Shopping carts
React Bootstrap 5 Shopping Carts component
Responsive Shopping Cart & checkout templates built with React Bootstrap 5. Multiple examples of various product summary & payment review designs. Ready to use pages and templates.Basic shopping cart template
A basic shopping card template with purchased products listing using product cards, payment form with credit card inputs fields and an avatar in the upper right corner - indicating that the user is already logged into his profile.
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBInput,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
import React from "react";
export default function Basic() {
return (
<section className="h-100 h-custom" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol>
<MDBCard>
<MDBCardBody className="p-4">
<MDBRow>
<MDBCol lg="7">
<MDBTypography tag="h5">
<a href="#!" className="text-body">
<MDBIcon fas icon="long-arrow-alt-left me-2" /> Continue
shopping
</a>
</MDBTypography>
<hr />
<div className="d-flex justify-content-between align-items-center mb-4">
<div>
<p className="mb-1">Shopping cart</p>
<p className="mb-0">You have 4 items in your cart</p>
</div>
<div>
<p>
<span className="text-muted">Sort by:</span>
<a href="#!" className="text-body">
price
<MDBIcon fas icon="angle-down mt-1" />
</a>
</p>
</div>
</div>
<MDBCard className="mb-3">
<MDBCardBody>
<div className="d-flex justify-content-between">
<div className="d-flex flex-row align-items-center">
<div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img1.webp"
fluid className="rounded-3" style={{ width: "65px" }}
alt="Shopping item" />
</div>
<div className="ms-3">
<MDBTypography tag="h5">
Iphone 11 pro
</MDBTypography>
<p className="small mb-0">256GB, Navy Blue</p>
</div>
</div>
<div className="d-flex flex-row align-items-center">
<div style={{ width: "50px" }}>
<MDBTypography tag="h5" className="fw-normal mb-0">
2
</MDBTypography>
</div>
<div style={{ width: "80px" }}>
<MDBTypography tag="h5" className="mb-0">
$900
</MDBTypography>
</div>
<a href="#!" style={{ color: "#cecece" }}>
<MDBIcon fas icon="trash-alt" />
</a>
</div>
</div>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-3">
<MDBCardBody>
<div className="d-flex justify-content-between">
<div className="d-flex flex-row align-items-center">
<div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img2.webp"
fluid className="rounded-3" style={{ width: "65px" }}
alt="Shopping item" />
</div>
<div className="ms-3">
<MDBTypography tag="h5">
Samsung galaxy Note 10
</MDBTypography>
<p className="small mb-0">256GB, Navy Blue</p>
</div>
</div>
<div className="d-flex flex-row align-items-center">
<div style={{ width: "50px" }}>
<MDBTypography tag="h5" className="fw-normal mb-0">
2
</MDBTypography>
</div>
<div style={{ width: "80px" }}>
<MDBTypography tag="h5" className="mb-0">
$900
</MDBTypography>
</div>
<a href="#!" style={{ color: "#cecece" }}>
<MDBIcon fas icon="trash-alt" />
</a>
</div>
</div>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-3">
<MDBCardBody>
<div className="d-flex justify-content-between">
<div className="d-flex flex-row align-items-center">
<div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img3.webp"
fluid className="rounded-3" style={{ width: "65px" }}
alt="Shopping item" />
</div>
<div className="ms-3">
<MDBTypography tag="h5">
Canon EOS M50
</MDBTypography>
<p className="small mb-0">Onyx Black</p>
</div>
</div>
<div className="d-flex flex-row align-items-center">
<div style={{ width: "50px" }}>
<MDBTypography tag="h5" className="fw-normal mb-0">
1
</MDBTypography>
</div>
<div style={{ width: "80px" }}>
<MDBTypography tag="h5" className="mb-0">
$1199
</MDBTypography>
</div>
<a href="#!" style={{ color: "#cecece" }}>
<MDBIcon fas icon="trash-alt" />
</a>
</div>
</div>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-3">
<MDBCardBody>
<div className="d-flex justify-content-between">
<div className="d-flex flex-row align-items-center">
<div>
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img4.webp"
fluid className="rounded-3" style={{ width: "65px" }}
alt="Shopping item" />
</div>
<div className="ms-3">
<MDBTypography tag="h5">
MacBook Pro
</MDBTypography>
<p className="small mb-0">1TB, Graphite</p>
</div>
</div>
<div className="d-flex flex-row align-items-center">
<div style={{ width: "50px" }}>
<MDBTypography tag="h5" className="fw-normal mb-0">
1
</MDBTypography>
</div>
<div style={{ width: "80px" }}>
<MDBTypography tag="h5" className="mb-0">
$1799
</MDBTypography>
</div>
<a href="#!" style={{ color: "#cecece" }}>
<MDBIcon fas icon="trash-alt" />
</a>
</div>
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol lg="5">
<MDBCard className="bg-primary text-white rounded-3">
<MDBCardBody>
<div className="d-flex justify-content-between align-items-center mb-4">
<MDBTypography tag="h5" className="mb-0">
Card details
</MDBTypography>
<MDBCardImage src="https://mdbcdn.b-cdn.net/img/Photos/Avatars/avatar-6.webp"
fluid className="rounded-3" style={{ width: "45px" }} alt="Avatar" />
</div>
<p className="small">Card type</p>
<a href="#!" type="submit" className="text-white">
<MDBIcon fab icon="cc-mastercard fa-2x me-2" />
</a>
