Free UI/UX design course
Learn how to create exceptional designs by reading my new tutorial.
Start learningGrid system
Bootstrap grid is a powerful system for building mobile-first websites. It uses a series of containers, rows, and columns to layout and align content. It’s built with flexbox and is fully responsive.
Some people even think that grid is the most important reason to use Bootstrap, so in this lesson, we will get to know this incredibly useful tool in depth.
Container
Bootstrap grid needs a container to work properly. We learned about containers in the previous lesson, so we won't dwell on them here.
In our current project, we have already added a container and it looks like this:
<div class="container" style="height: 500px; background-color: red">
</div>
And this is what the container should look like when rendered in our browser:
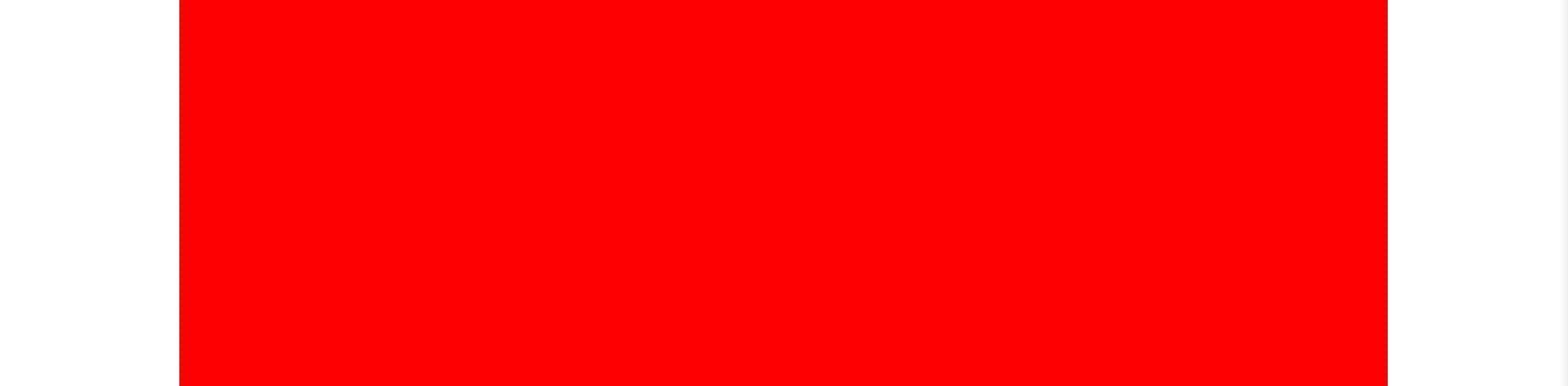
Row
Next, we need a row.
Rows are wrappers for columns. If you want to split your layout
horizontally, use .row
. Let's add 2 rows to our container:
<div class="container" style="height: 500px; background-color: red">
<div class="row" style="background-color: blue;">
I am a first row
</div>
<div class="row" style="background-color: lightblue;">
I am a second row
</div>
</div>
After saving the file and refreshing your browser, you should see 2 new rows.

Columns
Then it's time for the columns. Bootstrap grid allows you to add up to 12 columns in one line. If you add more than 12, the excess column will jump to the next line.
<div class="container">
<div class="row">
<div class="col">
1
</div>
<div class="col">
2
</div>
<div class="col">
3
</div>
<div class="col">
4
</div>
<div class="col">
5
</div>
<div class="col">
6
</div>
<div class="col">
7
</div>
<div class="col">
8
</div>
<div class="col">
9
</div>
<div class="col">
10
</div>
<div class="col">
11
</div>
<div class="col">
12
</div>
</div>
</div>
Columns are incredibly flexible. You can define how wide each column should be and how each of them should behave on different screen widths. Thanks to this, you can easily adjust your layout for both mobile devices and desktops.
For example, if you want to insert 2 columns of equal width, you can use the following code:
<div class="container">
<div class="row">
<div class="col-6">First column</div>
<div class="col-6">Second column</div>
</div>
</div>
As you can see, we've added the digits 6
to the col
class. This (col-6
class) means that we want each column to be 6 units wide.
You can freely set the width of the columns, just remember that the sum of their width units does not exceed 12.
col-6
+ col-6
together give 12 units, which means 2 identical columns.
If you would like the right column to be slightly larger than the left column, you can set it as follows:
<div class="container">
<div class="row">
<div class="col-4">First column</div>
<div class="col-8">Second column</div>
</div>
</div>
4 (col-4
) + 8 (col-8
) = 12
This way you've created a very typical layout with the main column on the right and the sidebar space on the left - that's exactly the scheme this tutorial uses!
However, there is one problem with the above example - no matter the screen size, our columns remain in the same aspect ratio.

The 4/8 ratio is very useful on large screens where there is plenty of space. However, on mobile devices, dividing a small screen additionally into 2 parts of 4 and 8 units is not acceptable. It would not be comfortable to use such a layout.
Here again, breakpoints come to the rescue. Do you remember the definition below from our previous lesson?
