Registration form
React Bootstrap 5 Registration form
Responsive React Registration form built with Bootstrap 5. Templates for signup forms, registration popups, register modal designs, registration validation & more.
Basic registration form example
This registration form card uses grid functionalities and simple input fields. Check out the validation documentation, to learn how to customize validation messages and types for your registration input fields.
Sign up
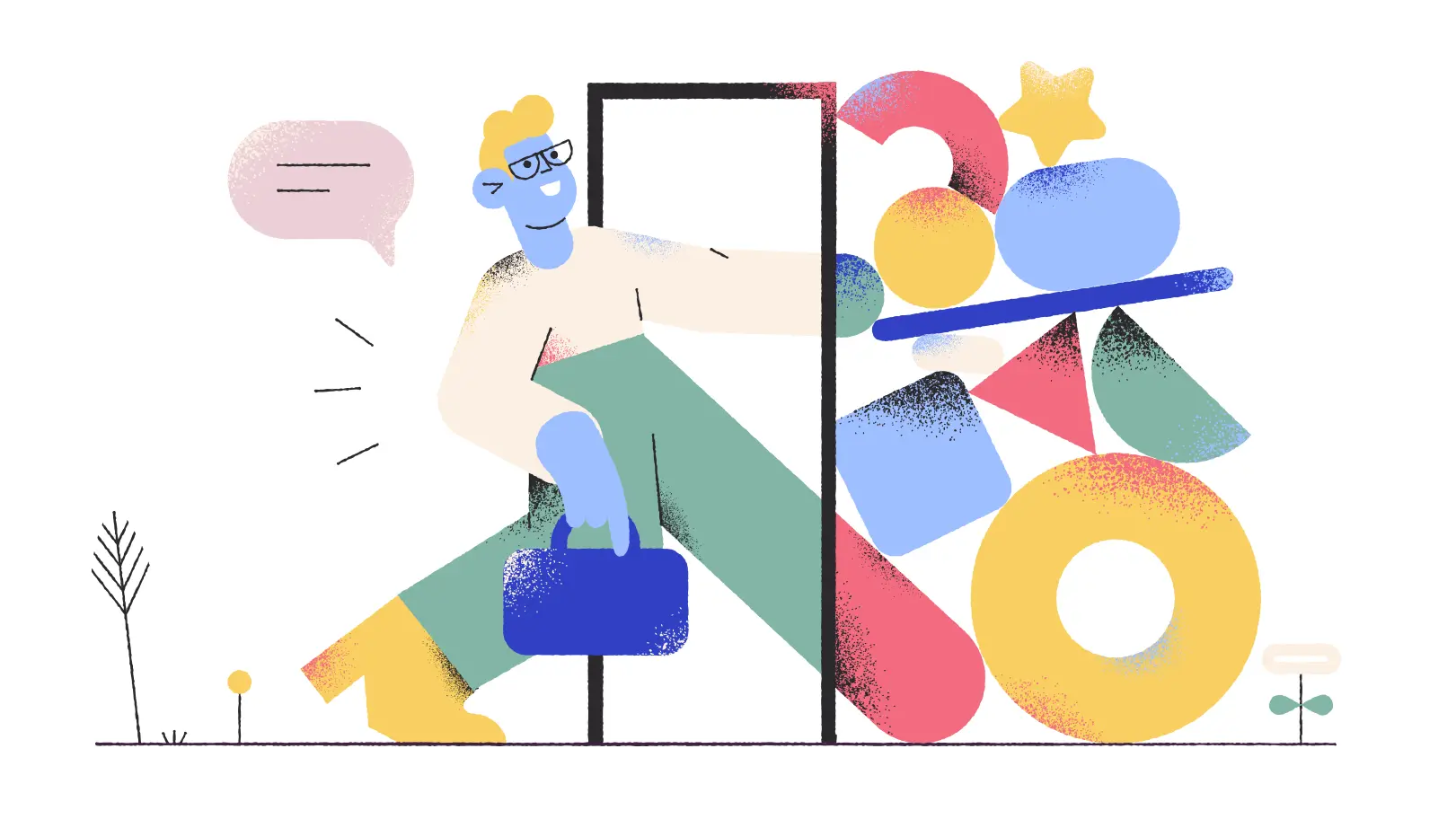
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBInput,
MDBIcon,
MDBCheckbox
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid>
<MDBCard className='text-black m-5' style={{borderRadius: '25px'}}>
<MDBCardBody>
<MDBRow>
<MDBCol md='10' lg='6' className='order-2 order-lg-1 d-flex flex-column align-items-center'>
<p classNAme="text-center h1 fw-bold mb-5 mx-1 mx-md-4 mt-4">Sign up</p>
<div className="d-flex flex-row align-items-center mb-4 ">
<MDBIcon fas icon="user me-3" size='lg'/>
<MDBInput label='Your Name' id='form1' type='text' className='w-100'/>
</div>
<div className="d-flex flex-row align-items-center mb-4">
<MDBIcon fas icon="envelope me-3" size='lg'/>
<MDBInput label='Your Email' id='form2' type='email'/>
</div>
<div className="d-flex flex-row align-items-center mb-4">
<MDBIcon fas icon="lock me-3" size='lg'/>
<MDBInput label='Password' id='form3' type='password'/>
</div>
<div className="d-flex flex-row align-items-center mb-4">
<MDBIcon fas icon="key me-3" size='lg'/>
<MDBInput label='Repeat your password' id='form4' type='password'/>
</div>
<div className='mb-4'>
<MDBCheckbox name='flexCheck' value='' id='flexCheckDefault' label='Subscribe to our newsletter' />
</div>
<MDBBtn className='mb-4' size='lg'>Register</MDBBtn>
</MDBCol>
<MDBCol md='10' lg='6' className='order-1 order-lg-2 d-flex align-items-center'>
<MDBCardImage src='https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-registration/draw1.webp' fluid/>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBContainer>
);
}
export default App;
Registration form with select
