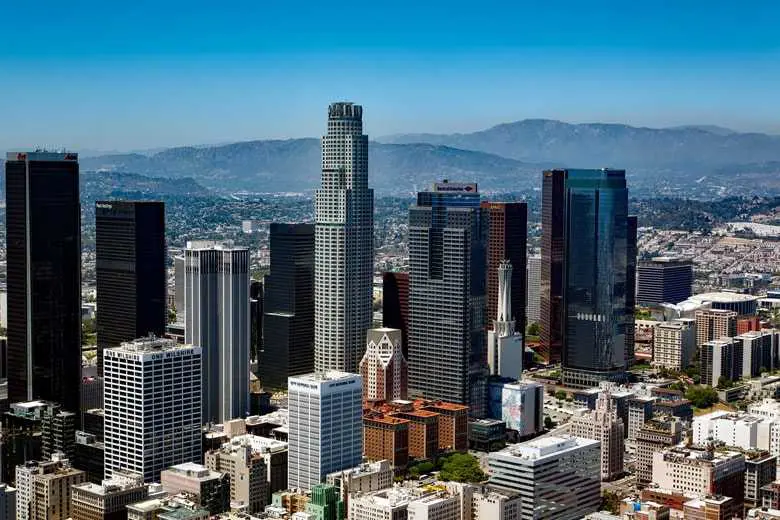
Lorem ipsum dolor sit amet
15.07.2020
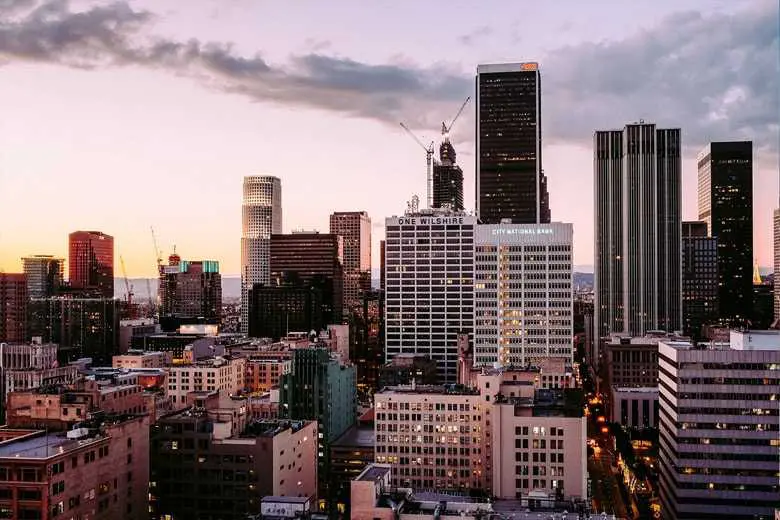
Lorem ipsum dolor sit amet
15.07.2020
import React from "react";
import {
MDBCard,
MDBContainer,
MDBCol,
MDBIcon,
MDBRipple,
MDBRow,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer className="py-5">
<MDBCard className="px-3 pt-3" style={{ maxWidth: "32rem" }}>
<div>
<MDBRipple
className="bg-image hover-overlay shadow-1-strong ripple rounded-5 mb-4"
rippleTag="div"
rippleColor="light"
>
<img
src="https://mdbcdn.b-cdn.net/img/new/fluid/city/113.webp"
className="img-fluid"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
<MDBRow className="mb-3">
<MDBCol col="6">
<a href="" className="text-info">
<MDBIcon fas icon="plane" className="me-1" />
Travels
</a>
</MDBCol>
<MDBCol col="6" className="text-end">
<u> 15.07.2020</u>
</MDBCol>
</MDBRow>
<a href="#!" className="text-dark">
<h5>This is title of the news</h5>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit,
iste aliquid. Sed id nihil magni, sint vero provident esse numquam
perferendis ducimus dicta adipisci iusto nam temporibus modi animi
laboriosam?
</p>
</a>
<hr />
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/041.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/042.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/043.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/044.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
</div>
</MDBCard>
</MDBContainer>
);
}
News aggregator
A magazine template page for with news and articles.
Facilis consequatur eligendi
Lorem ipsum dolor sit amet consectetur adipisicing elit. Facilis consequatur eligendi quisquam doloremque vero ex debitis veritatis placeat unde animi laborum sapiente illo possimus, commodi dignissimos obcaecati illum maiores corporis.