As in containers, we can also use breakpoints in columns and define from what width we want the column to extend to full width.
Taking the example from earlier, let's say we want both of our columns to be full-width on small and medium screens, and to change to a 4/8 ratio on large screens.
<div class="container">
<div class="row">
<div class="col-md-4">First column</div>
<div class="col-md-8">Second column</div>
</div>
</div>
All we have to do is add a breakpoint -md
(meaning "medium screen size") to the col
class, so that the Bootstrap grid knows that we want 4/8 columns ratio only on screens bigger than medium size, and on other screens (medium and small size) the columns should be stretched to full width.
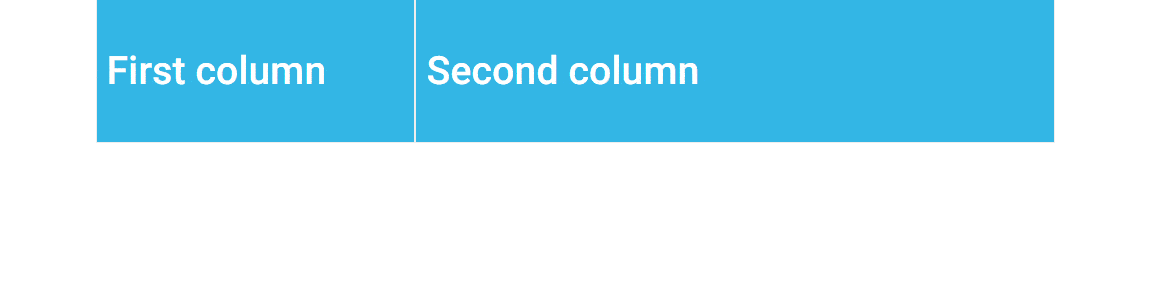
To sum up - by using class col-md-4
, we tell grid the following command:
- On screens smaller than 768px, I want this column to stretch to full width
- On screens larger than 768px, I want this column to be 4 units wide
Take a look at the table below and see what breakpoints you can use when creating a layout:
Breakpoint | Class infix | Dimensions |
---|---|---|
X-Small | None | 0–576px |
Small | sm |
≥576px |
Medium | md |
≥768px |
Large | lg |
≥992px |
Extra large | xl |
≥1200px |
Extra extra large | xxl |
≥1400px |
Okay, enough of this theory. Now let's work on some real life examples.
Three equal columns
Get three equal-width columns starting at desktops and scaling to large desktops. On mobile devices, tablets and below, the columns will automatically stack.
<div class="container">
<div class="row">
<div class="col-md-4">.col-md-4</div>
<div class="col-md-4">.col-md-4</div>
<div class="col-md-4">.col-md-4</div>
</div>
</div>
Three unequal columns
Get three columns starting at desktops and scaling to large desktops of various widths. Remember, grid columns should add up to twelve for a single horizontal block. More than that, and columns start stacking no matter the viewport.
<div class="container">
<div class="row">
<div class="col-md-3">.col-md-3</div>
<div class="col-md-6">.col-md-6</div>
<div class="col-md-3">.col-md-3</div>
</div>
</div>
Two columns with two nested columns
Nesting is easy—just put a row of columns within an existing column. This gives you two columns starting at desktops and scaling to large desktops, with another two (equal widths) within the larger column.
At mobile device sizes, tablets and down, these columns and their nested columns will stack.
<div class="container">
<!-- Outer row -->
<div class="row">
<div class="col-md-8">
.col-md-8
<!-- Inner row -->
<div class="row">
<div class="col-md-6">.col-md-6</div>
<div class="col-md-6">.col-md-6</div>
</div>
</div>
<div class="col-md-4">.col-md-4</div>
</div>
</div>
I think you now have a rough idea of how it works.
Bootstrap grid is a very advanced tool and at first its use can be overwhelming. If you feel that not everything is clear to you - that's completely normal and fine. It just takes practice. You will gain confidence and fluency over time.
It's been a long lesson, but don't hesitate to repeat it to yourself to consolidate your knowledge. If you want to practice on your own and have a look at more examples read the grid system documentation page or play with our grid generator.
If something is still unclear to you - feel free to hit me on twitter.
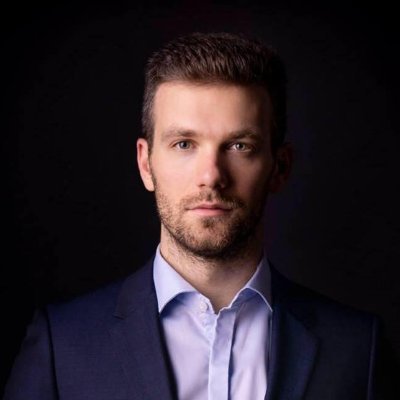
About author
Michal Szymanski
Co Founder at MDBootstrap / Listed in Forbes „30 under 30" / Open-source enthusiast / Dancer, nerd & book lover.
Author of hundreds of articles on programming, business, marketing and productivity. In the past, an educator working with troubled youth in orphanages and correctional facilities.