Order details
React Bootstrap 5 Order details component
Responsive React Order Details page built with the latest Bootstrap 5. eCommerce examples of shop pages with order summary, receipts, invoices, purchase lists & more.Basic example
Order details displayed inside of a popup. Check out the modal documentation to customize it further.
import {
MDBBtn,
MDBCol,
MDBContainer,
MDBIcon,
MDBModal,
MDBModalBody,
MDBModalContent,
MDBModalDialog,
MDBModalFooter,
MDBModalHeader,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
import React, { useState } from "react";
export default function Basic() {
const [basicModal, setBasicModal] = useState(false);
const toggleShow = () => setBasicModal(!basicModal);
return (
<>
<section className="vh-100" style={{ backgroundColor: "#35558a" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100 text-center">
<MDBCol>
<MDBBtn color="light" size="lg" onClick={toggleShow}>
<MDBIcon fas icon="info me-2" /> Get information
</MDBBtn>
<MDBModal show={basicModal} setShow={setBasicModal} tabIndex="-1">
<MDBModalDialog>
<MDBModalContent>
<MDBModalHeader className="border-bottom-0">
<MDBBtn
className="btn-close"
color="none"
onClick={toggleShow}
></MDBBtn>
</MDBModalHeader>
<MDBModalBody className="text-start text-black p-4">
<MDBTypography
tag="h5"
className="modal-title text-uppercase mb-5"
id="exampleModalLabel"
>
Johnatan Miller
</MDBTypography>
<MDBTypography
tag="h4"
className="mb-5"
style={{ color: "#35558a" }}
>
Thanks for your order
</MDBTypography>
<p className="mb-0" style={{ color: "#35558a" }}>
Payment summary
</p>
<hr
className="mt-2 mb-4"
style={{
height: "0",
backgroundColor: "transparent",
opacity: ".75",
borderTop: "2px dashed #9e9e9e",
}}
/>
<div className="d-flex justify-content-between">
<p className="fw-bold mb-0">Ether Chair(Qty:1)</p>
<p className="text-muted mb-0">$1750.00</p>
</div>
<div className="d-flex justify-content-between">
<p className="small mb-0">Shipping</p>
<p className="small mb-0">$175.00</p>
</div>
<div className="d-flex justify-content-between pb-1">
<p className="small">Tax</p>
<p className="small">$200.00</p>
</div>
<div className="d-flex justify-content-between">
<p className="fw-bold">Total</p>
<p className="fw-bold" style={{ color: "#35558a" }}>
$2125.00
</p>
</div>
</MDBModalBody>
<MDBModalFooter className="d-flex justify-content-center border-top-0 py-4">
<MDBBtn
size="lg"
style={{ backgroundColor: "#35558a" }}
className="mb-1"
>
Track your order
</MDBBtn>
</MDBModalFooter>
</MDBModalContent>
</MDBModalDialog>
</MDBModal>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
Order details #2
Order details card with detailed payment information and delivery status presented with a stepper.
Purchase Reciept
Date
10 April 2021
Order No.