Another variation of a simple sign up form. Note that, registration forms are different than login forms, since they are used to create a new account instead of logging into an existing one. They usually contain more input fields as well as different types of input fields to collect extensive data about the user during registration. Selects are one of the most common input types used in registration forms, the example below uses a standard select, input, but you could also consider with a multiselect or a select with custom "other" option input.
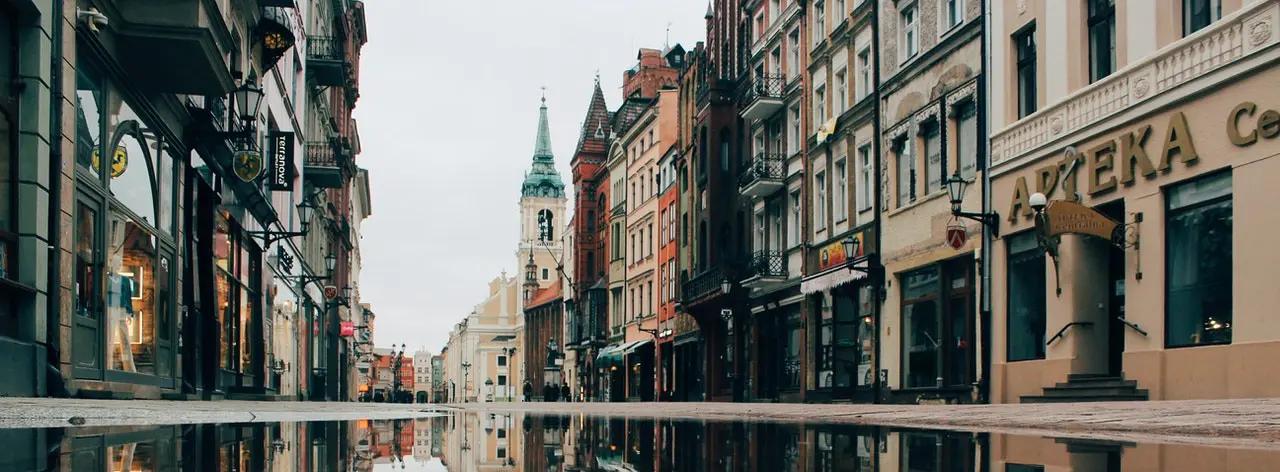
Registration Info
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBRow,
MDBCol,
MDBInput,
MDBSelect
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid>
<MDBRow className='d-flex justify-content-center align-items-center'>
<MDBCol lg='8'>
<MDBCard className='my-5 rounded-3' style={{maxWidth: '600px'}}>
<MDBCardImage src='https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-registration/img3.webp' className='w-100 rounded-top' alt="Sample photo"/>
<MDBCardBody className='px-5'>
<h3 className="mb-4 pb-2 pb-md-0 mb-md-5 px-md-2">Registration Info</h3>
<MDBInput wrapperClass='mb-4' label='Name' id='form1' type='text'/>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='datepicker mb-4' label='Select a date' id='form2' type='text'/>
</MDBCol>
<MDBCol md='6' className='mb-4'>
<MDBSelect
data={[
{ text: 'Gender', value: 1, disabled: true },
{ text: 'Female', value: 2 },
{ text: 'Male', value: 3 }
]}
/>
</MDBCol>
</MDBRow>
<MDBSelect
className='mb-4'
data={[
{ text: 'Class', value: 1, disabled: true },
{ text: 'Class 1', value: 2 },
{ text: 'Class 2', value: 3 },
{ text: 'Class 3', value: 3 }
]}
/>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Registration code' id='form3' type='text'/>
</MDBCol>
</MDBRow>
<MDBBtn color='success' className='mb-4' size='lg'>Submit</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #8fc4b7;
}
Registration form with radio buttons
This example utilizes the radio button component for the selection of gender.
Registration Form
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBInput,
MDBSelect,
MDBRadio
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid>
<MDBRow className='justify-content-center align-items-center m-5'>
