Touch
Bootstrap 5 Touch
This component enables you to enhance the user experience on mobile touch screens by invoking methods on the following custom events: pinch, swipe, tap, press, pan, and rotate.
Note: Read the API tab to find all available options and advanced customization
Note: This method is intended only for users of mobile touch screens, so it won't work for the mouse or keyboard events.
*
*
UMD autoinits are enabled
by default. This means that you don't need to initialize
the component manually. However if you are using MDBootstrap ES format then you should pass
the required components to the initMDB
method.
Press
Press calls the chosen method when the touch event on the element lasts longer than 250 milliseconds.
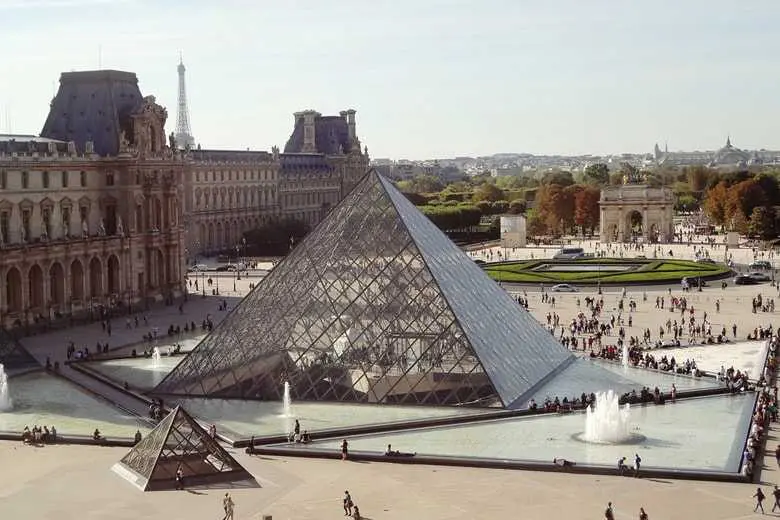
Hold the button to remove the mask from the image
<div>
<div class="bg-image">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" />
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)" id="remove-bg">
<div class="d-flex justify-content-center align-items-center h-100">
<p id="press-text" class="text-white mb-0"> Hold the button to remove the mask from the image</p>
</div>
</div>
</div>
<div class="my-3">
<button
type="button"
class="btn btn-primary btn-press"
data-mdb-ripple-init
data-mdb-touch-init
data-mdb-event="press"
data-mdb-time="2000">
Tap & hold to show image
</button>
</div>
</div>
import { Ripple, Touch, initMDB } from "mdb-ui-kit";
initMDB({ Ripple, Touch });
const btnPress = document.querySelector('.btn-press');
const removeBg = document.querySelector('#remove-bg');
const pressText = document.querySelector('#press-text');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
btnPress.addEventListener('press', (e) => {
removeBg.style.backgroundColor = 'rgba(0,0,0,0)';
pressText.innerText = "";
})
const btnPress = document.querySelector('.btn-press');
const removeBg = document.querySelector('#remove-bg');
const pressText = document.querySelector('#press-text');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
btnPress.addEventListener('press', (e) => {
removeBg.style.backgroundColor = 'rgba(0,0,0,0)';
pressText.innerText = "";
})
Press duration
Touch event press with custom duration.