This is title of the news
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit, iste aliquid. Sed id nihil magni, sint vero provident esse numquam perferendis ducimus dicta adipisci iusto nam temporibus modi animi laboriosam?
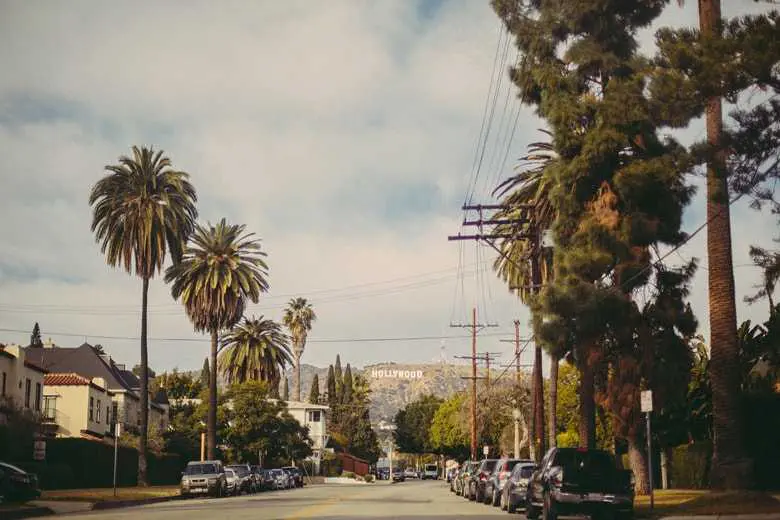
Lorem ipsum dolor sit amet
15.07.2020
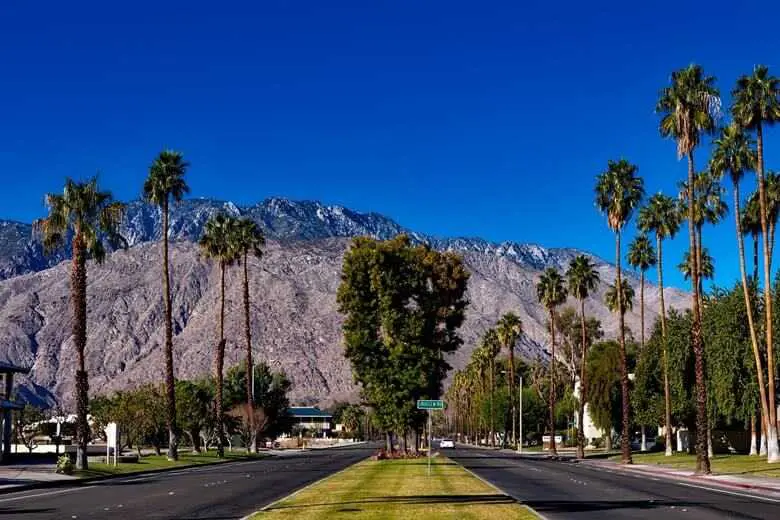
Lorem ipsum dolor sit amet
15.07.2020
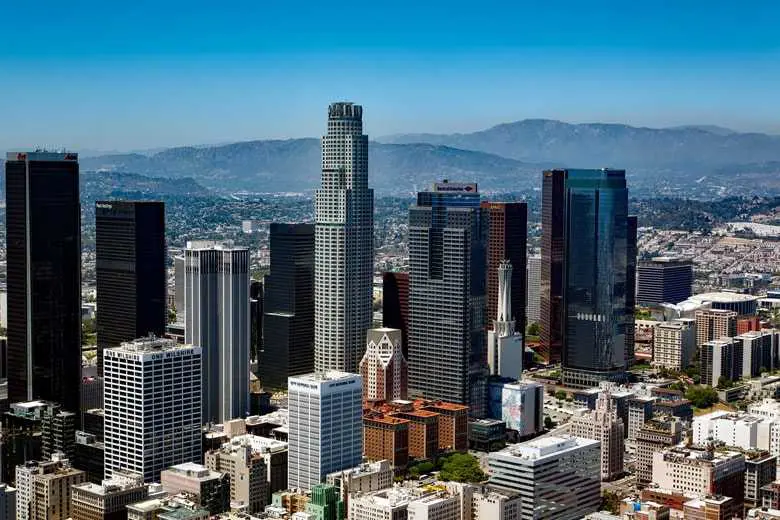
Lorem ipsum dolor sit amet
15.07.2020
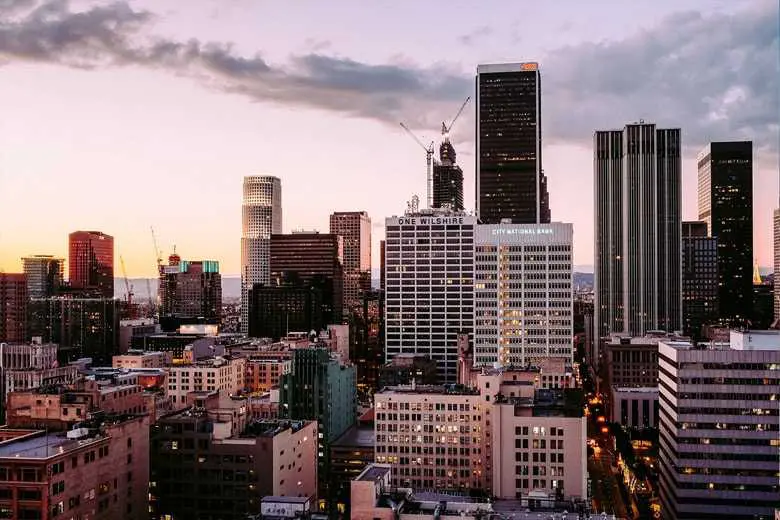
Lorem ipsum dolor sit amet
15.07.2020
This is title of the news