<a href="#!" type="submit" className="text-white">
<MDBIcon fab icon="cc-visa fa-2x me-2" />
</a>
<a href="#!" type="submit" className="text-white">
<MDBIcon fab icon="cc-amex fa-2x me-2" />
</a>
<a href="#!" type="submit" className="text-white">
<MDBIcon fab icon="cc-paypal fa-2x me-2" />
</a>
<form className="mt-4">
<MDBInput className="mb-4" label="Cardholder's Name" type="text" size="lg"
placeholder="Cardholder's Name" contrast />
<MDBInput className="mb-4" label="Card Number" type="text" size="lg"
minLength="19" maxLength="19" placeholder="1234 5678 9012 3457" contrast />
<MDBRow className="mb-4">
<MDBCol md="6">
<MDBInput className="mb-4" label="Expiration" type="text" size="lg"
minLength="7" maxLength="7" placeholder="MM/YYYY" contrast />
</MDBCol>
<MDBCol md="6">
<MDBInput className="mb-4" label="Cvv" type="text" size="lg" minLength="3"
maxLength="3" placeholder="●●●" contrast />
</MDBCol>
</MDBRow>
</form>
<hr />
<div className="d-flex justify-content-between">
<p className="mb-2">Subtotal</p>
<p className="mb-2">$4798.00</p>
</div>
<div className="d-flex justify-content-between">
<p className="mb-2">Shipping</p>
<p className="mb-2">$20.00</p>
</div>
<div className="d-flex justify-content-between">
<p className="mb-2">Total(Incl. taxes)</p>
<p className="mb-2">$4818.00</p>
</div>
<MDBBtn color="info" block size="lg">
<div className="d-flex justify-content-between">
<span>$4818.00</span>
<span>
Checkout{" "}
<i className="fas fa-long-arrow-alt-right ms-2"></i>
</span>
</div>
</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
@media (min-width: 1025px) {
.h-custom {
height: 100vh !important;
}
}
Shopping cart template with quantity edit
In the example below the user can change the quantity of purchased products using the numeric input. This template is equipped with a register button - for cases in which the user needs to go trough new account registration or log into an existing account before he completes the purchase. You can of course replace this with a "Next step" button leading straight to the payment step.
Shopping Cart
3 items
Summary
items 3
€ 132.00
Shipping
Give code
Total price
€ 137.00
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCardText,
MDBCol,
MDBContainer,
MDBIcon,
MDBInput,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
import React from "react";
export default function QuantityEdit() {
return (
<section className="h-100 h-custom" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol size="12">
<MDBCard className="card-registration card-registration-2" style={{ borderRadius: "15px" }}>
<MDBCardBody className="p-0">
<MDBRow className="g-0">
<MDBCol lg="8">
<div className="p-5">
<div className="d-flex justify-content-between align-items-center mb-5">
<MDBTypography tag="h1" className="fw-bold mb-0 text-black">
Shopping Cart
</MDBTypography>
<MDBTypography className="mb-0 text-muted">
3 items
</MDBTypography>
</div>
<hr className="my-4" />
<MDBRow className="mb-4 d-flex justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img5.webp"
fluid className="rounded-3" alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<MDBTypography tag="h6" className="text-muted">
Shirt
</MDBTypography>
<MDBTypography tag="h6" className="text-black mb-0">
Cotton T-shirt
</MDBTypography>
</MDBCol>
<MDBCol md="3" lg="3" xl="3" className="d-flex align-items-center">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput type="number" min="0" defaultValue={1} size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="text-end">
<MDBTypography tag="h6" className="mb-0">
€ 44.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-muted">
<MDBIcon fas icon="times" />
</a>
</MDBCol>
</MDBRow>
<hr className="my-4" />
<MDBRow className="mb-4 d-flex justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img6.webp"
fluid className="rounded-3" alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<MDBTypography tag="h6" className="text-muted">
Shirt
</MDBTypography>
<MDBTypography tag="h6" className="text-black mb-0">
Cotton T-shirt
</MDBTypography>
</MDBCol>
<MDBCol md="3" lg="3" xl="3" className="d-flex align-items-center">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput type="number" min="0" defaultValue={1} size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="text-end">
<MDBTypography tag="h6" className="mb-0">
€ 44.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-muted">
<MDBIcon fas icon="times" />
</a>
</MDBCol>
</MDBRow>
<hr className="my-4" />
<MDBRow className="mb-4 d-flex justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img7.webp"
fluid className="rounded-3" alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<MDBTypography tag="h6" className="text-muted">
Shirt
</MDBTypography>
<MDBTypography tag="h6" className="text-black mb-0">
Cotton T-shirt
</MDBTypography>
</MDBCol>
<MDBCol md="3" lg="3" xl="3" className="d-flex align-items-center">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput type="number" min="0" defaultValue={1} size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="text-end">
<MDBTypography tag="h6" className="mb-0">
€ 44.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-muted">
<MDBIcon fas icon="times" />