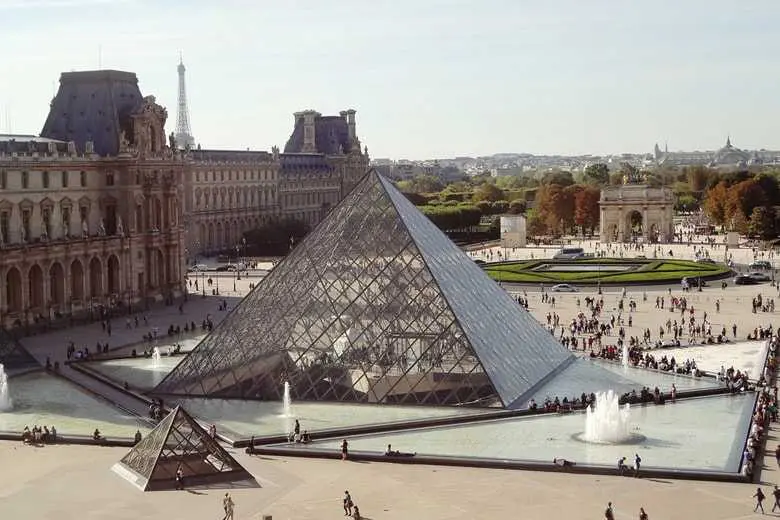
Hold the button to remove the mask from the image with 5 seconds
<div>
<div class="bg-image">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" />
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)" id="bg-press-duration">
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0" id="press-duration-text">Hold the button to remove the mask from the image with 5 seconds</p>
</div>
</div>
</div>
<div class="my-3">
<button
type="button"
class="btn btn-primary btn-press-duration"
data-mdb-ripple-init
data-mdb-touch-init
data-mdb-event="press"
data-mdb-time="5000">
Tap & hold to show image
</button>
</div>
</div>
import { Ripple, Touch, initMDB } from "mdb-ui-kit";
initMDB({ Ripple, Touch });
const btnPressDuration = document.querySelector('.btn-press-duration');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
}
const bgPressDuration = document.querySelector('#bg-press-duration');
const pressDurationText = document.querySelector('#press-duration-text');
btnPressDuration.addEventListener('press', () => {
bgPressDuration.style.backgroundColor = 'rgba(0,0,0,0)';
pressDurationText.innerText = "";
});
const btnPressDuration = document.querySelector('.btn-press-duration');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
}
const bgPressDuration = document.querySelector('#bg-press-duration');
const pressDurationText = document.querySelector('#press-duration-text');
btnPressDuration.addEventListener('press', () => {
bgPressDuration.style.backgroundColor = 'rgba(0,0,0,0)';
pressDurationText.innerText = "";
});
Tap
The callback on tap event is called with an object containing the origin field - the x and y coordinates of the user's touch.
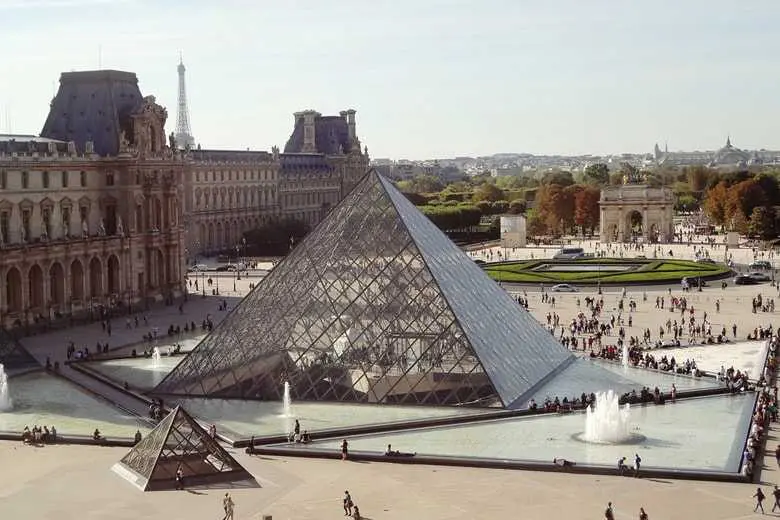
Tap button to change a color
<div>
<div class="bg-image">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" />
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)" id="bg-tap">
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0">Tap button to change a color</p>
</div>
</div>
</div>
<div class="my-3">
<button
type="button"
class="btn btn-primary btn-tap"
data-mdb-ripple-init
data-mdb-touch-init
data-mdb-event="tap">
Tap to change a color
</button>
</div>
</div>
import { Ripple, Touch, initMDB } from "mdb-ui-kit";
initMDB({ Ripple, Touch });
const btnTap = document.querySelector('.btn-tap');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const bgTap = document.querySelector('#bg-tap');
btnTap.addEventListener('tap', (e) => {
bgTap.style.backgroundColor = colorGen();
});
const btnTap = document.querySelector('.btn-tap');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const bgTap = document.querySelector('#bg-tap');
btnTap.addEventListener('tap', (e) => {
bgTap.style.backgroundColor = colorGen();
});
Double Tap
Set default taps to touch event.