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit, iste aliquid. Sed id nihil magni, sint vero provident esse numquam perferendis ducimus dicta adipisci iusto nam temporibus modi animi laboriosam?
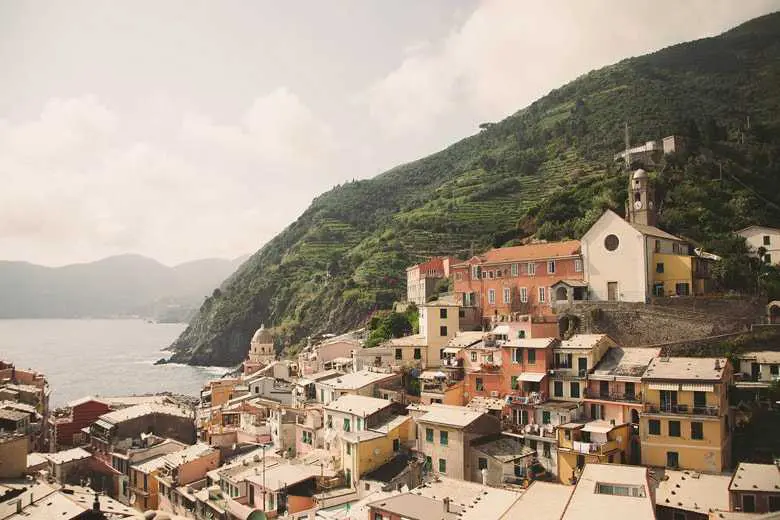
Lorem ipsum dolor sit amet
15.07.2020
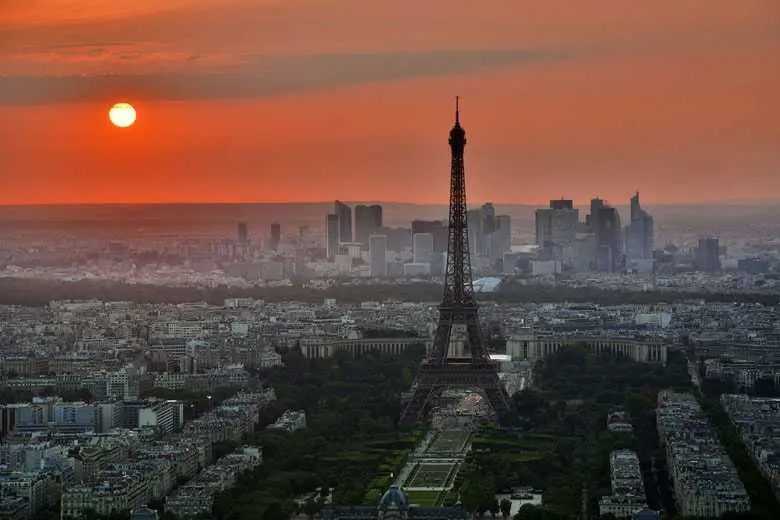
Lorem ipsum dolor sit amet
15.07.2020
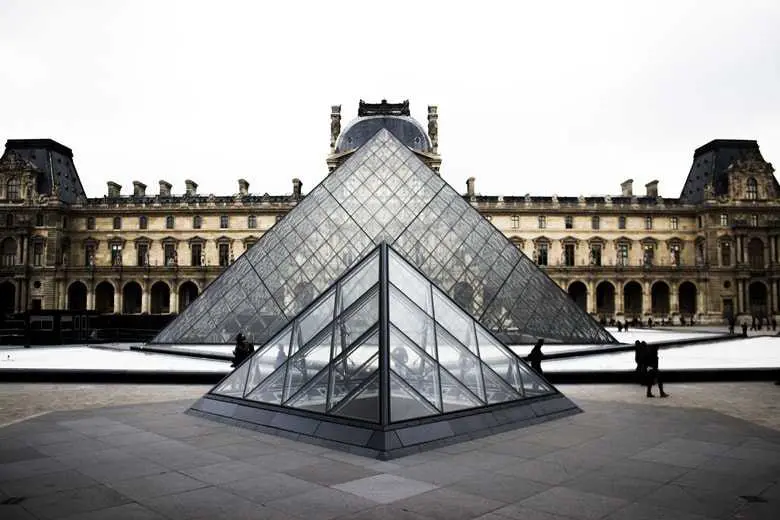
Lorem ipsum dolor sit amet
15.07.2020
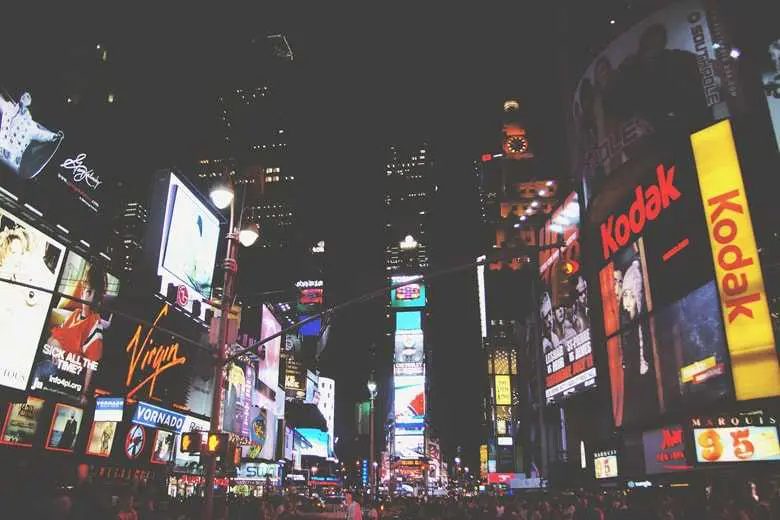
Lorem ipsum dolor sit amet
15.07.2020
This is title of the news
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit, iste aliquid. Sed id nihil magni, sint vero provident esse numquam perferendis ducimus dicta adipisci iusto nam temporibus modi animi laboriosam?
