Position
Bootstrap 5 Position
Use these helpers for quickly configuring the position of an element.
Basic examples
Fixed top
Position an element at the top of the viewport, from edge to edge. Be sure you understand the ramifications of fixed position in your project, as it might require additional CSS adjustments.
<div class="fixed-top">...</div>
Fixed bottom
Position an element at the bottom of the viewport, from edge to edge. Be sure you understand the ramifications of fixed position in your project, as it might require additional CSS adjustments.
<div class="fixed-bottom">...</div>
Sticky top
Position an element at the top of the viewport, from edge to edge, but only after you scroll
past it. The .sticky-top
utility uses CSS’s position: sticky
, which
isn’t fully supported in all browsers.
<div class="sticky-top">...</div>
Responsive sticky top
Responsive variations also exist for .sticky-top
utility.
<div class="sticky-sm-top">Stick to the top on viewports sized SM (small) or wider</div>
<div class="sticky-md-top">Stick to the top on viewports sized MD (medium) or wider</div>
<div class="sticky-lg-top">Stick to the top on viewports sized LG (large) or wider</div>
<div class="sticky-xl-top">Stick to the top on viewports sized XL (extra-large) or wider</div>
Position values
Quick positioning classes are available, though they are not responsive.
<div class="position-static">...</div>
<div class="position-relative">...</div>
<div class="position-absolute">...</div>
<div class="position-fixed">...</div>
<div class="position-sticky">...</div>
Arrange elements
Arrange elements easily with the edge positioning utilities. The format is
{property}-{position}
.
Where property is one of:
top
- for the verticaltop
positionleft
- for the horizontalleft
positionbottom
- for the verticalbottom
positionright
- for the horizontalright
position
Where position is one of:
0
- for0
edge position50
- for50%
edge position100
- for100%
edge position
You can add more position values by adding entries to the $position-values
Sass
map variable.
<div class="position-relative position-relative-example">
<div class="position-absolute top-0 start-0"></div>
<div class="position-absolute top-0 end-0"></div>
<div class="position-absolute top-50 start-50"></div>
<div class="position-absolute bottom-50 end-50"></div>
<div class="position-absolute bottom-0 start-0"></div>
<div class="position-absolute bottom-0 end-0"></div>
</div>
.position-relative-example {
height: 200px;
width: 100%;
background-color: var(--mdb-tertiary-bg);
}
.position-relative-example div {
width: 2em;
height: 2em;
background-color: var(--mdb-body-color);
border-radius: .25rem;
}
Center elements
In addition, you can also center the elements with the transform utility class
.translate-middle
.
This class applies the transformations translateX(-50%)
and
translateY(-50%)
to the element which, in combination with the edge positioning
utilities, allows you to absolute center an element.
<div class="position-relative position-relative-example">
<div class="position-absolute top-0 start-0 translate-middle"></div>
<div class="position-absolute top-0 start-50 translate-middle"></div>
<div class="position-absolute top-0 start-100 translate-middle"></div>
<div class="position-absolute top-50 start-0 translate-middle"></div>
<div class="position-absolute top-50 start-50 translate-middle"></div>
<div class="position-absolute top-50 start-100 translate-middle"></div>
<div class="position-absolute top-100 start-0 translate-middle"></div>
<div class="position-absolute top-100 start-50 translate-middle"></div>
<div class="position-absolute top-100 start-100 translate-middle"></div>
</div>
.position-relative-example {
height: 200px;
width: 100%;
background-color: var(--mdb-tertiary-bg);
}
.position-relative-example div {
width: 2em;
height: 2em;
background-color: var(--mdb-body-color);
border-radius: .25rem;
}
Additional examples
Here are some real life examples of these classes:
<button type="button" class="btn btn-primary position-relative" data-mdb-ripple-init data-mdb-ripple-unbound="true">
Mails
<span class="position-absolute top-0 start-100 translate-middle badge rounded-pill bg-secondary">
+99 <span class="visually-hidden">unread messages</span>
</span>
</button>
<button type="button" class="btn btn-dark position-relative" data-mdb-ripple-init data-mdb-ripple-unbound="true">
Marker
<svg width="1em" height="1em" viewBox="0 0 16 16"
class="position-absolute top-100 start-50 translate-middle bi bi-caret-down-fill" fill="#212529"
xmlns="http://www.w3.org/2000/svg">
<path
d="M7.247 11.14L2.451 5.658C1.885 5.013 2.345 4 3.204 4h9.592a1 1 0 0 1 .753 1.659l-4.796 5.48a1 1 0 0 1-1.506 0z" />
</svg>
</button>
<button type="button" class="btn btn-primary position-relative" data-mdb-ripple-init data-mdb-ripple-unbound="true">
Alerts
<span
class="position-absolute top-0 start-100 translate-middle badge border border-light rounded-circle bg-danger p-2">
<span class="visually-hidden">unread messages</span>
</span>
</button>
// Initialization for ES Users
import { Ripple, initMDB } from "mdb-ui-kit";
initMDB({ Ripple });
You can use these classes with existing components to create new ones. Remember that you can
extend its functionality by adding entries to the $position-values
variable.
