How to align an image in html
Vertically aligning an image in HTML can enhance the visual structure of your web page and make your content more appealing. There are several methods to achieve vertical alignment, each offering flexibility and control depending on your needs. In this article, we'll explore different techniques to vertically align images in HTML using CSS, Flexbox, and CSS Grid.
Using Bootstrap
Bootstrap is a popular front-end framework that makes it easy to align elements, including images.
- Use Bootstrap's
d-flex
class to create a flex container. - Use
justify-content-center
to center the image horizontally. - Use
align-items-center
to center the image vertically. - Set the container height to
30vh
to center the image within the viewport.
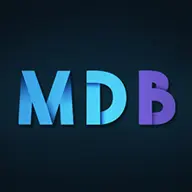
<div class="d-flex justify-content-center align-items-center" style="height: 30vh;">
<img src="path/to/your/image.jpg" alt="Centered Image">
</div>
Using CSS Flexbox
Flexbox is a modern CSS layout module that provides a straightforward way to vertically align elements.
- Create a
flex-container
withdisplay: flex;
. - Use
justify-content: center;
to center the image horizontally. - Use
align-items: center;
to center the image vertically. - Set the container height to
30vh
to center the image within the viewport.
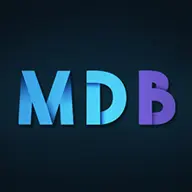
<div class="flex-container">
<img src="path/to/your/image.jpg" alt="Centered Image">
</div>
.flex-container {
display: flex;
justify-content: center;
align-items: center;
height: 30vh;
}
Using CSS Grid
CSS Grid is another powerful layout system that can be used to vertically align images.
- Create a
grid-container
withdisplay: grid;
. - Use
place-items: center;
to center the image both horizontally and vertically. - Set the container height to
30vh
to center the image within the viewport.
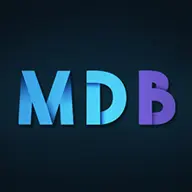
<div class="grid-container">
<img src="path/to/your/image.jpg" alt="Centered Image">
</div>
.grid-container {
display: grid;
place-items: center;
height: 30vh;
}
Using Inline Styles
Inline styles allow you to apply CSS directly to the HTML element for quick and specific customization.
- The
style
attribute applies CSS directly to thediv
element. - Using
display: flex;
,justify-content: center;
, andalign-items: center;
centers the image both horizontally and vertically. - Setting the container height to
30vh
ensures the image is centered within the viewport.
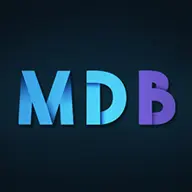
<div style="display: flex; justify-content: center; align-items: center; height: 30vh;">
<img src="path/to/your/image.jpg" alt="Centered Image">
</div>