</a>
</MDBCol>
</MDBRow>
<hr className="my-4" />
<div className="pt-5">
<MDBTypography tag="h6" className="mb-0">
<MDBCardText tag="a" href="#!" className="text-body">
<MDBIcon fas icon="long-arrow-alt-left me-2" /> Back
to shop
</MDBCardText>
</MDBTypography>
</div>
</div>
</MDBCol>
<MDBCol lg="4" className="bg-grey">
<div className="p-5">
<MDBTypography tag="h3" className="fw-bold mb-5 mt-2 pt-1">
Summary
</MDBTypography>
<hr className="my-4" />
<div className="d-flex justify-content-between mb-4">
<MDBTypography tag="h5" className="text-uppercase">
items 3
</MDBTypography>
<MDBTypography tag="h5">€ 132.00</MDBTypography>
</div>
<MDBTypography tag="h5" className="text-uppercase mb-3">
Shipping
</MDBTypography>
<div className="mb-4 pb-2">
<select className="select p-2 rounded bg-grey" style={{ width: "100%" }}>
<option value="1">Standard-Delivery- €5.00</option>
<option value="2">Two</option>
<option value="3">Three</option>
<option value="4">Four</option>
</select>
</div>
<MDBTypography tag="h5" className="text-uppercase mb-3">
Give code
</MDBTypography>
<div className="mb-5">
<MDBInput size="lg" label="Enter your code" />
</div>
<hr className="my-4" />
<div className="d-flex justify-content-between mb-5">
<MDBTypography tag="h5" className="text-uppercase">
Total price
</MDBTypography>
<MDBTypography tag="h5">€ 137.00</MDBTypography>
</div>
<MDBBtn color="dark" block size="lg">
Register
</MDBBtn>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
@media (min-width: 1025px) {
.h-custom {
height: 100vh !important;
}
}
.card-registration .select-input.form-control[readonly]:not([disabled]) {
font-size: 1rem;
line-height: 2.15;
padding-left: .75em;
padding-right: .75em;
}
.card-registration .select-arrow {
top: 13px;
}
.bg-grey {
background-color: #eae8e8;
}
@media (min-width: 992px) {
.card-registration-2 .bg-grey {
border-top-right-radius: 16px;
border-bottom-right-radius: 16px;
}
}
@media (max-width: 991px) {
.card-registration-2 .bg-grey {
border-bottom-left-radius: 16px;
border-bottom-right-radius: 16px;
}
}
Shopping cart page with payment methods
The example below is based on a subtle gradient background and includes a card with accepted credit card providers.
Cart - 2 items
Blue denim shirt
Color: blue
Size: M
$17.99
Red hoodie
Color: red
Size: M
$17.99
Expected shipping delivery
12.10.2020 - 14.10.2020
We accept

Summary
- Products $53.98
- Shipping Gratis
-
Total amount$53.98
(including VAT)
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardHeader,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBInput,
MDBListGroup,
MDBListGroupItem,
MDBRipple,
MDBRow,
MDBTooltip,
MDBTypography,
} from "mdb-react-ui-kit";
import React from "react";
export default function PaymentMethods() {
return (
<section className="h-100 gradient-custom">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center my-4">
<MDBCol md="8">
<MDBCard className="mb-4">
<MDBCardHeader className="py-3">
<MDBTypography tag="h5" className="mb-0">
Cart - 2 items
</MDBTypography>
</MDBCardHeader>
<MDBCardBody>
<MDBRow>
<MDBCol lg="3" md="12" className="mb-4 mb-lg-0">
<MDBRipple rippleTag="div" rippleColor="light"
className="bg-image rounded hover-zoom hover-overlay">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Vertical/12a.webp"
className="w-100" />
<a href="#!">
<div className="mask" style={{ backgroundColor: "rgba(251, 251, 251, 0.2)" , }}>
</div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol lg="5" md="6" className=" mb-4 mb-lg-0">
<p>
<strong>Blue denim shirt</strong>
</p>
<p>Color: blue</p>
<p>Size: M</p>
<MDBTooltip wrapperProps={{ size: "sm" }} wrapperClass="me-1 mb-2"
title="Remove item">
<MDBIcon fas icon="trash" />
</MDBTooltip>
<MDBTooltip wrapperProps={{ size: "sm" , color: "danger" }} wrapperClass="me-1 mb-2"
title="Move to the wish list">
<MDBIcon fas icon="heart" />
</MDBTooltip>
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4 mb-lg-0">
<div className="d-flex mb-4" style={{ maxWidth: "300px" }}>
<MDBBtn className="px-3 me-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput defaultValue={1} min={0} type="number" label="Quantity" />
<MDBBtn className="px-3 ms-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</div>
<p className="text-start text-md-center">
<strong>$17.99</strong>
</p>
</MDBCol>
</MDBRow>
<hr className="my-4" />
<MDBRow>
<MDBCol lg="3" md="12" className="mb-4 mb-lg-0">
<MDBRipple rippleTag="div" rippleColor="light"
className="bg-image rounded hover-zoom hover-overlay">
<img
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Vertical/13a.webp"
className="w-100" />
<a href="#!">
<div className="mask" style={{ backgroundColor: "rgba(251, 251, 251, 0.2)" , }}>
</div>
</a>
</MDBRipple>
</MDBCol>
<MDBCol lg="5" md="6" className=" mb-4 mb-lg-0">
<p>
<strong>Red hoodie</strong>
</p>
<p>Color: red</p>
<p>Size: M</p>
<MDBTooltip wrapperProps={{ size: "sm" }} wrapperClass="me-1 mb-2"
title="Remove item">
<MDBIcon fas icon="trash" />
</MDBTooltip>
<MDBTooltip wrapperProps={{ size: "sm" , color: "danger" }} wrapperClass="me-1 mb-2"
title="Move to the wish list">
<MDBIcon fas icon="heart" />
</MDBTooltip>
</MDBCol>
<MDBCol lg="4" md="6" className="mb-4 mb-lg-0">