012j1gvs356c
BEATS Solo 3 Wireless Headphones
£299.99
Shipping
£33.00
£262.99
Tracking Order
-
Ordered
-
Shipped
-
On the way
-
Delivered
Want any help? Please contact us
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBRow
} from "mdb-react-ui-kit";
export default function OrderDetails2() {
return (
<>
<section className="h-100 h-custom" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="8" xl="6">
<MDBCard className="border-top border-bottom border-3 border-color-custom">
<MDBCardBody className="p-5">
<p className="lead fw-bold mb-5" style={{ color: "#f37a27" }}>
Purchase Reciept
</p>
<MDBRow>
<MDBCol className="mb-3">
<p className="small text-muted mb-1">Date</p>
<p>10 April 2021</p>
</MDBCol>
<MDBCol className="mb-3">
<p className="small text-muted mb-1">Order No.</p>
<p>012j1gvs356c</p>
</MDBCol>
</MDBRow>
<div
className="mx-n5 px-5 py-4"
style={{ backgroundColor: "#f2f2f2" }}
>
<MDBRow>
<MDBCol md="8" lg="9">
<p>BEATS Solo 3 Wireless Headphones</p>
</MDBCol>
<MDBCol md="4" lg="3">
<p>£299.99</p>
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md="8" lg="9">
<p className="mb-0">Shipping</p>
</MDBCol>
<MDBCol md="4" lg="3">
<p className="mb-0">£33.00</p>
</MDBCol>
</MDBRow>
</div>
<MDBRow className="my-4">
<MDBCol md="4" className="offset-md-8 col-lg-3 offset-lg-9">
<p
className="lead fw-bold mb-0"
style={{ color: "#f37a27" }}
>
£262.99
</p>
</MDBCol>
</MDBRow>
<p
className="lead fw-bold mb-4 pb-2"
style={{ color: "#f37a27" }}
>
Tracking Order
</p>
<MDBRow>
<MDBCol lg="12">
<div className="horizontal-timeline">
<ul className="list-inline items d-flex justify-content-between">
<li className="list-inline-item items-list">
<p
className="py-1 px-2 rounded text-white"
style={{ backgroundColor: "#f37a27" }}
>
Ordered
</p>
</li>
<li className="list-inline-item items-list">
<p
className="py-1 px-2 rounded text-white"
style={{ backgroundColor: "#f37a27" }}
>
Shipped
</p>
</li>
<li className="list-inline-item items-list">
<p
className="py-1 px-2 rounded text-white"
style={{ backgroundColor: "#f37a27" }}
>
On the way
</p>
</li>
<li
className="list-inline-item items-list text-end"
style={{ marginRight: "-8px" }}
>
<p style={{ marginRight: "-8px" }}>Delivered</p>
</li>
</ul>
</div>
</MDBCol>
</MDBRow>
<p className="mt-4 pt-2 mb-0">
Want any help?{" "}
<a href="#!" style={{ color: "#f37a27" }}>
Please contact us
</a>
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
@media (min-width: 1025px) {
.h-custom {
height: 100vh !important;
}
}
.horizontal-timeline .items {
border-top: 2px solid #ddd;
}
.horizontal-timeline .items .items-list {
position: relative;
margin-right: 0;
}
.horizontal-timeline .items .items-list:before {
content: "";
position: absolute;
height: 8px;
width: 8px;
border-radius: 50%;
background-color: #ddd;
top: 0;
margin-top: -5px;
}
.horizontal-timeline .items .items-list {
padding-top: 15px;
}
.border-color-custom {
border-color: #f37a27 !important;
}
Order details #3
Order details page with product cards and delivery status presented with a progress bar.
Thanks for your Order, Anna!
Receipt
Receipt Voucher : 1KAU9-84UIL
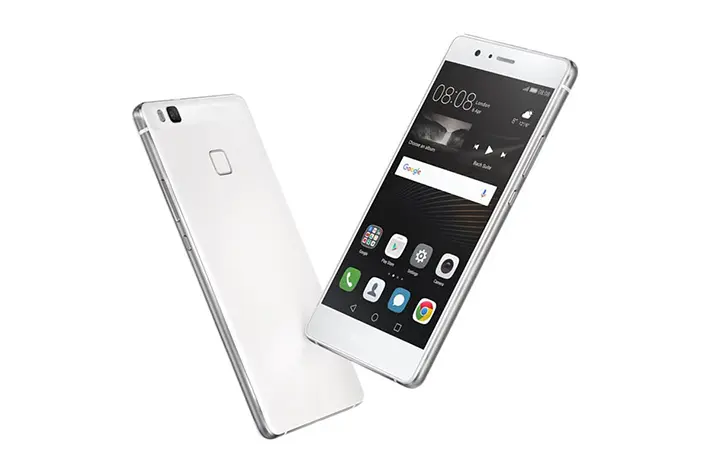
Samsung Galaxy
White
Capacity: 64GB
Qty: 1
$499
Track Order
Out for delivary
Delivered
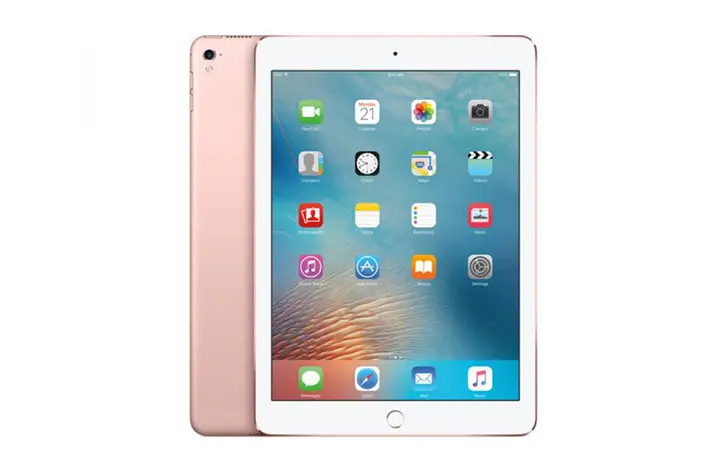
iPad
Pink rose
Capacity: 32GB
Qty: 1
$399
Track Order
Out for delivary
Delivered
Order Details
Total $898.00
Invoice Number : 788152
Discount $19.00
Invoice Date : 22 Dec,2019
GST 18% 123
Recepits Voucher : 18KU-62IIK
Delivery Charges Free
import {
MDBCard,
MDBCardBody,
MDBCardFooter,
MDBCardHeader,
MDBCardImage,
MDBCol,
MDBContainer,
MDBProgress,