<div class="position-relative" style="width: 100%">
<div class="progress" style="height: 1px">
<div class="progress-bar" role="progressbar" style="width: 50%" aria-valuenow="25" aria-valuemin="0"
aria-valuemax="100"></div>
</div>
<button type="button" data-mdb-ripple-init
class="position-absolute top-0 start-0 translate-middle btn btn-sm btn-primary rounded-pill"
style="width: 3rem; height: 3rem">
1
</button>
<button type="button" data-mdb-ripple-init
class="position-absolute top-0 start-50 translate-middle btn btn-sm btn-primary rounded-pill"
style="width: 3rem; height: 3rem">
2
</button>
<button type="button" data-mdb-ripple-init
class="position-absolute top-0 start-100 translate-middle btn btn-sm btn-secondary rounded-pill"
style="width: 3rem; height: 3rem">
3
</button>
</div>
// Initialization for ES Users
import { Ripple, initMDB } from "mdb-ui-kit";
initMDB({ Ripple });
Object Fit
It's an utility for controlling how the content of the replaced element is resized.
Resize the content of an item to cover its container with .object-cover
.
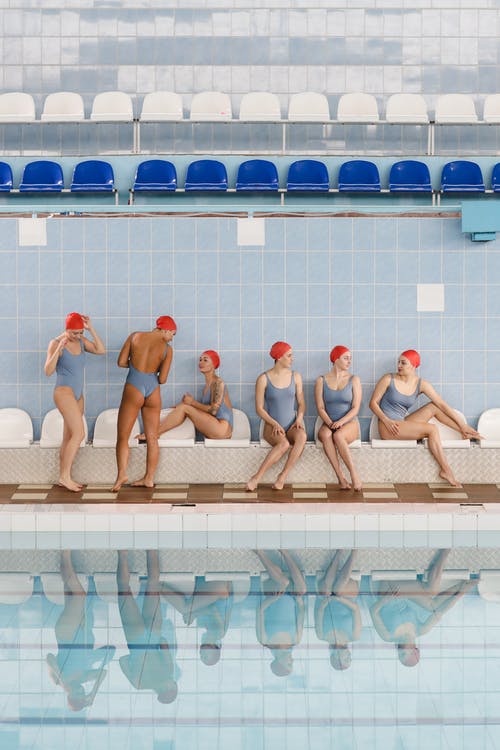
object-fit: cover;
<img
class="object-cover w-100"
style="height: 200px"
src="https://mdbootstrap.com/img/new/blog/2.jpeg"
alt=""
/>
Object Position
These are tools for controlling how the content of the replaced item should be placed in its container.
To specify how the contents of the replaced element should be placed in its container, use classes .object-top
, .object-center
or .object-bottom
.