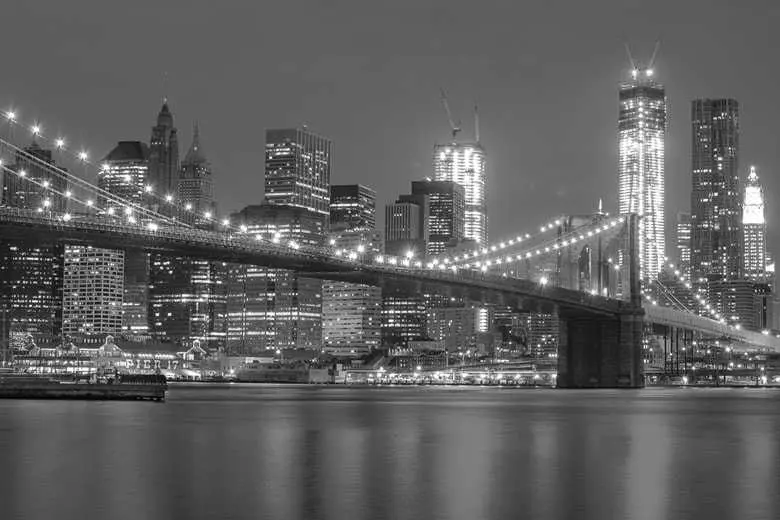
Lorem ipsum dolor sit amet
15.07.2020
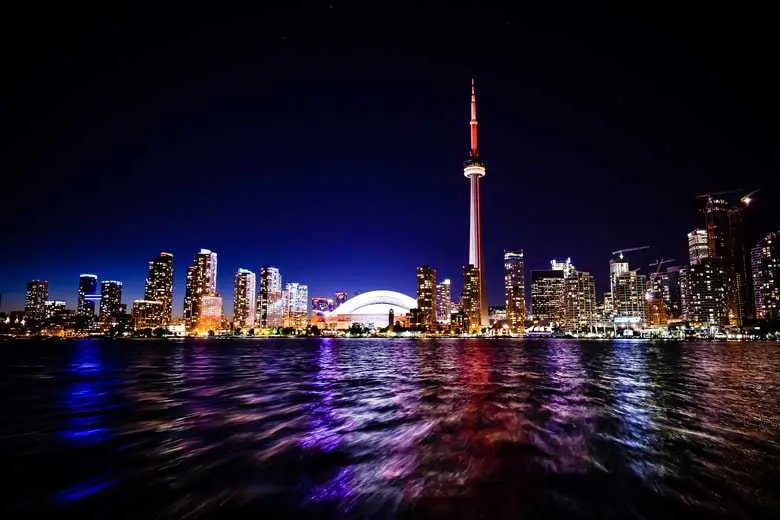
Lorem ipsum dolor sit amet
15.07.2020
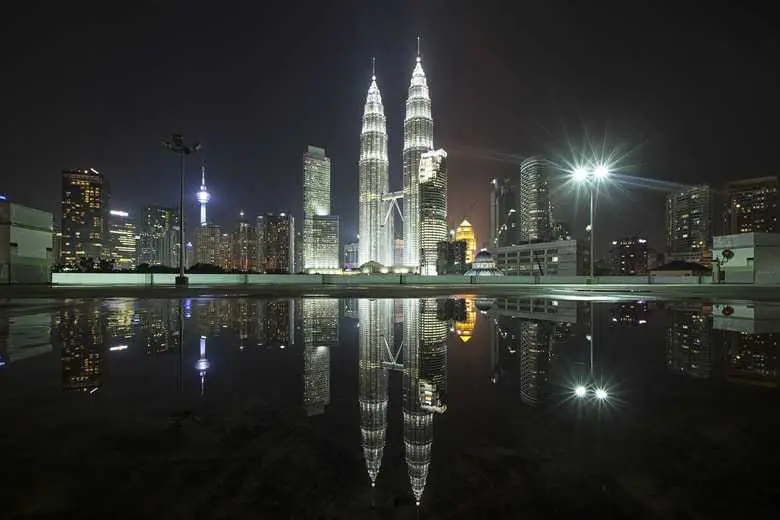
Lorem ipsum dolor sit amet
15.07.2020
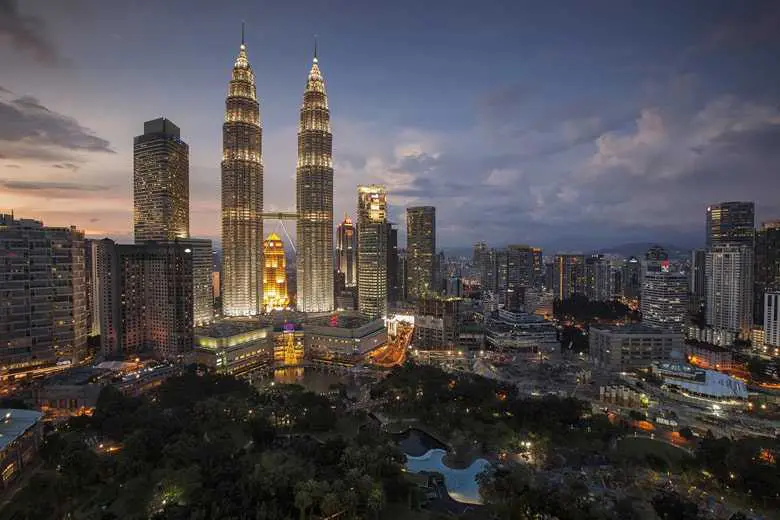
Lorem ipsum dolor sit amet
15.07.2020
import React from "react";
import {
MDBBtn,
MDBCard,
MDBContainer,
MDBCol,
MDBIcon,
MDBPagination,
MDBPaginationItem,
MDBPaginationLink,
MDBRipple,
MDBRow,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer className="py-5">
<MDBRow className="gx-5 border-bottom pb-4 mb-5">
<MDBCol md="6" className="mb-4">
<MDBRipple
className="bg-image hover-overlay ripple shadow-2-strong rounded-5"
rippleTag="div"
rippleColor="light"
>
<img
src="https://mdbcdn.b-cdn.net/img/new/slides/080.webp"
className="w-100"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol md="6" className="mb-4">
<span className="badge bg-danger px-2 py-1 shadow-1-strong mb-3">
News of the day
</span>
<h4>
<strong>Facilis consequatur eligendi</strong>
</h4>
<p className="text-muted">
Lorem ipsum dolor sit amet consectetur adipisicing elit. Facilis
consequatur eligendi quisquam doloremque vero ex debitis veritatis
placeat unde animi laborum sapiente illo possimus, commodi
dignissimos obcaecati illum maiores corporis.