<div className="d-flex mb-4" style={{ maxWidth: "300px" }}>
<MDBBtn className="px-3 me-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput defaultValue={1} min={0} type="number" label="Quantity" />
<MDBBtn className="px-3 ms-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</div>
<p className="text-start text-md-center">
<strong>$17.99</strong>
</p>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-4">
<MDBCardBody>
<p>
<strong>Expected shipping delivery</strong>
</p>
<p className="mb-0">12.10.2020 - 14.10.2020</p>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-4 mb-lg-0">
<MDBCardBody>
<p>
<strong>We accept</strong>
</p>
<MDBCardImage className="me-2" width="45px"
src="https://mdbcdn.b-cdn.net/wp-content/plugins/woocommerce-gateway-stripe/assets/images/visa.svg"
alt="Visa" />
<MDBCardImage className="me-2" width="45px"
src="https://mdbcdn.b-cdn.net/wp-content/plugins/woocommerce-gateway-stripe/assets/images/amex.svg"
alt="American Express" />
<MDBCardImage className="me-2" width="45px"
src="https://mdbcdn.b-cdn.net/wp-content/plugins/woocommerce-gateway-stripe/assets/images/mastercard.svg"
alt="Mastercard" />
<MDBCardImage className="me-2" width="45px"
src="https://mdbcdn.b-cdn.net/wp-content/plugins/woocommerce/includes/gateways/paypal/assets/images/paypal.png"
alt="PayPal acceptance mark" />
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md="4">
<MDBCard className="mb-4">
<MDBCardHeader>
<MDBTypography tag="h5" className="mb-0">
Summary
</MDBTypography>
</MDBCardHeader>
<MDBCardBody>
<MDBListGroup flush>
<MDBListGroupItem
className="d-flex justify-content-between align-items-center border-0 px-0 pb-0">
Products
<span>$53.98</span>
</MDBListGroupItem>
<MDBListGroupItem className="d-flex justify-content-between align-items-center px-0">
Shipping
<span>Gratis</span>
</MDBListGroupItem>
<MDBListGroupItem
className="d-flex justify-content-between align-items-center border-0 px-0 mb-3">
<div>
<strong>Total amount</strong>
<strong>
<p className="mb-0">(including VAT)</p>
</strong>
</div>
<span>
<strong>$53.98</strong>
</span>
</MDBListGroupItem>
</MDBListGroup>
<MDBBtn block size="lg">
Go to checkout
</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
.gradient-custom {
/* fallback for old browsers */
background: #6a11cb;
/* Chrome 10-25, Safari 5.1-6 */
background: -webkit-linear-gradient(to right, rgba(106, 17, 203, 1), rgba(37, 117, 252, 1));
/* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */
background: linear-gradient(to right, rgba(106, 17, 203, 1), rgba(37, 117, 252, 1))
}
Shopping cart with product cards
The shopping cart page template below includes a list of purchased products created with product cards with an delete icon button, as well as an input field for the discount code.
import React from "react";
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBInput,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function ProductCards() {
return (
<section className="h-100" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol md="10">
<div className="d-flex justify-content-between align-items-center mb-4">
<MDBTypography tag="h3" className="fw-normal mb-0 text-black">
Shopping Cart
</MDBTypography>
<div>
<p className="mb-0">
<span className="text-muted">Sort by:</span>
<a href="#!" className="text-body">
price <i className="fas fa-angle-down mt-1"></i>
</a>
</p>
</div>
</div>
<MDBCard className="rounded-3 mb-4">
<MDBCardBody className="p-4">
<MDBRow className="justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage className="rounded-3" fluid
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img1.webp"
alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<p className="lead fw-normal mb-2">Basic T-shirt</p>
<p>
<span className="text-muted">Size: </span>M{" "}
<span className="text-muted">Color: </span>Grey
</p>
</MDBCol>
<MDBCol md="3" lg="3" xl="2"
className="d-flex align-items-center justify-content-around">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput min={0} defaultValue={2} type="number" size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="offset-lg-1">
<MDBTypography tag="h5" className="mb-0">
$499.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-danger">
<MDBIcon fas icon="trash text-danger" size="lg" />
</a>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="rounded-3 mb-4">
<MDBCardBody className="p-4">
<MDBRow className="justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage className="rounded-3" fluid
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img1.webp"
alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<p className="lead fw-normal mb-2">Basic T-shirt</p>
<p>
<span className="text-muted">Size: </span>M{" "}
<span className="text-muted">Color: </span>Grey
</p>
</MDBCol>
<MDBCol md="3" lg="3" xl="2"
className="d-flex align-items-center justify-content-around">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput min={0} defaultValue={2} type="number" size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="offset-lg-1">
<MDBTypography tag="h5" className="mb-0">
$499.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-danger">
<MDBIcon fas icon="trash text-danger" size="lg" />