<MDBCard>
<MDBCardBody className='px-4'>
<h3 className="fw-bold mb-4 pb-2 pb-md-0 mb-md-5">Registration Form</h3>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='First Name' size='lg' id='form1' type='text'/>
</MDBCol>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Last Name' size='lg' id='form2' type='text'/>
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Birthday' size='lg' id='form3' type='text'/>
</MDBCol>
<MDBCol md='6' className='mb-4'>
<h6 className="fw-bold">Gender: </h6>
<MDBRadio name='inlineRadio' id='inlineRadio1' value='option1' label='Female' inline />
<MDBRadio name='inlineRadio' id='inlineRadio2' value='option2' label='Male' inline />
<MDBRadio name='inlineRadio' id='inlineRadio3' value='option3' label='Other' inline />
</MDBCol>
</MDBRow>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Email' size='lg' id='form4' type='email'/>
</MDBCol>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Phone Number' size='lg' id='form5' type='rel'/>
</MDBCol>
</MDBRow>
<MDBSelect
label='Choose option'
className='mb-4'
size='lg'
data={[
{ text: 'Choose option', value: 1, disabled: true },
{ text: 'Subject 1', value: 2 },
{ text: 'Subject 2', value: 3 },
{ text: 'Subject 3', value: 4 }
]}
/>
<MDBBtn className='mb-4' size='lg'>Submit</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
/* fallback for old browsers */
background: #f093fb;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to bottom right, rgba(240, 147, 251, 1), rgba(245, 87, 108, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to bottom right, rgba(240, 147, 251, 1), rgba(245, 87, 108, 1))
}
Full page registration template
If you need to add more input fields to your form, you should consider making it fullpage. We also recommend to add a "reset all" button in such cases to make it easy to clear all of the fields at once. Full page registration is commonly used in combination with credit card inputs or other payment forms when you need to create a user profile during the checkout process. Instead of making your form fullpage, you can also consider using a stepper component, to spread your inputs over many steps.