</p>
<MDBBtn>Read More</MDBBtn>
</MDBCol>
</MDBRow>
<MDBRow className="gx-lg-5">
<MDBCol lg="4" md="6" className="mb-4 mb-lg-0">
<div>
<MDBRipple
className="bg-image hover-overlay shadow-1-strong ripple rounded-5 mb-4"
rippleTag="div"
rippleColor="light"
>
<img
src="https://mdbcdn.b-cdn.net/img/new/fluid/city/113.webp"
className="img-fluid"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
<MDBRow className="mb-3">
<MDBCol col="6">
<a href="" className="text-info">
<MDBIcon fas icon="plane" className="me-1" />
Travels
</a>
</MDBCol>
<MDBCol col="6" className="text-end">
<u> 15.07.2020</u>
</MDBCol>
</MDBRow>
<a href="#!" className="text-dark">
<h5>This is title of the news</h5>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit,
iste aliquid. Sed id nihil magni, sint vero provident esse
numquam perferendis ducimus dicta adipisci iusto nam temporibus
modi animi laboriosam?
</p>
</a>
<hr />
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/041.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/042.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/043.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/044.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
</div>
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4 mb-lg-0">
<div>
<MDBRipple
className="bg-image hover-overlay shadow-1-strong ripple rounded-5 mb-4"
rippleTag="div"
rippleColor="light"
>
<img
src="https://mdbcdn.b-cdn.net/img/new/fluid/city/011.webp"
className="img-fluid"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
<MDBRow className="mb-3">
<MDBCol col="6">
<a href="" className="text-danger">
<MDBIcon fas icon="chart-pie" className="me-1" />
Business
</a>
</MDBCol>
<MDBCol col="6" className="text-end">
<u> 15.07.2020</u>
</MDBCol>
</MDBRow>
<a href="#!" className="text-dark">
<h5>This is title of the news</h5>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit,
iste aliquid. Sed id nihil magni, sint vero provident esse
numquam perferendis ducimus dicta adipisci iusto nam temporibus
modi animi laboriosam?
</p>
</a>
<hr />
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/031.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/032.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/033.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/034.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
</div>
</MDBCol>
<MDBCol lg="4" md="12" className="mb-4 mb-lg-0">
<div>
<MDBRipple
className="bg-image hover-overlay shadow-1-strong ripple rounded-5 mb-4"
rippleTag="div"
rippleColor="light"
>
<img
src="https://mdbcdn.b-cdn.net/img/new/fluid/city/018.webp"
className="img-fluid"
/>
<a href="#!">
<div
className="mask"
style={{ backgroundColor: "rgba(251, 251, 251, 0.15)" }}
></div>
</a>
</MDBRipple>
<MDBRow className="mb-3">
<MDBCol col="6">
<a href="" className="text-warning">
<MDBIcon fas icon="code" className="me-1" />
Technology
</a>
</MDBCol>
<MDBCol col="6" className="text-end">
<u> 15.07.2020</u>
</MDBCol>
</MDBRow>
<a href="#!" className="text-dark">
<h5>This is title of the news</h5>
<p>
Lorem ipsum dolor sit amet consectetur adipisicing elit. Odit,
iste aliquid. Sed id nihil magni, sint vero provident esse
numquam perferendis ducimus dicta adipisci iusto nam temporibus
modi animi laboriosam?