</a>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="rounded-3 mb-4">
<MDBCardBody className="p-4">
<MDBRow className="justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage className="rounded-3" fluid
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img1.webp"
alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<p className="lead fw-normal mb-2">Basic T-shirt</p>
<p>
<span className="text-muted">Size: </span>M{" "}
<span className="text-muted">Color: </span>Grey
</p>
</MDBCol>
<MDBCol md="3" lg="3" xl="2"
className="d-flex align-items-center justify-content-around">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput min={0} defaultValue={2} type="number" size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="offset-lg-1">
<MDBTypography tag="h5" className="mb-0">
$499.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-danger">
<MDBIcon fas icon="trash text-danger" size="lg" />
</a>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="rounded-3 mb-4">
<MDBCardBody className="p-4">
<MDBRow className="justify-content-between align-items-center">
<MDBCol md="2" lg="2" xl="2">
<MDBCardImage className="rounded-3" fluid
src="https://mdbcdn.b-cdn.net/img/Photos/new-templates/bootstrap-shopping-carts/img1.webp"
alt="Cotton T-shirt" />
</MDBCol>
<MDBCol md="3" lg="3" xl="3">
<p className="lead fw-normal mb-2">Basic T-shirt</p>
<p>
<span className="text-muted">Size: </span>M{" "}
<span className="text-muted">Color: </span>Grey
</p>
</MDBCol>
<MDBCol md="3" lg="3" xl="2"
className="d-flex align-items-center justify-content-around">
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput min={0} defaultValue={2} type="number" size="sm" />
<MDBBtn color="link" className="px-2">
<MDBIcon fas icon="plus" />
</MDBBtn>
</MDBCol>
<MDBCol md="3" lg="2" xl="2" className="offset-lg-1">
<MDBTypography tag="h5" className="mb-0">
$499.00
</MDBTypography>
</MDBCol>
<MDBCol md="1" lg="1" xl="1" className="text-end">
<a href="#!" className="text-danger">
<MDBIcon fas icon="trash text-danger" size="lg" />
</a>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-4">
<MDBCardBody className="p-4 d-flex flex-row">
<MDBInput label="Discound code" wrapperClass="flex-fill" size="lg" />
<MDBBtn className="ms-3" color="warning" outline size="lg">
Apply
</MDBBtn>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardBody>
<MDBBtn className="ms-3" color="warning" block size="lg">
Apply
</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Shopping cart checkout
The example below combines a shopping cart and a checkout in a single template. You can both edit your purchased products as well as credit card forms for setting up the payment.
import React from "react";
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBInput,
MDBRow,
MDBTypography,
} from "mdb-react-ui-kit";
export default function CartCheckout() {
return (
<section className="h-100 h-custom" style={{ backgroundColor: "#eee" }}>
<MDBContainer className="h-100 py-5">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol>
<MDBCard className="shopping-cart" style={{ borderRadius: "15px" }}>
<MDBCardBody className="text-black">
<MDBRow>
<MDBCol lg="7" className="px-5 py-4">
<MDBTypography
tag="h3"
className="mb-5 pt-2 text-center fw-bold text-uppercase"
>
Your products
</MDBTypography>
<div className="d-flex align-items-center mb-5">
<div className="flex-shrink-0">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/13.webp"
fluid
style={{ width: "150px" }}
alt="Generic placeholder image"
/>
</div>
<div className="flex-grow-1 ms-3">
<a href="#!" className="float-end text-black">
<MDBIcon fas icon="times" />
</a>
<MDBTypography tag="h5" className="text-primary">
Samsung Galaxy M11 64GB
</MDBTypography>
<MDBTypography tag="h6" style={{ color: "#9e9e9e" }}>
Color: white
</MDBTypography>
<div className="d-flex align-items-center">
<p className="fw-bold mb-0 me-5 pe-3">799$</p>
<div className="def-number-input number-input safari_only">
<button className="minus"></button>
<input
className="quantity fw-bold text-black"
min={0}
defaultValue={1}
type="number"
/>
<button className="plus"></button>
</div>
</div>
</div>
</div>
<div className="d-flex align-items-center mb-5">
<div className="flex-shrink-0">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/6.webp"
fluid
style={{ width: "150px" }}
alt="Generic placeholder image"
/>
</div>
<div className="flex-grow-1 ms-3">
<a href="#!" className="float-end text-black">
<MDBIcon fas icon="times" />
</a>
<MDBTypography tag="h5" className="text-primary">
Headphones Bose 35 II
</MDBTypography>
<MDBTypography tag="h6" style={{ color: "#9e9e9e" }}>
Color: red
</MDBTypography>
<div className="d-flex align-items-center">
<p className="fw-bold mb-0 me-5 pe-3">239$</p>
<div className="def-number-input number-input safari_only">
<button className="minus"></button>
<input
className="quantity fw-bold text-black"
min={0}
defaultValue={1}
type="number"
/>
<button className="plus"></button>
</div>
</div>
</div>
</div>
<div className="d-flex align-items-center mb-5">
<div className="flex-shrink-0">
<MDBCardImage
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/1.webp"
fluid