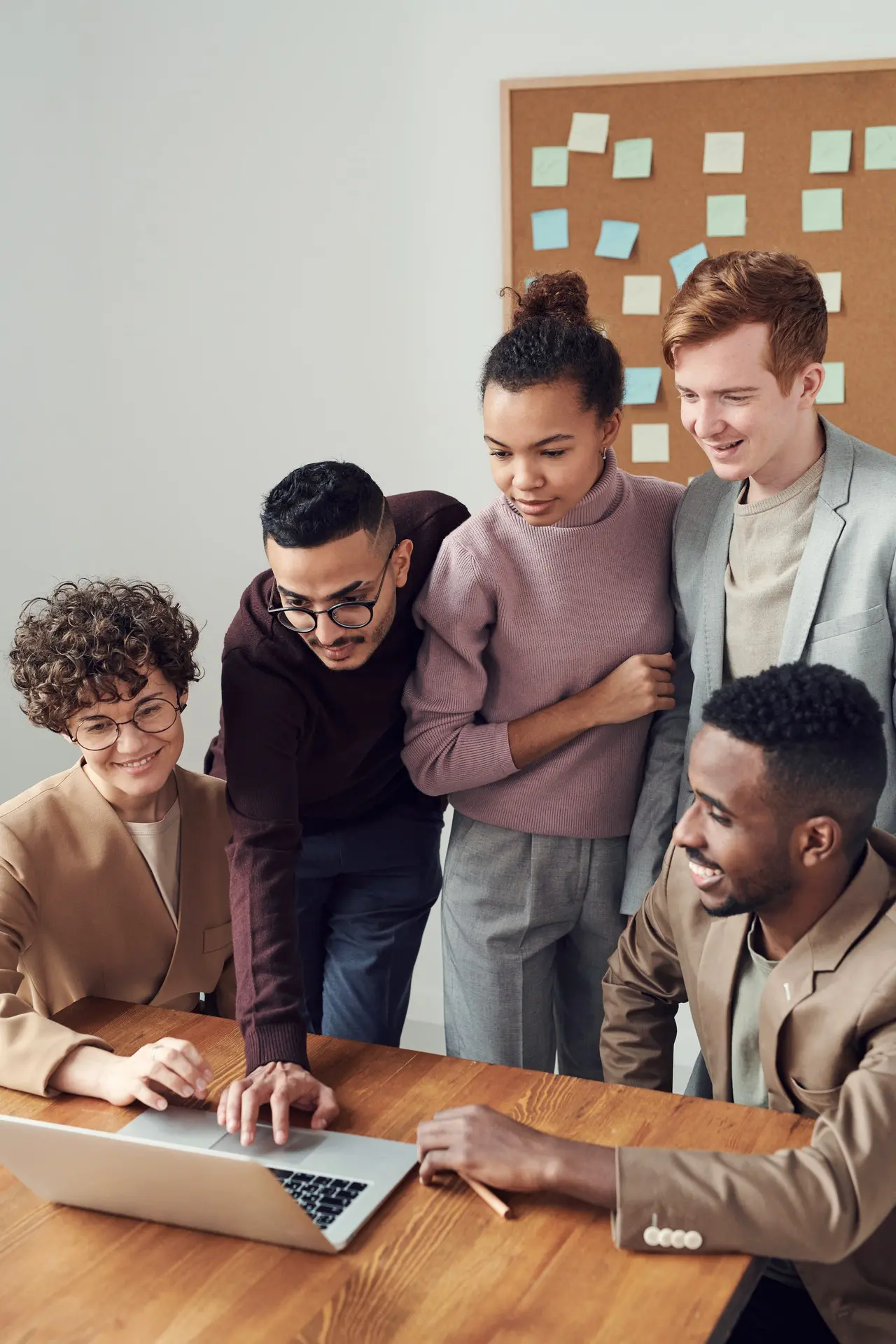
Student registration form
Gender:
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBRow,
MDBCol,
MDBInput,
MDBRadio,
MDBSelect
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid className='bg-dark'>
<MDBRow className='d-flex justify-content-center align-items-center h-100'>
<MDBCol>
<MDBCard className='my-4'>
<MDBRow className='g-0'>
<MDBCol md='6' className="d-none d-md-block">
<MDBCardImage src='https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-registration/img4.webp' alt="Sample photo" className="rounded-start" fluid/>
</MDBCol>
<MDBCol md='6'>
<MDBCardBody className='text-black d-flex flex-column justify-content-center'>
<h3 className="mb-5 text-uppercase fw-bold">Student registration form</h3>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='First Name' size='lg' id='form1' type='text'/>
</MDBCol>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Last Name' size='lg' id='form2' type='text'/>
</MDBCol>
</MDBRow>
<MDBInput wrapperClass='mb-4' label='Birthday' size='lg' id='form3' type='text'/>
<div className='d-md-flex ustify-content-start align-items-center mb-4'>
<h6 class="fw-bold mb-0 me-4">Gender: </h6>
<MDBRadio name='inlineRadio' id='inlineRadio1' value='option1' label='Female' inline />