</p>
</a>
<hr />
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/011.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/012.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/013.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
<a href="#!" className="text-dark">
<MDBRow className="mb-4 border-bottom pb-2">
<MDBCol size="3">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/014.webp"
className="img-fluid shadow-1-strong rounded"
alt="Hollywood Sign on The Hill"
/>
</MDBCol>
<MDBCol size="9">
<p className="mb-2">
<strong>Lorem ipsum dolor sit amet</strong>
</p>
<p>
<u> 15.07.2020</u>
</p>
</MDBCol>
</MDBRow>
</a>
</div>
</MDBCol>
</MDBRow>
<nav aria-label="...">
<MDBPagination circle className="mb-0 justify-content-center">
<MDBPaginationItem>
<MDBPaginationLink href="#" tabIndex={-1} aria-disabled="true">
Previous
</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">1</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem active>
<MDBPaginationLink href="#">
2 <span className="visually-hidden">(current)</span>
</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">3</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">Next</MDBPaginationLink>
</MDBPaginationItem>
</MDBPagination>
</nav>
</MDBContainer>
);
}
News feed
A compact newsfeed card with upvotes, downvotes and comments columns.
import React from "react";
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBContainer,
MDBPagination,
MDBPaginationItem,
MDBPaginationLink,
MDBIcon,
MDBTable,
MDBTableBody,
MDBTableHead,
} from "mdb-react-ui-kit";
export default function App() {
return (
<MDBContainer className="py-5">
<MDBCard className="d-flex" style={{ width: "48rem" }}>
<MDBCardBody>
<MDBTable hover forum responsive className="text-center">
<MDBTableHead>
<tr>
<th></th>
<th className="text-left">Topic</th>
<th>Comments</th>
</tr>
</MDBTableHead>
<MDBTableBody>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
Styling in the Shadow DOM With CSS Shadow Parts
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
5 obscure HTTP methods to impress your hipster friends
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
Rolling With The Punches: Shifting Attack Tactics &
Dropping Packets Faster & Cheaper At The Edge
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
Internship Experience: Cryptography Engineer
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
egghead: Nader Dabit - Full Stack Development in the Era of
Serverless Computing
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
10 Interesting JavaScript and CSS Libraries for April 2020
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
bash: Split a String into Parts with `cut` in Bash
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
Node v10.20.1 (LTS)
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
<tr>
<td scope="row" className="text-nowrap">
<MDBBtn
outline
color="dark"
size="sm"
className="p-1 m-0 waves-effect"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-up" className="ms-1" />
</MDBBtn>
<MDBBtn
outline
color="danger"
size="sm"
className="p-1 m-0 waves-effect mx-1"
>
<span className="value">0</span>
<MDBIcon fas icon="thumbs-down" className="ms-1" />
</MDBBtn>
</td>
<td className="text-start">
<a href="#" className="font-weight-bold blue-text">
React Digest Issue #247
</a>
<div className="my-2">
<a href="#" className="blue-text">
<strong>MDBootstrap</strong>
</a>
<span className="badge bg-secondary mx-1">staff</span>
<span className="badge bg-primary mx-1">pro</span>
<span className="badge bg-warning mx-1">premium</span>
<span>a year ago</span>
</div>
</td>
<td>
<a href="#" className="text-dark">
<span>0</span>
<MDBIcon fas icon="comments" className="ms-1" />
</a>
</td>
</tr>
</MDBTableBody>
</MDBTable>
<div className="d-flex justify-content-center">
<nav className="my-3 pt-2">
<MDBPagination circle className="mb-0">
<MDBPaginationItem>
<MDBPaginationLink
href="#"
tabIndex={-1}
aria-disabled="true"
>
Previous
</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">11</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">12</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem active>
<MDBPaginationLink href="#">
13 <span className="visually-hidden">(current)</span>
</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">14</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">15</MDBPaginationLink>
</MDBPaginationItem>
<MDBPaginationItem>
<MDBPaginationLink href="#">Next</MDBPaginationLink>
</MDBPaginationItem>
</MDBPagination>
</nav>
</div>
</MDBCardBody>
</MDBCard>
</MDBContainer>
);
}
Social media newsfeed v.1
Facebook-like newsfeed post with nested comments and user avatars built as media objects.
Lorem ipsum, dolor sit amet consectetur adipisicing elit. Atque ex non impedit corporis sunt nisi nam fuga dolor est, saepe vitae delectus fugit, accusantium qui nulla aut adipisci provident praesentium?