style={{ width: "150px" }}
alt="Generic placeholder image"
/>
</div>
<div className="flex-grow-1 ms-3">
<a href="#!" className="float-end text-black">
<MDBIcon fas icon="times" />
</a>
<MDBTypography tag="h5" className="text-primary">
iPad 9.7 6-gen WiFi 32GB
</MDBTypography>
<MDBTypography tag="h6" style={{ color: "#9e9e9e" }}>
Color: rose pink
</MDBTypography>
<div className="d-flex align-items-center">
<p className="fw-bold mb-0 me-5 pe-3">659$</p>
<div className="def-number-input number-input safari_only">
<button className="minus"></button>
<input
className="quantity fw-bold text-black"
min={0}
defaultValue={2}
type="number"
/>
<button className="plus"></button>
</div>
</div>
</div>
</div>
<hr
className="mb-4"
style={{
height: "2px",
backgroundColor: "#1266f1",
opacity: 1,
}}
/>
<div className="d-flex justify-content-between px-x">
<p className="fw-bold">Discount:</p>
<p className="fw-bold">95$</p>
</div>
<div
className="d-flex justify-content-between p-2 mb-2"
style={{ backgroundColor: "#e1f5fe" }}
>
<MDBTypography tag="h5" className="fw-bold mb-0">
Total:
</MDBTypography>
<MDBTypography tag="h5" className="fw-bold mb-0">
2261$
</MDBTypography>
</div>
</MDBCol>
<MDBCol lg="5" className="px-5 py-4">
<MDBTypography
tag="h3"
className="mb-5 pt-2 text-center fw-bold text-uppercase"
>
Payment
</MDBTypography>
<form className="mb-5">
<MDBInput
className="mb-5"
label="Card number"
type="text"
size="lg"
defaultValue="1234 5678 9012 3457"
/>
<MDBInput
className="mb-5"
label="Name on card"
type="text"
size="lg"
defaultValue="John Smith"
/>
<MDBRow>
<MDBCol md="6" className="mb-5">
<MDBInput
className="mb-4"
label="Expiration"
type="text"
size="lg"
minLength="7"
maxLength="7"
defaultValue="01/22"
placeholder="MM/YYYY"
/>
</MDBCol>
<MDBCol md="6" className="mb-5">
<MDBInput
className="mb-4"
label="Cvv"
type="text"
size="lg"
minLength="3"
maxLength="3"
placeholder="●●●"
defaultValue="●●●"
/>
</MDBCol>
</MDBRow>
<p className="mb-5">
Lorem ipsum dolor sit amet consectetur, adipisicing elit
<a href="#!"> obcaecati sapiente</a>.
</p>
<MDBBtn block size="lg">
Buy now
</MDBBtn>
<MDBTypography
tag="h5"
className="fw-bold mb-5"
style={{ position: "absolute", bottom: "0" }}
>
<a href="#!">
<MDBIcon fas icon="angle-left me-2" />
Back to shopping
</a>
</MDBTypography>
</form>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
@media (min-width: 1025px) {
.h-custom {
height: 100vh !important;
}
}
.number-input input[type="number"] {
-webkit-appearance: textfield;
-moz-appearance: textfield;
appearance: textfield;
}
.number-input input[type=number]::-webkit-inner-spin-button,
.number-input input[type=number]::-webkit-outer-spin-button {
-webkit-appearance: none;
}
.number-input button {
-webkit-appearance: none;
background-color: transparent;
border: none;
align-items: center;
justify-content: center;
cursor: pointer;
margin: 0;
position: relative;
}
.number-input button:before,
.number-input button:after {
display: inline-block;
position: absolute;
content: '';
height: 2px;
transform: translate(-50%, -50%);
}
.number-input button.plus:after {
transform: translate(-50%, -50%) rotate(90deg);
}
.number-input input[type=number] {
text-align: center;
}
.number-input.number-input {
border: 1px solid #ced4da;
width: 10rem;
border-radius: .25rem;
}
.number-input.number-input button {
width: 2.6rem;
height: .7rem;
}
.number-input.number-input button.minus {
padding-left: 10px;
}
.number-input.number-input button:before,
.number-input.number-input button:after {
width: .7rem;
background-color: #495057;
}
.number-input.number-input input[type=number] {
max-width: 4rem;
padding: .5rem;
border: 1px solid #ced4da;
border-width: 0 1px;
font-size: 1rem;
height: 2rem;
color: #495057;
}
@media not all and (min-resolution:.001dpcm) {
@supports (-webkit-appearance: none) and (stroke-color:transparent) {
.number-input.def-number-input.safari_only button:before,
.number-input.def-number-input.safari_only button:after {
margin-top: -.3rem;
}
}
}
.shopping-cart .def-number-input.number-input {
border: none;
}
.shopping-cart .def-number-input.number-input input[type=number] {
max-width: 2rem;
border: none;
}
.shopping-cart .def-number-input.number-input input[type=number].black-text,
.shopping-cart .def-number-input.number-input input.btn.btn-link[type=number],
.shopping-cart .def-number-input.number-input input.md-toast-close-button[type=number]:hover,
.shopping-cart .def-number-input.number-input input.md-toast-close-button[type=number]:focus {
color: #212529 !important;
}
.shopping-cart .def-number-input.number-input button {
width: 1rem;
}
.shopping-cart .def-number-input.number-input button:before,
.shopping-cart .def-number-input.number-input button:after {
width: .5rem;
}
.shopping-cart .def-number-input.number-input button.minus:before,
.shopping-cart .def-number-input.number-input button.minus:after {
background-color: #9e9e9e;
}
.shopping-cart .def-number-input.number-input button.plus:before,
.shopping-cart .def-number-input.number-input button.plus:after {
background-color: #4285f4;
}
Shopping cart review page
This shopping cart example includes an additional column for the final review of the product color.