<MDBRadio name='inlineRadio' id='inlineRadio2' value='option2' label='Male' inline />
<MDBRadio name='inlineRadio' id='inlineRadio3' value='option3' label='Other' inline />
</div>
<MDBRow>
<MDBCol md='6'>
<MDBSelect
className='mb-4'
size='lg'
data={[
{ text: 'State', value: 1 },
{ text: 'Option 1', value: 2 },
{ text: 'Option 2', value: 3 },
{ text: 'Option 3', value: 4 }
]}
/>
</MDBCol>
<MDBCol md='6'>
<MDBSelect
className='mb-4'
size='lg'
data={[
{ text: 'City', value: 1 },
{ text: 'Option 1', value: 2 },
{ text: 'Option 2', value: 3 },
{ text: 'Option 3', value: 4 }
]}
/>
</MDBCol>
</MDBRow>
<MDBInput wrapperClass='mb-4' label='Pincode' size='lg' id='form4' type='text'/>
<MDBInput wrapperClass='mb-4' label='Course' size='lg' id='form5' type='text'/>
<MDBInput wrapperClass='mb-4' label='Email ID' size='lg' id='form6' type='text'/>
<div className="d-flex justify-content-end pt-3">
<MDBBtn color='light' size='lg'>Reset all</MDBBtn>
<MDBBtn className='ms-2' color='warning' size='lg'>Submit form</MDBBtn>
</div>
</MDBCardBody>
</MDBCol>
</MDBRow>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
Application form with upload
The example below gives the user an option to upload a pdf with their CV using a file input. For more examples and different designs of the file input (i.e. using drag & drop), check out our file upload plugin.