MDBProgressBar,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
import React from "react";
export default function OrderDetails3() {
return (
<>
<section
className="h-100 gradient-custom"
style={{ backgroundColor: "#eee" }}
>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol lg="10" xl="8">
<MDBCard style={{ borderRadius: "10px" }}>
<MDBCardHeader className="px-4 py-5">
<MDBTypography tag="h5" className="text-muted mb-0">
Thanks for your Order,{" "}
<span style={{ color: "#a8729a" }}>Anna</span>!
</MDBTypography>
</MDBCardHeader>
<MDBCardBody className="p-4">
<div className="d-flex justify-content-between align-items-center mb-4">
<p
className="lead fw-normal mb-0"
style={{ color: "#a8729a" }}
>
Receipt
</p>
<p className="small text-muted mb-0">
Receipt Voucher : 1KAU9-84UIL
</p>
</div>
<MDBCard className="shadow-0 border mb-4">
<MDBCardBody>
<MDBRow>
<MDBCol md="2">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/13.webp"
fluid
alt="Phone"
/>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0">Samsung Galaxy</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">White</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">
Capacity: 64GB
</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">Qty: 1</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">$499</p>
</MDBCol>
</MDBRow>
<hr
className="mb-4"
style={{ backgroundColor: "#e0e0e0", opacity: 1 }}
/>
<MDBRow className="align-items-center">
<MDBCol md="2">
<p className="text-muted mb-0 small">Track Order</p>
</MDBCol>
<MDBCol md="10">
<MDBProgress
style={{ height: "6px", borderRadius: "16px" }}
>
<MDBProgressBar
style={{
borderRadius: "16px",
backgroundColor: "#a8729a",
}}
width={65}
valuemin={0}
valuemax={100}
/>
</MDBProgress>
<div className="d-flex justify-content-around mb-1">
<p className="text-muted mt-1 mb-0 small ms-xl-5">
Out for delivary
</p>
<p className="text-muted mt-1 mb-0 small ms-xl-5">
Delivered
</p>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="shadow-0 border mb-4">
<MDBCardBody>
<MDBRow>
<MDBCol md="2">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/1.webp"
fluid
alt="Phone"
/>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0">iPad</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">Pink rose</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">
Capacity: 32GB
</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">Qty: 1</p>
</MDBCol>
<MDBCol
md="2"
className="text-center d-flex justify-content-center align-items-center"
>
<p className="text-muted mb-0 small">$399</p>
</MDBCol>
</MDBRow>
<hr
className="mb-4"
style={{ backgroundColor: "#e0e0e0", opacity: 1 }}
/>
<MDBRow className="align-items-center">
<MDBCol md="2">
<p className="text-muted mb-0 small">Track Order</p>
</MDBCol>
<MDBCol md="10">
<MDBProgress
style={{ height: "6px", borderRadius: "16px" }}
>
<MDBProgressBar
style={{
borderRadius: "16px",
backgroundColor: "#a8729a",
}}
width={20}
valuemin={0}
valuemax={100}
/>
</MDBProgress>
<div className="d-flex justify-content-around mb-1">
<p className="text-muted mt-1 mb-0 small ms-xl-5">
Out for delivary
</p>
<p className="text-muted mt-1 mb-0 small ms-xl-5">
Delivered
</p>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<div className="d-flex justify-content-between pt-2">
<p className="fw-bold mb-0">Order Details</p>
<p className="text-muted mb-0">
<span className="fw-bold me-4">Total</span> $898.00
</p>
</div>
<div className="d-flex justify-content-between pt-2">
<p className="text-muted mb-0">Invoice Number : 788152</p>
<p className="text-muted mb-0">
<span className="fw-bold me-4">Discount</span> $19.00
</p>
</div>
<div className="d-flex justify-content-between">
<p className="text-muted mb-0">