Shopping Cart (1 item in your cart)
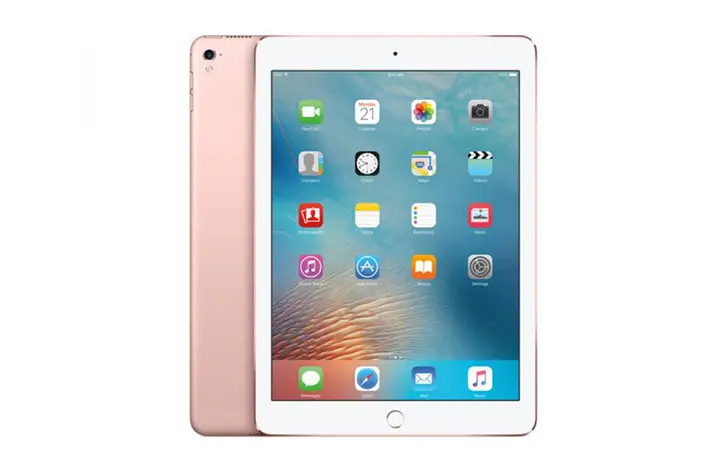
Name
iPad Air
Color
pink rose
Quantity
1
Price
$799
Total
$799
Order total: $799
import React from "react";
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCardImage,
MDBCol,
MDBContainer,
MDBIcon,
MDBRow,
} from "mdb-react-ui-kit";
export default function ReviewPage() {
return (
<section className="vh-100" style={{ backgroundColor: "#fdccbc" }}>
<MDBContainer className="h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol>
<p>
<span className="h2">Shopping Cart </span>
<span className="h4">(1 item in your cart)</span>
</p>
<MDBCard className="mb-4">
<MDBCardBody className="p-4">
<MDBRow className="align-items-center">
<MDBCol md="2">
<MDBCardImage
fluid
src="https://mdbcdn.b-cdn.net/img/Photos/Horizontal/E-commerce/Products/1.webp"
alt="Generic placeholder image"
/>
</MDBCol>
<MDBCol md="2" className="d-flex justify-content-center">
<div>
<p className="small text-muted mb-4 pb-2">Name</p>
<p className="lead fw-normal mb-0">iPad Air</p>
</div>
</MDBCol>
<MDBCol md="2" className="d-flex justify-content-center">
<div>
<p className="small text-muted mb-4 pb-2">Color</p>
<p className="lead fw-normal mb-0">
<MDBIcon
fas
icon="circle me-2"
style={{ color: "#fdd8d2" }}
/>
pink rose
</p>
</div>
</MDBCol>
<MDBCol md="2" className="d-flex justify-content-center">
<div>
<p className="small text-muted mb-4 pb-2">Quantity</p>
<p className="lead fw-normal mb-0">1</p>
</div>
</MDBCol>
<MDBCol md="2" className="d-flex justify-content-center">
<div>
<p className="small text-muted mb-4 pb-2">Price</p>
<p className="lead fw-normal mb-0">$799</p>
</div>
</MDBCol>
<MDBCol md="2" className="d-flex justify-content-center">
<div>
<p className="small text-muted mb-4 pb-2">Total</p>
<p className="lead fw-normal mb-0">$799</p>
</div>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
<MDBCard className="mb-5">
<MDBCardBody className="p-4">
<div className="float-end">
<p className="mb-0 me-5 d-flex align-items-center">
<span className="small text-muted me-2">Order total:</span>
<span className="lead fw-normal">$799</span>
</p>
</div>
</MDBCardBody>
</MDBCard>
<div className="d-flex justify-content-end">
<MDBBtn color="light" size="lg" className="me-2">
Continue shopping
</MDBBtn>
<MDBBtn size="lg">Add to cart</MDBBtn>
</div>
</MDBCol>
</MDBRow>
</MDBContainer>
</section>
);
}
Shopping cart summary page
Another template combining both shopping cart and checkout on a single page providing an extensive summary or purchased products, subtotals, shipping cost and total price.