Apply for a job
Full name
Email address
Full name
Upload CV
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBInput,
MDBTextArea,
MDBFile
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid>
<MDBRow className='d-flex justify-content-center align-items-center'>
<MDBCol lg='9' className='my-5'>
<h1 class="text-white mb-4">Apply for a job</h1>
<MDBCard>
<MDBCardBody className='px-4'>
<MDBRow className='align-items-center pt-4 pb-3'>
<MDBCol md='3' className='ps-5'>
<h6 className="mb-0">Full name</h6>
</MDBCol>
<MDBCol md='9' className='pe-5'>
<MDBInput label='Email' size='lg' id='form1' type='text'/>
</MDBCol>
</MDBRow>
<hr className="mx-n3" />
<MDBRow className='align-items-center pt-4 pb-3'>
<MDBCol md='3' className='ps-5'>
<h6 className="mb-0">Email address</h6>
</MDBCol>
<MDBCol md='9' className='pe-5'>
<MDBInput label='example@example.com' size='lg' id='form2' type='email'/>
</MDBCol>
</MDBRow>
<hr className="mx-n3" />
<MDBRow className='align-items-center pt-4 pb-3'>
<MDBCol md='3' className='ps-5'>
<h6 className="mb-0">Message</h6>
</MDBCol>
<MDBCol md='9' className='pe-5'>
<MDBTextArea label='Message' id='textAreaExample' rows={3} />
</MDBCol>
</MDBRow>
<hr className="mx-n3" />
<MDBRow className='align-items-center pt-4 pb-3'>
<MDBCol md='3' className='ps-5'>
<h6 className="mb-0">Upload CV</h6>
</MDBCol>
<MDBCol md='9' className='pe-5'>
<MDBFile size='lg' id='customFile' />
<div className="small text-muted mt-2">Upload your CV/Resume or any other relevant file. Max file size 50 MB</div>
</MDBCol>
</MDBRow>
<hr className="mx-n3" />
<MDBBtn className='my-4' size='lg'>send application</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
background-color: #2779e2;
}
Registration form template with address
The example below contains an extended space dedicated to address forms and a checkbox for accepting "Terms and Conditions".
General Infomation
Contact Details
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBRow,
MDBCol,
MDBCard,
MDBCardBody,
MDBSelect,
MDBInput,
MDBCheckbox
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid className='h-custom'>
<MDBRow className='d-flex justify-content-center align-items-center h-100'>
<MDBCol col='12' className='m-5'>
<MDBCard className='card-registration card-registration-2' style={{borderRadius: '15px'}}>
<MDBCardBody className='p-0'>
<MDBRow>
<MDBCol md='6' className='p-5 bg-white'>
<h3 className="fw-normal mb-5" style={{color: '#4835d4'}}>General Infomation</h3>
<MDBSelect
className='mb-4'
size='lg'
data={[
{ text: 'Titile', value: 1 },
{ text: 'Two', value: 2 },
{ text: 'Three', value: 3 },
{ text: 'Four', value: 4 }
]}
/>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='First Name' size='lg' id='form1' type='text'/>
</MDBCol>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Last Name' size='lg' id='form2' type='text'/>
</MDBCol>
</MDBRow>
<MDBSelect
className='mb-4'
size='lg'
data={[
{ text: 'Position', value: 1 },
{ text: 'Two', value: 2 },
{ text: 'Three', value: 3 },
{ text: 'Four', value: 4 }
]}
/>
<MDBInput wrapperClass='mb-4' label='Position' size='lg' id='form3' type='text'/>
<MDBRow>
<MDBCol md='6'>
<MDBInput wrapperClass='mb-4' label='Bussines Arena' size='lg' id='form4' type='text'/>
</MDBCol>
<MDBCol md='6'>
<MDBSelect
className='mb-4'
size='lg'
data={[
{ text: 'Employees', value: 1 },
{ text: 'Two', value: 2 },
{ text: 'Three', value: 3 },
{ text: 'Four', value: 4 }
]}
/>
</MDBCol>
</MDBRow>
</MDBCol>
<MDBCol md='6' className='bg-indigo p-5'>
<h3 className="fw-normal mb-5 text-white" style={{color: '#4835d4'}}>Contact Details</h3>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Street + Nr' size='lg' id='form5' type='text'/>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Additional Information' size='lg' id='form6' type='text'/>
<MDBRow>
<MDBCol md='5'>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Zip Code' size='lg' id='form6' type='text'/>
</MDBCol>
<MDBCol md='7'>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Place' size='lg' id='form7' type='text'/>