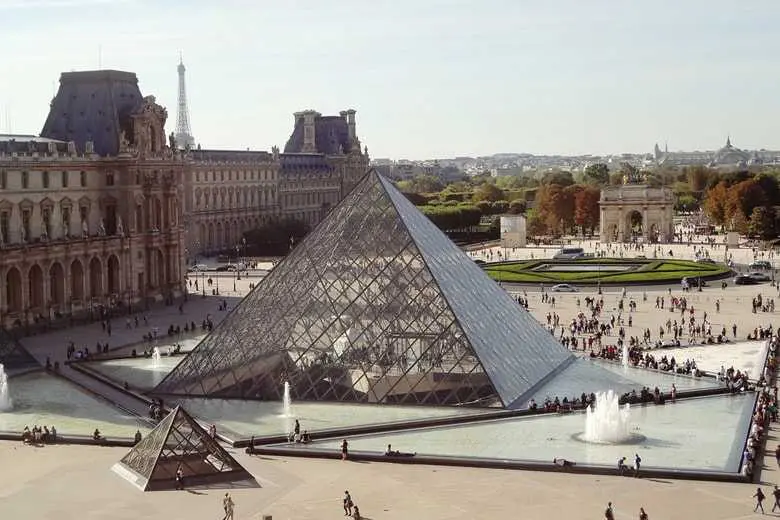
Change background color with 2 taps
<div>
<div class="bg-image">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" />
<div class="mask" style="background-color: rgba(0, 0, 0, 0.6)" id="bg-double-tap">
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0">Change background color with 2 taps</p>
</div>
</div>
</div>
<div class="my-3">
<button
type="button"
class="btn btn-primary btn-double-tap"
data-mdb-ripple-init
data-mdb-touch-init
data-mdb-event="tap"
data-mdb-taps="2">
Tap button twice
</button>
</div>
</div>
import { Ripple, Touch, initMDB } from "mdb-ui-kit";
initMDB({ Ripple, Touch });
const btnDoubleTap = document.querySelector('.btn-double-tap');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const doubleTapBg = document.querySelector('#bg-double-tap')
btnDoubleTap.addEventListener('tap', (e) => {
doubleTapBg.style.backgroundColor = colorGen();
});
const btnDoubleTap = document.querySelector('.btn-double-tap');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const doubleTapBg = document.querySelector('#bg-double-tap')
btnDoubleTap.addEventListener('tap', (e) => {
doubleTapBg.style.backgroundColor = colorGen();
});
Pan
The pan event is useful for dragging elements. Each time the user moves a finger on the element to which the directive is attached, the given method is being called with an argument consisting of two keys: x and y (representing horizontal and vertical translation).
<div>
<div class="bg-image" id="pan">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp"
class="img-fluid"
id="img-pan"
data-mdb-touch-init
data-mdb-event="pan"
/>
</div>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const panEl = document.querySelector("#pan");
const imgPan = document.querySelector('#img-pan')
let moveElement = {
x: null,
y: null
};
panEl.addEventListener('pan', (e) => {
moveElement = {
x: moveElement.x + e.x,
y: moveElement.y + e.y,
};
imgPan.style.transform = `translate(${moveElement.x}px, ${moveElement.y}px)`
});
const panEl = document.querySelector("#pan");
const imgPan = document.querySelector('#img-pan')
let moveElement = {
x: null,
y: null
};
panEl.addEventListener('pan', (e) => {
moveElement = {
x: moveElement.x + e.x,
y: moveElement.y + e.y,
};
imgPan.style.transform = `translate(${moveElement.x}px, ${moveElement.y}px)`
});
Pan Left
Pan with only left direction.
<div>
<div class="bg-image" id="pan-left">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp"
class="img-fluid"
id="img-pan-left"
data-mdb-touch-init
data-mdb-event="pan"
data-mdb-direction="left"
/>
</div>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const panLeftEl = document.querySelector('#pan-left');
const imgPanLeft = document.querySelector('#img-pan-left');
let moveElementLeft = {
x: null,
};
panLeftEl.addEventListener('panleft', (e) => {
moveElementLeft = {
x: moveElementLeft.x + e.x,
};
imgPanLeft.style.transform = `translate(${moveElementLeft.x}px, 0px)`;
});
const panLeftEl = document.querySelector('#pan-left');
const imgPanLeft = document.querySelector('#img-pan-left');
let moveElementLeft = {
x: null,
};
panLeftEl.addEventListener('panleft', (e) => {
moveElementLeft = {
x: moveElementLeft.x + e.x,
};
imgPanLeft.style.transform = `translate(${moveElementLeft.x}px, 0px)`;
});
Pan Right
Pan with only right direction.