Invoice Date : 22 Dec,2019
</p>
<p className="text-muted mb-0">
<span className="fw-bold me-4">GST 18%</span> 123
</p>
</div>
<div className="d-flex justify-content-between mb-5">
<p className="text-muted mb-0">
Recepits Voucher : 18KU-62IIK
</p>
<p className="text-muted mb-0">
<span className="fw-bold me-4">Delivery Charges</span>{" "}
Free
</p>
</div>
</MDBCardBody>
<MDBCardFooter
className="border-0 px-4 py-5"
style={{
backgroundColor: "#a8729a",
borderBottomLeftRadius: "10px",
borderBottomRightRadius: "10px",
}}
>
<MDBTypography
tag="h5"
className="d-flex align-items-center justify-content-end text-white text-uppercase mb-0"
>
Total paid: <span className="h2 mb-0 ms-2">$1040</span>
</MDBTypography>
</MDBCardFooter>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
.gradient-custom {
/* fallback for old browsers */
background: #cd9cf2;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to top left, rgba(205, 156, 242, 1), rgba(246, 243, 255, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to top left, rgba(205, 156, 242, 1), rgba(246, 243, 255, 1))
}
Order details #4
Order status popup with detailed shipper and address information.
import React, { useState } from "react";
import {
MDBBtn,
MDBCol,
MDBContainer,
MDBIcon,
MDBModal,
MDBModalBody,
MDBModalContent,
MDBModalDialog,
MDBModalHeader,
MDBRow
} from "mdb-react-ui-kit";
export default function OrderDetails4() {
const [basicModal, setBasicModal] = useState(false);
const toggleShow = () => setBasicModal(!basicModal);
return (
<>
<section className="vh-100" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100 text-center">
<MDBCol>
<MDBBtn size="lg" onClick={toggleShow}>
Track your order
</MDBBtn>
<MDBModal show={basicModal} setShow={setBasicModal} tabIndex="-1">
<MDBModalDialog size="lg">
<MDBModalContent
className="text-white"
style={{ backgroundColor: "#6d5b98", borderRadius: "10px" }}
>
<MDBModalHeader className="border-bottom-0">
<MDBBtn
className="btn-close btn-close-white"
color="none"
onClick={toggleShow}
></MDBBtn>
</MDBModalHeader>
<MDBModalBody className="text-start px-4 pt-0 pb-4">
<div className="text-center">
<h5 className="mb-3">Order Status</h5>
<h5 className="mb-5">AWB Number-5335T5S</h5>
</div>
<div className="d-flex justify-content-between mb-5">
<div className="text-center">
<i className="fas fa-circle"></i>
<p>Order placed</p>
</div>
<div className="text-center">
<i className="fas fa-circle"></i>
<p>In Transit</p>
</div>
<div className="text-center">
<i className="fas fa-circle"></i>
<p className="lead fw-normal">Out of Delivery</p>
</div>
<div className="text-center">
<i
className="fas fa-circle"
style={{ color: "#979595" }}
></i>
<p style={{ color: "#979595" }}>Delivered</p>
</div>
</div>
<MDBRow className="justify-content-center">
<div className="col-md-4 text-center">
<p className="lead fw-normal">Shipped with</p>
</div>
<div className="col-md-4 text-center">
<p className="lead fw-normal">UPS Expedited</p>
</div>
<div className="col-md-2 text-center">
<MDBIcon fas icon="phone" size="lg" />
</div>
</MDBRow>
<MDBRow className="justify-content-center">
<div className="col-md-4 text-center">
<p className="lead fw-normal">Estimated Delivery</p>
</div>
<div className="col-md-4 text-center">
<p className="lead fw-normal">02/12/2017</p>
</div>
<div className="col-md-2 text-center">
<MDBIcon fas icon="envelope" size="lg" />
</div>
</MDBRow>
</MDBModalBody>
</MDBModalContent>
</MDBModalDialog>
</MDBModal>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
Order details #5
A detailed product card with simple delivery status. Ideal for replacing item cards after purchase on shopping cart pages.
