Free UI/UX design course
Learn how to create exceptional designs by reading my new tutorial.
Start learningOptimization
As you have probably noticed, Bootstrap and MDBootstrap are very powerful tools, with many features and components. This is, of course, their great advantage, but it rarely happens that you need all these wonderful things at once, in one project.
That is why optimization, i.e. reducing the weight of the package, is so important. This will make your code lighter and faster.
In this lesson, I'll show you how you can reduce the weight of an MDBootstrap package by up to 90% by automatically removing unused CSS classes and JavaScript code.
The PurgeCSS tool will help us in this important optimization task.
CSS optimization with PurgeCSS
PurgeCSS is great tool which analyzes content of your HTML, JavaScript and CSS files and reduces CSS by deleting unused selectors.
Normally, setting up and using PurgeCSS is quite complicated and cumbersome. However, with our Vite starter, it's child's play.
The Vite MDB launcher contains a ready-to-use configuration for PurgeCSS, so all we have to do is run the appropriate command in the terminal, and the rest of the magic will happen behind the scenes.
Create build
In order to generate a lightweight package, we need to create the so-called "build". They are simply compiled, minified and optimized files that any browser will be able to handle.
Unlike the source files we work on, the build contains unreadable for a human code, because at this stage it is supposed to be readable only by machines.
To create a build, run the following command in the terminal (make sure your terminal is set to the path where your Vite launcher project is located):
npm run build
After a few seconds, you will see information about what files were generated and where, and how much they weigh.
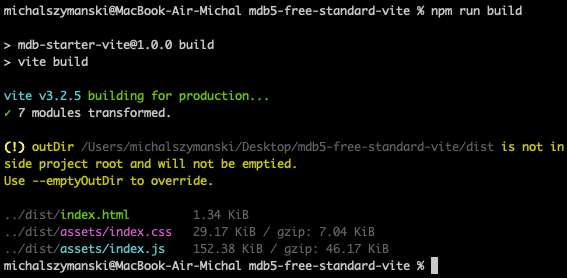
As you can see, they are in the /dist/
folder and in my case they weigh:
index.css
: 49.77 KiB (compared to 260 KiB of normal MDB CSS file)index.js
: 123.55 KiB (compared to 707 KiB of normal MDB JS file)
That's a huge improvement, don't you think?
But you can do even more. Let's also optimize our JavaScript
JavaScript optimization with ES modules
MDB UI Kit provides a ES format that allows, instead of importing the entire library, to choose only the modules that are used in the project. Thanks to this, we significantly reduce the amount of KB downloaded by the application. It can be reduced even several times!
For Example: Importing and using
only Input
component, the build weights close to
70 kB
. Using the same code but importing the entire library,
the build weights a little bit over 630 kB
.
The only thing you have to remember is to always init the component using
initMDB
method provided in MDB UI Kit
library
otherwise the components will not work properly.
How to do it?
All MDB components that use JavaScript have two tabs in our documentation - HTML and JavaScript. Take, for example, the Input component mentioned above:
(Click "SHOW CODE" button to see the code)
<div class="form-outline" data-mdb-input-init>
<input type="text" id="form12" class="form-control" />
<label class="form-label" for="form12">Example label</label>
</div>
// Initialization for ES Users
import { Input, initMDB } from "mdb-ui-kit";
initMDB({ Input });
If you do not use ES modules
, but only the default version of UMD
(we used it in all previous tutorials so far), you are only interested in the HTML tab.
However, if you are using ES modules, in addition to copying the HTML code, you also need to copy the JavaScript code to initialize the component.
// Initialization for ES Users
import { Input, initMDB } from "mdb-ui-kit";
initMDB({ Input });
At first glance, this may seem like an unnecessary additional step, but thanks to this, only the necessary components (i.e. only those that we have initiated) will be added to our final package.
Let's check it in practice. Add HTML code of the Input
to the index.html
file (I put it in the .container
to give it some margin)
<!-- Start your project here-->
<div class="container my-5">
<div class="form-outline" data-mdb-input-init>
<input type="text" id="form12" class="form-control" />
<label class="form-label" for="form12">Example label</label>
</div>
</div>
<!-- End your project here-->
Then copy the JavaScript code of the Input
and insert it into the src\js\main.js
file.
Note that there is already code in this file that imports the necessary files. Don't delete anything, just put the Input
code below.
import '../scss/styles.scss';
import * as mdb from 'mdb-ui-kit'; // lib
window.mdb = mdb;
// Initialization for ES Users
import { Input, initMDB } from 'mdb-ui-kit';
initMDB({ Input });
Then run the command again:
npm run build
A new build will be again created in the /dist
folder and its weight will be even smaller thanks to optimization using ES modules
.
If you are interested in additional optimization methods, please visit our optimization documentation page.
And as always - if you have any questions or if something doesn't work as it should - don't hesitate to hit me up on twitter 😉
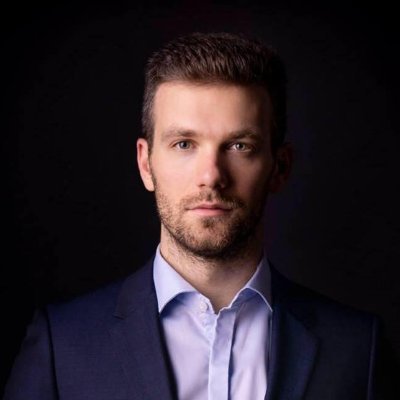
About author
Michal Szymanski
Co Founder at MDBootstrap / Listed in Forbes „30 under 30" / Open-source enthusiast / Dancer, nerd & book lover.
Author of hundreds of articles on programming, business, marketing and productivity. In the past, an educator working with troubled youth in orphanages and correctional facilities.