<div>
<div class="bg-image" id="pan-right">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp"
class="img-fluid"
id="img-pan-right"
data-mdb-touch-init
data-mdb-event="pan"
data-mdb-direction="right"
/>
</div>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const panRightEl = document.querySelector('#pan-right');
const imgPanRight = document.querySelector('#img-pan-right');
let moveElementRight = {
x: null,
};
panRightEl.addEventListener('panright', (e) => {
moveElementRight = {
x: moveElementRight.x + e.x,
};
imgPanRight.style.transform = `translate(${moveElementRight.x}px, 0px)`;
});
const panRightEl = document.querySelector('#pan-right');
const imgPanRight = document.querySelector('#img-pan-right');
let moveElementRight = {
x: null,
};
panRightEl.addEventListener('panright', (e) => {
moveElementRight = {
x: moveElementRight.x + e.x,
};
imgPanRight.style.transform = `translate(${moveElementRight.x}px, 0px)`;
});
Pan Up/Down
Pan with only up/down direction.
<div>
<div class="bg-image" id="pan-up-down">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" id="img-pan-up-down"/>
</div>
</div>
import { Touch } from "mdb-ui-kit";
const panUpDown = document.querySelector('#pan-up-down');
const imgPanUpDown = document.querySelector('#img-pan-up-down');
const touchDown = new Touch(panUpDown, 'pan', { direction: 'down' });
const touchUp = new Touch(panUpDown, 'pan', { direction: 'up' });
let moveElementUpDown = {
y: null
};
panUpDown.addEventListener('panup', (e) => {
moveElementUpDown = {
y: moveElementUpDown.y + e.y,
};
imgPanUpDown.style.transform = `translate(0px, ${moveElementUpDown.y}px)`;
});
panUpDown.addEventListener('pandown', (e) => {
moveElementUpDown = {
y: moveElementUpDown.y + e.y,
};
imgPanUpDown.style.transform = `translate(0px, ${moveElementUpDown.y}px)`;
});
const panUpDown = document.querySelector('#pan-up-down');
const imgPanUpDown =document.querySelector('#img-pan-up-down');
const touchDown = new mdb.Touch(panUpDown, 'pan', { direction: 'down' });
const touchUp = new mdb.Touch(panUpDown, 'pan', { direction: 'up' });
let moveElementUpDown = {
y: null
};
panUpDown.addEventListener('panup', (e) => {
moveElementUpDown = {
y: moveElementUpDown.y + e.y,
};
imgPanUpDown.style.transform = `translate(0px, ${moveElementUpDown.y}px)`;
});
panUpDown.addEventListener('pandown', (e) => {
moveElementUpDown = {
y: moveElementUpDown.y + e.y,
};
imgPanUpDown.style.transform = `translate(0px, ${moveElementUpDown.y}px)`;
});
Pinch
The pinch event calls the given method with an object containing two keys - ratio and origin. The first one is the ratio of the distance between user's fingers on touchend to the same distance on touchstart - it's particularly useful for scaling images. The second one, similarly as in doubleTap event, is a pair of coordinates indicating the middle point between the user's fingers.
<div class="bg-image" id="div-pinch">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp"
class="img-fluid"
id="pinch"
data-mdb-touch-init
data-mdb-event="pinch"
/>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const pinchEl = document.querySelector("#pinch");
pinchEl.addEventListener('pinch', (e) => {
e.target.style.transform = `scale(${e.ratio})`;
e.target.style.transformOrigin = `translate(${e.origin.x}px, ${e.origin.y}px,)`
});
const pinchEl = document.querySelector("#pinch");
pinchEl.addEventListener('pinch', (e) => {
e.target.style.transform = `scale(${e.ratio})`;
e.target.style.transformOrigin = `translate(${e.origin.x}px, ${e.origin.y}px,)`
});
Swipe Left/Right
The swipe event comes with several modifiers (left, right, up, down). Each modifier will ensure that event will fire only on swipe in that particular direction. If the directive is used without any modifier, the callback method will be called each time the swiping occurs, and the string indicating the direction will be passed as an argument.
The following example demonstrates usage with 'left' and 'right' modifiers.