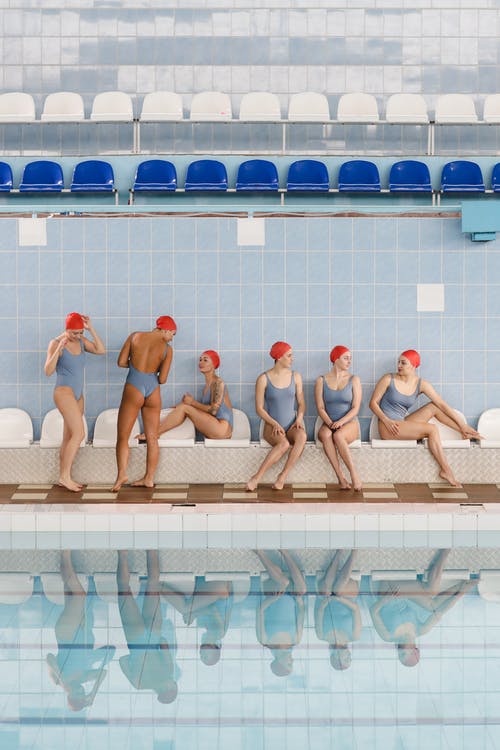
object-position: top;
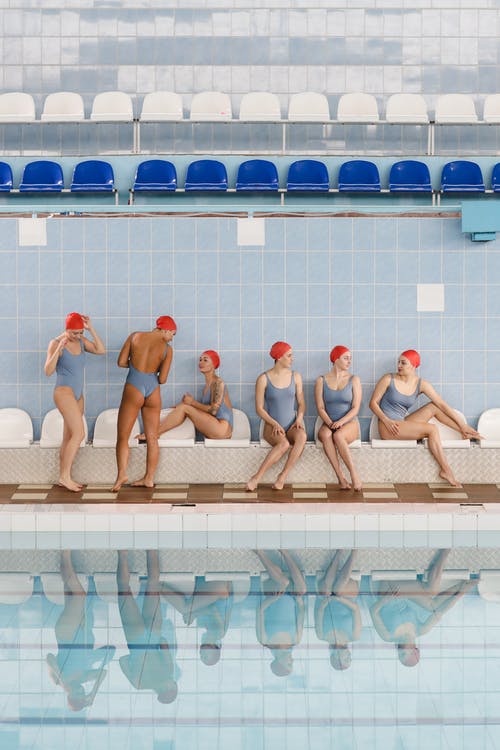
object-position: center;
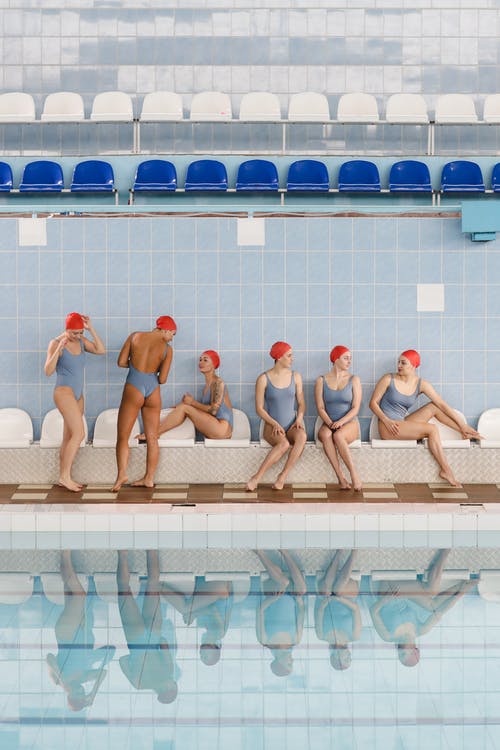
object-position: bottom;
<img
class="object-cover object-top w-100"
style="height: 250px;"
src="https://mdbootstrap.com/img/new/blog/2.jpeg"
alt=""
/>
<img
class="object-cover object-center w-100"
style="height: 250px;"
src="https://mdbootstrap.com/img/new/blog/2.jpeg"
alt=""
/>
<img
class="object-cover object-bottom w-100"
style="height: 250px;"
src="https://mdbootstrap.com/img/new/blog/2.jpeg"
alt=""
/>