Order ID 1222528743
Place On 12,March 2019
Headphones Bose 35 II
Qt: 1 item
$ 299 via (COD)
Tracking Status on: 11:30pm, Today
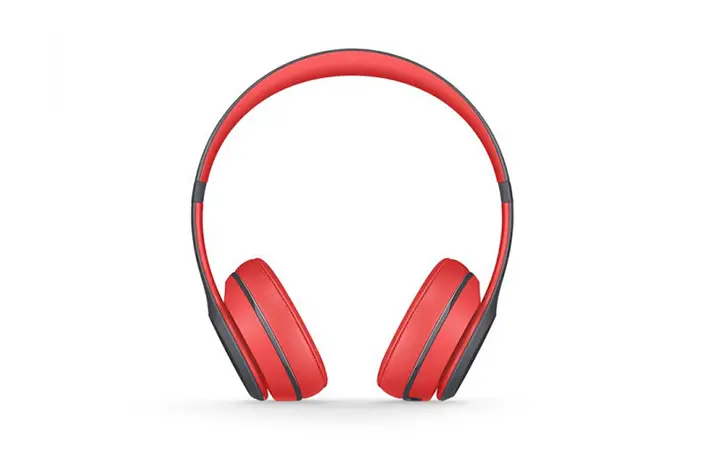
- PLACED
- SHIPPED
- DELIVERED
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCardFooter,
MDBCardHeader,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function OrderDetails5() {
return (
<>
<section className="vh-100 gradient-custom-2">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol md="10" lg="8" xl="6">
<MDBCard
className="card-stepper"
style={{ borderRadius: "16px" }}
>
<MDBCardHeader className="p-4">
<div className="d-flex justify-content-between align-items-center">
<div>
<p className="text-muted mb-2">
{" "}
Order ID{" "}
<span className="fw-bold text-body">1222528743</span>
</p>
<p className="text-muted mb-0">
{" "}
Place On{" "}
<span className="fw-bold text-body">
12,March 2019
</span>{" "}
</p>
</div>
<div>
<MDBTypography tag="h6" className="mb-0">
{" "}
<a href="#">View Details</a>{" "}
</MDBTypography>
</div>
</div>
</MDBCardHeader>
<MDBCardBody className="p-4">
<div className="d-flex flex-row mb-4 pb-2">
<div className="flex-fill">
<MDBTypography tag="h5" className="bold">
Headphones Bose 35 II
</MDBTypography>
<p className="text-muted"> Qt: 1 item</p>
<MDBTypography tag="h4" className="mb-3">
{" "}
$ 299{" "}
<span className="small text-muted"> via (COD) </span>
</MDBTypography>
<p className="text-muted">
Tracking Status on:{" "}
<span className="text-body">11:30pm, Today</span>
</p>
</div>
<div>
<MDBCardImage
fluid
className="align-self-center"
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/6.webp"
width="250"
/>
</div>
</div>
<ul
id="progressbar-1"
className="mx-0 mt-0 mb-5 px-0 pt-0 pb-4"
>
<li className="step0 active" id="step1">
<span style={{ marginLeft: "22px", marginTop: "12px" }}>
PLACED
</span>
</li>
<li className="step0 active text-center" id="step2">
<span>SHIPPED</span>
</li>
<li className="step0 text-muted text-end" id="step3">
<span style={{ marginRight: "22px" }}>DELIVERED</span>
</li>
</ul>
</MDBCardBody>
<MDBCardFooter className="p-4">
<div className="d-flex justify-content-between">
<MDBTypography tag="h5" className="fw-normal mb-0">
<a href="#!">Track</a>
</MDBTypography>
<div className="border-start h-100"></div>
<MDBTypography tag="h5" className="fw-normal mb-0">
<a href="#!">Cancel</a>
</MDBTypography>
<div className="border-start h-100"></div>
<MDBTypography tag="h5" className="fw-normal mb-0">
<a href="#!">Pre-pay</a>
</MDBTypography>
<div className="border-start h-100"></div>
<MDBTypography tag="h5" className="fw-normal mb-0">
<a href="#!" className="text-muted">
<MDBIcon fas icon="ellipsis-v" />
</a>
</MDBTypography>
</div>
</MDBCardFooter>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
.gradient-custom-2 {
/* fallback for old browsers */
background: #a1c4fd;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(161, 196, 253, 1), rgba(194, 233, 251, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(161, 196, 253, 1), rgba(194, 233, 251, 1))
}
#progressbar-1 {
color: #455A64;
}
#progressbar-1 li {
list-style-type: none;
font-size: 13px;
width: 33.33%;
float: left;
position: relative;
}
#progressbar-1 #step1:before {
content: "1";
color: #fff;
width: 29px;
margin-left: 22px;
padding-left: 11px;
}
#progressbar-1 #step2:before {
content: "2";
color: #fff;
width: 29px;
}
#progressbar-1 #step3:before {
content: "3";
color: #fff;