</MDBCol>
</MDBRow>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Country' size='lg' id='form8' type='text'/>
<MDBRow>
<MDBCol md='5'>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Code +' size='lg' id='form9' type='text'/>
</MDBCol>
<MDBCol md='7'>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Phone Number' size='lg' id='form10' type='text'/>
</MDBCol>
</MDBRow>
<MDBInput wrapperClass='mb-4' labelClass='text-white' label='Your Email' size='lg' id='form8' type='email'/>
<MDBCheckbox name='flexCheck' id='flexCheckDefault' labelClass='text-white mb-4' label='I do accept the Terms and Conditions of your site.' />
<MDBBtn color='light' size='lg'>Register</MDBBtn>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
);
}
export default App;
body {
/* fallback for old browsers */
background: #a1c4fd;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(161, 196, 253, 1), rgba(194, 233, 251, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(161, 196, 253, 1), rgba(194, 233, 251, 1))
}
.bg-indigo {
background-color: #4835d4;
}
@media (min-width: 1025px) {
.h-custom {
height: 100vh !important;
}
}
@media (min-width: 759px) {
.card-registration-2 .bg-indigo {
border-top-right-radius: 15px;
border-bottom-right-radius: 15px;
}
}
@media (max-width: 758px) {
.card-registration-2 .bg-indigo {
border-bottom-left-radius: 15px;
border-bottom-right-radius: 15px;
}
}
@media (min-width: 759px) {
.card-registration-2 .bg-white {
border-top-left-radius: 15px;
border-bottom-left-radius: 15px;
}
}
@media (max-width: 758px) {
.card-registration-2 .bg-white {
border-top-left-radius: 15px;
border-top-right-radius: 15px;
}
}
Registration modal
This example is a simple registration popup. Check out our modals documentation, to learn how to show the modal, customize modal size and styles.
Create an account
import React from 'react';
import {
MDBBtn,
MDBContainer,
MDBCard,
MDBCardBody,
MDBInput,
MDBCheckbox
}
from 'mdb-react-ui-kit';
function App() {
return (
<MDBContainer fluid className='d-flex align-items-center justify-content-center bg-image' style={{backgroundImage: 'url(https://mdbcdn.b-cdn.net/img/Photos/new-templates/search-box/img4.webp)'}}>
<div className='mask gradient-custom-3'></div>
<MDBCard className='m-5' style={{maxWidth: '600px'}}>
<MDBCardBody className='px-5'>
<h2 className="text-uppercase text-center mb-5">Create an account</h2>
<MDBInput wrapperClass='mb-4' label='Your Name' size='lg' id='form1' type='text'/>
<MDBInput wrapperClass='mb-4' label='Your Email' size='lg' id='form2' type='email'/>
<MDBInput wrapperClass='mb-4' label='Password' size='lg' id='form3' type='password'/>
<MDBInput wrapperClass='mb-4' label='Repeat your password' size='lg' id='form4' type='password'/>
<div className='d-flex flex-row justify-content-center mb-4'>
<MDBCheckbox name='flexCheck' id='flexCheckDefault' label='I agree all statements in Terms of service' />
</div>
<MDBBtn className='mb-4 w-100 gradient-custom-4' size='lg'>Register</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBContainer>
);
}
export default App;
.gradient-custom-3 {
/* fallback for old browsers */
background: #84fab0;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(132, 250, 176, 0.5), rgba(143, 211, 244, 0.5));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(132, 250, 176, 0.5), rgba(143, 211, 244, 0.5))
}
.gradient-custom-4 {
/* fallback for old browsers */
background: #84fab0;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(132, 250, 176, 1), rgba(143, 211, 244, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(132, 250, 176, 1), rgba(143, 211, 244, 1))
}
Registration form with PHP or Node.js
Learn how to create a registration form with PHP using a pre-defined backend template for and Admin Dashboard using PHP and Laravel.
PHP registration TutorialLearn how to create a registration form with Node using a pre-defined backend template for and Admin Dashboard using Node.js and Express.js.
Node registration Tutorial