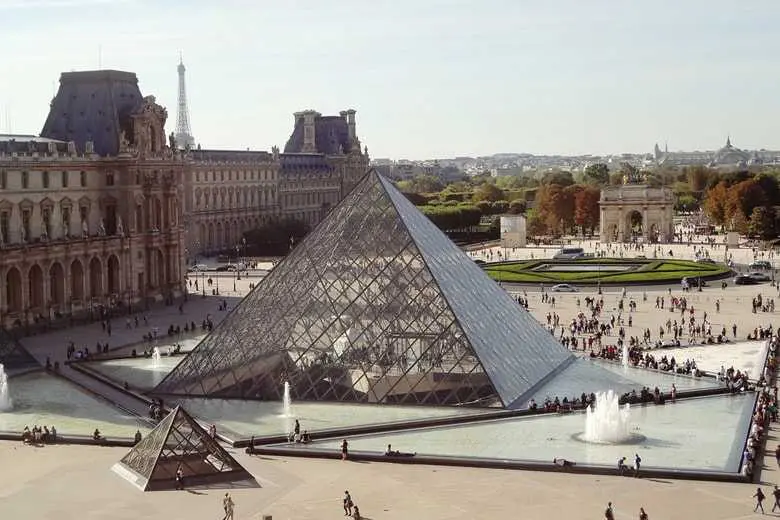
Swipe Left-Right to change a color
<div class="bg-image">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" />
<div
class="mask"
style="background-color: rgba(0, 0, 0, 0.6)"
id="swipe-left-right"
data-mdb-touch-init
data-mdb-event="swipe"
data-mdb-threshold="100"
>
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0">Swipe Left-Right to change a color</p>
</div>
</div>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const swipeLeftRight = document.querySelector('#swipe-left-right')
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
swipeLeftRight.addEventListener('swipeleft', (e) => {
e.target.style.backgroundColor = colorGen();
});
swipeLeftRight.addEventListener('swiperight', (e) => {
e.target.style.backgroundColor = colorGen();
});
const swipeLeftRight = document.querySelector('#swipe-left-right')
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
swipeLeftRight.addEventListener('swipeleft', (e) => {
e.target.style.backgroundColor = colorGen();
});
swipeLeftRight.addEventListener('swiperight', (e) => {
e.target.style.backgroundColor = colorGen();
});
Swipe Up/Down
The following example demonstrates usage with 'up' and 'down' modifiers.
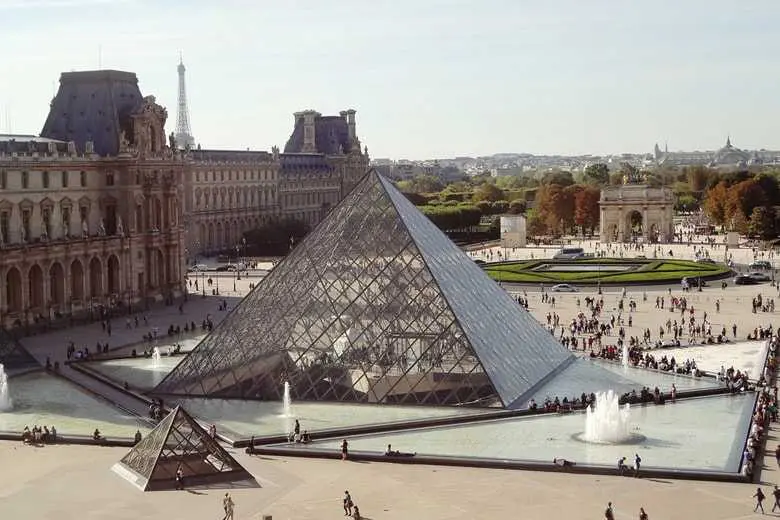
Swipe Up-Down to change a color
<div class="bg-image">
<img src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp" class="img-fluid" />
<div
class="mask"
style="background-color: rgba(0, 0, 0, 0.6);"
id="swipe-up-down"
data-mdb-touch-init
data-mdb-event="swipe"
data-mdb-threshold="120"
>
<div class="d-flex justify-content-center align-items-center h-100">
<p class="text-white mb-0">Swipe Up-Down to change a color</p>
</div>
</div>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const swipeUpDown = document.querySelector('#swipe-up-down')
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
swipeUpDown.addEventListener('swipeup', (e) => {
e.target.style.backgroundColor = colorGen();
});
swipeUpDown.addEventListener('swipedown', (e) => {
e.target.style.backgroundColor = colorGen();
});
const swipeUpDown = document.querySelector('#swipe-up-down')
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
swipeUpDown.addEventListener('swipeup', (e) => {
e.target.style.backgroundColor = colorGen();
});
swipeUpDown.addEventListener('swipedown', (e) => {
e.target.style.backgroundColor = colorGen();
});
Rotate
This example shows example with rotate.