width: 29px;
margin-right: 22px;
text-align: center;
}
#progressbar-1 li:before {
line-height: 29px;
display: block;
font-size: 12px;
background: #455A64;
border-radius: 50%;
margin: auto;
}
#progressbar-1 li:after {
content: '';
width: 121%;
height: 2px;
background: #455A64;
position: absolute;
left: 0%;
right: 0%;
top: 15px;
z-index: -1;
}
#progressbar-1 li:nth-child(2):after {
left: 50%
}
#progressbar-1 li:nth-child(1):after {
left: 25%;
width: 121%
}
#progressbar-1 li:nth-child(3):after {
left: 25%;
width: 50%;
}
#progressbar-1 li.active:before,
#progressbar-1 li.active:after {
background: #1266f1;
}
.card-stepper {
z-index: 0
}
Order details #6
Product delivery status card with invoice number and simple status tracking with slick icons.
INVOICE #Y34XDHR
Expected Arrival 01/12/19
USPS 234094567242423422898
Order
Processed
Order
Shipped
Order
En Route
Order
Arrived
import React from "react";
import {
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function OrderDetails6() {
return (
<>
<section className="vh-100" style={{ backgroundColor: "#8c9eff" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol size="12">
<MDBCard
className="card-stepper text-black"
style={{ borderRadius: "16px" }}
>
<MDBCardBody className="p-5">
<div className="d-flex justify-content-between align-items-center mb-5">
<div>
<MDBTypography tag="h5" className="mb-0">
INVOICE{" "}
<span className="text-primary font-weight-bold">
#Y34XDHR
</span>
</MDBTypography>
</div>
<div className="text-end">
<p className="mb-0">
Expected Arrival <span>01/12/19</span>
</p>
<p className="mb-0">
USPS{" "}
<span className="font-weight-bold">
234094567242423422898
</span>
</p>
</div>
</div>
<ul
id="progressbar-2"
className="d-flex justify-content-between mx-0 mt-0 mb-5 px-0 pt-0 pb-2"
>
<li className="step0 active text-center" id="step1"></li>
<li className="step0 active text-center" id="step2"></li>
<li className="step0 active text-center" id="step3"></li>
<li className="step0 text-muted text-end" id="step4"></li>
</ul>
<div className="d-flex justify-content-between">
<div className="d-lg-flex align-items-center">
<MDBIcon fas icon="clipboard-list me-lg-4 mb-3 mb-lg-0" size="3x" />
<div>
<p className="fw-bold mb-1">Order</p>
<p className="fw-bold mb-0">Processed</p>
</div>
</div>
<div className="d-lg-flex align-items-center">
<MDBIcon fas icon="box-open me-lg-4 mb-3 mb-lg-0" size="3x" />
<div>
<p className="fw-bold mb-1">Order</p>
<p className="fw-bold mb-0">Shipped</p>
</div>
</div>
<div className="d-lg-flex align-items-center">
<MDBIcon fas icon="shipping-fast me-lg-4 mb-3 mb-lg-0" size="3x" />
<div>
<p className="fw-bold mb-1">Order</p>
<p className="fw-bold mb-0">En Route</p>
</div>
</div>
<div className="d-lg-flex align-items-center">
<MDBIcon fas icon="home me-lg-4 mb-3 mb-lg-0" size="3x" />
<div>
<p className="fw-bold mb-1">Order</p>
<p className="fw-bold mb-0">Arrived</p>
</div>
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
.card-stepper {
z-index: 0
}
#progressbar-2 {
color: #455A64;
}
#progressbar-2 li {
list-style-type: none;
font-size: 13px;
width: 33.33%;
float: left;
position: relative;
}
#progressbar-2 #step1:before {
content: '\f058';
font-family: "Font Awesome 5 Free";
color: #fff;
width: 37px;
margin-left: 0px;
padding-left: 0px;
}
#progressbar-2 #step2:before {
content: '\f058';
font-family: "Font Awesome 5 Free";
color: #fff;
width: 37px;
}
#progressbar-2 #step3:before {