Shopping Bag | Format | Quantity | Price |
---|---|---|---|
![]() Thinking, Fast and Slow Daniel Kahneman |
Digital |
|
$9.99 |
![]() Homo Deus: A Brief History of Tomorrow Yuval Noah Harari |
Paperback |
|
$13.50 |
Subtotal
$23.49
Shipping
$2.99
Total (tax included)
$26.48
import React from "react";
import {
MDBBtn,
MDBCard,
MDBCardBody,
MDBCol,
MDBContainer,
MDBIcon,
MDBInput,
MDBRadio,
MDBRow,
MDBTable,
MDBTableBody,
MDBTableHead,
} from "mdb-react-ui-kit";
export default function SummaryPage() {
return (
<section className="h-100 h-custom">
<MDBContainer className="py-5 h-100">
<MDBRow className="justify-content-center align-items-center h-100">
<MDBCol>
<MDBTable responsive>
<MDBTableHead>
<tr>
<th scope="col" className="h5">
Shopping Bag
</th>
<th scope="col">Format</th>
<th scope="col">Quantity</th>
<th scope="col">Price</th>
</tr>
</MDBTableHead>
<MDBTableBody>
<tr>
<th scope="row">
<div className="d-flex align-items-center">
<img
src="https://i.imgur.com/2DsA49b.webp"
fluid
className="rounded-3"
style={{ width: "120px" }}
alt="Book"
/>
<div className="flex-column ms-4">
<p className="mb-2">Thinking, Fast and Slow</p>
<p className="mb-0">Daniel Kahneman</p>
</div>
</div>
</th>
<td className="align-middle">
<p className="mb-0" style={{ fontWeight: "500" }}>
Digital
</p>
</td>
<td className="align-middle">
<div class="d-flex flex-row align-items-center">
<MDBBtn className="px-2" color="link">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput
min={0}
type="number"
size="sm"
style={{ width: "50px" }}
defaultValue={2}
/>
<MDBBtn className="px-2" color="link">
<MDBIcon fas icon="plus" />
</MDBBtn>
</div>
</td>
<td className="align-middle">
<p className="mb-0" style={{ fontWeight: "500" }}>
$9.99
</p>
</td>
</tr>
<tr>
<th scope="row">
<div className="d-flex align-items-center">
<img
src="https://i.imgur.com/Oj1iQUX.webp"
fluid
className="rounded-3"
style={{ width: "120px" }}
alt="Book"
/>
<div className="flex-column ms-4">
<p className="mb-2">
Homo Deus: A Brief History of Tomorrow
</p>
<p className="mb-0">Yuval Noah Harari</p>
</div>
</div>
</th>
<td className="align-middle">
<p className="mb-0" style={{ fontWeight: "500" }}>
Paperback
</p>
</td>
<td className="align-middle">
<div class="d-flex flex-row align-items-center">
<MDBBtn className="px-2" color="link">
<MDBIcon fas icon="minus" />
</MDBBtn>
<MDBInput
min={0}
type="number"
size="sm"
style={{ width: "50px" }}
defaultValue={1}
/>
<MDBBtn className="px-2" color="link">
<MDBIcon fas icon="plus" />
</MDBBtn>
</div>
</td>
<td className="align-middle">
<p className="mb-0" style={{ fontWeight: "500" }}>
$13.50
</p>
</td>
</tr>
</MDBTableBody>
</MDBTable>
</MDBCol>
<MDBCard
className="shadow-2-strong mb-5 mb-lg-0"
style={{ borderRadius: "16px" }}
>
<MDBCardBody className="p-4">
<MDBRow>
<MDBCol md="6" lg="4" xl="3" className="mb-4 mb-md-0">
<form>
<div className="d-flex flex-row pb-3">
<div className="d-flex align-items-center pe-2">
<MDBRadio
type="radio"
name="radio1"
checked
value=""
aria-label="..."
/>
</div>
<div className="rounded border w-100 p-3">
<p className="d-flex align-items-center mb-0">
<MDBIcon
fab
icon="cc-mastercard fa-2x text-dark pe-2"
/>
Credit Card
</p>
</div>
</div>
<div className="d-flex flex-row pb-3">
<div className="d-flex align-items-center pe-2">
<MDBRadio
type="radio"
name="radio1"
checked
value=""
aria-label="..."
/>
</div>
<div className="rounded border w-100 p-3">
<p className="d-flex align-items-center mb-0">
<MDBIcon fab icon="cc-visa fa-2x text-dark pe-2" />
Debit Card
</p>
</div>
</div>
<div className="d-flex flex-row pb-3">
<div className="d-flex align-items-center pe-2">
<MDBRadio
type="radio"
name="radio1"
checked
value=""
aria-label="..."
/>
</div>
<div className="rounded border w-100 p-3">
<p className="d-flex align-items-center mb-0">
<MDBIcon fab icon="cc-paypal fa-2x text-dark pe-2" />
PayPal
</p>
</div>
</div>
</form>
</MDBCol>
<MDBCol md="6" lg="4" xl="6">
<MDBRow>
<MDBCol size="12" xl="6">
<MDBInput
className="mb-4 mb-xl-5"
label="Name on card"
placeholder="John Smiths"
size="lg"
/>
<MDBInput
className="mb-4 mb-xl-5"
label="Expiration"
placeholder="MM/YY"
size="lg"
maxLength={7}
minLength={7}
/>
</MDBCol>
<MDBCol size="12" xl="6">
<MDBInput
className="mb-4 mb-xl-5"
label="Card Number"
placeholder="1111 2222 3333 4444"
size="lg"
minlength="19"
maxlength="19"
/>
<MDBInput
className="mb-4 mb-xl-5"
label="Cvv"
placeholder="●●●"
size="lg"
minlength="3"
maxlength="3"
type="password"
/>
</MDBCol>
</MDBRow>
</MDBCol>
<MDBCol lg="4" xl="3">
<div
className="d-flex justify-content-between"
style={{ fontWeight: "500" }}
>
<p className="mb-2">Subtotal</p>
<p className="mb-2">$23.49</p>
</div>
<div
className="d-flex justify-content-between"
style={{ fontWeight: "500" }}
>
<p className="mb-0">Shipping</p>
<p className="mb-0">$2.99</p>
</div>
<hr className="my-4" />
<div
className="d-flex justify-content-between mb-4"
style={{ fontWeight: "500" }}
>
<p className="mb-2">Total (tax included)</p>
<p className="mb-2">$26.48</p>
</div>
<MDBBtn block size="lg">
<div className="d-flex justify-content-between">
<span>Checkout</span>
<span>$26.48</span>
</div>
</MDBBtn>
</MDBCol>
</MDBRow>
</MDBCardBody>
</MDBCard>
</MDBRow>
</MDBContainer>
</section>
);
}
@media (min-width: 1025px) {
.h-custom {
height: 100vh !important;
}
}