<div class="bg-image">
<img
src="https://mdbcdn.b-cdn.net/img/new/standard/city/053.webp"
class="img-fluid"
id="rotate"
data-mdb-touch-init
data-mdb-event="rotate"
/>
</div>
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
const rotate = document.querySelector('#rotate');
rotate.addEventListener('rotate', (e) => {
rotate.style.transform = `rotate(${e.currentAngle}turn)`;
})
const rotate = document.querySelector('#rotate');
rotate.addEventListener('rotate', (e) => {
rotate.style.transform = `rotate(${e.currentAngle}turn)`;
})
Touch - API
Import
Importing components depends on how your application works. If you intend to use the MDBootstrap ES
format, you must
first import the component and then initialize it with the initMDB
method. If you are going to use the UMD
format,
just import the mdb-ui-kit
package.
import { Touch, initMDB } from "mdb-ui-kit";
initMDB({ Touch });
import 'mdb-ui-kit';
Usage
Via data attributes
Using the Touch method doesn't require any additional JavaScript code - simply add
data-mdb-touch-init
attribute to
element
and use other data attributes to set all options.
For ES
format, you must first import and call the initMDB
method.
<button
type="button"
class="btn btn-primary btn-press"
data-mdb-ripple-init
data-mdb-touch-init
data-mdb-event="press"
data-mdb-time="2000">
Tap & hold to show image
</button>
Via JavaScript
const myTouch = new Touch(document.getElementById('id'), event, options)
const myTouch = new mdb.Touch(document.getElementById('id'), event, options)
Via jQuery
Note: By default, MDB does not include jQuery and you have to add it to the project on your own.
$(document).ready(() => {
$('.example-class').touch(event, options);
});
Options
Options can be passed via data attributes or JavaScript. For data attributes, append the option name to
data-mdb-
, as in data-mdb-time="250"
.
Tap
Name | Type | Default | Description |
---|---|---|---|
interval
|
number | 500 |
Set interval to tap |
time
|
number | 250 |
Set delays time to tap event |
taps
|
number | 1 |
Set default value of number for taps |
pointers
|
number | 1 |
Set default value of number for pointers |
Press
Name | Type | Default | Description |
---|---|---|---|
time
|
number | 250 |
Set time delays to take tap |
pointers
|
number | 1 |
Set default value of number for pointers |
Swipe
Name | Type | Default | Description |
---|---|---|---|
threshold
|
number | 10 |
Adjust the time interval between event triggers |
direction
|
string | all |
Set direction to swipe. Available options: all, right, left, top, up. |
Rotate
Name | Type | Default | Description |
---|---|---|---|
angle
|
number | 0 |
Set started angle to rotate. |
pointers
|
number | 2 |
Set default value of number for pointers |
Pan
Name | Type | Default | Description |
---|---|---|---|
threshold
|
number | 20 |
Adjust the time interval between event triggers |
direction
|
string | all |
Set direction to pan. Available options: all, right, left, top, up. |
pointers
|
number | 1 |
Set default value of number for pointers |
Pinch
Name | Type | Default | Description |
---|---|---|---|
pointers
|
number | 2 |
Option for tap event, set duration to how long it takes to take a tap
|
threshold
|
number | 10 |
Adjust the time interval between event triggers |
Methods
Name | Description | Example |
---|---|---|
dispose
|
Destroy component with this method |
element.dispose()
|
getInstance
|
Static method which allows you to get the touch instance associated to a DOM element. |
Touch.getInstance(element)
|
getOrCreateInstance
|
Static method which returns the touch instance associated to a DOM element or create a new one in case it wasn't initialized. |
Touch.getOrCreateInstance(element)
|
const element = document.getElementById('element-id');
const myTouch = new Touch(element, 'tap');
myTouch.dispose();
const element = document.getElementById('element-id');
const myTouch = new mdb.Touch(element, 'tap');
myTouch.dispose();
Events
Name | Description |
---|---|
tap
|
This event fires tap touch funcionality. |
press
|
This event fires press touch funcionality. |
pan
|
This event fires pan touch funcionality. |
pinch
|
This event fires pinch touch funcionality. |
swipe
|
This event fires swipe touch funcionality. |
rotate
|
This event fires rotate touch funcionality. |
const element = document.getElementById('element-id');
element.addEventListener('rotate', (e) => {
// do something...
})