content: '\f058';
font-family: "Font Awesome 5 Free";
color: #fff;
width: 37px;
margin-right: 0;
text-align: center;
}
#progressbar-2 #step4:before {
content: '\f111';
font-family: "Font Awesome 5 Free";
color: #fff;
width: 37px;
margin-right: 0;
text-align: center;
}
#progressbar-2 li:before {
line-height: 37px;
display: block;
font-size: 12px;
background: #c5cae9;
border-radius: 50%;
}
#progressbar-2 li:after {
content: '';
width: 100%;
height: 10px;
background: #c5cae9;
position: absolute;
left: 0%;
right: 0%;
top: 15px;
z-index: -1;
}
#progressbar-2 li:nth-child(1):after {
left: 1%;
width: 100%
}
#progressbar-2 li:nth-child(2):after {
left: 1%;
width: 100%;
}
#progressbar-2 li:nth-child(3):after {
left: 1%;
width: 100%;
background: #c5cae9 !important;
}
#progressbar-2 li:nth-child(4) {
left: 0;
width: 37px;
}
#progressbar-2 li:nth-child(4):after {
left: 0;
width: 0;
}
#progressbar-2 li.active:before,
#progressbar-2 li.active:after {
background: #6520ff;
}
Order details #7
Compact status card with a horizontal timeline.
import React from "react";
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardFooter,
MDBCardHeader,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function OrderDetails7() {
return (
<>
<section className="vh-100" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol>
<MDBCard
className="card-stepper"
style={{ borderRadius: "10px" }}
>
<MDBCardBody className="p-4">
<div className="d-flex justify-content-between align-items-center">
<div className="d-flex flex-column">
<span className="lead fw-normal">
Your order has been delivered
</span>
<span className="text-muted small">
by DHFL on 21 Jan, 2020
</span>
</div>
<div>
<MDBBtn outline>Track order details</MDBBtn>
</div>
</div>
<hr className="my-4" />
<div className="d-flex flex-row justify-content-between align-items-center align-content-center">
<span className="dot"></span>
<hr className="flex-fill track-line" />
<span className="dot"></span>
<hr className="flex-fill track-line" />
<span className="dot"></span>
<hr className="flex-fill track-line" />
<span className="dot"></span>
<hr className="flex-fill track-line" />
<span className="d-flex justify-content-center align-items-center big-dot dot">
<MDBIcon icon="check text-white" />
</span>
</div>
<div className="d-flex flex-row justify-content-between align-items-center">
<div className="d-flex flex-column align-items-start">
<span>15 Mar</span>
<span>Order placed</span>
</div>
<div className="d-flex flex-column justify-content-center">
<span>15 Mar</span>
<span>Order placed</span>
</div>
<div className="d-flex flex-column justify-content-center align-items-center">
<span>15 Mar</span>
<span>Order Dispatched</span>
</div>
<div className="d-flex flex-column align-items-center">
<span>15 Mar</span>
<span>Out for delivery</span>
</div>
<div className="d-flex flex-column align-items-end">
<span>15 Mar</span>
<span>Delivered</span>
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
</>
);
}
.track-line {
height: 2px !important;
background-color: #488978;
opacity: 1;
}
.dot {
height: 10px;
width: 10px;
margin-left: 3px;
margin-right: 3px;
margin-top: 0px;
background-color: #488978;
border-radius: 50%;
display: inline-block
}
.big-dot {
height: 25px;
width: 25px;
margin-left: 0px;
margin-right: 0px;
margin-top: 0px;
background-color: #488978;
border-radius: 50%;
display: inline-block;
}
.big-dot i {
font-size: 12px;
}
.card-stepper {
z-index: 0
}